Laravel implements MVC by separating the application into Model (data and logic), View (presentation), and Controller (user input handling). In Laravel, this is supported by tools and conventions that enhance development efficiency. For example, a BookController fetches books via the Book model and passes them to the index view for display.
In the world of web development, choosing the right architecture can be the difference between a smooth sailing project and a chaotic mess. Today, let's dive deep into the MVC (Model-View-Controller) architecture, particularly how it's implemented in Laravel, a popular PHP framework. If you've ever wondered how to structure your web app for scalability and maintainability, this discussion is for you.
When I first encountered MVC in Laravel, it was like a revelation. It's not just about organizing code; it's about creating a workflow that enhances productivity and clarity. Laravel's implementation of MVC is particularly elegant, making it easier to manage complex applications. But why choose MVC, and what makes Laravel's approach stand out?
MVC splits your application into three interconnected components: the Model, which manages data and business logic; the View, responsible for presenting data to the user; and the Controller, which handles user input and performs operations on the Model and View. In Laravel, these components are not just theoretical constructs but are supported by a robust set of tools and conventions that streamline development.
Let's jump right into the heart of MVC in Laravel. Imagine you're building an online bookstore. In this scenario, the Model might represent books, customers, and orders. The View could be the webpage where users see books, add them to a cart, and checkout. The Controller would manage the flow between these, like processing a user's request to add a book to their cart.
Here's a peek at how you might structure a simple book listing feature:
// app/Http/Controllers/BookController.php namespace App\Http\Controllers; use App\Models\Book; use Illuminate\Http\Request; class BookController extends Controller { public function index() { $books = Book::all(); return view('books.index', ['books' => $books]); } }
// app/Models/Book.php namespace App\Models; use Illuminate\Database\Eloquent\Model; class Book extends Model { protected $fillable = ['title', 'author', 'price']; }
// resources/views/books/index.blade.php <!DOCTYPE html> <html> <head> <title>Book List</title> </head> <body> <h1 id="Books">Books</h1> <ul> @foreach ($books as $book) <li>{{ $book->title }} by {{ $book->author }} - ${{ $book->price }}</li> @endforeach </ul> </body> </html>
This setup is straightforward, but it encapsulates the essence of MVC. The BookController
fetches all books from the database through the Book
model and passes them to the index
view. The view then displays the books in a simple list format.
One of the strengths of Laravel's MVC implementation is its routing system, which seamlessly integrates with controllers. Here's how you might define a route for our book listing:
// routes/web.php use App\Http\Controllers\BookController; Route::get('/books', [BookController::class, 'index']);
This approach not only keeps your application organized but also makes it easier to test and maintain. When I've worked on larger projects, having a clear separation of concerns has been invaluable, especially when multiple developers are involved.
However, MVC isn't without its challenges. One common pitfall is overcomplicating the controller layer. It's tempting to add business logic there, but remember, the controller should primarily handle the flow of data between models and views. If you find your controllers getting bloated, it's a sign to refactor and move logic to the models or perhaps even introduce service classes.
Another aspect to consider is the performance impact of MVC. While Laravel's Eloquent ORM makes working with databases a breeze, it can lead to the N 1 query problem if not managed properly. To mitigate this, eager loading relationships in your models can significantly improve performance. Here's an example:
// Eager loading to avoid N 1 query problem $books = Book::with('author')->get();
This approach loads the related author
data in a single query, preventing multiple database hits.
In terms of best practices, always keep your views as lean as possible. Laravel's Blade templating engine is powerful, but stuffing too much logic into your views can make them hard to maintain. Instead, use controller methods or even separate view composers to prepare data for your views.
Finally, let's touch on testing. Laravel's built-in testing features make it easier to ensure your MVC components are working as expected. Here's a simple test for our BookController
:
// tests/Feature/BookControllerTest.php namespace Tests\Feature; use Illuminate\Foundation\Testing\RefreshDatabase; use Tests\TestCase; class BookControllerTest extends TestCase { use RefreshDatabase; /** @test */ public function it_can_display_a_list_of_books() { $book = Book::factory()->create(); $response = $this->get('/books'); $response->assertStatus(200); $response->assertSee($book->title); } }
This test ensures that our book listing page displays correctly and that the book data is visible.
In wrapping up, Laravel's MVC architecture is a powerful tool for building web applications. It encourages a clean separation of concerns, which leads to more maintainable and scalable code. However, it requires discipline to use effectively—avoid overcomplicating controllers, manage database queries wisely, and keep your views focused on presentation. By following these guidelines and leveraging Laravel's robust features, you can build robust web applications that stand the test of time.
The above is the detailed content of MVC Architecture: Building Web App with Laravel. For more information, please follow other related articles on the PHP Chinese website!
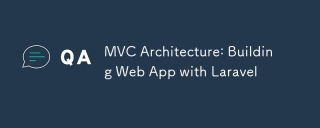
LaravelimplementsMVCbyseparatingtheapplicationintoModel(dataandlogic),View(presentation),andController(userinputhandling).InLaravel,thisissupportedbytoolsandconventionsthatenhancedevelopmentefficiency.Forexample,aBookControllerfetchesbooksviatheBookm
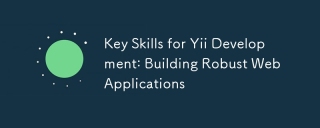
TobuildrobustwebapplicationswithYii,mastertheseskills:1)MVCarchitectureforstructuringapplications,2)ActiveRecordforefficientdatabaseoperations,3)WidgetsystemforreusableUIcomponents,4)Validationandsecuritymeasures,5)Cachingforperformanceoptimization,a
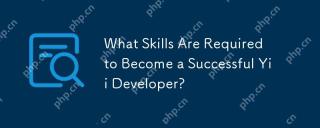
TobecomeasuccessfulYiideveloper,youneed:1)PHPmastery,2)understandingofMVCarchitecture,3)Yiiframeworkproficiency,4)databasemanagementskills,5)front-endknowledge,6)APIdevelopmentexpertise,7)testinganddebuggingcapabilities,8)versioncontrolproficiency,9)
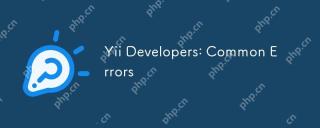
ThemostcommonerrorsinYiiframeworkare"UnknownProperty","InvalidConfiguration","ClassNotFound",and"ValidationErrors".1."UnknownProperty"errorsoccurwhenaccessingnon-existentproperties;ensurepropertiesexi
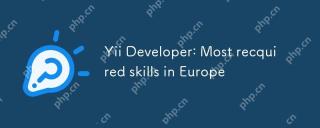
The key skills that European Yii developers need to possess include: 1. Yii framework proficiency, 2. PHP proficiency, 3. Database management, 4. Front-end skills, 5. RESTful API development, 6. Version control system, 7. Testing and debugging, 8. Security knowledge, 9. Agile methodology, 10. Soft skills, 11. Localization and internationalization, 12. Continuous learning, these skills make developers stand out in the European market.
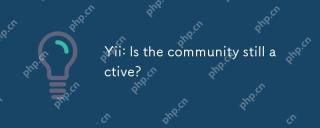
Yes,theYiicommunityisstillactiveandvibrant.1)TheofficialYiiforumremainsaresourcefordiscussionsandsupport.2)TheGitHubrepositoryshowsregularcommitsandpullrequests,indicatingongoingdevelopment.3)StackOverflowcontinuestohostYii-relatedquestionsandhigh-qu
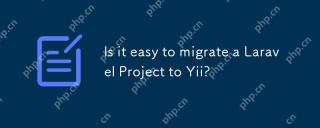
Migratingalaravel Projecttoyiiishallingbutachieffable WITHIEFLEFLANT.1) Mapoutlaravel component likeroutes, Controllers, Andmodels.2) Translatelaravel's SartisancommandeloequentTooyii's giiandetiverecordeba
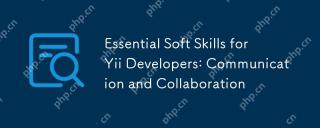
Soft skills are crucial to Yii developers because they facilitate team communication and collaboration. 1) Effective communication ensures that the project is progressing smoothly, such as through clear API documentation and regular meetings. 2) Collaborate to enhance team interaction through Yii's tools such as Gii to improve development efficiency.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Zend Studio 13.0.1
Powerful PHP integrated development environment
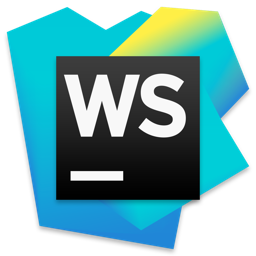
WebStorm Mac version
Useful JavaScript development tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

SublimeText3 Chinese version
Chinese version, very easy to use

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
