


JavaScript lacks block-level scope and has no private modifier, but it does have function scope. The benefit of scope is that inner functions can access the parameters and variables of their outer functions (except this and argument. This in the inner function points to the global object, and argument points to the function parameters of the inner function). We can use this property to simulate private properties in object-oriented.
var myObject=function(value){
var value=value || 0;
return{
increment:function(num){
value =typeof num==='number' ? num : 0;
},
setValue:function(num){
value = typeof num==='number' ? num : value;
},
getValue:function(){
return value;
}
}
}(10)
//alert(myObject.getValue()); //10
myObject.setValue(20);
//alert(myObject.getValue() ); //20
myObject.increment(5);
alert(myObject.getValue()); //25
As in the above example, myObject is returned after execution of the anonymous function object. The variable value in the anonymous function is inaccessible to the outside of the anonymous function, but it is accessible to the functions inside it. The execution of the anonymous function ends. Since the variable value is still accessed by the returned myObject object, value is occupied by it. The memory is not destroyed. At this time, the internal variable value is just like the private variable of the myObject object.
var myObject=function(value){
var name='MyObject';
return{
increment:function(num){
value =typeof num==='number' ? num : 0;
},
setValue:function (num){
value = typeof num==='number' ? num : value;
},
getValue:function(){
//alert(this);
return value;
},
getName:function(){
return name;
},
setName:function(nameStr){
name=nameStr;
},
toString:function(){
return '[Object:' name ']';
}
}
}
var obj=myObject(5);
obj. increment(6);
//alert(obj.getValue()); // 11
//alert(obj); //[Object:MyObject]
obj.setName('temp object 01 ');
alert(obj) //[Object:temp object 01]
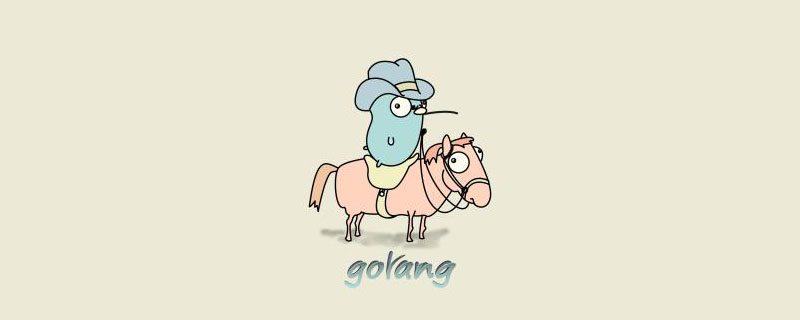
闭包(closure)是一个函数以及其捆绑的周边环境状态(lexical environment,词法环境)的引用的组合。 换而言之,闭包让开发者可以从内部函数访问外部函数的作用域。 闭包会随着函数的创建而被同时创建。
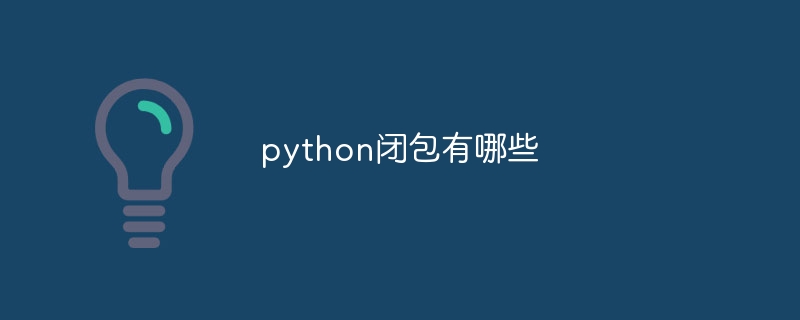
python闭包主要包括函数闭包和装饰器闭包。详细介绍:1、函数闭包是指在一个函数内部返回另一个函数,并且返回的函数能够访问到其内部变量。这样的返回函数就是函数闭包,函数闭包在程序中可以被反复使用,因此可以用来实现一些功能上的封装;2、装饰器闭包是指在使用装饰器时,被装饰的函数并没有直接被调用,而是被包装在一个函数内部,并返回一个新的函数。这个新的函数就是一个装饰器闭包等等。
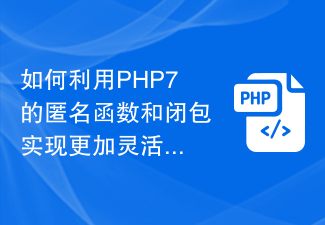
如何利用PHP7的匿名函数和闭包实现更加灵活的代码逻辑处理?在PHP7之前,我们经常使用函数来封装一段特定的逻辑,然后在代码中调用这些函数来实现特定的功能。然而,有时候我们可能需要在代码中定义一些临时的逻辑块,这些逻辑块没有必要创建一个独立的函数,同时又不想在代码中引入太多的全局变量。PHP7引入了匿名函数和闭包,可以很好地解决这个问题。匿名函数是一种没有名
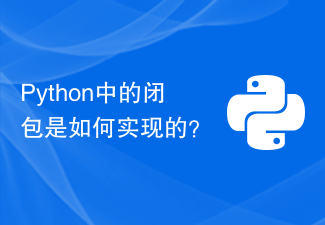
Python中的闭包是如何实现的?闭包是一种函数内部定义的函数,并且在函数内部引用了外部函数的变量。这种特性使得内部函数可以访问外部函数的变量,并且在外部函数执行完毕后,闭包仍然可以访问和操作外部函数的变量。闭包在Python中通过以下几个步骤来实现:定义外部函数,并在其中定义内部函数:首先,我们需要在外部函数内部定义一个内部函数。这个内部函数即是闭包。de
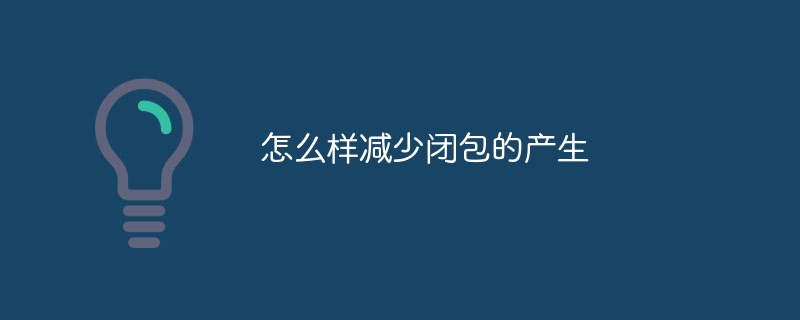
减少闭包产生的方法有避免不必要的闭包、控制闭包的返回值、使用弱引用、减少不必要的全局变量、合理使用循环和递归、使用事件代理、编写单元测试、遵循设计原则和使用工具进行代码分析等。详细介绍:1、避免不必要的闭包,在很多情况下,闭包并非必需的,可以用模块模式来实现私有变量,避免使用闭包;2、控制闭包的返回值,在使用闭包时,应该尽量控制闭包的返回值,如果闭包返回的是基本数据类型等等。
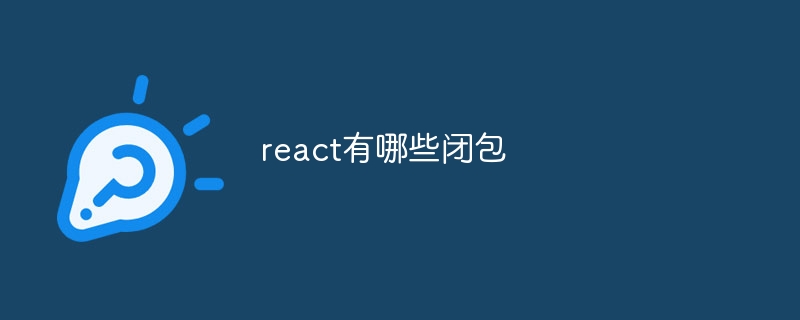
react有事件处理函数、useEffect和useCallback、高阶组件等等闭包。详细介绍:1、事件处理函数闭包:在React中,当我们在组件中定义一个事件处理函数时,该函数会形成一个闭包,可以访问组件作用域内的状态和属性。这样可以在事件处理函数中使用组件的状态和属性,实现交互逻辑;2、useEffect和useCallback中的闭包等等。
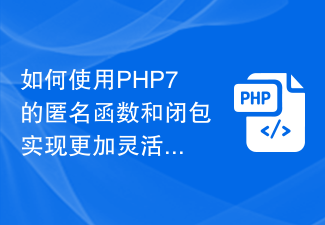
如何使用PHP7的匿名函数和闭包实现更加灵活和可复用的代码逻辑?在PHP编程领域中,匿名函数和闭包是非常有价值和强大的工具。PHP7引入了一些新的语言特性,使得使用匿名函数和闭包更加方便和灵活。本文将介绍如何使用PHP7的匿名函数和闭包来实现更加灵活和可复用的代码逻辑,并提供一些具体的代码示例。一、匿名函数匿名函数是一种没有名称的函数。在PHP中,可以将匿名
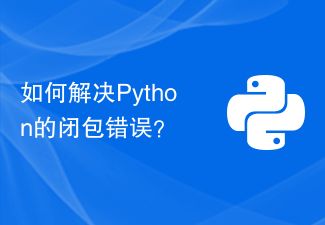
Python是一种非常流行的编程语言,因为它非常易学易用,同时也具备了强大的功能。其中,闭包是Python中的一种函数,它可以在函数的内部定义另一个函数,并返回这个函数作为函数的返回值。尽管闭包非常方便,但有时会出现某些错误,比如闭包错误。本文将介绍如何解决Python的闭包错误。初步了解闭包在Python中,闭包是由一个内部函数和一个定义在内部函数之外的函


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

Notepad++7.3.1
Easy-to-use and free code editor

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

Atom editor mac version download
The most popular open source editor

SublimeText3 Linux new version
SublimeText3 Linux latest version
