Scope allocation and variable access rules
In ECMAScript, functions are also objects. Function objects are created from a function declaration during variable instantiation, or when a function expression is evaluated or the Function constructor is called. (For information on 'function objects', please see "Understanding Javascript_08_Function Objects"). Every function object has an internal [[scope]] property, which is also composed of a list (chain) of objects. The internal [[scope]] attribute refers to the scope chain of the execution environment in which they were created, and the active objects of the current execution environment are added to the top of the object list. When we access a variable inside a function, it is actually the process of finding the variable on the scope chain.
It’s too theoretical (summarizing it to death!), let’s take a look at a piece of code:
The following figure clearly shows the memory allocation and scope allocation of the above code:
Let’s explain it below:
1. Load the code and create a global execution environment. At this time, the outer variable will be added to the variable object (window), which points to the function object. outer, at this time there is only the window object in the scope chain.
2. Execute the code. When the program executes outer(), it will look for the outer variable in the global object and call it successfully.
3. Create the execution environment of outer. At this time, a new active object will be created, add variable i, set the value to 10, add variable inner, point to the function object inner. And push the active object into the scope chain. And point the [[scope]] attribute of the function object outer to the active object outer. At this time, the scope chain is outer's active object window.
4. Execute the code and successfully assign a value to i. When the program executes inner(), it will look for the inner variable in [[scope]] of the function object outer. Called successfully after finding.
5. Create the execution environment of inner, create a new active object, add variable j, assign the value to 100, and push the active object into the scope chain, and point the [[scope]] attribute of the function object inner to the active object inner. At this time, the scope chain is: inner's active object and outer's active object global object.
6. The execution code assigns a value to j. When accessing i and j, the corresponding value is successfully found in the scope and output, and When accessing the variable adf, it was not found in the scope and an access error occurred.
Note: Through the memory graph, we will find that the scope chain and the prototype chain are so similar. This explains a lot of problems... (Be benevolent and wise, find out the answer for yourself!)
Principle of Closure
After we understand the problem of scope, the problem of closure is already very simple . What is a closure? A closure is an inner function that encloses the variables in the scope of the outer function.
Let’s look at a typical closure application: generate increment value
The outer anonymous function returns an inline function, and the inline function uses the local variable id of the outer anonymous function. Logically speaking, the local variables of the outer anonymous function go out of scope when returned, so the increment() call cannot be used. This is closure, that is, the function call returns an embedded function, and the embedded function refers to the local variables, parameters and other resources of the external function that should be closed (Close). What's going on? Let's find the answer:
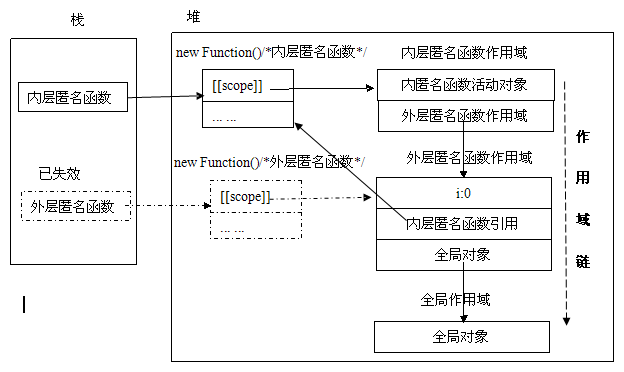
According to the understanding of Scope Chain, it can be explained that the returned inline function already holds the Scope Chain when it was constructed, although outer returns cause these objects to go out of scope. lifetime scope, but JavaScript uses automatic garbage collection to release object memory: it is checked periodically according to the rules and the object is released only if it does not have any references. So the above code runs correctly.
Reference:
http://www.cnblogs.com/RicCC/archive/2008/02/15/JavaScript-Object-Model-Execution-Model.html
http://www .cn-cuckoo.com/2007/08/01/understand-javascript-closures-72.html