Java Platform Independence: Benefits, Limitations, and Implementation
Java achieves platform independence through the Java Virtual Machine (JVM) and bytecode. 1) The JVM interprets bytecode, allowing the same code to run on any platform with a JVM. 2) Bytecode is compiled from Java source code and is platform-independent. However, limitations include potential performance issues and the necessity of a JVM on the target machine.
Java's platform independence is often touted as one of its most compelling features. When I first delved into Java, the promise of "write once, run anywhere" was both intriguing and a bit daunting. Let's dive into the benefits, limitations, and the nitty-gritty of how Java achieves this independence.
Java's platform independence primarily stems from its use of the Java Virtual Machine (JVM). The JVM acts as an intermediary between the compiled Java bytecode and the underlying operating system. This abstraction layer allows Java programs to run on any platform that has a JVM installed, without needing to recompile the code.
Here's a simple example to illustrate how this works:
public class HelloWorld { public static void main(String[] args) { System.out.println("Hello, World!"); } }
This code, when compiled, turns into bytecode that the JVM can interpret and execute on any platform. The beauty of this approach is that you can write this code on a Windows machine, compile it, and then run it on a Linux server without any modifications.
However, while the concept is straightforward, the reality is a bit more complex. The JVM itself needs to be implemented for each platform, and subtle differences in these implementations can sometimes lead to unexpected behavior. This is where the limitations come into play.
One of the main limitations is performance. The JVM's role as an interpreter adds a layer of abstraction that can slow down execution compared to native code. While modern JVMs have made significant strides in performance optimization, there's still a gap. Additionally, certain platform-specific features or libraries might not be available across all JVMs, which can limit the "run anywhere" promise.
Another limitation is the need for a JVM on the target machine. If you're deploying to an environment where installing a JVM is not feasible, Java's platform independence becomes a moot point. This is particularly relevant in embedded systems or certain cloud environments.
Now, let's talk about how Java achieves this independence. The key is the bytecode. When you compile a Java program, it's not compiled into machine code but into an intermediate format called bytecode. This bytecode is platform-independent and can be executed by any JVM. Here's a peek at what the bytecode for our HelloWorld
class might look like:
// Bytecode for HelloWorld class public class HelloWorld { public HelloWorld(); Code: 0: aload_0 1: invokespecial #1 // Method java/lang/Object."<init>":()V 4: return public static void main(java.lang.String[]); Code: 0: getstatic #2 // Field java/lang/System.out:Ljava/io/PrintStream; 3: ldc #3 // String Hello, World! 5: invokevirtual #4 // Method java/io/PrintStream.println:(Ljava/lang/String;)V 8: return }
This bytecode is what the JVM interprets and executes. The JVM then translates this bytecode into machine-specific instructions, ensuring that the same bytecode can run on different platforms.
In my experience, one of the most powerful aspects of Java's platform independence is its impact on development and deployment workflows. I've worked on projects where the development team used different operating systems, yet we could seamlessly integrate and test our code. The ability to deploy the same codebase to different environments without worrying about recompilation is a huge time-saver.
However, it's crucial to be aware of the potential pitfalls. I once encountered a situation where a Java application worked perfectly on our development machines but failed in production due to a subtle difference in the JVM versions. This taught me the importance of thorough testing across different JVMs and platforms.
To mitigate these risks, here are some strategies I've found useful:
- Cross-Platform Testing: Regularly test your application on different operating systems and JVM versions to catch any platform-specific issues early.
- Use of Standard Libraries: Stick to Java's standard libraries as much as possible to ensure maximum compatibility across different JVMs.
- Performance Profiling: Use tools like JProfiler or VisualVM to identify and address any performance bottlenecks introduced by the JVM.
In conclusion, Java's platform independence is a powerful feature that offers significant benefits in terms of development flexibility and deployment ease. However, it's not without its limitations, particularly in terms of performance and the need for a JVM. Understanding how Java achieves this independence through bytecode and the JVM is crucial for leveraging its full potential while being mindful of its constraints. By adopting best practices and being aware of potential pitfalls, you can make the most out of Java's "write once, run anywhere" promise.
The above is the detailed content of Java Platform Independence: Benefits, Limitations, and Implementation. For more information, please follow other related articles on the PHP Chinese website!
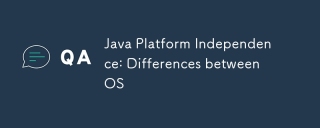
There are subtle differences in Java's performance on different operating systems. 1) The JVM implementations are different, such as HotSpot and OpenJDK, which affect performance and garbage collection. 2) The file system structure and path separator are different, so it needs to be processed using the Java standard library. 3) Differential implementation of network protocols affects network performance. 4) The appearance and behavior of GUI components vary on different systems. By using standard libraries and virtual machine testing, the impact of these differences can be reduced and Java programs can be ensured to run smoothly.
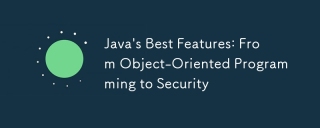
Javaoffersrobustobject-orientedprogramming(OOP)andtop-notchsecurityfeatures.1)OOPinJavaincludesclasses,objects,inheritance,polymorphism,andencapsulation,enablingflexibleandmaintainablesystems.2)SecurityfeaturesincludetheJavaVirtualMachine(JVM)forsand
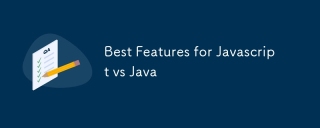
JavaScriptandJavahavedistinctstrengths:JavaScriptexcelsindynamictypingandasynchronousprogramming,whileJavaisrobustwithstrongOOPandtyping.1)JavaScript'sdynamicnatureallowsforrapiddevelopmentandprototyping,withasync/awaitfornon-blockingI/O.2)Java'sOOPf
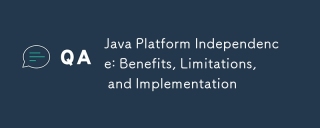
JavaachievesplatformindependencethroughtheJavaVirtualMachine(JVM)andbytecode.1)TheJVMinterpretsbytecode,allowingthesamecodetorunonanyplatformwithaJVM.2)BytecodeiscompiledfromJavasourcecodeandisplatform-independent.However,limitationsincludepotentialp
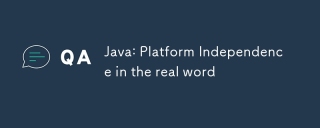
Java'splatformindependencemeansapplicationscanrunonanyplatformwithaJVM,enabling"WriteOnce,RunAnywhere."However,challengesincludeJVMinconsistencies,libraryportability,andperformancevariations.Toaddressthese:1)Usecross-platformtestingtools,2)
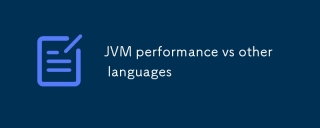
JVM'sperformanceiscompetitivewithotherruntimes,offeringabalanceofspeed,safety,andproductivity.1)JVMusesJITcompilationfordynamicoptimizations.2)C offersnativeperformancebutlacksJVM'ssafetyfeatures.3)Pythonisslowerbuteasiertouse.4)JavaScript'sJITisles

JavaachievesplatformindependencethroughtheJavaVirtualMachine(JVM),allowingcodetorunonanyplatformwithaJVM.1)Codeiscompiledintobytecode,notmachine-specificcode.2)BytecodeisinterpretedbytheJVM,enablingcross-platformexecution.3)Developersshouldtestacross
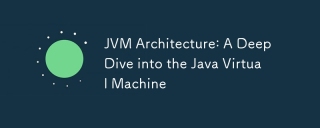
TheJVMisanabstractcomputingmachinecrucialforrunningJavaprogramsduetoitsplatform-independentarchitecture.Itincludes:1)ClassLoaderforloadingclasses,2)RuntimeDataAreafordatastorage,3)ExecutionEnginewithInterpreter,JITCompiler,andGarbageCollectorforbytec


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SublimeText3 English version
Recommended: Win version, supports code prompts!

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
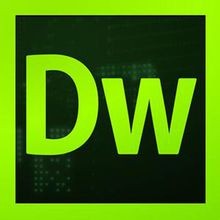
Dreamweaver CS6
Visual web development tools
