DateFormat PHP Class (php 处理日期)
代码:
<?php class FormatDate { var $theTime; function FormatDate($string) { //Set constructor $this->theTime = $string; } //Returns numerical day function Day() { return date("j", $this->theTime); } //Returns weekday function WeekDay() { return date("l", $this->theTime); } //Returns full month function Month() { return date("F", $this->theTime); } //Returns short-hand month function MonthShort() { return date("M", $this->theTime); } //Numeric for month function MonthNum() { return date("n", $this->theTime); } //Full 4 digit year function YearFull() { return date("Y", $this->theTime); } //Short 2 digit year function Year() { return date("y", $this->theTime); } //24 Hr with Seconds function MilitaryFull() { return date("G:i:s", $this->theTime); } //24 Hr without Seconds function Military() { return date("G:i", $this->theTime); } //Standard with seconds function StandardFull() { return date("g:i:s a", $this->theTime); } //Standard without seconds function Standard() { return date("g:i a", $this->theTime); } //Date & Month & Year Full function TextDate() { $string = $this->Month()." ".$this->Day()." ".$this->YearFull(); return $string; } //Date & Month & Year Shorthand function TextDateShort(){ $string = $this->MonthShort()." ".$this->Day()." ".$this->Year(); return $string; } //Numerical Date & Month & Year function NumDate() { $string = $this->MonthNum()."/".$this->Day()."/".$this->YearFull(); return $string; } //Numerical Date & Month & Year Shorthand function NumDateShort() { $string = $this->MonthNum()."/".$this->Day()."/".$this->Year(); return $string; } //Month & Day Full function MonthDay() { $string = $this->Month()." ".$this->Day(); return $string; } //Month & Day Short function MonthDayShort(){ $string = $this->MonthShort()." ".$this->Day(); return $string; } function TimeSince($old_stamp) { $difference = $this->theTime - $old_stamp; $loop = true; while($loop) { if(round($difference/3153600, 2) >= 1) { return "Over a year..."; } elseif(round($difference/2592000, 2) >= 2) { return "Over ".round($difference/2592000,0)." months ago..."; } elseif(round($difference/2592000, 2) >= 1.20) { return "Over a month ago..."; } elseif(round($difference/604800, 2) >= 2) { return "Over ".round($difference/604800,0)." weeks ago.."; } elseif(round($difference/604800, 2) >= 1.20) { return "Over a week ago.."; } elseif(round($difference/86400, 2) >= 1.9) { return "Over a few days ago...";} elseif(round($difference/3600, 2) >= 3) { return "Just a few hours ago.."; } elseif(round($difference/3600, 2) >= 8) { return "About half a day ago..."; } elseif(round($difference/3600, 2) ? <p>实例:</p> <pre name="code" class="php">$date = new FormatDate(time()); echo $date->Day().'<br>'; // 2 echo $date->WeekDay().'<br>'; // Tuesday echo $date->Month().'<br>'; // August echo $date->MonthShort().'<br>'; // Aug echo $date->MonthNum().'<br>'; // 8 echo $date->YearFull().'<br>'; // 2011 echo $date->Year().'<br>'; // 11 echo $date->MilitaryFull().'<br>'; // 9:08:40 echo $date->Military().'<br>'; // 9:08 echo $date->StandardFull().'<br>'; // 9:08:40 am echo $date->Standard().'<br>'; // 9:08 am echo $date->TextDate().'<br>'; // August 2 2011 echo $date->TextDateShort().'<br>'; // Aug 2 11 echo $date->NumDate().'<br>'; // 8/2/2011 echo $date->NumDateShort().'<br>'; // 8/2/11 echo $date->MonthDay().'<br>'; // August 2 echo $date->MonthDayShort().'<br>'; // Aug 2 echo $date->TimeSince(time()).'<br>'; // Less than an hour ago...
?
DateFormat Class Documentation
Initialize Class
$date = new FormatDate(time());
Numerical Day
$date->Day();
Text Day
$date->WeekDay();
Month (Full)
$date->Month();
Month (Short)
$date->MonthShort();
Month (Numerical)
$date->MonthNum();
Year (Full)
$date->YearFull();
Year (Short)
$date->Year();
Military (Seconds)
$date->MilitaryFull();
Military (No seconds)
$date->Military();
Standard (Full)
$date->StandardFull();
Standard
$date->Standard();
Text Date (Full)
$date->TextDate();
Text Date (Short)
$date->TextDateShort();
Numerical Date (Full)
$date->NumDate();
Numerical Date (Short)
$date->NumDateShort();
Month and Day (Full)
$date->MonthDay();
Month and Day (Short)
$date->MonthDayShort();
Time Since
$date->TimeSince($timestamp);
?
格式: http://php.net/manual/en/function.date.php
Day | --- | --- |
d | Day of the month, 2 digits with leading zeros | 01 to 31 |
D | A textual representation of a day, three letters | Mon through Sun |
j | Day of the month without leading zeros | 1 to 31 |
l (lowercase 'L') | A full textual representation of the day of the week | Sunday through Saturday |
N | ISO-8601 numeric representation of the day of the week (added in PHP 5.1.0) | 1 (for Monday) through 7 (for Sunday) |
S | English ordinal suffix for the day of the month, 2 characters | st , nd , rd or th . Works well with j |
w | Numeric representation of the day of the week | 0 (for Sunday) through 6 (for Saturday) |
z | The day of the year (starting from 0) | 0 through 365 |
Week | --- | --- |
W | ISO-8601 week number of year, weeks starting on Monday (added in PHP 4.1.0) | Example: 42 (the 42nd week in the year) |
Month | --- | --- |
F | A full textual representation of a month, such as January or March | January through December |
m | Numeric representation of a month, with leading zeros | 01 through 12 |
M | A short textual representation of a month, three letters | Jan through Dec |
n | Numeric representation of a month, without leading zeros | 1 through 12 |
t | Number of days in the given month | 28 through 31 |
Year | --- | --- |
L | Whether it's a leap year | 1 if it is a leap year, 0 otherwise. |
o | ISO-8601 year number. This has the same value as Y , except that if the ISO week number (W ) belongs to the previous or next year, that year is used instead. (added in PHP 5.1.0) | Examples: 1999 or 2003 |
Y | A full numeric representation of a year, 4 digits | Examples: 1999 or 2003 |
y | A two digit representation of a year | Examples: 99 or 03 |
Time | --- | --- |
a | Lowercase Ante meridiem and Post meridiem | am or pm |
A | Uppercase Ante meridiem and Post meridiem | AM or PM |
B | Swatch Internet time | 000 through 999 |
g | 12-hour format of an hour without leading zeros | 1 through 12 |
G | 24-hour format of an hour without leading zeros | 0 through 23 |
h | 12-hour format of an hour with leading zeros | 01 through 12 |
H | 24-hour format of an hour with leading zeros | 00 through 23 |
i | Minutes with leading zeros | 00 to 59 |
s | Seconds, with leading zeros | 00 through 59 |
u | Microseconds (added in PHP 5.2.2) | Example: 654321 |
Timezone | --- | --- |
e | Timezone identifier (added in PHP 5.1.0) | Examples: UTC , GMT , Atlantic/Azores |
I (capital i) | Whether or not the date is in daylight saving time | 1 if Daylight Saving Time, 0 otherwise. |
O | Difference to Greenwich time (GMT) in hours | Example: +0200 |
P | Difference to Greenwich time (GMT) with colon between hours and minutes (added in PHP 5.1.3) | Example: +02:00 |
T | Timezone abbreviation | Examples: EST , MDT ... |
Z | Timezone offset in seconds. The offset for timezones west of UTC is always negative, and for those east of UTC is always positive. | -43200 through 50400 |
Full Date/Time | --- | --- |
c | ISO 8601 date (added in PHP 5) | 2004-02-12T15:19:21+00:00 |
r | ??RFC 2822 formatted date | Example: Thu, 21 Dec 2000 16:01:07 +0200 |
U | Seconds since the Unix Epoch (January 1 1970 00:00:00 GMT) | See also time() |
Unrecognized characters in the format string will be printed as-is. The Z format will always return 0 when using gmdate() .
Note :
Since this function only accepts integer timestamps the u format character is only useful when using the date_format() function with user based timestamps created with date_create() .
timestamp
The optional timestamp
parameter is an
integer
Unix timestamp that defaults to the current
local time if a timestamp
is not given. In other
words, it defaults to the value of time()
.
?
?
?
?
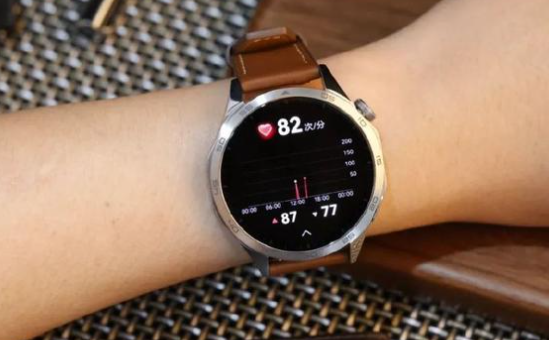
许多用户在选择智能手表的时候都会选择的华为的品牌,其中华为GT3pro和GT4都是非常热门的选择,不少用户都很好奇华为GT3pro和GT4有什么区别,下面就就给大家介绍一下二者。华为GT3pro和GT4有什么区别一、外观GT4:46mm和41mm,材质是玻璃表镜+不锈钢机身+高分纤维后壳。GT3pro:46.6mm和42.9mm,材质是蓝宝石玻璃表镜+钛金属机身/陶瓷机身+陶瓷后壳二、健康GT4:采用最新的华为Truseen5.5+算法,结果会更加的精准。GT3pro:多了ECG心电图和血管及安
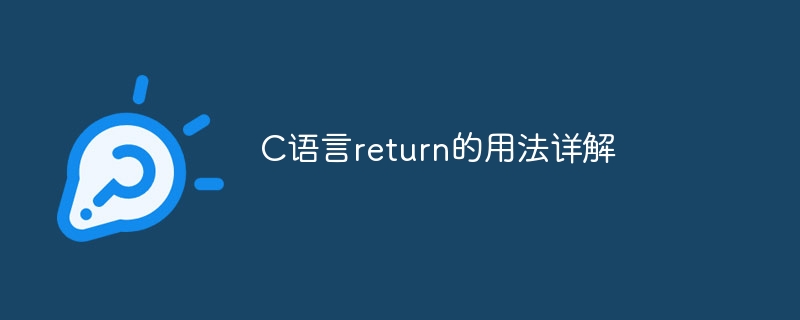
C语言return的用法有:1、对于返回值类型为void的函数,可以使用return语句来提前结束函数的执行;2、对于返回值类型不为void的函数,return语句的作用是将函数的执行结果返回给调用者;3、提前结束函数的执行,在函数内部,我们可以使用return语句来提前结束函数的执行,即使函数并没有返回值。
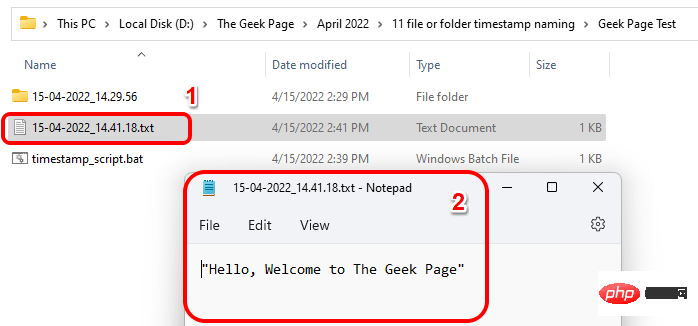
如果您正在寻找根据系统时间戳自动创建文件和文件夹并为其命名的方法,那么您来对地方了。有一种超级简单的方法可以用来完成这项任务。然后,创建的文件夹或文件可用于各种目的,例如存储文件备份、根据日期对文件进行排序等。在本文中,我们将通过一些非常简单的步骤解释如何在Windows11/10中自动创建文件和文件夹,并根据系统的时间戳对其进行命名。使用的方法是批处理脚本,非常简单。希望你喜欢阅读这篇文章。第1节:如何根据系统当前时间戳自动创建文件夹并命名第1步:首先,导航到要在其中创建文件夹的父文件夹,
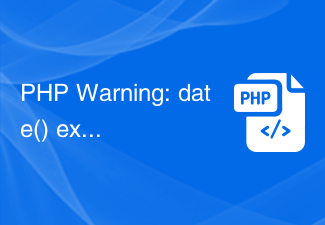
在使用PHP程序开发时,经常会碰到一些警告或者错误的提示信息。其中,可能出现的一个错误提示就是:PHPWarning:date()expectsparameter2tobelong,stringgiven。这个错误的提示信息意思是:函数date()的第二个参数期望是长整型(long),但是实际传递给它的是字符串(string)。那么,我们
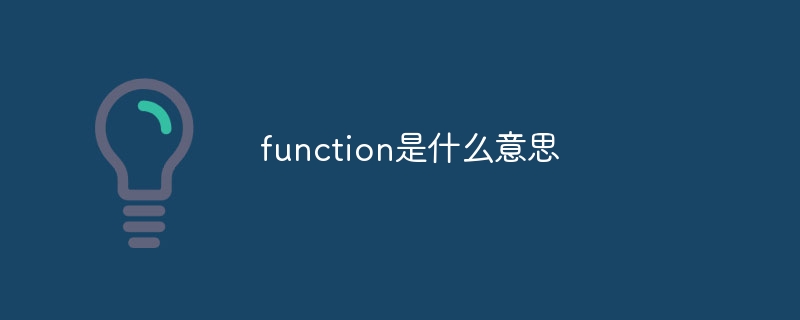
function是函数的意思,是一段具有特定功能的可重复使用的代码块,是程序的基本组成单元之一,可以接受输入参数,执行特定的操作,并返回结果,其目的是封装一段可重复使用的代码,提高代码的可重用性和可维护性。
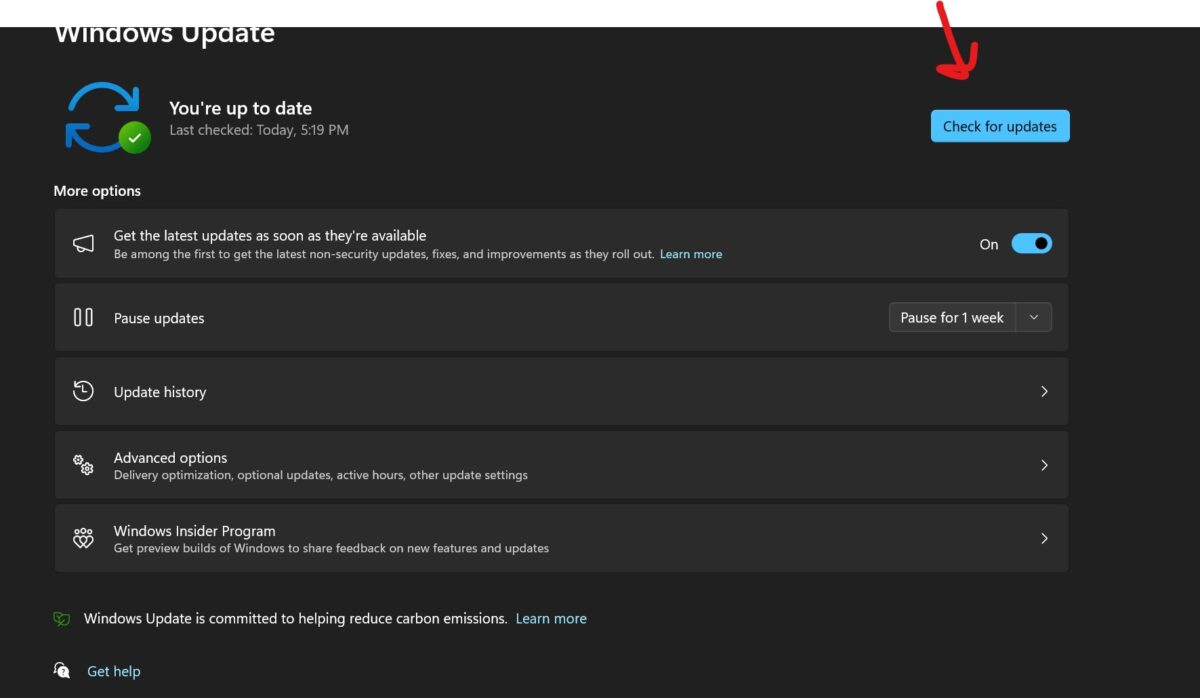
为什么截图工具在Windows11上不起作用了解问题的根本原因有助于找到正确的解决方案。以下是截图工具可能无法正常工作的主要原因:对焦助手已打开:这可以防止截图工具打开。应用程序损坏:如果截图工具在启动时崩溃,则可能已损坏。过时的图形驱动程序:不兼容的驱动程序可能会干扰截图工具。来自其他应用程序的干扰:其他正在运行的应用程序可能与截图工具冲突。证书已过期:升级过程中的错误可能会导致此issu简单的解决方案这些适合大多数用户,不需要任何特殊的技术知识。1.更新窗口和Microsoft应用商店应用程
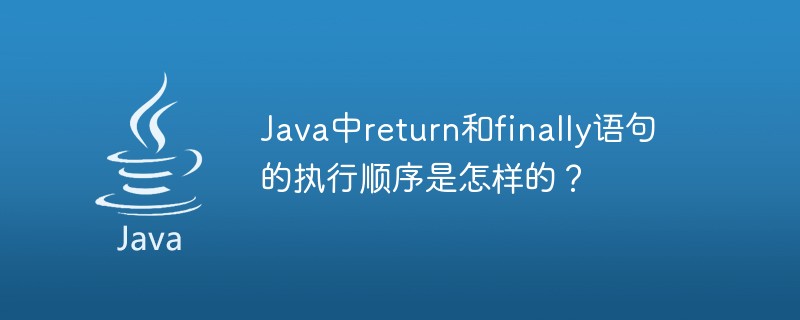
源码:publicclassReturnFinallyDemo{publicstaticvoidmain(String[]args){System.out.println(case1());}publicstaticintcase1(){intx;try{x=1;returnx;}finally{x=3;}}}#输出上述代码的输出可以简单地得出结论:return在finally之前执行,我们来看下字节码层面上发生了什么事情。下面截取case1方法的部分字节码,并且对照源码,将每个指令的含义注释在
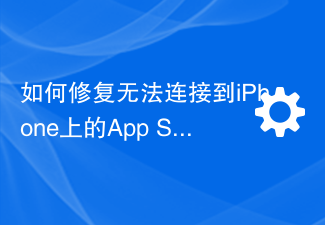
第1部分:初始故障排除步骤检查苹果的系统状态:在深入研究复杂的解决方案之前,让我们从基础知识开始。问题可能不在于您的设备;苹果的服务器可能会关闭。访问Apple的系统状态页面,查看AppStore是否正常工作。如果有问题,您所能做的就是等待Apple修复它。检查您的互联网连接:确保您拥有稳定的互联网连接,因为“无法连接到AppStore”问题有时可归因于连接不良。尝试在Wi-Fi和移动数据之间切换或重置网络设置(“常规”>“重置”>“重置网络设置”>设置)。更新您的iOS版本:


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
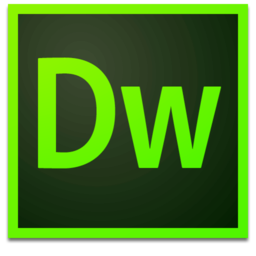
Dreamweaver Mac version
Visual web development tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SublimeText3 Chinese version
Chinese version, very easy to use

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
