


Select all, invert selection and unselect functions implemented by jQuery_html/css_WEB-ITnose
Suitable for scenarios where batch operations are required after multiple selections on a web page (such as batch deletion, etc.). If there are any questions, I hope you can correct me. Thank you~~
HTML
We have a song list on our page that lists multiple lines of song names with matching checkboxes for users to choose, and there is a row of action buttons below the list
<ul id="list"> <li><label><input type="checkbox" value="1"> 1.时间都去哪儿了</label></li> <li><label><input type="checkbox" value="2"> 2.海阔天空</label></li> <li><label><input type="checkbox" value="3"> 3.真的爱你</label></li> <li><label><input type="checkbox" value="4"> 4.不再犹豫</label></li> <li><label><input type="checkbox" value="5"> 5.光辉岁月</label></li> <li><label><input type="checkbox" value="6"> 6.喜欢?</label></li> </ul> <input type="checkbox" id="all"> <input type="button" value="全选" class="btn" id="selectAll"> <input type="button" value="全不选" class="btn" id="unSelect"> <input type="button" value="反选" class="btn" id="reverse"> <input type="button" value="获得选中的所有值" class="btn" id="getValue">
Of course don’t forget to load the jQuery library file first:
jQuery Baidu CDN
<script src="http://libs.baidu.com/jquery/1.9.0/jquery.js"></script>
<script src="http://libs.baidu.com/jquery/2.0.0/jquery.min.js"></script>
Jquery支持的版本有:2.0.3, 2.0.2, 2.0.1, 2.0.0, 1.10.2, 1.10.1, 1.10.0, 1.9.1, 1.9.0, 1.8.3, 1.8.2, 1.8.1, 1.8.0, 1.7.2, 1.7.1, 1.7.0, 1.6.4, 1.6.3, 1.6.2, 1.6.1, 1.6.0, 1.5.2, 1.5.1, 1.5.0, 1.4.4, 1.4.3, 1.4.2, 1.4.1, 1.4.0, 1.3.2, 1.3.1, 1.3.0, 1.2.6, 1.2.3
jQuery
1. Select all or none. When the checkbox #all next to the Select All button #selectAll is checked, all options in the list are selected. Otherwise, when unchecked, all options in the list are unchecked.
$("#all").click(function(){ if(this.checked){ $("#list :checkbox").attr("checked", true); }else{ $("#list :checkbox").attr("checked", false); } });
2. Select all. When you click the Select All button #selectAll or check the checkbox #all next to the Select All button, all options in the list will be selected, including the checkbox next to Select All.
$("#selectAll").click(function () { $("#list :checkbox,#all").attr("checked", true); });
3. Select none. When the #unSelect button is clicked, all options in the list are unselected, including #all.
$("#unSelect").click(function () { $("#list :checkbox,#all").attr("checked", false); });
4. Counter selection. When you click the #reverse button, all selected options in the list become unselected, and all unselected options become selected. Of course, you should also pay attention to the status of #all.
$("#reverse").click(function () { $("#list :checkbox").each(function () { $(this).attr("checked", !$(this).attr("checked")); }); allchk(); });
The above code traverses the option list, then changes the checked attribute and calls the function allchk(). Don’t worry, we will introduce it later.
5. Get all selected values. If we want to interact with the background program, we must get the value of the selected item in the list. We traverse the array, store the value of the selected item in the array, and finally form a string separated by commas (,). Developers can get The corresponding operation is performed on this string.
$("#getValue").click(function(){ var valArr = new Array; $("#list :checkbox[checked]").each(function(i){ valArr[i] = $(this).val(); }); var vals = valArr.join(',');//转换为逗号隔开的字符串 alert(vals); });
In order to improve the selection option function, when we click an option in the list, if the checked item happens to meet all the selected conditions, #all should also respond accordingly becomes selected. Similarly, if all options are selected in advance, when an option is unchecked, #all will also become unselected accordingly.
//设置全选复选框 $("#list :checkbox").click(function(){ allchk(); });
The function allchk() is used to detect whether the all-select box #all should be selected or unselected. Please see the code.
function allchk(){ var chknum = $("#list :checkbox").size();//选项总个数 var chk = 0; $("#list :checkbox").each(function () { if($(this).attr("checked")==true){ chk++; } }); if(chknum==chk){//全选 $("#all").attr("checked",true); }else{//不全选 $("#all").attr("checked",false); } }
Summary
jQuery operates the checked and unchecked state of the checkbox very simply, use attr() to set the value of the "checked" attribute, true Unselected, false means unselected. During the entire process of selecting all and deselecting, pay attention to handling the selected state of the select all check box and obtaining the value of the selected option. Below I have compiled all the jQuery code together for your reference.
$(function () { //全选或全不选 $("#all").click(function(){ if(this.checked){ $("#list :checkbox").attr("checked", true); }else{ $("#list :checkbox").attr("checked", false); } }); //全选 $("#selectAll").click(function () { $("#list :checkbox,#all").attr("checked", true); }); //全不选 $("#unSelect").click(function () { $("#list :checkbox,#all").attr("checked", false); }); //反选 $("#reverse").click(function () { $("#list :checkbox").each(function () { $(this).attr("checked", !$(this).attr("checked")); }); allchk(); }); //设置全选复选框 $("#list :checkbox").click(function(){ allchk(); }); //获取选中选项的值 $("#getValue").click(function(){ var valArr = new Array; $("#list :checkbox[checked]").each(function(i){ valArr[i] = $(this).val(); }); var vals = valArr.join(','); alert(vals); }); }); function allchk(){ var chknum = $("#list :checkbox").size();//选项总个数 var chk = 0; $("#list :checkbox").each(function () { if($(this).attr("checked")==true){ chk++; } }); if(chknum==chk){//全选 $("#all").attr("checked",true); }else{//不全选 $("#all").attr("checked",false); } }
Examples of the select all, invert selection and unselect functions implemented by jQuery:
<!DOCTYPE HTML><html><head><meta charset="utf-8"><title>演示:jQuery实现的全选、反选和不选功能</title><style>.top_title{text-align:center;}.demo{width:520px; margin:40px auto 0 auto; min-height:250px;}ul li{line-height:30px; padding:4px 0; font-size:14px}.btn{overflow: hidden;display:inline-block;*display:inline;padding:4px 20px 4px;font-size:14px;line-height:18px;*line-height:20px;color:#fff;text-align:center;vertical-align:middle;cursor:pointer;background-color:#5bb75b;border:1px solid #cccccc;border-color:#e6e6e6 #e6e6e6 #bfbfbf;border-bottom-color:#b3b3b3;-webkit-border-radius:4px;-moz-border-radius:4px;border-radius:4px; margin-left:2px}</style><script src="http://libs.baidu.com/jquery/1.7.2/jquery.js"></script><script> $(function () { //全选或全不选 $("#all").click(function(){ if(this.checked){ $("#list :checkbox").attr("checked", true); }else{ $("#list :checkbox").attr("checked", false); } }); //全选 $("#selectAll").click(function () { $("#list :checkbox,#all").attr("checked", true); }); //全不选 $("#unSelect").click(function () { $("#list :checkbox,#all").attr("checked", false); }); //反选 $("#reverse").click(function () { $("#list :checkbox").each(function () { $(this).attr("checked", !$(this).attr("checked")); }); allchk(); }); //设置全选复选框 $("#list :checkbox").click(function(){ allchk(); }); //获取选中选项的值 $("#getValue").click(function(){ var valArr = new Array; $("#list :checkbox[checked]").each(function(i){ valArr[i] = $(this).val(); }); var vals = valArr.join(','); alert(vals); });}); function allchk(){ var chknum = $("#list :checkbox").size();//选项总个数 var chk = 0; $("#list :checkbox").each(function () { if($(this).attr("checked")==true){ chk++; } }); if(chknum==chk){//全选 $("#all").attr("checked",true); }else{//不全选 $("#all").attr("checked",false); }}</script> </head><body><div id="main"> <h2 id="a-href-http-www-cnblogs-com-phpfensi-p-html-jQuery实现的全选-反选和不选功能-a"><a href="http://www.cnblogs.com/phpfensi/p/3853337.html ">jQuery实现的全选、反选和不选功能</a></h2> <div class="demo"> <ul id="list"> <li><label><input type="checkbox" value="1"> 1.时间都去哪儿了</label></li> <li><label><input type="checkbox" value="2"> 2.海阔天空</label></li> <li><label><input type="checkbox" value="3"> 3.真的爱你</label></li> <li><label><input type="checkbox" value="4"> 4.不再犹豫</label></li> <li><label><input type="checkbox" value="5"> 5.光辉岁月</label></li> <li><label><input type="checkbox" value="6"> 6.喜欢?</label></li> </ul> <input type="checkbox" id="all"> <input type="button" value="全选" class="btn" id="selectAll"> <input type="button" value="全不选" class="btn" id="unSelect"> <input type="button" value="反选" class="btn" id="reverse"> <input type="button" value="获得选中的所有值" class="btn" id="getValue"> </div></div></body></html>

HTML is used to build websites with clear structure. 1) Use tags such as, and define the website structure. 2) Examples show the structure of blogs and e-commerce websites. 3) Avoid common mistakes such as incorrect label nesting. 4) Optimize performance by reducing HTTP requests and using semantic tags.

ToinsertanimageintoanHTMLpage,usethetagwithsrcandaltattributes.1)UsealttextforaccessibilityandSEO.2)Implementsrcsetforresponsiveimages.3)Applylazyloadingwithloading="lazy"tooptimizeperformance.4)OptimizeimagesusingtoolslikeImageOptimtoreduc

The core purpose of HTML is to enable the browser to understand and display web content. 1. HTML defines the web page structure and content through tags, such as, to, etc. 2. HTML5 enhances multimedia support and introduces and tags. 3.HTML provides form elements to support user interaction. 4. Optimizing HTML code can improve web page performance, such as reducing HTTP requests and compressing HTML.

HTMLtagsareessentialforwebdevelopmentastheystructureandenhancewebpages.1)Theydefinelayout,semantics,andinteractivity.2)SemantictagsimproveaccessibilityandSEO.3)Properuseoftagscanoptimizeperformanceandensurecross-browsercompatibility.

A consistent HTML encoding style is important because it improves the readability, maintainability and efficiency of the code. 1) Use lowercase tags and attributes, 2) Keep consistent indentation, 3) Select and stick to single or double quotes, 4) Avoid mixing different styles in projects, 5) Use automation tools such as Prettier or ESLint to ensure consistency in styles.
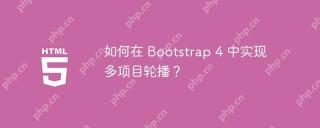
Solution to implement multi-project carousel in Bootstrap4 Implementing multi-project carousel in Bootstrap4 is not an easy task. Although Bootstrap...
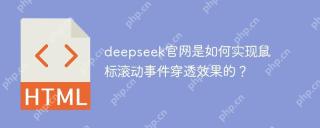
How to achieve the effect of mouse scrolling event penetration? When we browse the web, we often encounter some special interaction designs. For example, on deepseek official website, �...
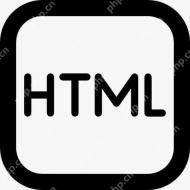
The default playback control style of HTML video cannot be modified directly through CSS. 1. Create custom controls using JavaScript. 2. Beautify these controls through CSS. 3. Consider compatibility, user experience and performance, using libraries such as Video.js or Plyr can simplify the process.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor
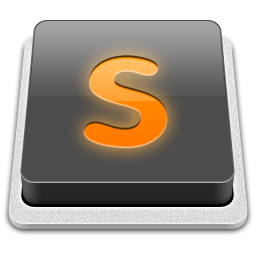
SublimeText3 Mac version
God-level code editing software (SublimeText3)

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
