The window.confirm method in javascript is very useful. It can pop up a confirmation dialog box
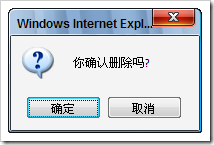
The reason why we pop up this dialog box may be because the operation is very dangerous, so the user is required to confirm. But if "OK" is selected by default, this principle may be violated.
In addition, the buttons of the confirm dialog box are fixed at "OK" and "Cancel". It may not be very intuitive sometimes.
So, you can consider using msgbox in vbscript to rewrite this behavior. The following is an example
<%@ Page Language="C#" AutoEventWireup="true" CodeBehind="Default.aspx.cs" Inherits="WebApplication1._Default" %>
<script> <br>function deleteConfirm(msg) <br>{ <br> function window.confirm(str) <br>{ <br>str= str.replace(/'/g, "'& chr(39) &'").replace(/rn/g, "'& VBCrLf &' "); <br>execScript("n = msgbox('" str "', 289, 'Delete box')", "vbscript"); <br>return(n == 1); <br>} <br>return window.confirm(msg); <br>} <br></script>
For specific details of the msgbox method, you can also refer to the following introduction
The MsgBox function
displays a message in the dialog box, waits for the user to click the button, and returns a value indicating that the user has clicked the button. button to click.
MsgBox(prompt[, buttons][, title][, helpfile, context])
Parameters
prompt
A string expression that is displayed as a message in the dialog box. The maximum length of prompt is approximately 1024 characters, depending on the character width used. If the prompt contains multiple lines, you can use carriage returns (Chr(13)), line feeds (Chr(10)), or a combination of carriage returns and line feeds (Chr(13) & Chr(10)) between the lines. ) separates the lines.
Buttons
Numerical expression, which is the sum of the values that specify the number and type of buttons to be displayed, the icon style used, the identity of the default button, and the message box style. See the Settings section for values. If omitted, the default value for buttons is 0.
Title
A string expression displayed in the title bar of the dialog box. If title is omitted, the application's name is displayed in the title bar.
Helpfile
String expression that identifies the help file that provides context-sensitive help for the dialog box. If helpfile is provided, context must be provided. Not available on 16-bit system platforms.
Context
A numeric expression that identifies the context number assigned to a help topic by the author of the help file. If context is provided, helpfile must be provided. Not available on 16-bit system platforms.
Settings The
buttons parameter can have the following values:
Constant |
Value |
Description |
vbOKOnly |
0 |
Only the OK button will be displayed. |
vbOKCancel |
1 |
Displays the OK and Cancel buttons. |
vbAbortRetryIgnore |
2 |
Shows the Abandon, Retry and Ignore buttons. |
vbYesNoCancel |
3 |
Shows Yes, No and Cancel buttons. |
vbYesNo |
4 |
Displays Yes and No buttons. |
vbRetryCancel |
5 |
Displays the Retry and Cancel buttons. |
vbCritical |
16 |
Display the critical information icon. |
vbQuestion |
32 |
Display the Warning Query icon. |
vbExclamation |
48 |
Display Warning Message icon. |
vbInformation |
64 |
Displays the Information message icon. |
vbDefaultButton1 |
0 |
The first button is the default button. |
vbDefaultButton2 |
256 |
The second button is the default button. |
vbDefaultButton3 |
512 |
The third button is the default button. |
vbDefaultButton4 |
768 |
The fourth button is the default button. |
vbApplicationModal |
0 |
Application Mode: The user must respond to the message box to continue working in the current application. |
vbSystemModal |
4096 |
系统模式:在用户响应消息框前,所有应用程序都被挂起。 |
第一组值 (0 - 5) 用于描述对话框中显示的按钮类型与数目;第二组值 (16, 32, 48, 64) 用于描述图标的样式;第三组值 (0, 256, 512) 用于确定默认按钮;而第四组值 (0, 4096) 则决定消息框的样式。在将这些数字相加以生成 buttons 参数值时,只能从每组值中取用一个数字。
返回值
MsgBox 函数有以下返回值:
常数 |
值 |
按钮 |
vbOK |
1 |
确定 |
vbCancel |
2 |
取消 |
vbAbort |
3 |
放弃 |
vbRetry |
4 |
重试 |
vbIgnore |
5 |
忽略 |
vbYes |
6 |
是 |
vbNo |
7 |
否 |
설명
helpfile과 context가 모두 제공되는 경우 사용자는 F1 키를 눌러 해당 context에 해당하는 도움말 항목을 볼 수 있습니다.
대화 상자에 취소 버튼이 표시되는 경우 ESC 키를 누르면 취소를 클릭하는 것과 같은 효과가 있습니다. 대화 상자에 도움말 버튼이 포함된 경우 대화 상자에 상황에 맞는 도움말이 제공됩니다. 그러나 다른 버튼을 클릭할 때까지 아무 값도 반환되지 않습니다.
Microsoft Internet Explorer가 MsgBox 기능을 사용할 때 모든 대화 상자의 제목에는 표준 대화 상자와 구별하기 위해 항상 "VBScript"가 포함됩니다.
다음 예는 MsgBox 함수의 사용법을 보여줍니다.
Dim MyVar
MyVar = MsgBox ("Hello World!", 65, "MsgBox 예제")
' MyVar에는 클릭한 버튼에 따라 1 또는 2가 포함됩니다.