


First of all, it is rare that this problem occurs because there are too many better solutions. When doing ajax today, an interesting thing is that a get request must be sent in each iteration. Because the iteration speed is too fast, the next iteration will be carried out before a request is completed. On chrome and ff, except for the last one Except for the request, all other requests have been cancelled. So what to do? Set a delay (not so good) or some other way?
There are many ways, such as setting sleep, iteration, etc. I use two other solutions.
1. Synchronous ajax request , and ajax request is asynchronous by default, so it should be set to false.
function creatXMLHttpRequest() { var xmlHttp; if (window.ActiveXObject) { return xmlHttp = new ActiveXObject("Microsoft.XMLHTTP"); } else if (window.XMLHttpRequest) { return xmlHttp = new XMLHttpRequest(); } } function disButton(name, actionName, resquestParmName) { var path = document.getElementById("path").value; var xmlHttp = creatXMLHttpRequest(); var invoiceIds = new Array(); invoiceIds = document.getElementsByName(name); // 迭代的速度快于发送请求+收到回复的时间 所以一次get请求都还没有完成就进行了下一次请求 for (i = 0; i < invoiceIds.length; i++) { var invoiceId = invoiceIds[i].value; var url = path + "/" + actionName + ".action?" + resquestParmName + "=" + invoiceId; xmlHttp.onreadystatechange = function() { if (xmlHttp.readyState == 4) { if (xmlHttp.status == 200) { var result = xmlHttp.responseText; if (result == "0") { document.getElementById("btn" + invoiceId).disabled = "disabled"; } } } } xmlHttp.open("GET", url, false); xmlHttp.send(null); } }
In this way, using synchronous ajax request, you will wait for the server to respond, execute the code, and then continue to iterate. But it seems that this is not recommended.
2. Use an asynchronous method , but remember that a new XMLHttpRequest object must be created for each iteration and cannot be reused.
function creatXMLHttpRequest() { var xmlHttp; if (window.ActiveXObject) { return xmlHttp = new ActiveXObject("Microsoft.XMLHTTP"); } else if (window.XMLHttpRequest) { return xmlHttp = new XMLHttpRequest(); } } function disButton(name, actionName, resquestParmName) { var xmlHttp; var path = document.getElementById("path").value; var invoiceIds = new Array(); invoiceIds = document.getElementsByName(name); // 迭代的速度快于发送请求+收到回复的时间 所以一次get请求都还没有完成就进行了下一次请求 for (i = 0; i < invoiceIds.length; i++) { xmlHttp = creatXMLHttpRequest(); var invoiceId = invoiceIds[i].value; var url = path + "/" + actionName + ".action?" + resquestParmName + "=" + invoiceId; fu(xmlHttp,url,invoiceId); } } function fu(xmlHttp,url,invoiceId){ xmlHttp.onreadystatechange = function() { if (xmlHttp.readyState == 4) { if (xmlHttp.status == 200) { var result = xmlHttp.responseText; if (result == "0") { document.getElementById("btn" + invoiceId).disabled = "disabled"; } } } } // xmlHttp.open("GET", url, true); xmlHttp.send(null); }
Since the JS for loop and ajax run asynchronously, the for loop ends but ajax has not yet been executed. If an asynchronous request method is used, if a new XMLHttpRequest is made during each iteration, each request can be completed, but the result is still inaccurate, and some programs have not been executed.
Understand, it turns out that each iteration is to execute a few lines of code. The code for sending asynchronous ajax requests should be placed in a function, and this function should be called every iteration. That's it.
In terms of performance, For this iterative ajax request, it seems that the synchronous method has higher performance.
This problem has been solved and my understanding of ajax and http has been deepened.
The above introduces the problem of sending AJAX requests in the JavaScript for loop. I hope it will be helpful to friends who are interested in Javascript tutorials.
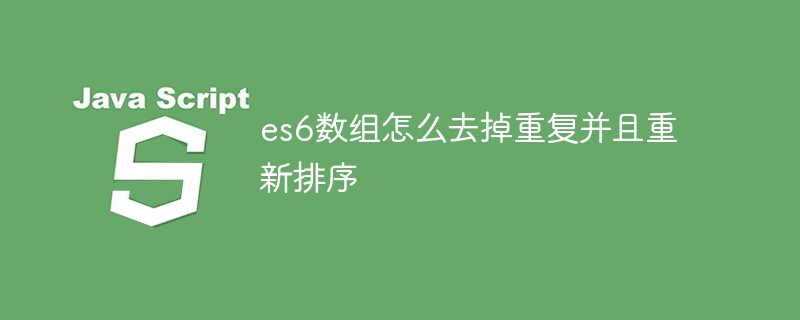
去掉重复并排序的方法:1、使用“Array.from(new Set(arr))”或者“[…new Set(arr)]”语句,去掉数组中的重复元素,返回去重后的新数组;2、利用sort()对去重数组进行排序,语法“去重数组.sort()”。
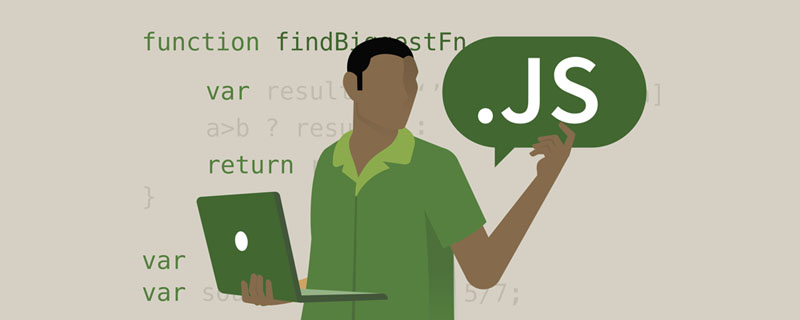
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于Symbol类型、隐藏属性及全局注册表的相关问题,包括了Symbol类型的描述、Symbol不会隐式转字符串等问题,下面一起来看一下,希望对大家有帮助。
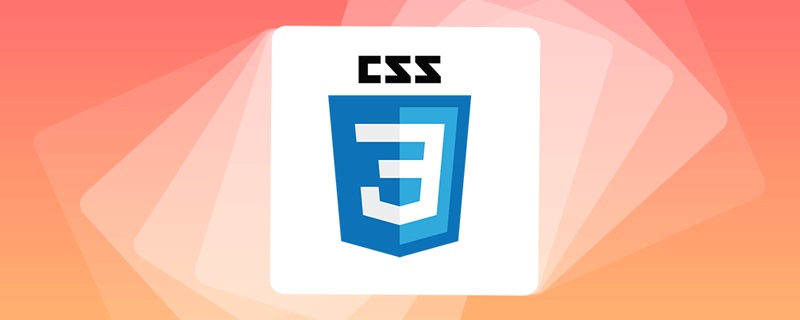
怎么制作文字轮播与图片轮播?大家第一想到的是不是利用js,其实利用纯CSS也能实现文字轮播与图片轮播,下面来看看实现方法,希望对大家有所帮助!
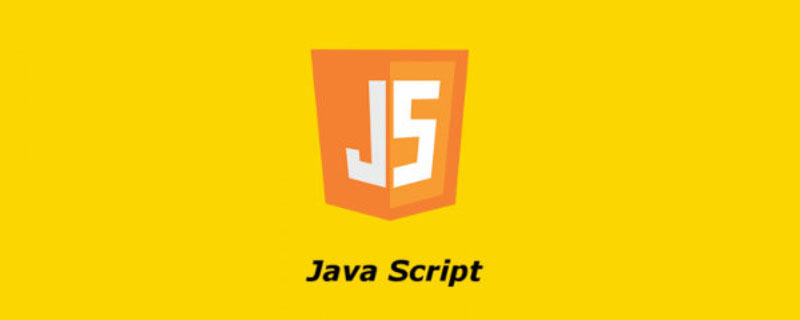
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于对象的构造函数和new操作符,构造函数是所有对象的成员方法中,最早被调用的那个,下面一起来看一下吧,希望对大家有帮助。
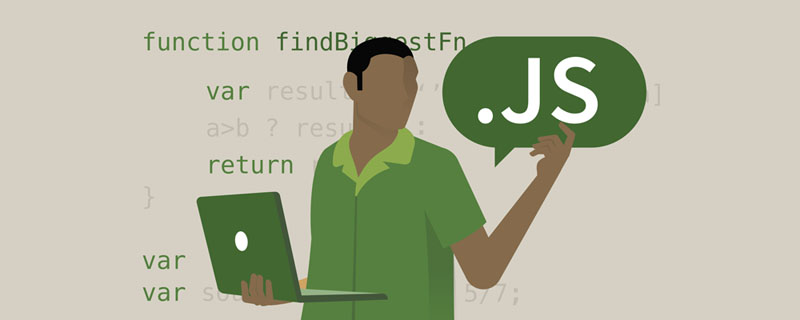
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于面向对象的相关问题,包括了属性描述符、数据描述符、存取描述符等等内容,下面一起来看一下,希望对大家有帮助。
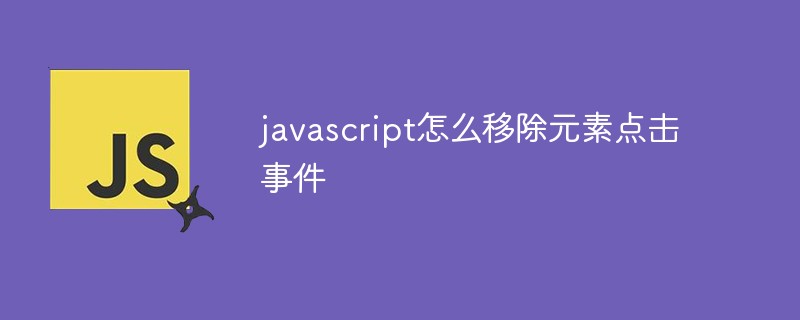
方法:1、利用“点击元素对象.unbind("click");”方法,该方法可以移除被选元素的事件处理程序;2、利用“点击元素对象.off("click");”方法,该方法可以移除通过on()方法添加的事件处理程序。
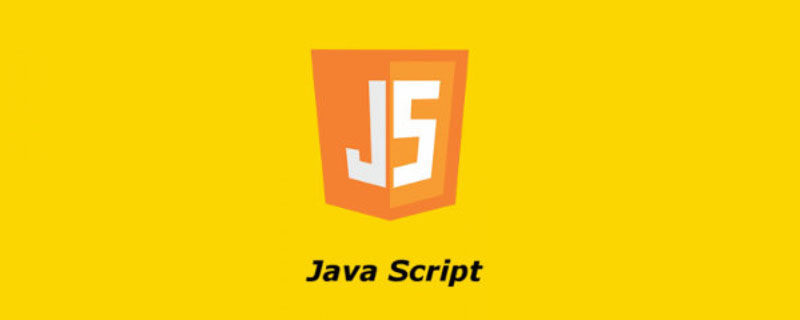
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于BOM操作的相关问题,包括了window对象的常见事件、JavaScript执行机制等等相关内容,下面一起来看一下,希望对大家有帮助。
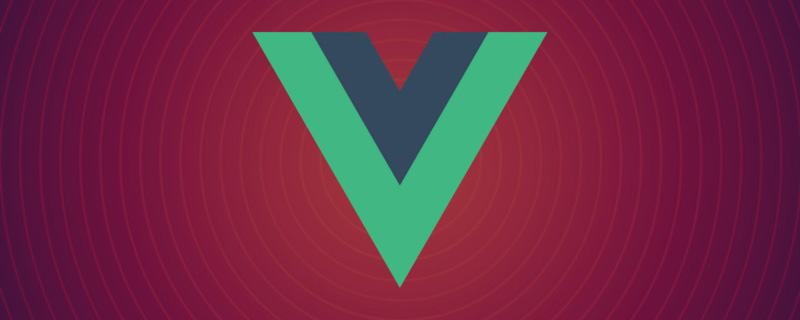
本篇文章整理了20+Vue面试题分享给大家,同时附上答案解析。有一定的参考价值,有需要的朋友可以参考一下,希望对大家有所帮助。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
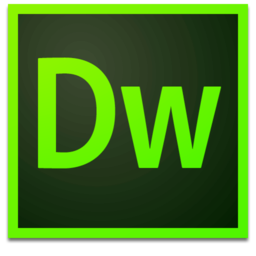
Dreamweaver Mac version
Visual web development tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
