var rgb2hex = function(rgb) {
rgb = rgb. match(/^rgb((d ),s*(d ),s*(d ))$/);
return "#" tohex(rgb[1]) tohex(rgb[2]) tohex(rgb [3])
}
var tohex = function(x) {
var hexDigits = ['0','1','2','3','4','5', '6','7','8','9','A','B','C','D','E','F'];
return isNaN(x) ? '00' : hexDigits[(x - x % 16) / 16] hexDigits[x % 16];
}
We use regular expressions to detect whether it is in rgb format, yes Just use rgb2hex to convert it. But if it's red, green, etc., Firefox goes against the norm and converts it to hex format, but IE remains the same. We have no choice but to make a hash ourselves, put in all the commonly used colors, and then match them one by one.
if(style.search(/background|color/) != -1) {
var color = {
aqua: '#0ff',
black: '#000',
blue: '#00f',
gray: '# 808080',
purple: '#800080',
fuchsia: '#f0f',
green: '#008000',
lime: '#0f0',
maroon: '# 800000',
navy: '#000080',
olive: '#808000',
orange:'#ffa500',
red: '#f00',
silver: '# c0c0c0',
teal: '#008080',
transparent:'rgba(0,0,0,0)',
white: '#fff',
yellow: '#ff0'
}
if(!!color[value]){
value = color[value]
}
if(value == "inherit"){
return getStyle(el .parentNode,style);
}
if(/^rgb((d ),s*(d ),s*(d ))$/.test(value)){
return rgb2hex( value)
}else if(/^#/.test(value)){
value = value.replace('#', '');
return "#" (value.length == 3? value.replace(/^(w)(w)(w)$/, '$1$1$2$2$3$3') : value);
}
return value;
}
Basically, this is the exact value of CSS. Obviously it still has many shortcomings, but it has implemented both layout and commonly used styles. A constant q is also provided to determine the page rendering mode. For convenience, the method name has the same name as JQuery (it can only take values, not assign values. I will slowly integrate it with my addSheet function when I have time in the future).
(function(){
var isQuirk = (document.documentMode) ? (document.documentMode==5) ? true : false : ((document.compatMode=="CSS1Compat") ? false : true);
var isElement = function(el) {
return !!(el && el.nodeType == 1);
}
var propCache = [];
var propFloat = ! "v1" ? 'styleFloat' : 'cssFloat';
var camelize = function(attr){
return attr.replace(/-(w)/g, function(all, letter){
return letter.toUpperCase();
});
}
var memorize = function(prop) { //意思为:check out form cache
return propCache[prop] || (propCache[prop] = prop == 'float' ? propFloat : camelize(prop));
}
var getIEOpacity = function(el){
var filter;
if(!!window.XDomainRequest){
filter = el.style.filter.match(/progid:DXImageTransform.Microsoft.Alpha(.?opacity=(.*).?)/i);
}else{
filter = el.style.filter.match(/alpha(opacity=(.*))/i);
}
if(filter){
var value = parseFloat(filter[1]);
if (!isNaN(value)) {
return value ? value / 100 : 0;
}
}
return 1;
}
var convertPixelValue = function(el, value){
var style = el.style,left = style.left,rsLeft = el.runtimeStyle.left;
el.runtimeStyle.left = el.currentStyle.left;
style.left = value || 0;
var px = style.pixelLeft;
style.left = left;//还原数据
el.runtimeStyle.left = rsLeft;//还原数据
return px "px"
}
var rgb2hex = function(rgb) {
rgb = rgb.match(/^rgb((d ),s*(d ),s*(d ))$/);
return "#" tohex(rgb[1]) tohex(rgb[2]) tohex(rgb[3])
}
var tohex = function(x) {
var hexDigits = ['0','1','2','3','4','5','6','7','8','9','A','B','C','D','E','F'];
return isNaN(x) ? '00' : hexDigits[(x - x % 16) / 16] hexDigits[x % 16];
}
var getStyle = function (el, style){
var value;
if(! "v1"){
//特殊处理IE的opacity
if(style == "opacity"){
return getIEOpacity(el)
}
value = el.currentStyle[memorize(style)];
//特殊处理IE的height与width
if (/^(height|width)$/.test(style)){
var values = (style == 'width') ? ['left', 'right'] : ['top', 'bottom'], size = 0;
if(isQuirk){
return el[camelize("offset-" style)] "px"
}else{
var client = parseFloat(el[camelize("client-" style)]),
paddingA = parseFloat(getStyle(el, "padding-" values[0])),
paddingB = parseFloat(getStyle(el, "padding-" values[1]));
return (client - paddingA - paddingB) "px";
}
}
}else{
if(style == "float"){
style = propFloat;
}
value = document.defaultView.getComputedStyle(el, null).getPropertyValue(style)
}
//下面部分全部用来转换上面得出的非精确值
if(!/^d px$/.test(value)){
//转换可度量的值
if(/(em|pt|mm|cm|pc|in|ex|rem|vw|vh|vm|ch|gr)$/.test(value)){
return convertPixelValue(el,value);
}
//转换百分比,不包括字体
if(/%$/.test(value) && style != "font-size"){
return parseFloat(getStyle(el.parentNode,"width")) * parseFloat(value) /100 "px"
}
//转换border的thin medium thick
if(/^(border). (width)$/.test(style)){
var s = style.replace("width","style"),
b = {
thin:["1px","2px"],
medium:["3px","4px"],
thick:["5px","6px"]
};
if(value == "medium" && getStyle(el,s) == "none"){
return "0px";
}
return !!window.XDomainRequest ? b[value][0] : b[value][1];
}
//转换margin的auto
if(/^(margin). /.test(style) && value == "auto"){
var father = el.parentNode;
if(/MSIE 6/.test(navigator.userAgent) && getStyle(father,"text-align") == "center"){
var fatherWidth = parseFloat(getStyle(father,"width")),
_temp = getStyle(father,"position");
father.runtimeStyle.postion = "relative";
var offsetWidth = el.offsetWidth;
father.runtimeStyle.postion = _temp;
return (fatherWidth - offsetWidth)/2 "px";
}
return "0px";
}
//转换top|left|right|bottom的auto
if(/(top|left|right|bottom)/.test(style) && value == "auto"){
return el.getBoundingClientRect()[style];
}
//转换颜色
if(style.search(/background|color/) != -1) {
var color = {
aqua: '#0ff',
black: '#000',
blue: '#00f',
gray: '#808080',
purple: '#800080',
fuchsia: '#f0f',
green: '#008000',
lime: '#0f0',
maroon: '#800000',
navy: '#000080',
olive: '#808000',
orange:'#ffa500',
red: '#f00',
silver: '#c0c0c0',
teal: '#008080',
transparent:'rgba(0,0,0,0)',
white: '#fff',
yellow: '#ff0'
}
if(!!color[value]){
value = color[value]
}
if(value == "inherit"){
return getStyle(el.parentNode,style);
}
if(/^rgb((d ),s*(d ),s*(d ))$/.test(value)){
return rgb2hex(value)
}else if(/^#/.test(value)){
value = value.replace('#', '');
return "#" (value.length == 3 ? value.replace(/^(w)(w)(w)$/, '$1$1$2$2$3$3') : value);
}
return value;
}
}
return value;//such as 0px
}
var css = function(){
var a = arguments;
if(a.length == 1){
return getStyle (this,a[0])
}
}
var _ = function(el){
var el = isElement(el)? el :document.getElementById(el);
var gene = !el.constructor ? el : el.constructor.prototype;
gene.css = css;
gene.width = function(){
return getStyle(this,"width");
};
gene.height = function(){
return getStyle(this,"height");
};
return el
}
if(!window. _){ //In order to avoid conflicts with JQuery's $, I use _ as the only global variable in the class library
window['_'] =_;
}
_.q = isQuirk;
})()
Usage is as follows:
window.onload = function(){
alert(_("ccc").css("background-color"))
alert(_("aaa").css(" width"))
alert(_(document.body).width())
};
We can use this thing to study document.body and document.documentElement.
function text(){
var body = document .body,html = document.documentElement;
_("w1").innerHTML = _(body).width();
_("w2").innerHTML = _(html).width() ;
_("h1").innerHTML = _(body).height();
_("h2").innerHTML = _(html).height();
_("ml1 ").innerHTML = _(body).css("margin-left");
_("ml2").innerHTML = _(html).css("margin-left");
_( "mr1").innerHTML = _(body).css("margin-right");
_("mr2").innerHTML = _(html).css("margin-right");
_("mt1").innerHTML = _(body).css("margin-top");
_("mt2").innerHTML = _(html).css("margin-top");
_("mb1").innerHTML = _(body).css("margin-bottom");
_("mb2").innerHTML = _(html).css("margin-bottom") ;
_("pl1").innerHTML = _(body).css("padding-left");
_("pl2").innerHTML = _(html).css("padding-left" ");
_("pr1").innerHTML = _(body).css("padding-right");
_("pr2").innerHTML = _(html).css("padding -right");
_("bl1").innerHTML = _(body).css("border-left-width");
_("bl2").innerHTML = _(html). css("border-left-width");
_("br1").innerHTML = _(body).css("border-right-width");
_("br2").innerHTML = _(html).css("border-right-width");
_("qqq").innerHTML = !_.q ? "Standard Mode" : "Quirk Mode";
_(" t1").innerHTML = _(body).css("top");
_("t2").innerHTML = _(html).css("top");
_("l1" ).innerHTML = _(body).css("left");
_("l2").innerHTML = _(html).css("left");
_("ot1"). innerHTML = body.offsetTop;
_("ot2").innerHTML = html.offsetTop;
_("ol1").innerHTML = body.offsetLeft;
_("ol2").innerHTML = html.offsetLeft;
_("ct1").innerHTML = body.clientTop;
_("ct2").innerHTML = html.clientTop;
_("cl1").innerHTML = body. clientLeft;
_("cl2").innerHTML = html.clientLeft;
_("cw1").innerHTML = body.clientWidth;
_("cw2").innerHTML = html.clientWidth;
_("ow1").innerHTML = body.offsetWidth;
_("ow2").innerHTML = html.offsetWidth;
_("sw1").innerHTML = body.scrollWidth;
_("sw2").innerHTML = html.scrollWidth;
}
In standard mode, Firefox In the browser, we see that the maximum offsetWidth value is 1007, because Firefox's offsetWidth is not greater than clientWidth, and clientWidth does not include scroll bars (the width of the scroll bars is fixed at 17px). In IE, offsetWidth has two more borders than clientWidth. From this, the problem is discovered. 1024-1003-17=4. 4 should be generated by two autos, and this auto should be the value of border. These two borders are in IE. It is fixed and cannot be modified by the following means.
In other words, in standard mode, IE's html has an unmodifiable width. 2px border. In other words, there is a bug in my program, which does not correctly display the border of html as 2px, which is embarrassing.
Let’s look at quirks mode.
There is no so-called quirks mode in Firefox and others. Just look at IE. I found that the mysterious 2px appeared again, now in the clientTop and clientLeft of document.body. So what are the concepts of clientTop and clientLeft of document.body in quirks mode equivalent to CSS? Let's look at an old picture provided by Microsoft. At that time, IE5 was the only one in the world. There was no so-called standard mode and quirks mode, so everything in this picture was represented by quirks mode.
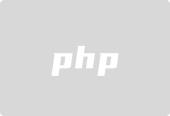
It is not difficult to see that clientLeft is equivalent to borderLeft and clientTop is equivalent to borderTop. As for the border-left-width and border-right-width above, don't read them. It's a mistake because I didn't consider the problems of these two elements in standard mode and quirks mode. Since the border area of document.body reaches 1024px, where should the face of the html element be placed? ! Sorry, in Microsoft's early assumptions, the body element represented the document (a strong evidence of this is that in quirks mode, the scroll bar of the web page is located in the body element). The model-accurate model, along with the various "standards" promoted by the losers in Firefox that have no market share, are all supported by Microsoft with great reluctance. Look, does this cumbersome and silly name like documentElement look like it was given by Microsoft? ! If it were Microsoft, it should be called html, as concise as document.boy. It turns out that in standard mode, when we select scrollLeft, we need to use document.documentElement.scrollLeft, because there is no scroll bar on the body at this time; in quirks mode, we need to use document.body, although Microsoft added document through patching. documentElement (that's a mouthful, no wonder Netscape failed), but the position of the scroll bar doesn't just change.
http://www.jb51.net/article/21719.htm