Interface Inheritance Code Optimization Thoughts
Let me first share what I think is a very good js programming tip to achieve great code commonality! Because many js libraries will perform direct prototype extensions on native objects, this is a very bad habit. It not only increases the memory consumption of each new instance object, but also easily causes polluting misunderstandings (thinking that there is such a thing)! And this is also a guideline for building js libraries: as few prototype extensions as possible, especially for root-level objects!
JS Database Building Guidelines
JS Database Building Guidelines (Dean Edwards’s experience when developing base2) Translated version: http://biaoge.me/2009/12/239 A good place to learn about building a js library: http://ejohn.org/blog/building-a-javascript-library/ If you have time, take a look at a super cutting-edge document on building a js library, including css3, the latest browser API (such as querySelector) build-a-javascript-framework
Use inheritance to improve code commonality
Because it does not extend on the native object, an external namespace is needed, and in this object There will be some interfaces for external calls, and in order to improve the robustness of the js library itself, it is necessary to minimize the external interfaces (the ideal is to only save the interfaces that users need)! So there is a problem here, let me instantiate it:
var namespace={
IOfirst:function(){},
IOsecond:function(){}
}
There is something under the object namespace that needs to be given to IOfirst Shared with IOsecond without exposing this interface! I wrap all external interfaces through a parent interface through inheritance, and then assign values uniformly under an init method. Under the init method, due to the role of closures, code commonality is achieved! Specific methods:
Dynamicly add external interfaces to enhance code flexibility
addIO:function(str){
var arrs = str.split("."),
o = this;
for (i=(arrs[0] == " Date$") ? 1 : 0; i0)
{
var data=arrs[0]
o[arrs[i]]=o[arrs[i]] ||function(){return this.parIO.apply(null,[data,arguments])};
o=o[arrs[i]];
}
}
InitFuns:function(){
var that=this;
var funs=this.actionFun;
//init interface for all functions(test successfully)
var interfaces=["testLeap","disDayNum","todayBetween","getMonthByDay" ,"getNextWeekByDay","getWeekByDay","newDate","compareDate"]
var shift;
do{
shift=interfaces.shift()
that.addIO(shift);
funs[shift]=function(){};
}while(interfaces.length>0)
//set the common object and variable
//for browsers test
var br={
ie:false,//IE6~8
ff:false,//Firefox
ch:false//Chrome
}
var nav=navigator.userAgent;
if(!-[1,]) br.ie=true;
else if(nav.indexOf("Firefox")>0) br.ff=true;
else if(nav.indexOf( "Chrome")>0) br.ch=true;
//continue to set IO
}
Output the initialized interface on the console:
So all external interfaces are bound to parIO, so that you can save a lot of code when there are many interfaces! ParIO, which is the key to maintaining internal and external hubs, is responsible for finding sub-interfaces, passing parameters, and returning
parIO:function(){
if(Date$.actionFun[arguments[0]])
{
var customFun=Date$.actionFun[arguments[0]]
return customFun.apply(null,[arguments[1]]);
}
else
console&&cosnole.log("empty");
},
You can see that there are three parts:
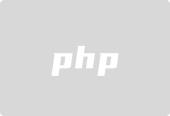
In //set the common object and variable, we can write our public functions and variables, such as judging the browser, adding a similar background sqlHelp function, and then the initialization interface Now:
funs.newDate=function(){
return formatDate(arguments[0][0])
}
funs.getWeekByDay=function(){
return getWeekByDay(arguments[0][0]);
}
funs .getNextWeekByDay=function(){
var speDate=formatDate(arguments[0][0]);
speDate.setDate(speDate.getDate() 7);
return getWeekByDay(speDate);
}
And another advantage of this is that your code will not be copied and used casually by others, because the internal connections of the interface are very strong! For example, funs.getWeekByDay and funs.getNextWeekByDay share the getWeekByDay() method above! Finally, I attach my immature js library and interface declaration. I hope Daniel can help improve it. Thank you very much
/*
//function: to compare two dates and return information "equal"/"more"/"less"
//arguments num:2
//arguments type: Date,Date
//arguments declaration:1.the target object you need to compare(Subtrahend);2.the compare object(Minuend)
//return data -- type: String ; three value: "more"(if target is larger),"equal"(if target is equal to compared object),"less"(if target is smaller)
compareDate:function (objDate,comDate)
{
},
//function:to format the string to Date ,and return
//arguments num:1
//arguments type: for this interface apply for overload, so String or Date is allowed
//arguments declaration:if you pass String ,it should format to "YYYY-MM-DD" or "YYYY/MM/DD" or "YYYY:MM:DD" like "2008/10/12"
//return date : type:Date;one value:the date you want after formatting
newDate :function (str)
{
},
//function:get the start date and end date of the week of the date you have passed and return the Json including {startDay,endDay}
//arguments num:1
//arguments type:for this interface apply for overload , so String or Date is allowed
//arguments declaration:the day you want to get the first day and last day of in this week;if you pass String ,it should format to "YYYY-MM-DD" or "YYYY/MM/DD " or "YYYY:MM:DD" like "2008/10/12"
//return data--type :Json ; one value:{startDay:"",endDay:""} ,you can get it by var.startDay(var is your custom variable)
getWeekByDay :function (day)
{
},
//function:get the start date and end date of next week of the date you have passed and return the Json including {startDay,endDay}
//arguments num:1
//arguments type:for this interface apply for overload , so String or Date is allowed
//arguments declaration:the day you want to get the first day and last day of in this week;if you pass String ,it should format to "YYYY-MM-DD" or "YYYY/MM/DD" or "YYYY:MM:DD" like " 2008/10/12"
//return data--type:Json; one value:{startDay:"",endDay:""} , you can get it by var.startDay(var is your custom variable)
getNextWeekByDay :function (day)
{
};
//function:get the start date and end date of the month of the date you have passed and return the Json including {startDay,endDay}
//arguments num:1
//arguments type:Date
//arguments declaration: the day you want to get the first day and last day of in this month
//return data- -type :Json ; one value:{startDay:"",endDay:""} ,you can get it by var.startDay(var is your custom variable)
getMonthByDay :function (day)
{
},
//function:to test if including today between the two dates you pass and return in boolean
//arguments num:2
//arguments type:Date,Date
// arguments declaration: the procedure will test the larger and sort automatically ,so the order is no matter
//return data-- type: boolean ; one value :true if today is between the two dates
todayBetween :function ( startDate,endDate)
{
},
//function:to caculate the difference between two dates in days
//arguments num:2
//arguments type:Date,Date
//arguments declaration:1.startDate(the one be reduced) 2.endDate(the one to reduce)
//return data--type:Number; one value:the different between these two dates
disDayNum:function (startDate,endDate)
{
},
//function:test the year of the date you have passed leap or not and return in boolean
//arguments num:1
//arguments type: for this interface apply for overload, so String or Date is allowed
//arguments declaration: if you pass String ,it should format to "YYYY-MM-DD" or "YYYY/MM/ DD" or "YYYY:MM:DD" like "2008/10/12"
//return data -- type:boolean ;one value: true if it is leap year or false
testLeap :function (date )
{
} */
Welcome to download:
Date$.js