


All JS functions have a prototype attribute, which refers to an object, the prototype object, also referred to as the prototype. This function includes constructors and ordinary functions. We are talking more about the prototype of the constructor, but we cannot deny that ordinary functions also have prototypes. For example, an ordinary function:
function F(){
alert(F.prototype instanceof Object) //true;
}
Constructor, that is, constructing an object. First, let’s understand the process of instantiating an object through the constructor.
function A(x){
this.x =x;
}
var obj=new A(1);
There are three steps to instantiate the obj object:
1. Create the obj object: obj=new Object();
2. Point the internal __proto__ of obj to the prototype of the function A that constructs it. At the same time, obj.constructor===A.prototype.constructor (This is always true, even if A.prototype no longer points to the original A prototype, that is to say: the constructor property of the instance object of the class always points to the prototype.constructor of the "constructor"), thus making obj.constructor.prototype point to A.prototype (obj.constructor.prototype===A.prototype, this is not true when A.prototype changes, as will be encountered below). obj.constructor.prototype and the internal _proto_ are two different things. _proto_ is used when instantiating an object. obj does not have a prototype attribute, but it has an internal __proto__. You can use __proto__ to obtain the prototype attributes on the prototype chain. and prototype methods, FireFox exposes __proto__, which can be alerted in FireFox (obj.__proto__);
3. Use obj as this to call constructor A to set members (i.e. object properties and object methods) and initialized.
When these three steps are completed, the obj object has no connection with the constructor A. At this time, even if the constructor A adds any members, it will no longer affect the instantiated obj object. At this time, the obj object has the x attribute and all members of the prototype object of constructor A. Of course, the prototype object has no members at this time.
The prototype object is initially empty, that is, it does not have a member (ie prototype properties and prototype methods). You can verify how many members a prototype object has by using the following method.
var num=0;
for(o in A.prototype) {
alert(o);//alert outputs the prototype attribute name
num ;
}
alert("member: " num);//alert outputs the number of all members of the prototype .
However, once prototype properties or prototype methods are defined, all objects instantiated through the constructor inherit these prototype properties and prototype methods, which is done internally The _proto_ chain is implemented.
For example:
A.prototype.say=function(){alert("Hi")};
Then all A objects have a say method, this The say method of the prototype object is the only copy shared by everyone, rather than every object having a copy of the say method.
2. Prototype and inheritance
First, let’s look at a simple inheritance implementation.
function A(x){
this.x =x;
}
function B(x,y){
this.tmpObj=A;
this.tmpObj(x);
delete this.tmpObj;
this. y=y;
}
Lines 5, 6, and 7: Create a temporary attribute tmpObj to reference constructor A, then execute it inside B, and delete it after execution. When this.x=x is executed inside B (this here is the object of B), B will of course have the x attribute. Of course, the x attribute of B and the x attribute of A are independent, so they are not strictly inheritance. Lines 5, 6, and 7 have a simpler implementation, which is through the call(apply) method: A.call(this,x);
Both methods pass this to the execution of A , this points to the object of B, which is why A(x) is not used directly. This inheritance method is class inheritance (js does not have classes, here it only refers to constructors). Although it inherits all the attribute methods of A's constructed object, it cannot inherit the members of A's prototype object. To achieve this goal, we need to add prototypal inheritance on this basis.
Through the following examples, you can have a deep understanding of prototypes and the perfect inheritance that prototypes participate in. (The core of this article is here^_^)
function A( x){
this.x = x;
}
A.prototype.a = "a";
function B(x,y){
this.y = y;
A.call(this,x);
}
B.prototype.b1 = function(){
alert("b1");
}
B.prototype = new A();
B.prototype.b2 = function(){
alert("b2");
}
B.prototype.constructor = B;
var obj = new B (1,3);
This example is about B inheriting A. Line 7 class inheritance: A.call(this.x); As mentioned above. Prototypal inheritance is implemented in line 12: B.prototype = new A();
That means that the prototype of B points to an instance object of A. This instance object has the x attribute, which is undefined. Has an attribute with a value of "a". So the B prototype also has these two attributes (or in other words, B and A have established a prototype chain, and B is a subordinate of A). Because of the class inheritance just now, the instance object of B also has the x attribute, which means that the obj object has two x attributes with the same name. At this time, the prototype attribute x has to give way to the instance object attribute x, so obj.x is 1 , rather than undefined. Line 13 also defines the prototype method b2, so the B prototype also has b2. Although the prototype method b1 is set in lines 9 to 11, you will find that after the execution of line 12, the B prototype no longer has the b1 method, that is, obj.b1 is undefined. Because line 12 changes the B prototype pointer, the original prototype object with b1 is abandoned, and naturally there is no b1.
After line 12 is executed, B prototype (B.prototype) points to the instance object of A, and the constructor of the instance object of A is constructor A, so B.prototype.constructor is the constructor object A. (In other words, A constructs the prototype of B).
alert(B.prototype.constructor) comes out as "function A(x){...}". Similarly, obj.constructor is also an A constructor object. After alert(obj.constructor) comes out, it is "function A(x){...}", that is to say, B.prototype.constructor===obj.constructor(true) , but B.prototype===obj.constructor.prototype(false), because the former is the prototype of B and has members: x, a, b2, and the latter is the prototype of A and has members: a. How to fix this problem? On line 16, redirect the constructor of B prototype to the B constructor, then B.prototype===obj.constructor.prototype(true), all have members: x, a, b2 .
If there is no line 16, will obj = new B(1,3) call the A constructor for instantiation? The answer is no, you will find that obj.y=3, so it is still instantiated by the called B constructor. Although obj.constructor===A(true), for the behavior of new B(), the three steps mentioned above to create an instance object through the constructor are performed. The first step is to create an empty object; the second step is to create an empty object. Step, obj.__proto__ === B.prototype, B.prototype has x, a, b2 members, obj.constructor points to B.prototype.constructor, that is, constructor A; the third step, called constructor B To set and initialize members, have attributes x, y. Although not adding 16 lines does not affect the properties of obj, as mentioned in the previous paragraph, it does affect obj.constructor and obj.constructor.prototype. Therefore, after using prototypal inheritance, correction operations must be performed.
Regarding lines 12 and 16, in short, line 12 makes the prototype of B inherit all members of the prototype object of A, but also makes the prototype of the constructor of the instance object of B point to the prototype of A. So this flaw needs to be corrected through line 16.
Finished.
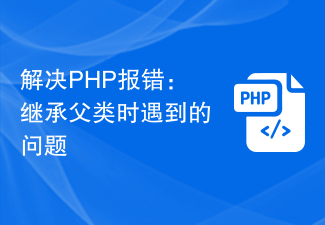
解决PHP报错:继承父类时遇到的问题在PHP中,继承是一种重要的面向对象编程的特性。通过继承,我们能够重用已有的代码,并且能够在不修改原有代码的情况下,对其进行扩展和改进。尽管继承在开发中应用广泛,但有时候在继承父类时可能会遇到一些报错问题,本文将围绕解决继承父类时遇到的常见问题进行讨论,并提供相应的代码示例。问题一:未找到父类在继承父类的过程中,如果系统无
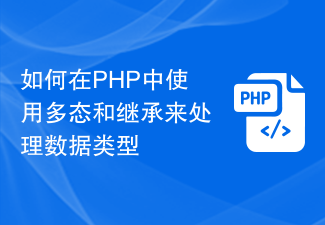
如何在PHP中使用多态和继承来处理数据类型引言:在PHP中,多态和继承是两个重要的面向对象编程(OOP)概念。通过使用多态和继承,我们可以更加灵活地处理不同的数据类型。本文将介绍如何在PHP中使用多态和继承来处理数据类型,并通过代码示例展示它们的实际应用。一、继承的基本概念继承是面向对象编程中的一种重要概念,它允许我们创建一个类,该类可以继承父类的属性和方法
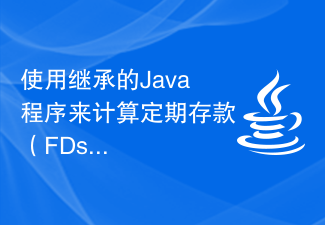
继承是一个概念,它允许我们从一个类访问另一个类的属性和行为。被继承方法和成员变量的类被称为超类或父类,而继承这些方法和成员变量的类被称为子类或子类。在Java中,我们使用“extends”关键字来继承一个类。在本文中,我们将讨论使用继承来计算定期存款和定期存款的利息的Java程序。首先,在您的本地机器IDE中创建这四个Java文件-Acnt.java−这个文件将包含一个抽象类‘Acnt’,用于存储账户详情,如利率和金额。它还将具有一个带有参数‘amnt’的抽象方法‘calcIntrst’,用于计
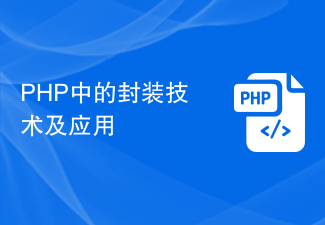
PHP中的封装技术及应用封装是面向对象编程中的一个重要概念,它指的是将数据和对数据的操作封装在一起,以便提供对外部程序的统一访问接口。在PHP中,封装可以通过访问控制修饰符和类的定义来实现。本文将介绍PHP中的封装技术及其应用场景,并提供一些具体的代码示例。一、封装的访问控制修饰符在PHP中,封装主要通过访问控制修饰符来实现。PHP提供了三个访问控制修饰符,
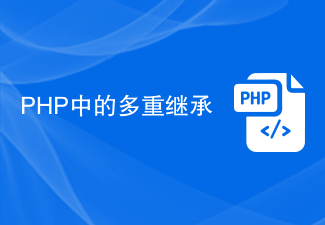
继承:继承是面向对象编程(OOP)中的一个基本概念,它允许类从其他类继承属性和行为。它是一种基于现有类创建新类的机制,促进代码重用并建立类之间的层次关系。继承基于"父子"或"超类-子类"关系的概念。从中继承的类被称为超类或基类,而继承超类的类被称为子类或派生类。子类继承其超类的所有属性(变量)和方法(函数),还可以添加自己独特的属性和方法或覆盖继承的属性和方法继承的类型在面向对象编程(OOP)中,继承是一个基本概念,它允许类从其他类中继承属性和行为。它促进
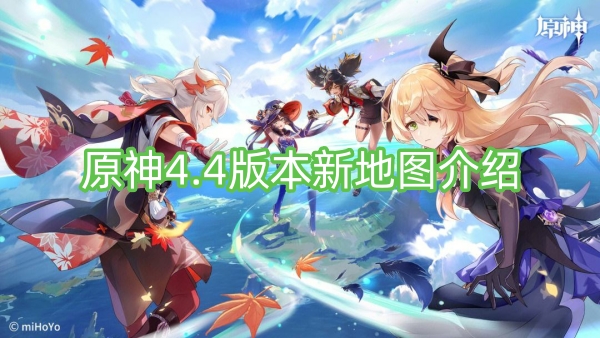
原神4.4版本新地图介绍,小伙伴们原神这次4.4版本也是迎来了璃月的海灯节,同时将在4.4版本推出一个新的地图区域,名为沉玉谷。根据提供的信息,沉玉谷实际上是翘英庄的一部分,但玩家更习惯称其为沉玉谷。下面就让小编来给大家介绍一下新地图吧。原神4.4版本新地图介绍4.4版本将开放璃月北部的「沉玉谷·上谷」、「沉玉谷·南陵」和「来歆山」,在「沉玉谷·上谷」已为旅行者开启传送锚点。※完成魔神任务序章·第三幕巨龙与自由之歌」后,将自动解锁该传送锚点。二、翘英庄当春日温煦的柔风再度抚过沉玉的山野,那馥郁的
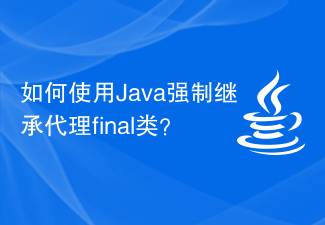
如何使用Java强制继承代理final类?在Java中,final关键字用于修饰类、方法和变量,表示它们不可被继承、重写和修改。然而,在某些情况下,我们可能需要强制继承一个final类,以实现特定的需求。本文将讨论如何使用代理模式来实现这样的功能。代理模式是一种结构型设计模式,它允许我们创建一个中间对象(代理对象),该对象可以控制对另一个对象(被代理对象)的
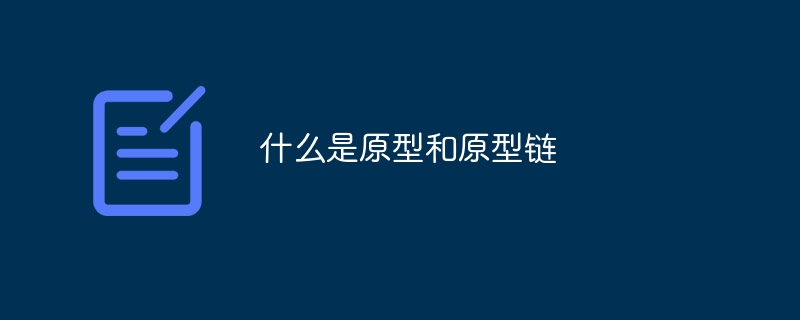
原型,js中的一个对象,用于定义其他对象的属性和方法,每个构造函数都有一个prototype属性,这个属性是一个指针,指向一个原型对象,当创建新对象时,这个新对象会从其构造函数的prototype属性继承属性和方法。原型链,当试图访问一个对象的属性时,js会首先检查这个对象是否有这个属性,如果没有,那么js会转向这个对象的原型,如果原型对象也没有这个属性,会继续查找原型的原型。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
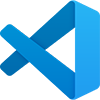
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

Notepad++7.3.1
Easy-to-use and free code editor
