Let’s first look at a simple document tree
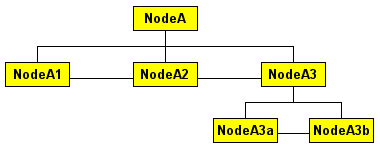
It is obvious that the top node of the tree is the NodeA node, and then it can be moved to the tree by specifying the appropriate node. At any point, you can better understand the relationship between the nodes of this tree by combining the following code:
NodeA.firstChild = NodeA1
NodeA.lastChild = NodeA3
NodeA.childNodes.length = 3
NodeA.childNodes[0] = NodeA1
NodeA.childNodes[1] = NodeA2
NodeA.childNodes[2] = NodeA3
NodeA1.parentNode = NodeA
NodeA1.nextSibling = NodeA2
NodeA3.prevSibling = NodeA2
NodeA3.nextSibling = null
NodeA.lastChild.firstChild = NodeA3a
NodeA3b.parentNode.parentNode = NodeA
DOM definition provides a node structure for operating a document object It provides practical methods such as performing object insertion, update, deletion, cloning, etc.
insertBefore()--Insert a new child node before the reference child node. If the reference child node is null, the new child node will be inserted as the last child node of the calling node.
replaceChild()--Use the specified newChild to replace oldChild in the childNodes collection; if the replacement is successful, oldChild is returned; if newChild is null, just delete oldChild.
removeChild()--Remove the node specified by removeChild from the node's ChildNodes collection. If the deletion is successful, the deleted child node is returned.
appendChild()--Adds a new node to the end of the childNodes collection, and returns the new node if successful.
cloneNode()--Create a new, copied node, and if the parameter passed in is true, the child node will also be copied. If the node is an element, the corresponding attributes will also be copied and the new node will be returned. .
In order to access or create a new node in a document tree, you can use the following methods:
getElementById()
getElementsByTagName()
createElement()
createAttribute( )
createTextNode()
Note: There is only one document object in a page. Except for getElementsByTagName(), other methods can only be called through document.methodName().
Look at the following example again:
This is a sample paragraph.
The results will be displayed" P", here are some explanations
document.documentElement - gives the page's HTML tag.
lastChild - gives the BODY tag.
firstChild - gives the first element in the BODY.
tagName - gives that element's tag name, "P" in this case.
Another:
This is a sample paragraph.
There is no big difference between this example and the above, there is just one more blank line, but in NS, a node will be automatically added to the blank line, so the return value is "undefined", while in IE it will be skipped. The empty line still points to the P tag.
More commonly used methods:
This is a sample paragraph.
...
alert(document.getElementById( "myParagraph").tagName);
With this method, you don't need to care where the node is in the document tree, but only need to ensure that its ID number is unique in the page.
The next way to access element nodes is document.getElementsByTagName(). Its return value is an array. For example, you can change the connection of the entire page through the following example:
var nodeList = document .getElementsByTagName("A");
for (var i = 0; i < nodeList.length; i )
nodeList[i].style.color = "#ff0000";
attributes and attributes
Attribute objects are related to elements, but are not considered part of the document tree, so attributes cannot be used as part of a collection of child nodes.
There are three ways to create new attributes for elements
1.
var attr = document.createAttribute("myAttribute");
attr.value = "myValue";
var el = document.getElementById("myParagraph");
el.setAttributeNode(attr);
2.
var el = document.getElementById("myParagraph");
el.setAttribute("myAttribute", "myValue");
3.
var el = document.getElementById("myParagraph");
el.myAttribute = "myValue";
You can define your own attributes in the html tag:
This is a sample paragraph.
...
alert(document.getElementById("myParagraph").getAttribute ("myAttribute"));
The return value will be "myValue". But please note that getAttribute must be used here, not AttributeName, because some browsers do not support custom attributes.
Attributes can also be easily removed from an element by using removeAttribute() or by pointing element.attributeName to a null value.
We can produce some dynamic effects through attributes:
Text in a paragraph element.
... code for the links ...
document.getElementById('sample1').setAttribute('align', 'left');
document.getElementById('sample1').setAttribute('align', 'right') ;
Another:
Text in a paragraph
element.
.. . code for the links ...
document.getElementById('sample2').style.textAlign = 'left';
document.getElementById('sample2').style.textAlign = 'right';
Similar to the above example, it shows that the attributes in style can be modified through elements, even in class. The only thing to mention is that textAlign evolved from text-align in style. There is a basic rule, That is, if the attribute in style appears - it will be removed in the dom and the subsequent letter will be changed to uppercase. Another point is that the above example can be used even if there is no style attribute in the element.
text nodes:
Let’s take a look at the example first:
This is the initial text.
... code for the links ...
document.getElementById('sample1').firstChild.nodeValue =
'Once upon a time...';
document.getElementById('sample1').firstChild.nodeValue =
'...in a galaxy far, far away';
First of all, text nodes do not have id attributes like elements, so they cannot be accessed directly through document.getElementById() or document.getElementsByTagName()
It may be clearer to look at the following structure:
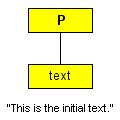
It can be seen that text can be read or set by document.getElementById('sample1').firstChild.nodeValue nodes value.
Another more complex example:
This is the initial text.
it The document structure of
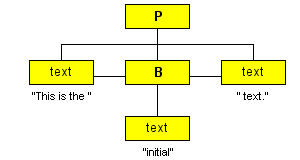
here is just changing "This is the"
and
initial text. will not change. Here you should see the difference between it and innerHTML. Of course you can also use it like this: document.getElementById('sample3').firstChild.nodeValue =
'< ;b>Once upon a time...';
document.getElementById('sample3').firstChild.nodeValue =
'...in a galaxy
far, far< /i> away';
The html codes in it will not be interpreted, and the browser will display them as ordinary text.
Create and delete text nodes:
var myTextNode = document.createTextNode("my text");
With the above code you can create a new text node, but it is not a document tree To display it on the page, it must become a child of a node in the document tree. Because
text nodes cannot have children, you cannot add it to a text node, and attribute does not belong to it. Part of the document tree, this path is not possible, now only elements nodes
are left. The following example shows how to add and delete a text node:
Initial text within a paragraph element.
... code to add a text node ...
var text = document.createTextNode(" new text " ( counter1));
var el = document.getElementById("sample1");
el.appendChild(text);
... code to remove the last child node ...
var el = document.getElementById("sample1");
if (el.hasChildNodes())
el.removeChild(el.lastChild);
It is very easy to add text. The above code creates a new text node and adds it through the appendChild() method. It is added to the end of the childNodes array, and a counter1 global variable is set, which is convenient for observing whether the return value of
hasChildNodes() is true or false; it is used to determine whether the current node still has children, so as to prevent it from being blocked when it has no children. An error occurs when calling removeChild().
Create element nodes
With the above foundation, it should be easier to understand. Let’s take a look at the following code first
This text is in a DIV element .
... code for the link ...
var paraEl, boldEl;
paraEl = document.createElement("p");
boldEl = document.createElement( "b");
paraEl.appendChild(document.createTextNode("This is a new paragraph with "));
boldEl.appendChild(document.createTextNode("bold"));
paraEl.appendChild (boldEl);
paraEl.appendChild(document.createTextNode(" text added to the DIV"));
document.getElementById("sample1").appendChild(paraEl);
You can also directly Set attributes for newly added element nodes. Both of the following are acceptable:
boldEl.style.color = "#ffff00";
paraEl.appendChild(boldEl);
or:
paraEl.appendChild( boldEl);
boldEl.style.color = "#ffff00";