Scope
Global scope
Local scope
Scope chain
Execution context
Active object
Closure
Closure optimization
Appears in JavaScript I learned a concept that I have never learned before - closure. What is closure? From the surface understanding, it is a closed package, which is related to scope. Therefore, before talking about closures, let’s talk about scope.
Scope
Generally speaking, variables and functions used in a piece of program code are not always available. The scope that limits their availability is the scope. The use of scopes improves It improves the locality of program logic, enhances the reliability of the program, and reduces name conflicts.
Global Scope
Objects that can be accessed anywhere in the code have global scope. The following situations have global scope:
1. The outermost function and variables defined outside the outermost function have global scope, for example:
var outSide="var outside";
function outFunction(){
var name="var inside";
function inSideFunction(){
alert (name);
}
inSideFunction();
}
alert(outSide); //Correct
alert(name); //Wrong
outFunction(); // Correct
inSideFunction() //Error
2. Variables that are not defined for direct assignment are automatically declared to have global scope, for example:
blogName="CSDN Li Da"
3. The properties of all window objects have global Scope, for example: the built-in properties of the window object have global scope, such as window.name, window.location, window.top, etc.
Local Scope
function outFunction(){
var name="inside name";
function inFunction(){
alert(name);
}
inFunction();
}
alert(name); //Error
inFunction(); //Error
scope chain (scope chain)
In JavaScript, everything in JavaScript is an object. Includes functions. Function objects, like other objects, have properties that can be accessed through code and a set of internal properties that are only accessible to the JavaScript engine. One of the internal properties is the scope, which contains the collection of objects in the scope in which the function was created, called the function's scope chain, which determines what data can be accessed by the function.
When a function is created, its scope chain will be filled with data objects accessible in the scope in which the function was created. For example, function:
function add(num1,num2) {
var sum = num1 num2;
return sum;
}
When the function add is created, its scope chain will be filled with a global object, which contains All global variables are included, as shown in the figure below (note: the picture only illustrates a part of all variables):
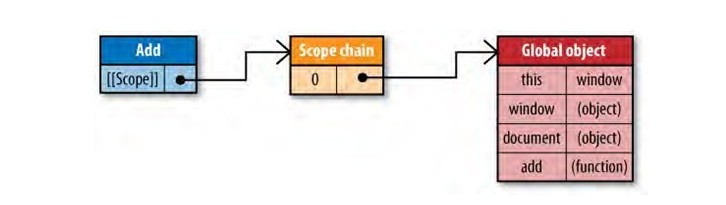
It can be seen that the scope chain of a function is created when the function is created.
Execute context
The scope of function add will be used during execution, for example:
var total = add(5,10);
When the add function is executed, JavaScript will create an Execute context (execution context), which contains all the information needed by the add function during runtime. Execute context also has its own Scope chain. When the function runs, the JavaScript engine will first initialize the execution context's scope chain from the scope chain using the add function.
Active Object
Then the JavaScript engine will create an Active Object, and these values are copied to the scope chain of the runtime context in the order they appear in the function. Together they form a new object - the "activation object". This object contains all local variables, parameters, and this and other variables during the function runtime. This object will be pushed into the front end of the scope chain. When the runtime context is destroyed, the active object is also destroyed. The new scope chain is as shown below:
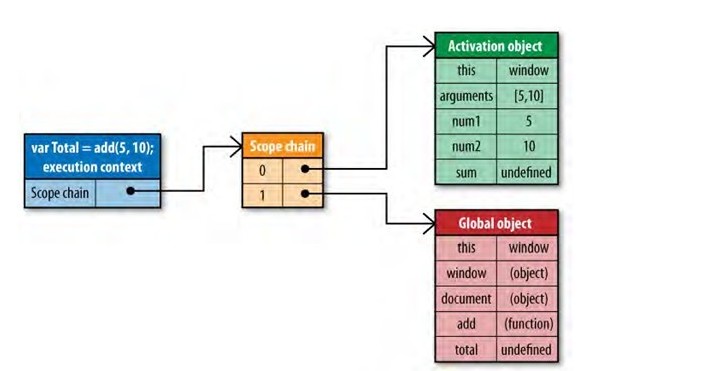
Execution context is a dynamic concept. It is created when the function is running. Active Object is also a dynamic concept. It is referenced by the scope chain of the execution context. It can be concluded that both execution context and active objects are dynamic concepts, and the scope chain of the execution context is initialized by the function scope chain.
During the execution of the function, every time a variable is encountered, it will retrieve where the data is obtained and stored. This process starts from the head of the scope chain, that is, from the active object, and looks for the identifier with the same name. If found Just use the variable corresponding to this identifier. If not, continue to search for the next object in the scope chain. If all objects are not found after searching, the identifier is considered undefined. During the execution of the function, each identifier Go through this search process.
Closure (closure)
Let’s look at an example first, javascript code:
Run result: Prompt corresponding prompt information
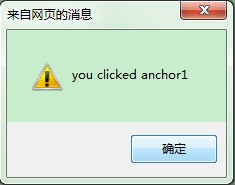
Result analysis: The optimized result is because we separate the declared variables from the click event. Use the scope chain explained above: When the page is loaded, the listener function is executed first, and the listener function requires the anchor variable. If it is not found in the listener function scope chain, it will enter the next level scope chain, that is, find the anchor in newLoad, because In the listener, it has been determined which anchor click corresponds to which prompt information, so we just look for the corresponding anchor in newload, and will not wait for the loop to complete to generate anchor4.
Because I have only been exposed to JavaScript for a short time, please correct me if I have any misunderstandings.