return function CompareTRs(TR1, TR2){
var value1,value2;
value1=convert(TR1.cells[Col].firstChild.nodeValue,DataType);
value2=convert(TR2.cells[Col].firstChild.nodeValue, DataType);
if(value1 < value2)
return -1;
else if(value1 > value2)
return 1;
else
return 0;
};
Of course, being able to write it in this form is thanks to closures. In the sort method, iterate through each item in the array (each item stores each row of the table) and pass the parameters into CompareTRs(TR1,TR2), and then return the result.
In fact, this is OK, but what if you want to sort the pictures?
What type of picture is it? I don’t know, so let’s try a trick and use the title of the picture or the alt attribute. They can always be strings. Give them a custom property customvalue and sort by its value. Just when implementing
to determine whether it contains this attribute, then the CompareTRs method needs to be modified.
function CustomCompare(Col,DataType){
return function CompareTRs(TR1,TR2){
var value1,value2;
//Determine whether there is a customvalue attribute
if(TR1.cells[Col].getAttribute("customvalue")){
value1=convert(TR1.cells[Col].getAttribute("customvalue"),DataType);
value2=convert(TR2.cells[Col].getAttribute("customvalue"),DataType);
}
else{
value1=convert(TR1.cells[Col].firstChild.nodeValue,DataType);
value2=convert(TR2.cells[Col].firstChild.nodeValue,DataType);
}
if(value1 < value2)
return -1;
else if(value1 > value2)
return 1;
else
return 0;
};
}
The sorting of pictures has also been solved. What if the user wants to sort multiple times and click multiple times? Do we still need to modify the CompareTRs method?
Obviously not necessary, there is a reverse() method in JavaScript that can reverse each item in the array. The modification to the SortTable method only needs to be like this
function SortTable( TableID,Col,DataType){
var DTable=document.getElementById(TableID);
var DBody=DTable.tBodies[0];
var DataRows=DBody.rows;
var MyArr=new Array;
for(var i=0;iMyArr[i]=DataRows[i];
}
//Judge the last sorted column sum Is it the same column this time?
if(DBody.CurrentCol==Col){
MyArr.reverse(); //Invert the array
}
else{
MyArr.sort(CustomCompare (Col,DataType));
}
//Create a document fragment and add all the lines to it, which is equivalent to a temporary storage shelf. The purpose is (if added directly to document.body, it will be inserted One row will be refreshed once. If there is too much data, it will affect the user experience)
//First put all the rows in the temporary storage shelf, and then add the rows in the temporary storage shelf to document.body, so that the table only It will be refreshed once.
//Just like when you go shopping in a store, you first write down all the items (rows) you want to buy on a list (document fragments), and then buy them all in the supermarket without thinking of just one thing. Then
var frag=document.createDocumentFragment();
for(var i=0;ifrag.appendChild(MyArr[i]); //Add all rows in the array To the document fragment
}
DBody.appendChild(frag);//Add all the rows in the document fragment to the body
DBody.CurrentCol=Col; //Record the currently sorted column
}
Be sure to pay attention to the capitalization in JavaScript, as it is easy to make mistakes.
The above code was tested successfully, and the effect of sorting dates is as shown below
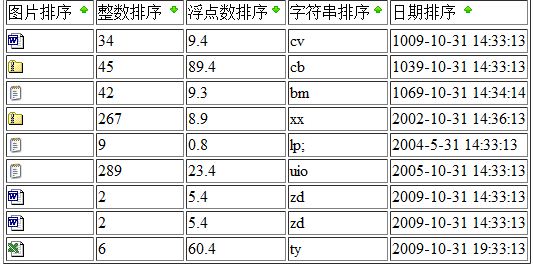
All codes:
Table sorting
图片排序 |
整数排序  |
浮点数排序 |
字符串排序 |
日期排序  |
 |
2 |
5.4 |
zd |
2009-10-31 14:33:13 |
 |
267 |
8.9 |
xx |
2002-10-31 14:36:13 |
 |
6 |
60.4 |
ty |
2009-10-31 19:33:13 |
 |
9 |
0.8 |
lp; |
2004-5-31 14:33:13 |
 |
34 |
9.4 |
cv |
1009-10-31 14:33:13 |
 |
289 |
23.4 |
uio |
2005-10-31 14:33:13 |
 |
45 |
89.4 |
cb |
1039-10-31 14:33:13 |
 |
2 |
5.4 |
zd |
2009-10-31 14:33:13 |
 |
42 |
9.3 |
bm |
1069-10-31 14:34:14 |