In fact, object-oriented thinking is independent of programming languages. For example, in C#, in a static method of a static class, a series of static functions are called according to procedural development. It is difficult to say that this is object-oriented programming. On the contrary, it is like Excellent JavaScript libraries such as jquery and extjs embody object-oriented design ideas everywhere. This article does not intend to discuss whether JavaScript can be regarded as an object-oriented programming language. This issue is something that people who value Chinese-style exams should pay attention to. I will just briefly explain how to use object-oriented programming ideas in JavaScript.
Object-oriented must first have objects. Creating an object in javascript is very simple:
var o= {};
This creates an object, and we can easily add properties and methods to this object:
o.name="object name";
o.showName=function(){
alert(o.name);
}
However, most people are still used to putting the properties and methods of an object inside a pair of {} that defines the object:
var o = {
name: "object name",
showName: function() {
alert(o.name);
}
}
There are two ways to access properties and methods, the first one:
alert(o.name);
o.showName();
This way of writing is very common, and it is also the same way to call the properties and methods of objects in C#. There is also a special one in JavaScript, which uses the name of the attribute or method as an index to access:
alert(o["name"]);
o["showName"]();
This seems to be a bit like Kong Yiji's "fennel" There are several ways to write the word "fennel". In fact, few people use indexes to call the properties or methods of objects.
In addition to our custom properties and methods, our object also has a constructor property and toString() and other methods. These properties and methods come from the Object built-in object, and all objects will have these properties and methods. The constructor attribute points to the constructor that constructed the object. We did not use the constructor to create the object. In fact, the js interpreter will use the Object constructor. If we define the constructor ourselves, we can create objects through the constructor, so that the created objects have the same properties and methods, which starts to feel a bit object-oriented. Okay, let’s start with a simple example to see how to create a constructor:
function Person(name, sex, age) {
this.name = name;
this.sex = sex;
this.age = age;
this.showInfo = function() {
alert("Name: " this.name " Gender: " this.sex " Age: " this.age);
}
}
us A constructor named Person is defined. The constructor has three attributes and one method. It is very simple to generate an object and call the method through the constructor:
var zhangsan = new Person("Zhang San", "Male", 18);
zhangsan.showInfo();
After running, we can see a dialog box pop up, showing the information of the person named Zhang San:
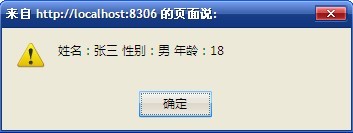
We can also look at the constructor property of the object. See if the constructor of zhangsan is the Person we defined:
alert(zhangsan.constructor);
The result is as shown in the figure:
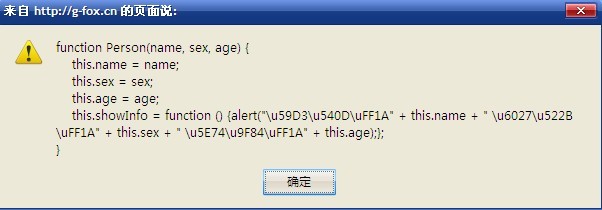
As you can see, it is our Person constructor.
However, there is still a problem here. Every time we construct an object, memory space will be allocated in memory for properties and methods. In fact, all objects can use the same method, and there is no need to have multiple methods. copy, which is a waste of memory space. Now that we are aware of this problem, let's think about how to solve it. A natural idea is that since we only want to allocate memory space for the method once, we can set a value to identify whether the memory space of the method has been allocated. Following this idea, we will make the following modifications to the constructor:
function Person(name, sex, age) {
this. name = name;
this.sex = sex;
this.age = age;
if (typeof Person._initialized == "undefined") {
this.showInfo = function() {
alert("Name: " this.name " Gender: " this.sex " Age: " this.age);
}
Person._initialized = true;
}
}
Here, we use a member _initialized to indicate whether memory space has been allocated for the method. When the first object is constructed, _initialized is not defined, so our judgment statement is true. At this time, the method is defined and memory space is allocated, and then the value of _initialized is set to true to indicate the method. Memory space has been allocated. When the second object is constructed, no judgment will be entered, so memory space will not be allocated again. There seems to be no problem. Run it and see that Zhang San's information is still displayed normally. Although it's not hard work, I solved a small problem, so let's celebrate. Let's have a plate of twice-cooked pork. I want to enjoy it. Before the meal started, a girl named Li Si also wanted her personal information to pop up on the computer. OK, it’s very simple. Just construct an object and call the showInfo method:
var lisi = new Person("李思", "女", 28);
lisi.showInfo();
In order to take care of MM, I also put this paragraph Put it in front of Zhang San. MM's information is displayed correctly, but Zhang San's information is missing. This time Zhang San was not happy. It was okay to be ranked behind MM, but he still had to have a name. This is hard on me as a programmer. It seems that I can’t eat the twice-cooked pork. Let’s fix the bug first. Open firebug, and after seeing the MM information displayed, an error occurs. The prompt is: zhangsan.showInfo is not a function. Set a breakpoint and take a look. After constructing the zhangsi object, we found that there is no showInfo method. It turns out that although there is only one showInfo method, it exists in the first object and cannot be accessed by the second object. So, how can we make objects generated by the same constructor share the same function? The prototype in javascript provides us with this function. According to the JavaScript specification, each constructor has a prototype attribute for inheritance and attribute sharing. Our showInfo method can also be viewed as a property that points to a reference to a function. Now we use prototype to make our methods shareable. The code change is very simple. Just change this.showInfo to Person.prototype.showInfo. The code after the change is as follows:
function Person(name, sex, age) {
this.name = name;
this .sex = sex;
this.age = age;
if (typeof Person._initialized == "undefined") {
Person.prototype.showInfo = function() {
alert("Name : " this.name " Gender: " this.sex " Age: " this.age);
}
Person._initialized = true;
}
}
Use this constructor to generate two objects:
var lisi = new Person("李Si", "Female", 28);
lisi.showInfo();
var zhangsan = new Person("Zhang San", "Male", 18);
zhangsan .showInfo();
After running, the information of John Si will be displayed first, and then the information of Zhang San. Now both of us are satisfied, but unfortunately my twice-cooked pork is already cold.