


We have already used the prototype attribute in Chapter 1 to simulate the implementation of classes and inheritance. The prototype property is essentially a JavaScript object. And every function has a default prototype attribute.
If this function is used in the scenario of creating a custom object, we call this function a constructor. For example, a simple scenario below:
//Constructor
function Person(name) {
this.name = name;
}
// Define the prototype of Person. The attributes in the prototype can be referenced by custom objects
Person.prototype = {
getName: function() {
return this.name;
}
}
var zhang = new Person("ZhangSan");
console.log(zhang.getName() ); // "ZhangSan"
As an analogy, let's consider the data types in JavaScript - String, Number, Array, Object, Date (Date) etc. We have reason to believe that these types are implemented as constructors inside JavaScript, such as:
// Define the constructor of the array, as a predefined type of JavaScript
function Array() {
// ...
}
// Instance of initializing array
var arr1 = new Array(1, 56, 34, 12);
// However, we prefer the following syntax definition:
var arr2 = [1, 56, 34 , 12];
Many methods of operating on arrays at the same time (such as concat, join, push) should also be defined in the prototype attribute.
In fact, all intrinsic data types of JavaScript have read-only prototype attributes (this is understandable: because if the prototype attributes of these types are modified, the predefined methods disappear), but we can Add your own extension methods to it.
//Extend one to the JavaScript inherent type Array to get the minimum value Method of
Array.prototype.min = function() {
var min = this[0];
for (var i = 1; i if (this[i] min = this[i];
}
}
return min;
};
// on any instance of Array Call the min method
console.log([1, 56, 34, 12].min()); // 1
Note: There is a trap here, add it to the prototype of Array After the extension method, when using for-in to loop the array, this extension method will also be looped out.
The following code illustrates this (assuming that the min method has been extended to the Array prototype):
var arr = [1, 56, 34, 12];
var total = 0;
for (var i in arr) {
total = parseInt( arr[i], 10);
}
console.log(total); // NaN
The solution is also very simple:
var arr = [1, 56, 34, 12];
var total = 0;
for (var i in arr) {
if (arr.hasOwnProperty(i)) {
total = parseInt(arr[i], 10);
}
}
console .log(total); // 103
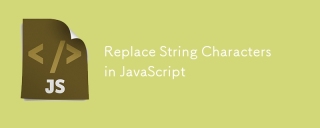
Detailed explanation of JavaScript string replacement method and FAQ This article will explore two ways to replace string characters in JavaScript: internal JavaScript code and internal HTML for web pages. Replace string inside JavaScript code The most direct way is to use the replace() method: str = str.replace("find","replace"); This method replaces only the first match. To replace all matches, use a regular expression and add the global flag g: str = str.replace(/fi
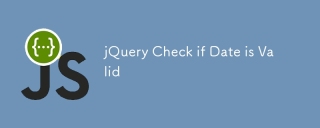
Simple JavaScript functions are used to check if a date is valid. function isValidDate(s) { var bits = s.split('/'); var d = new Date(bits[2] '/' bits[1] '/' bits[0]); return !!(d && (d.getMonth() 1) == bits[1] && d.getDate() == Number(bits[0])); } //test var
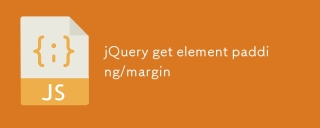
This article discusses how to use jQuery to obtain and set the inner margin and margin values of DOM elements, especially the specific locations of the outer margin and inner margins of the element. While it is possible to set the inner and outer margins of an element using CSS, getting accurate values can be tricky. // set up $("div.header").css("margin","10px"); $("div.header").css("padding","10px"); You might think this code is
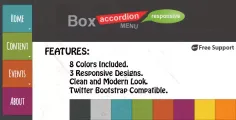
This article explores ten exceptional jQuery tabs and accordions. The key difference between tabs and accordions lies in how their content panels are displayed and hidden. Let's delve into these ten examples. Related articles: 10 jQuery Tab Plugins
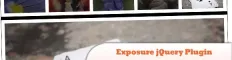
Discover ten exceptional jQuery plugins to elevate your website's dynamism and visual appeal! This curated collection offers diverse functionalities, from image animation to interactive galleries. Let's explore these powerful tools: Related Posts: 1
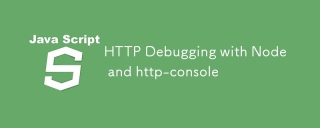
http-console is a Node module that gives you a command-line interface for executing HTTP commands. It’s great for debugging and seeing exactly what is going on with your HTTP requests, regardless of whether they’re made against a web server, web serv
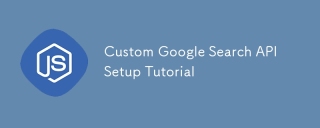
This tutorial shows you how to integrate a custom Google Search API into your blog or website, offering a more refined search experience than standard WordPress theme search functions. It's surprisingly easy! You'll be able to restrict searches to y
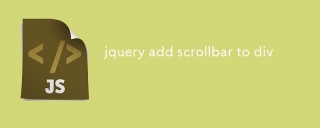
The following jQuery code snippet can be used to add scrollbars when the div content exceeds the container element area. (No demonstration, please copy it directly to Firebug) //D = document //W = window //$ = jQuery var contentArea = $(this), wintop = contentArea.scrollTop(), docheight = $(D).height(), winheight = $(W).height(), divheight = $('#c


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
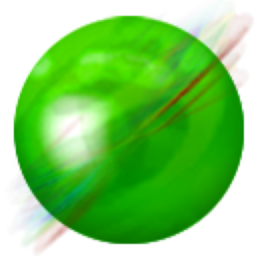
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
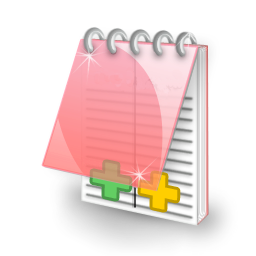
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Atom editor mac version download
The most popular open source editor
