Adds new elements to an array and returns the new length value of the array.
arrayObj.push([item1 [item2 [. . . [itemN ]]]])
Parameters
arrayObj Required. An Array object.
item, item2,. . . itemN optional. The new element of this Array.
Description
The push method will add new elements in the order they appear. If one of the arguments is an array, the array is added to the array as a single element. If you want to combine elements from two or more arrays, use the concat method.
Example
[Ctrl A Select all Note: If you need to introduce external Js, you need to refresh to execute
Requires
version 5.5 pop method
Removes the last element in the array and returns that element.
arrayObj.pop()
The required arrayObj reference is an Array object.
Explanation
If the array is empty, undefined will be returned.
Example If you need to introduce external Js, you need to refresh to execute
]
Requires version 5.5 The
shift method
removes the first element in the array and returns that element.
arrayObj.shift( )
Parameters
The required arrayObj reference is an Array object.
Description
The shift method removes the first element in an array and returns that element.
Requires Version 5.5
unshift method
Inserts the specified element into the array at the beginning and returns the array.
arrayObj.unshift([item1[, item2 [, . . . [, itemN]]]])
Parameters
arrayObj Required. An Array object.
item1, item2,. . .,itemN optional. The element to be inserted at the beginning of this Array.
Description
The unshift method inserts these elements into the beginning of an array, so the elements will appear in the array in the order they are in the argument sequence.
Requires Version 5.5
concat method (Array)
Returns a new array composed of two or more arrays.
array1.concat([item1[, item2[, . . . [, itemN]]]])
Parameters
array1 is required. The Array object to which all other arrays are to be concatenated.
item1,. . ., itemN optional. The other item to be connected to the end of array1.
Description
The concat method returns an Array object containing the connections between array1 and any other items provided.
The items to be added (item1...itemN) will be added to the array in order from left to right. If an item is an array, add its contents to the end of array1. If the item is not an array, it is added to the end of the array as a single array element.
The following copies elements from the source array to the result array:
For object parameters copied from the array being connected to the new array, they still point to the same object after copying. No matter which one of the new array and the source array is changed, it will cause the other to change.
Copy only the value of a numeric or string concatenated into a new array. Changing the values in one array does not affect the values in the other array.
Example
function ConcatArrayDemo(){
var a, b, c, d;
a = new Array(1,2,3);
b = "JScript";
c = new Array(42, "VBScript);
d = a.concat(b, c);
// Return array [1, 2, 3, "JScript", 42, "VBScript" ]
return(d);
要求
版本 3
join 方法
返回字符串值,其中包含了连接到一起的数组的所有元素,元素由指定的分隔符分隔开来。
arrayObj.join(separator)
参数
arrayObj 必选项。Array 对象。
separator 必选项。是一个 String 对象,作为最终的 String 对象中对数组元素之间的分隔符。如果省略了这个参数,那么数组元素之间就用一个逗号来分隔。
说明
如果数组中有元素没有定义或者为 null,将其作为空字符串处理。
示例
下面这个例子说明了 join 方法的用法。
function JoinDemo(){
var a, b;
a = new Array(0,1,2,3,4);
b = a.join("-");
return(b);
}
要求
版本 2
sort 方法
返回一个元素已经进行了排序的 Array 对象。
arrayobj.sort(sortfunction)
参数
arrayObj 必选项。任意 Array 对象。
sortFunction 可选项。是用来确定元素顺序的函数的名称。如果这个参数被省略,那么元素将按照 ASCII 字符顺序进行升序排列。
说明
sort 方法将 Array 对象进行适当的排序;在执行过程中并不会创建新的 Array 对象。
如果为 sortfunction 参数提供了一个函数,那么该函数必须返回下列值之一:
(1)负值,如果所传递的第一个参数比第二个参数小。
(2)零,如果两个参数相等。
(3)正值,如果第一个参数比第二个参数大。
示例
[Ctrl+A 全选 注:如需引入外部Js需刷新才能执行]
要求
版本 2
slice 方法 (Array)
返回一个数组的一段。
arrayObj.slice(start, [end])
参数
arrayObj 必选项。一个 Array 对象。
start 必选项。arrayObj 中所指定的部分的开始元素是从零开始计算的下标。
end 可选项。arrayObj 中所指定的部分的结束元素是从零开始计算的下标。
说明
slice 方法返回一个 Array 对象,其中包含了 arrayObj 的指定部分。
slice 方法一直复制到 end 所指定的元素,但是不包括该元素。如果 start 为负,将它作为 length + start处理,此处 length 为数组的长度。如果 end 为负,就将它作为 length + end 处理,此处 length 为数组的长度。如果省略 end ,那么 slice 方法将一直复制到 arrayObj 的结尾。如果 end 出现在 start 之前,不复制任何元素到新数组中。
示例
在下面这个例子中,除了最后一个元素之外,myArray 中所有的元素都被复制到 newArray 中:
newArray = myArray.slice(0, -1)
splice 方法
从一个数组中移除一个或多个元素,如果必要,在所移除元素的位置上插入新元素,返回所移除的元素。
arrayObj.splice(start, deleteCount, [item1[, item2[, . . . [,itemN]]]])
参数
arrayObj 必选项。一个 Array 对象。
start 必选项。指定从数组中移除元素的开始位置,这个位置是从 0 开始计算的。
deleteCount 必选项。要移除的元素的个数。
item1, item2,. . .,itemN 必选项。要在所移除元素的位置上插入的新元素。
说明
splice 方法可以移除从 start 位置开始的指定个数的元素并插入新元素,从而修改 arrayObj。返回值是一个由所移除的元素组成的新 Array 对象。
要求
版本 5.5
reverse 方法
返回一个元素顺序被反转的 Array 对象。
arrayObj.reverse( )
参数
arrayObj 必选项,该参数为 Array 对象。
说明
reverse 方法将一个 Array 对象中的元素位置进行反转。在执行过程中,这个方法并不会创建一个新的 Array 对象。
如果数组是不连续的,reverse 方法将在数组中创建元素以便填充数组中的间隔。这样所创建的全部元素的值都是 undefined。
示例
下面这个例子说明了 reverse 方法的用法:
function ReverseDemo(){
var a, l; // Declare variables.
a = new Array(0,1,2,3,4); // Create an array and assign values.
l = a.reverse(); // Reverse the contents of the array.
return(l); // Return the result array.
}
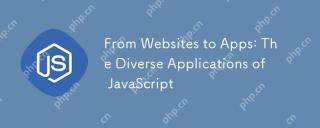
JavaScript is widely used in websites, mobile applications, desktop applications and server-side programming. 1) In website development, JavaScript operates DOM together with HTML and CSS to achieve dynamic effects and supports frameworks such as jQuery and React. 2) Through ReactNative and Ionic, JavaScript is used to develop cross-platform mobile applications. 3) The Electron framework enables JavaScript to build desktop applications. 4) Node.js allows JavaScript to run on the server side and supports high concurrent requests.
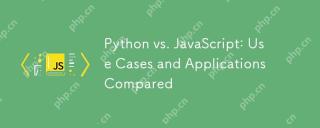
Python is more suitable for data science and automation, while JavaScript is more suitable for front-end and full-stack development. 1. Python performs well in data science and machine learning, using libraries such as NumPy and Pandas for data processing and modeling. 2. Python is concise and efficient in automation and scripting. 3. JavaScript is indispensable in front-end development and is used to build dynamic web pages and single-page applications. 4. JavaScript plays a role in back-end development through Node.js and supports full-stack development.
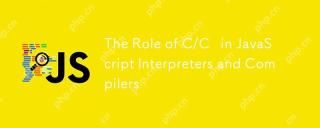
C and C play a vital role in the JavaScript engine, mainly used to implement interpreters and JIT compilers. 1) C is used to parse JavaScript source code and generate an abstract syntax tree. 2) C is responsible for generating and executing bytecode. 3) C implements the JIT compiler, optimizes and compiles hot-spot code at runtime, and significantly improves the execution efficiency of JavaScript.
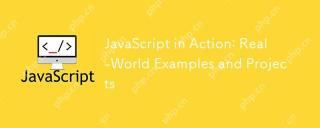
JavaScript's application in the real world includes front-end and back-end development. 1) Display front-end applications by building a TODO list application, involving DOM operations and event processing. 2) Build RESTfulAPI through Node.js and Express to demonstrate back-end applications.
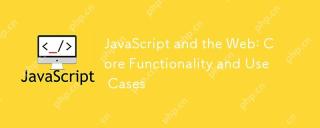
The main uses of JavaScript in web development include client interaction, form verification and asynchronous communication. 1) Dynamic content update and user interaction through DOM operations; 2) Client verification is carried out before the user submits data to improve the user experience; 3) Refreshless communication with the server is achieved through AJAX technology.
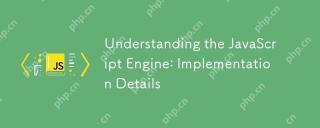
Understanding how JavaScript engine works internally is important to developers because it helps write more efficient code and understand performance bottlenecks and optimization strategies. 1) The engine's workflow includes three stages: parsing, compiling and execution; 2) During the execution process, the engine will perform dynamic optimization, such as inline cache and hidden classes; 3) Best practices include avoiding global variables, optimizing loops, using const and lets, and avoiding excessive use of closures.

Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
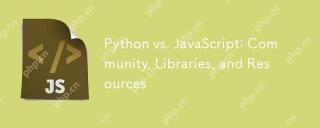
Python and JavaScript have their own advantages and disadvantages in terms of community, libraries and resources. 1) The Python community is friendly and suitable for beginners, but the front-end development resources are not as rich as JavaScript. 2) Python is powerful in data science and machine learning libraries, while JavaScript is better in front-end development libraries and frameworks. 3) Both have rich learning resources, but Python is suitable for starting with official documents, while JavaScript is better with MDNWebDocs. The choice should be based on project needs and personal interests.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
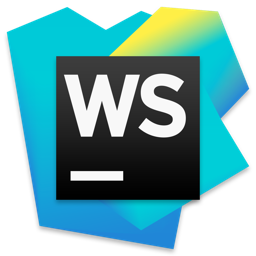
WebStorm Mac version
Useful JavaScript development tools

Atom editor mac version download
The most popular open source editor
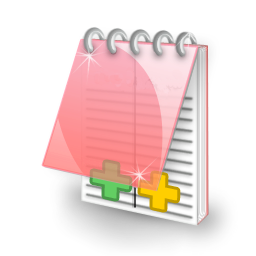
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software