1. Important knowledge points about prototype objects
First of all, you must know a very important knowledge point, in one sentence: all objects have prototype objects.
2. Comparing the understanding of other languages
Prototype objects are static properties and static methods in classes in other languages. They are always static. The principle is: there is only one object in the memory.
3. Image map in memory:
First of all, before generating the js object, we need to create a constructor (I don’t know this, then Don’t read any further), as follows:
function Person (name_, age_) {
this.name = name_;
this.age = age_;
}
Next, we need the new object, here , we have three new (Person) objects, "Zhang San" "Li Lei" "Han Meimei", they come from the same constructor Person:
That’s it in the memory. Each object has its own name and age memory. How many objects are new here, how many blocks of name and age memory will be allocated .
Seeing this, it should be relatively easy to understand. Next we add an attribute.location attribute, as follows:
function Person(name_, age_) {
this.name = name_;
this.age = age_;
this.location = "Earth";
}
At this time we have three new people. The memory situation is as follows:
Here we see that the three objects all have a memory space of "Earth". You have to use your brain here. Three people have the memory of the Earth. Can we do this?
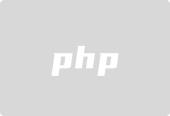
Do you think this is a good idea? This only requires one earth and everyone can use it. Seeing this, okay, if the common space is an object, It's the so-called prototype object. Hungry? That's it?
Yes, that's it.
The most important function of the prototype object is to separate the constants and methods into itself. Provide others" Use your own object. The final picture is as follows:
4. Introduce prototype objects from the code level. The above picture is an object in memory. We now start from the code level Operation from above.
function Person(name_, age_) {
this.name = name_;
this.age = age_;
this.location = "Earth";
}
// Three specific objects
var zhangsan = new Person("zhangsan", 21);
var lilei = new Person("lilei", 21);
var hanmeimei = new Person("hanmeimei", 21);
// Their prototypes The object is
Person.prototype.location = "Earth";
Person.prototype.killPerson = function() {
return "Kill";
};
There is a problem here. We know the prototype object, but how do we access the properties in the prototype object. That is, how do we get the location and use the killPerson method?
Please see:
alert(zhangsan.location);
alert(zhangsan.killPerson());
In this way, you can access it, but the premise is that location and killPerson are not defined in your object attributes. Otherwise, the original object will be overwritten. This involves a prototype problem, that is,
In zhangsan.location First, we check the zhangsan object itself. From the picture, we know that Zhang San has name, age and prototype pointer attributes. There is no location. After it is not found, it will continue to search the original object to see if it can find the location attribute. , if so, the properties of the original object will be called.
So
alert(zhangsan.location) will output "Earth"
alert(zhangsan.killPerson() ) will output "kill"
The above is my personal understanding of prototype objects. Hope it helps everyone.