HTTP Middleware
Introduction
HTTP middleware provide a convenient mechanism for filtering HTTP requests entering your application. For example, Laravel includes a middleware that verifies the user of your application is authenticated. If the user is not authenticated, the middleware will redirect the user to the login screen. However, if the user is authenticated, the middleware will allow the request to proceed further into the application.
Of course, additional middleware can be written to perform a variety of tasks besides authentication. A CORS middleware might be responsible for adding the proper headers to all responses leaving your application. A logging middleware might log all incoming requests to your application.
There are several middleware included in the Laravel framework, including middleware for maintenance, authentication, CSRF protection, and more. All of these middleware are located in the app/Http/Middlewaredirectory.
Defining Middleware
To create a new middleware, use the make:middlewareArtisan command:
创建中间件可以使用工具创建,artisan是个帮助你创建一些中间件或者其他的好工具,
php artisan make:middleware AgeMiddleware
这个命令会创建一个中间件(其实是一个class)在你的 app/Http/Middleware目录里面,并且文件里面已经包含了一些写好的模板,例如这里,
cat app/Http/Middleware/AgeMiddleware.php< ?phpnamespace App\Http\Middleware;use Closure;class AgeMiddleware{ /** * Handle an incoming request. * * @param \Illuminate\Http\Request $request * @param \Closure $next * @return mixed */ public function handle($request, Closure $next)//支持2个参数,一个是request,另外是一个闭包(暂时未知) { return $next($request); //返回一个处理过的$request }}//这个只是默认样例。
This command will place a new AgeMiddlewareclass within your app/Http/Middlewaredirectory. In this middleware, we will only allow access to the route if the supplied ageis greater than 200. Otherwise, we will redirect the users back to the “home” URI.
这个中间件是修改过的,改成只允许支持age大于200的请求进入路由,否则重定向到home页面
<?phpnamespace App\Http\Middleware;use Closure;class AgeMiddleware{ /** * Run the request filter. * * @param \Illuminate\Http\Request $request * @param \Closure $next * @return mixed */ public function handle($request, Closure $next) { if ($request->input('age') < = 200) {//调用input方法,并且传入参数age, return redirect('home'); } return $next($request); }}
As you can see, if the given ageis less than or equal to 200, the middleware will return an HTTP redirect to the client; otherwise, the request will be passed further into the application. To pass the request deeper into the application (allowing the middleware to “pass”), simply call the $nextcallback with the $request.
中间件有点像过滤器,好像一系列的过滤层去不断过滤http请求。
It’s best to envision middleware as a series of “layers” HTTP requests must pass through before they hit your application. Each layer can examine the request and even reject it entirely.
Before/ AfterMiddleware
Whether a middleware runs before or after a request depends on the middleware itself. For example, the following middleware would perform some task beforethe request is handled by the application:
一个中间件是在请求到达之前或者之后是要看中间件本身的,下面这个是在请求来之前执行的
< ?phpnamespace App\Http\Middleware;use Closure;class BeforeMiddleware //明显的标志BeforeMiddleware{ public function handle($request, Closure $next) { // Perform action return $next($request); }}
However, this middleware would perform its task afterthe request is handled by the application:
<?phpnamespace App\Http\Middleware;use Closure;class AfterMiddleware //这是请求之后的{ public function handle($request, Closure $next) { $response = $next($request); // Perform action return $response; }}
Registering Middleware
Global Middleware
If you want a middleware to be run during every HTTP request to your application, simply list the middleware class in the $middlewareproperty of your app/Http/Kernel.phpclass.
如果需要中间件对每个http请求都操作的话,简单的写法是,将中间件写在 app/Http/Kernel.php的
$middleware属性里面,例如
protected $middleware = [ \Illuminate\Foundation\Http\Middleware\CheckForMaintenanceMode::class, ];
Assigning Middleware To Routes
分配给路由的中间件,需要在你的app/Http/Kernel.php里(如下例)为中间件创建一个短名,
If you would like to assign middleware to specific routes, you should first assign the middleware a short-hand key in your app/Http/Kernel.phpfile. By default, the $routeMiddlewareproperty of this class contains entries for the middleware included with Laravel. To add your own, simply append it to this list and assign it a key of your choosing. For example:
// Within App\Http\Kernel Class...protected $routeMiddleware = [ 'auth' => \App\Http\Middleware\Authenticate::class, //就好像这样,将名字绑定中间件 'auth.basic' => \Illuminate\Auth\Middleware\AuthenticateWithBasicAuth::class, 'guest' => \App\Http\Middleware\RedirectIfAuthenticated::class, 'throttle' => \Illuminate\Routing\Middleware\ThrottleRequests::class,];
Once the middleware has been defined in the HTTP kernel, you may use the middlewarekey in the route options array:
Route::get('admin/profile', ['middleware' => 'auth', function () { //在路由里面就能够指定使用的中间件了 }]);
Use an array to assign multiple middleware to the route:
Route::get('/', ['middleware' => ['first', 'second'], function () { //分配多个中间件可以用数组}]);
Instead of using an array, you may also chain the middlewaremethod onto the route definition:
Route::get('/', function () { // 这里用链式方式写,使用middleware方法定义})->middleware(['first', 'second']);
When assigning middleware, you may also pass the fully qualified class name:
use App\Http\Middleware\FooMiddleware;Route::get('admin/profile', ['middleware' => FooMiddleware::class, function () { //传递一个完整的中间件类名,还需要加上use那部分,因为要寻找位置}]);
Middleware Groups
Sometimes you may want to group several middleware under a single key to make them easier to assign to routes. You may do this using the $middlewareGroupsproperty of your HTTP kernel.
使用中间件组,使用 $middlewareGroups属性
Out of the box, Laravel comes with weband apimiddleware groups that contains common middleware you may want to apply to web UI and your API routes:
默认laravel就在5.2版本提供了web和api的中间件组,他包含了普遍的中间件
/** * The application's route middleware groups. * * @var array */protected $middlewareGroups = [ //这里就是$middlewareGroups 'web' => [ \App\Http\Middleware\EncryptCookies::class, \Illuminate\Cookie\Middleware\AddQueuedCookiesToResponse::class, \Illuminate\Session\Middleware\StartSession::class, \Illuminate\View\Middleware\ShareErrorsFromSession::class, \App\Http\Middleware\VerifyCsrfToken::class, ],//各自定义了一些中间件,例如一些cookie,session的中间件 'api' => [ 'throttle:60,1', 'auth:api', ],];
Middleware groups may be assigned to routes and controller actions using the same syntax as individual middleware. Again, middleware groups simply make it more convenient to assign many middleware to a route at once:使用相同的命令可以像使用单个中间件一样去将中间件组分配到路由或者控制器里,
Route::group(['middleware' => ['web']], function () { //这里即使用了$middlewareGroups来编制中间件组,但是依然可以用单个中间件的使用方式来调用。});
Middleware Parameters
Middleware can also receive additional custom parameters. For example, if your application needs to verify that the authenticated user has a given “role” before performing a given action, you could create a RoleMiddlewarethat receives a role name as an additional argument.
中间件会收到额外的参数,举例,如果你的应用需要确认验证用户在执行动作之前已经有一个role,你应该建立一个 RoleMiddleware来接收这个额外的参数。
Additional middleware parameters will be passed to the middleware after the $nextargument:
额外的中间件参数会被传递到中间件的 $next的后面
< ?phpnamespace App\Http\Middleware;use Closure;class RoleMiddleware{ /** * Run the request filter. * * @param \Illuminate\Http\Request $request * @param \Closure $next * @param string $role * @return mixed */ public function handle($request, Closure $next, $role) //增加了一个$role参数 { if (! $request->user()->hasRole($role)) { // Redirect... } return $next($request); }}
Middleware parameters may be specified when defining the route by separating the middleware name and parameters with a :. Multiple parameters should be delimited by commas:
Route::put('post/{id}', ['middleware' => 'role:editor', function ($id) { //用冒号分隔,中间件的名字:参数,这样也可以指定中间件的参数}]);
Terminable Middleware
有时候需要一个处理一些http回应已经发到浏览器之后的事情的中间件,例如session中间件包括了laravel写session数据并存储,在http回应发到浏览器之后,
Sometimes a middleware may need to do some work after the HTTP response has already been sent to the browser. For example, the “session” middleware included with Laravel writes the session data to storage afterthe response has been sent to the browser. To accomplish this, define the middleware as “terminable” by adding a terminatemethod to the middleware:
要实现这些,需要顶一个terminable中间件,增加一个terminate方法
<?phpnamespace Illuminate\Session\Middleware;use Closure;class StartSession{ public function handle($request, Closure $next) { return $next($request); } public function terminate($request, $response) { // Store the session data... 这个方法就是terminate方法,会接收请求和回应作为参数,当你已经定义一个这样的中间件的时候,你应该将它增加到全局的中间件列表里面去,kernel.php }}
The terminatemethod should receive both the request and the response. Once you have defined a terminable middleware, you should add it to the list of global middlewares in your HTTP kernel.
当中间件的 terminate方法调用的时候,laravel会从service container中分解一个fresh 中间件实例,如果handle和terminate方法被调用的时候,你使用一个相同的中间件实例的话,会使用这个container的singleton方法注册这个中间件
When calling the terminatemethod on your middleware, Laravel will resolve a fresh instance of the middleware from the service container. If you would like to use the same middleware instance when the handleand terminatemethods are called, register the middleware with the container using the container’s singletonmethod.
参考引用:
https://laravel.com/docs/5.2/middleware
http://laravelacademy.org/post/2803.html本文由 PeterYuan 创作,采用 署名-非商业性使用 2.5 中国大陆 进行许可。 转载、引用前需联系作者,并署名作者且注明文章出处。神一样的少年 » laravel5.2-http middleware学习
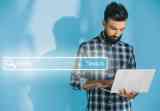
Long URLs, often cluttered with keywords and tracking parameters, can deter visitors. A URL shortening script offers a solution, creating concise links ideal for social media and other platforms. These scripts are valuable for individual websites a
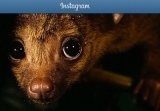
Following its high-profile acquisition by Facebook in 2012, Instagram adopted two sets of APIs for third-party use. These are the Instagram Graph API and the Instagram Basic Display API.As a developer building an app that requires information from a
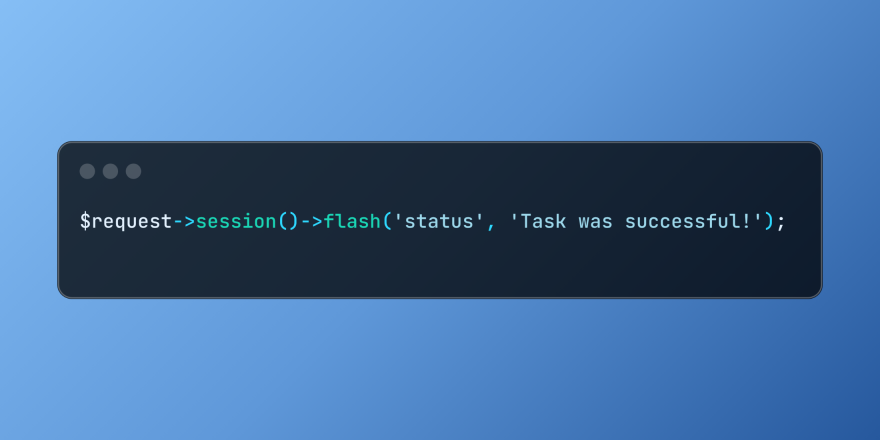
Laravel simplifies handling temporary session data using its intuitive flash methods. This is perfect for displaying brief messages, alerts, or notifications within your application. Data persists only for the subsequent request by default: $request-
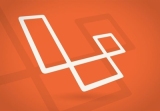
This is the second and final part of the series on building a React application with a Laravel back-end. In the first part of the series, we created a RESTful API using Laravel for a basic product-listing application. In this tutorial, we will be dev
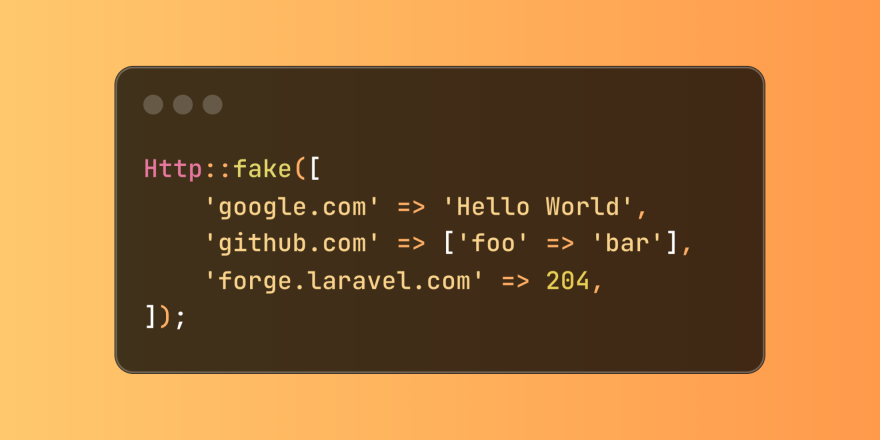
Laravel provides concise HTTP response simulation syntax, simplifying HTTP interaction testing. This approach significantly reduces code redundancy while making your test simulation more intuitive. The basic implementation provides a variety of response type shortcuts: use Illuminate\Support\Facades\Http; Http::fake([ 'google.com' => 'Hello World', 'github.com' => ['foo' => 'bar'], 'forge.laravel.com' =>
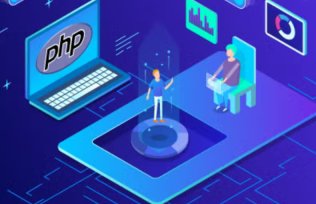
The PHP Client URL (cURL) extension is a powerful tool for developers, enabling seamless interaction with remote servers and REST APIs. By leveraging libcurl, a well-respected multi-protocol file transfer library, PHP cURL facilitates efficient execution of various network protocols, including HTTP, HTTPS, and FTP. This extension offers granular control over HTTP requests, supports multiple concurrent operations, and provides built-in security features.
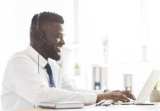
Do you want to provide real-time, instant solutions to your customers' most pressing problems? Live chat lets you have real-time conversations with customers and resolve their problems instantly. It allows you to provide faster service to your custom
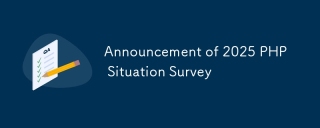
The 2025 PHP Landscape Survey investigates current PHP development trends. It explores framework usage, deployment methods, and challenges, aiming to provide insights for developers and businesses. The survey anticipates growth in modern PHP versio


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
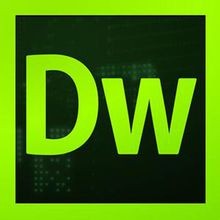
Dreamweaver CS6
Visual web development tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
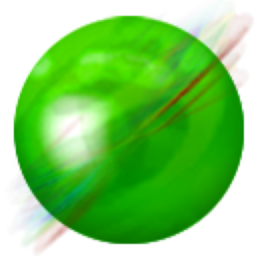
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
