JavaScript introductory tutorial (3) js object-oriented_basic knowledge
JavaScript 是使用“对象化编程”的,或者叫“面向对象编程”的。所谓“对象化编程”,意思是把 JavaScript 能涉及的范围划分成大大小小的对象,对象下面还继续划分对象直至非常详细为止,所有的编程都以对象为出发点,基于对象。小到一个变量,大到网页文档、窗口甚至屏幕,都是对象。这一章将“面向对象”讲述 JavaScript 的运行情况。
对象的基本知识
对象是可以从 JavaScript“势力范围”中划分出来的一小块,可以是一段文字、一幅图片、一个表单(Form)等等。每个对象有它自己的属性、方法和事件。对象的属性是反映该对象某些特定的性质的,例如:字符串的长度、图像的长宽、文字框(Textbox)里的文字等等;对象的方法能对该对象做一些事情,例如,表单的“提交”(Submit),窗口的“滚动”(Scrolling)等等;而对象的事件就能响应发生在对象上的事情,例如提交表单产生表单的“提交事件”,点击连接产生的“点击事件”。不是所有的对象都有以上三个性质,有些没有事件,有些只有属性。引用对象的任一“性质”用“.”这种方法。
基本对象
现在我们要复习以上学过的内容了——把一些数据类型用对象的角度重新学习一下。
Number “数字”对象。这个对象用得很少,作者就一次也没有见过。不过属于“Number”的对象,也就是“变量”就多了。
AttributesString String object. The simplest, quickest, most effective, and commonly used way to declare a string object is to assign it directly.
MAX_VALUE Usage: Number.MAX_VALUE; Returns the "maximum value".
MIN_VALUE Usage: Number.MIN_VALUE; Returns the "minimum value".
NaN Usage: Number.NaN or NaN; returns "NaN". "NaN" (not a number) was introduced very early on.
NEGATIVE_INFINITY Usage: Number.NEGATIVE_INFINITY; Return: negative infinity, a value smaller than the "minimum value".
POSITIVE_INFINITY Usage: Number.POSITIVE_INFINITY; Returns: positive infinity, a value greater than the "maximum value".
Method
toString() Usage:.toString(); Returns: a numerical value in the form of a string. For example: if a == 123; then a.toString() == '123'.
AttributesArray Array object. An array object is a collection of objects, and the objects inside can be of different types. Each member object of the array has a "subscript" used to indicate its position in the array (since it is a "position", it also starts from zero).
length Usage:.length; Returns the length of the string.
Method
charAt() Usage:.charAt( ); Returns the string at the A single character of bits. Note: One character in the string is at position 0, the second character is at position 1, and the last character is at position length - 1.
charCodeAt() Usage:.charCodeAt( ); Returns the ASCII code of a single character of the string located at the position.
fromCharCode() Usage: String.fromCharCode(a, b, c...); Returns a string in which the ASCII code of each character is composed of a, b, c.. . Wait to be sure.
indexOf() Usage:.indexOf( [, ]); This method starts from in the string object> (if is given, ignore the previous position), if found, return its position, if not found, return "- 1".All "positions" start from scratch.
lastIndexOf() Usage:.lastIndexOf( [, ]); similar to indexOf() , but start looking from the back.
split() Usage:.split( ); Returns an array that is separated from The determines the separation point and will not itself be included in the returned array. For example: '1&2&345&678'.split('&') returns array: 1,2,345,678. Regarding arrays, we will discuss them in a moment.
substring() Usage:.substring( [, ]); Returns the substring of the original string, the character A string is a segment of the original string from the position to the previous position of the position. - = Returns the length of the string (length). If is not specified or exceeds the string length, the substring is taken from the position to the end of the original string. If a string cannot be returned at the specified location, an empty string is returned.
substr() Usage:.substr( [, ]); Returns the substring of the original string, the character A string is a section of the original string starting from the position and having a length of . If is not specified or exceeds the string length, the substring is taken from the position to the end of the original string. If a string cannot be returned at the specified location, an empty string is returned.
toLowerCase() Usage:.toLowerCase(); returns a string in which all uppercase letters of the original string are changed to lowercase.
toUpperCase() Usage:.toUpperCase(); returns a string in which all lowercase letters of the original string are changed to uppercase.
How to define an array:
varThis defines an empty array. To add array elements in the future, use:= new Array();
Note that the square brackets here do not mean "can be omitted". The subscript of the array is expressed using square brackets. enclosed in parentheses.[ ] = ...;
If you want to initialize the data directly when defining the array, please use:
varFor example, var myArray = new Array( 1, 4.5, 'Hi'); defines an array myArray, the elements inside are: myArray[0] == 1; myArray[1] == 4.5; myArray[2] == 'Hi'.= new Array( , , ...);
However, if there is only one element in the element list, and this element is a positive integer, this will define an array containing
Note: JavaScript only has one-dimensional arrays! Never use the stupid method "Array(3,4)" to define a 4 x 5 two-dimensional array, or use the method "myArray[2,3]" to return the elements in a "two-dimensional array" . Any call of the form "myArray[...,3]" actually only returns "myArray[3]".To use multidimensional arrays, use this dummy method:
var myArray = new Array(new Array(), new Array(), new Array(), ...);In fact, this is a one-dimensional array, and each element in it is an array. When calling the elements of this "two-dimensional array": myArray[2][3] = ...;
AttributesMath A "math" object that provides mathematical calculations on data. The properties and methods mentioned below will not explain the "usage" in detail. When using them, please remember to use the format "Math.
length Usage:.length; Returns: the length of the array, that is, how many elements there are in the array. It is equal to the index of the last element in the array plus one. So, if you want to add an element, just: myArray[myArray.length] = ....
Method
join() Usage:.join( ); returns a string that The elements in the array are strung together and placed between elements with . This method does not affect the original contents of the array.
reverse() Usage:.reverse(); reverses the order of elements in the array. If you use this method on the array [1, 2, 3], it will make the array become: [3, 2, 1].
slice() Usage:.slice( [, ]); returns an array, which is a subset of the original array , begins with and ends with . If is not given, the subset is taken until the end of the original array.
sort() Usage:.sort([ ]); arranges the elements in the array in a certain order. If is not specified, alphabetical order is used. In this case, 80 is ranked higher than 9. If is specified, the sorting method specified by is sorted. are difficult to describe. Here we only introduce some useful to you.
Arrange numbers in ascending order:
function sortMethod(a, b) {Sort the numbers in descending order: put the above "a - b" should become "b - a".
return a - b;
}
myArray.sort(sortMethod);
For functions, please see below.
PropertiesDate Date object. This object can store any date from 0001 to 9999, and can be accurate to milliseconds (1/1000th of a second). Internally, a date object is an integer that is the number of milliseconds since midnight, January 1, 1970, to the date pointed to by the date object. If the date referred to is earlier than 1970, it is a negative number. All dates and times, if no time zone is specified, use the "UTC" (Universal Time) time zone, which is numerically the same as "GMT" (Greenwich Mean Time).
E Returns the constant e (2.718281828...).
LN2 Returns the natural logarithm of 2 (ln 2).
LN10 Returns the natural logarithm of 10 (ln 10).
LOG2E Returns the logarithm of e (log2e) with 2 as the low value.
LOG10E Returns the logarithm of e with 10 as the low (log10e).
PI Returns π (3.1415926535...).
SQRT1_2 Returns the square root of 1/2.
SQRT2 Returns the square root of 2.
Methods
abs(x) Returns the absolute value of x.
acos(x) Returns the inverse cosine of x (the cosine equals the angle of x), expressed in radians.
asin(x) Returns the arcsine of x.
atan(x) Returns the arctangent of x.
atan2(x, y) Returns the argument of the complex number corresponding to the point (x, y) in the complex plane, expressed in radians, and its value is between -π and π.
ceil(x) Returns the smallest integer greater than or equal to x.
cos(x) Returns the cosine of x.
exp(x) Returns e raised to the x power (ex).
floor(x) Returns the largest integer less than or equal to x.
log(x) Returns the natural logarithm of x (ln x).
max(a, b) Returns the larger number among a, b.
min(a, b) Returns the smaller number among a, b.
pow(n, m) Returns n raised to the m power (nm).
random() Returns a random number greater than 0 and less than 1.
round(x) Returns the rounded value of x.
sin(x) Returns the sine of x.
sqrt(x) Returns the square root of x.
tan(x) Returns the tangent of x.
Define a date object:
var d = new Date;This method makes d a date object and has an initial value: the current time. If you want to customize the initial value, you can use:
var d = new Date(99, 10, 1); //October 1, 1999etc. The best way is to strictly define time using the "method" introduced below.
var d = new Date('Oct 1, 1999'); //October 1, 1999
Methods
There are many methods like "g/set[UTC]XXX" below, which means there are both "getXXX" method and "setXXX" method. "Get" is to get a certain value, and "set" is to set a certain value. If there is a "UTC" letter, it means that the value obtained/set is based on UTC time; if there is no letter, it means that it is based on local time or the default time of the browsing period.
If there is no description, the usage format of the method is: "
Global object
The global object never appears. It can be said to be virtual. The purpose is to turn the global function into an "object" change". In the Microsoft JScript Language Reference, it is called the "Global object", but its methods and properties are never referenced with "Global.xxx" (and doing so will cause an error), but directly with "xxx".
Attribute
NaN I said it early.
Methods
eval() Operates the string enclosed in parentheses as a standard statement or expression.
isFinite() Returns true if the number in the brackets is "finite" (between Number.MIN_VALUE and Number.MAX_VALUE); otherwise returns false.
isNaN() Returns true if the value inside the brackets is "NaN" and false otherwise.
parseInt() Returns the value after converting the content in parentheses into an integer. If the brackets are a string, the numeric part at the beginning of the string is converted to an integer, if it starts with a letter, "NaN" is returned.
parseFloat() Returns the value after converting the string in brackets into a floating point number. The numeric part at the beginning of the string is converted into a floating point number. If it starts with a letter, "NaN" is returned.
toString() Usage:
FunctionDefinition of function
The so-called "function" has a return value An object or an object's methods.
Types of functions
Common functions include: constructor, such as Array(), which can construct an array; global function, which is a method in the global object; self Define functions; etc.
Custom function
Use the following statement to define a function:
function function name ([parameter set]) {where , the braces used after the function and at the end of the function cannot be omitted, even if the entire function has only one sentence.
...
[return[];]
...
}
Function names have the same naming rules as variable names, that is, they only contain letters, numbers, underscores, leading letters, and cannot repeat reserved words.
The parameter set is optional, but the parentheses must be present.
Parameters are the bridge that transmits information from the outside of the function to the inside of the function. For example, if you want to ask a function to return the cube of 3, you have to let the function know the value "3". At this time, there must be a Variables to receive values are called parameters.
The parameter set is a set of one or more parameters separated by commas, such as: a, b, c.
There are one or more lines of statements inside the function. These statements will not be executed immediately, but will only be executed when other programs call it. These statements may contain "return" statements. When executing a function, when a return statement is encountered, the function immediately stops execution and returns to the program that called it. If "return" is followed by
Within the function, parameters can be used directly as variables, and some new variables can be created using the var statement, but these variables cannot be called by procedures outside the function. To enable the information inside the function to be called externally, either use the "return" return value or use global variables.
Global variables The variables defined by the "var" statement at the "root" of the Script (not inside the function) are global variables, which can be called and changed anywhere in the entire process.
Example
function addAll(a, b, c) {This example creates A function called "addAll" is created, which has three parameters: a, b, c. Its function is to return the result of the addition of three numbers. Outside the function, use "var total = addAll(3, 4, 5);" to receive the return value of the function.
return a b c;
}
var total = addAll(3, 4, 5);
More often, functions have no return value. This kind of function is called "procedure" in some languages that emphasize strictness, such as "Sub" in Basic language and "procedure" in Pascal language.
Attributes
arguments An array reflecting the parameters specified when the external program calls the function. Usage: Call "arguments" directly inside the function.
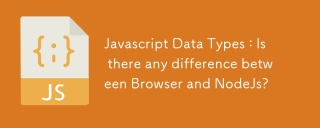
JavaScript core data types are consistent in browsers and Node.js, but are handled differently from the extra types. 1) The global object is window in the browser and global in Node.js. 2) Node.js' unique Buffer object, used to process binary data. 3) There are also differences in performance and time processing, and the code needs to be adjusted according to the environment.
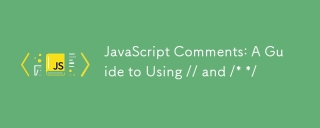
JavaScriptusestwotypesofcomments:single-line(//)andmulti-line(//).1)Use//forquicknotesorsingle-lineexplanations.2)Use//forlongerexplanationsorcommentingoutblocksofcode.Commentsshouldexplainthe'why',notthe'what',andbeplacedabovetherelevantcodeforclari
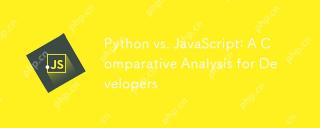
The main difference between Python and JavaScript is the type system and application scenarios. 1. Python uses dynamic types, suitable for scientific computing and data analysis. 2. JavaScript adopts weak types and is widely used in front-end and full-stack development. The two have their own advantages in asynchronous programming and performance optimization, and should be decided according to project requirements when choosing.
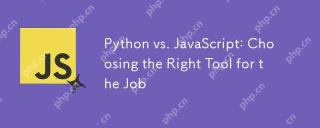
Whether to choose Python or JavaScript depends on the project type: 1) Choose Python for data science and automation tasks; 2) Choose JavaScript for front-end and full-stack development. Python is favored for its powerful library in data processing and automation, while JavaScript is indispensable for its advantages in web interaction and full-stack development.
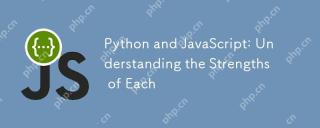
Python and JavaScript each have their own advantages, and the choice depends on project needs and personal preferences. 1. Python is easy to learn, with concise syntax, suitable for data science and back-end development, but has a slow execution speed. 2. JavaScript is everywhere in front-end development and has strong asynchronous programming capabilities. Node.js makes it suitable for full-stack development, but the syntax may be complex and error-prone.
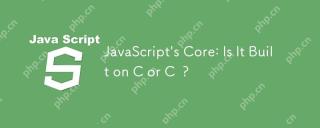
JavaScriptisnotbuiltonCorC ;it'saninterpretedlanguagethatrunsonenginesoftenwritteninC .1)JavaScriptwasdesignedasalightweight,interpretedlanguageforwebbrowsers.2)EnginesevolvedfromsimpleinterpreterstoJITcompilers,typicallyinC ,improvingperformance.
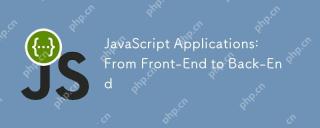
JavaScript can be used for front-end and back-end development. The front-end enhances the user experience through DOM operations, and the back-end handles server tasks through Node.js. 1. Front-end example: Change the content of the web page text. 2. Backend example: Create a Node.js server.
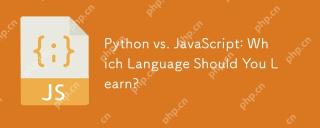
Choosing Python or JavaScript should be based on career development, learning curve and ecosystem: 1) Career development: Python is suitable for data science and back-end development, while JavaScript is suitable for front-end and full-stack development. 2) Learning curve: Python syntax is concise and suitable for beginners; JavaScript syntax is flexible. 3) Ecosystem: Python has rich scientific computing libraries, and JavaScript has a powerful front-end framework.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SublimeText3 Chinese version
Chinese version, very easy to use

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Zend Studio 13.0.1
Powerful PHP integrated development environment

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
