If you want to get the DOM element after jQuery's $ call, you can use the get method, as follows
//Method 1
$('div').get(1); // Get the second div in the page
Of course, you can also use the array index method to get it
// Method 2
$('div' )[1]; // Get the second div in the page
Both of the above methods can get a specific DOM element, but to get the set of DOM elements, you need to use the toArray method
$('div').toArray(); // Return to page Put all the divs in the array one by one
Look at the source code of the get method
get: function( num ) {
return num == null ?
// Return a 'clean' array
this.toArray() :
// Return just the object
( num < 0 ? this[ this.length num ] : this[ num ] );
},
get just a three Meta operator, i.e. two branches.
Branch 1, when no parameters are passed, all DOM elements will be obtained (call toArray)
Branch 2, when num is a number (index), a specified DOM element will be returned (reverse acquisition when the number is negative)
Branch 1 actually converts the jQuery object (pseudo array) into a real array, and branch 2 actually takes the "pseudo array" elements (using square brackets []). The entire calling process is described below with $('div')
1. Get the page div element (document.getElementsByTagName('div'))
2. Convert the collection HTMLCollection/NodeList into a real array
3. Convert the array into a pseudo array/ArrayLike (jQuery object)
as shown in the figure
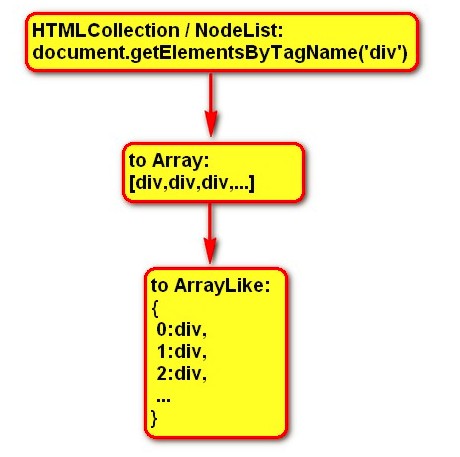
The first step is what the selector does;
The second step is to convert the HTMLCollection/NodeList into an array. This has been discussed before;
The third step is to convert the array into a pseudo array/ ArrayLike (jQuery object), just call the push of the array.
Maybe the following example is easy to understand
var jqObj = {0:'one',1:'two',2:'three',length:3}, // Pseudo array (ArrayLike)
ary = ['one','two','three']; //Array
// Convert pseudo array (ArrayLike) into array
function jqObjToArray(json){
var slice = Array.prototype.slice;
return slice.call(json,0) ;
}
// Convert the array into a pseudo array (ArrayLike)
function ArrayToJqObj(ary){
var obj = {}, push = Array.prototype.push;
push. apply(obj,ary);
return obj;
}
console.log(jqObjToArray(jqObj));
console.log(ArrayToJqObj(ary));
jQuery also provides first/last/eq/slice methods, which are different from the get/toArray mentioned above. They return jQuery objects instead of HTMLElements. The following html fragment
$('div').first() returns the first element of the jq object collection, that is, Div[id=a], structure: {0:div,length:1,...}; //. ..Indicates that other attributes are omitted
$('div').last() returns the last element of the jq object collection, that is, Div[id=d], structure: {0:div,length:1,.. .};
$('div').eq(2) returns the third element matched by the jq object, that is, Div[id=c], structure: {0:div,length:1,...} ;
Check the source code and find out:
1, the eq method is directly called in the first and last methods.
2. The slice method is called in the eq method.
So the slice method is fundamental. This method makes us naturally think of the slice method of the array. In fact, jQuery uses the push and slice methods of Array.
1, use the Array.prototype.slice method to convert the pseudo array into an array
2, use the Array.prototype.push method to convert the array into a pseudo array
Of course, the slice method in jQuery calls pushStack. I abbreviated slice here as follows
slice : function() {
var ret = $();//The key sentence is to construct a new jq object,
var ary = slice.apply(this,arguments);//This here is the jq object, according to the parameters Convert to array subset
push.apply(ret,ary);//Convert to jq object, that is, pseudo-array form
return ret;
},
Related:
Convert HTMLCollection/NodeList/pseudo array to array zChain-05.rar Statement:The content of this article is voluntarily contributed by netizens, and the copyright belongs to the original author. This site does not assume corresponding legal responsibility. If you find any content suspected of plagiarism or infringement, please contact admin@php.cn