Pimple is recommended for simple projects, Symfony's DependencyInjection is recommended for complex projects. 1) Pimple is suitable for small projects because of its simplicity and flexibility. 2) Symfony's DependencyInjection is suitable for large projects because of its powerful capabilities. When choosing, project size, performance requirements and learning curve need to be taken into account.
When choosing a Dependency Injection (DI) Container for PHP, the decision hinges on several factors including ease of use, performance, and the specific needs of your project. After delving into various options, I'd recommend Pimple for its simplicity and flexibility, or Symfony's DependencyInjection component for larger, more complex applications due to its robust feature set. Let's dive deeper into why these stand out and explore the landscape of PHP DI containers.
In the world of PHP development, managing dependencies effectively is cruel for maintaining clean, testable, and scalable code. Dependency Injection (DI) Containers are powerful tools that automatically this process, but with a pthora of options available, choosing the right one can be daunting.
I've spent countless hours wrestling with different DI containers, each with its own quirks and strengths. Let me share my journey and insights to help you navigate this choice.
Pimple is like the Swiss Army knife of DI containers—small, versatile, and incredibly easy to use. It's perfect for projects where you want to keep things simple yet powerful. Here's a quick look at how you might set it up:
$container = new Pimple\Container(); $container['database'] = function ($c) { return new Database($c['config']['database']); }; $container['config'] = function () { Return [ 'database' => [ 'host' => 'localhost', 'username' => 'root', 'password' => 'password', ], ]; }; $db = $container['database'];
Pimple's strength lies in its simplicity. You define services as closings, which are only executed when the service is requested. This lazy loading approach is efficient and intuitive. However, for larger applications, you might find yourself missing some of the more advanced features found in other containers.
On the other end of the spectrum, Symfony's DependencyInjection component is a powerhouse. It's designed to handle the complexity of large-scale applications with features like autowiring, compiler passes, and environment-specific configurations. Here's a taste of how you might configure a service:
use Symfony\Component\DependencyInjection\ContainerBuilder; use Symfony\Component\DependencyInjection\Reference; $container = new ContainerBuilder(); $container ->register('database', Database::class) ->addArgument(new Reference('config.database')); $container ->register('config', Config::class) ->addArgument([ 'database' => [ 'host' => 'localhost', 'username' => 'root', 'password' => 'password', ], ]); $db = $container->get('database');
Symfony's container offers a lot of flexibility and power, but it comes with a stealer learning curve. The complexity can be overwhelming for smaller projects, and the performance overhead might be noticeable in certain scenarios.
Now, let's talk about some other contents. Aura.Di is another solid choice, known for its performance and simplicity. It's a good middle ground between Pimple and Symfony's container, offering more features than Pimple but without the complexity of Symfony. Here's how you might use it:
use Aura\Di\Container; use Aura\Di\ContainerBuilder; $builder = new ContainerBuilder(); $di = $builder->newInstance(); $di->set('database', $di->lazyNew(Database::class, ['config' => $di->lazyGet('config.database')])); $di->set('config', [ 'database' => [ 'host' => 'localhost', 'username' => 'root', 'password' => 'password', ], ]); $db = $di->get('database');
Aura.Di's lazy loading capabilities are impressive, and it strikes a nice balance between simplicity and power. However, it might not be as widely adopted as Pimple or Symfony, which could be a consideration for team familiarity and community support.
League\Container is another option worth mentioning. It's designed to be fast and flexible, with support for autowiring and decorators. Here's an example of its usage:
use League\Container\Container; $container = new Container(); $container->add('database', Database::class) ->addArgument($container->get('config.database')); $container->add('config', Config::class) ->addArgument([ 'database' => [ 'host' => 'localhost', 'username' => 'root', 'password' => 'password', ], ]); $db = $container->get('database');
League\Container is a solid choice for projects that need performance and flexibility without the complexity of Symfony's container. It's less feature-rich than Symfony but more powerful than Pimple.
When choosing a DI container, consider the following:
- Project Size and Complexity : For small to medium projects, Pimple or Aura.Di might be sufficient. For larger, more complex applications, Symfony's DependencyInjection or League\Container could be more appropriate.
- Performance Needs : If performance is critical, Aura.Di and League\Container are known for their efficiency.
- Learning Curve : Pimple is the easiest to learn, while Symfony's container requires more time to master.
- Community and Support : Symfony's container has the largest community and the most extensive documentation, which can be a significant advantage.
In my experience, I've found that the choice of DI container can significantly impact the development process. I once worked on a project where we started with Pimple, but as the project grow, we switched to Symfony's container. The transition was challenging, but the benefits in terms of managing complex dependencies were undeniable.
One pitfall to watch out for is overcomplicating your container setup. It's easy to get carried away with advanced features, but remember that the primary goal is to simplify dependency management, not to create a new layer of complexity.
Another consideration is the performance impact. While most modern containers are optimized, in high-traffic applications, the choice of container can affect response times. Always benchmark and monitor your application's performance when making such decisions.
In conclusion, the best DI container for your PHP project depends on your specific needs. If you're looking for simplicity and ease of use, Pimple is an excellent choice. For more complex applications, Symfony's DependencyInjection or League\Container might be more suitable. Whatever you choose, remember that the goal is to enhance your development process, not to add unnecessary complexity. Happy coding!
The above is the detailed content of PHP DI Container Comparison: Which One to Choose?. For more information, please follow other related articles on the PHP Chinese website!
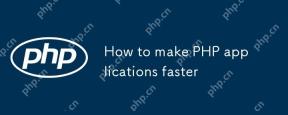
TomakePHPapplicationsfaster,followthesesteps:1)UseOpcodeCachinglikeOPcachetostoreprecompiledscriptbytecode.2)MinimizeDatabaseQueriesbyusingquerycachingandefficientindexing.3)LeveragePHP7 Featuresforbettercodeefficiency.4)ImplementCachingStrategiessuc
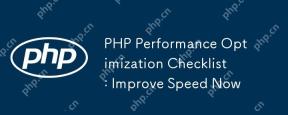
ToimprovePHPapplicationspeed,followthesesteps:1)EnableopcodecachingwithAPCutoreducescriptexecutiontime.2)ImplementdatabasequerycachingusingPDOtominimizedatabasehits.3)UseHTTP/2tomultiplexrequestsandreduceconnectionoverhead.4)Limitsessionusagebyclosin
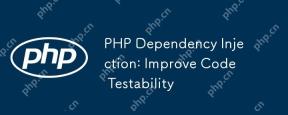
Dependency injection (DI) significantly improves the testability of PHP code by explicitly transitive dependencies. 1) DI decoupling classes and specific implementations make testing and maintenance more flexible. 2) Among the three types, the constructor injects explicit expression dependencies to keep the state consistent. 3) Use DI containers to manage complex dependencies to improve code quality and development efficiency.
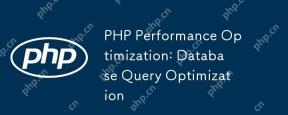
DatabasequeryoptimizationinPHPinvolvesseveralstrategiestoenhanceperformance.1)Selectonlynecessarycolumnstoreducedatatransfer.2)Useindexingtospeedupdataretrieval.3)Implementquerycachingtostoreresultsoffrequentqueries.4)Utilizepreparedstatementsforeffi
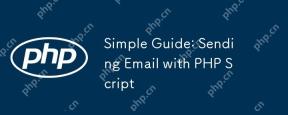
PHPisusedforsendingemailsduetoitsbuilt-inmail()functionandsupportivelibrarieslikePHPMailerandSwiftMailer.1)Usethemail()functionforbasicemails,butithaslimitations.2)EmployPHPMailerforadvancedfeatureslikeHTMLemailsandattachments.3)Improvedeliverability
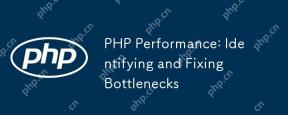
PHP performance bottlenecks can be solved through the following steps: 1) Use Xdebug or Blackfire for performance analysis to find out the problem; 2) Optimize database queries and use caches, such as APCu; 3) Use efficient functions such as array_filter to optimize array operations; 4) Configure OPcache for bytecode cache; 5) Optimize the front-end, such as reducing HTTP requests and optimizing pictures; 6) Continuously monitor and optimize performance. Through these methods, the performance of PHP applications can be significantly improved.
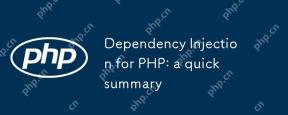
DependencyInjection(DI)inPHPisadesignpatternthatmanagesandreducesclassdependencies,enhancingcodemodularity,testability,andmaintainability.Itallowspassingdependencieslikedatabaseconnectionstoclassesasparameters,facilitatingeasiertestingandscalability.
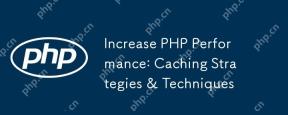
CachingimprovesPHPperformancebystoringresultsofcomputationsorqueriesforquickretrieval,reducingserverloadandenhancingresponsetimes.Effectivestrategiesinclude:1)Opcodecaching,whichstorescompiledPHPscriptsinmemorytoskipcompilation;2)DatacachingusingMemc


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
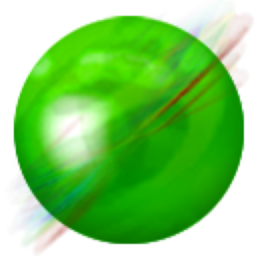
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SublimeText3 Linux new version
SublimeText3 Linux latest version
