MySQL string types impact storage and performance as follows: 1) CHAR is fixed-length, always using the same storage space, which can be faster but less space-efficient. 2) VARCHAR is variable-length, more space-efficient but potentially slower. 3) TEXT is for large text, stored outside rows, which may slow queries. 4) ENUM is efficient for fixed values but hard to modify. Best practices include using CHAR for fixed-length data, VARCHAR for variable-length, TEXT for large text, being cautious with ENUM, indexing wisely, normalizing data, and considering collations and prefix indexes for optimization.
When it comes to MySQL, choosing the right string type can significantly impact your database's performance and storage efficiency. So, let's dive into the world of MySQL string types, exploring their storage mechanisms, performance implications, and some best practices that can save you from common pitfalls.
Let's start by tackling the burning question: how do different MySQL string types affect storage and performance, and what are the best practices to follow? MySQL offers various string types like CHAR, VARCHAR, TEXT, and ENUM, each with unique characteristics that can influence your database's efficiency. Understanding these nuances is crucial for optimizing your database design.
Take CHAR and VARCHAR, for example. CHAR is fixed-length, meaning it always uses the same amount of storage space regardless of the actual data length. If you define a CHAR(10), it will always take up 10 bytes, even if you store a string like "hi". On the other hand, VARCHAR is variable-length, so a VARCHAR(10) storing "hi" would only use 3 bytes (2 for the length prefix and 1 for the data). This difference can be a game-changer for storage efficiency, especially in large databases.
But it's not just about storage. Performance-wise, CHAR can be faster for operations because the database knows exactly how much space to allocate. However, VARCHAR can be more space-efficient, which is a trade-off you need to consider based on your specific use case.
Now, let's talk about TEXT types. These are ideal for storing large amounts of text, but they come with their own set of considerations. TEXT types are stored outside of the row data, which can lead to additional I/O operations and potentially slower query performance. If you're dealing with large text fields, you might want to think about whether you really need to store all that data in the database or if you could offload some of it to external storage.
ENUM is another interesting type. It's great for when you have a fixed set of values, like status codes or country codes. ENUMs are stored internally as numbers, which can be more efficient than storing strings. However, be cautious with ENUMs because changing the list of allowed values can be a headache.
Now, let's look at some code to illustrate these concepts. Here's an example of how you might define different string types in a table:
CREATE TABLE example_table ( id INT AUTO_INCREMENT PRIMARY KEY, fixed_length CHAR(10), variable_length VARCHAR(255), long_text TEXT, status ENUM('active', 'inactive', 'pending') );
In this table, fixed_length
uses CHAR, variable_length
uses VARCHAR, long_text
uses TEXT, and status
uses ENUM. When designing your tables, consider the nature of the data you're storing and choose the appropriate type accordingly.
As for best practices, here are some tips to keep in mind:
- Use CHAR for fixed-length data: If you know your data will always be the same length, like country codes or status flags, CHAR can be more efficient.
- Choose VARCHAR for variable-length data: For fields where the length can vary, like names or addresses, VARCHAR is usually the better choice.
- Use TEXT for large text fields: If you need to store large amounts of text, like article content or user comments, TEXT is the way to go. But consider if you really need to store all that data in the database.
- Be cautious with ENUM: ENUM can be efficient, but changing the list of allowed values can be cumbersome. Use it sparingly and only when you're sure the list won't change frequently.
- Index wisely: If you frequently search or sort by a string column, consider adding an index. But remember, indexing large TEXT fields can be costly in terms of performance and storage.
- Normalize your data: Sometimes, breaking down large text fields into smaller, more manageable pieces can improve performance and make your data easier to work with.
One common pitfall to watch out for is overusing TEXT types. It's tempting to use TEXT for everything, but this can lead to bloated databases and slower performance. Always evaluate if a smaller type like VARCHAR would suffice.
Another thing to consider is the impact of collations. MySQL uses collations to determine how to compare and sort strings. Choosing the right collation can affect query performance and the results of string operations. For example, if you're working with international data, you might need to use a Unicode collation like utf8mb4_unicode_ci
.
In terms of performance optimization, one technique to consider is using prefix indexes on VARCHAR fields. Instead of indexing the entire field, you can index just the first few characters, which can save space and improve query performance. Here's how you might do that:
CREATE TABLE users ( id INT AUTO_INCREMENT PRIMARY KEY, username VARCHAR(255), INDEX username_prefix (username(10)) );
In this example, we're indexing only the first 10 characters of the username
field. This can be especially useful for fields where the beginning of the string is most important for searching or sorting.
Finally, let's talk about some real-world experience. I once worked on a project where we had a large table with a VARCHAR(255) field for user comments. Over time, this field grew to contain thousands of characters, leading to performance issues. We ended up splitting the comments into multiple fields and using TEXT for the longer content, which significantly improved our query performance. It was a lesson in the importance of choosing the right data type and being willing to refactor as your data grows.
In conclusion, understanding MySQL string types and their implications for storage and performance is crucial for building efficient databases. By choosing the right type, following best practices, and being mindful of potential pitfalls, you can optimize your database design and ensure it performs well under load. Remember, there's no one-size-fits-all solution, so always consider your specific use case and data patterns when making these decisions.
The above is the detailed content of MySQL String Types: Storage, Performance, and Best Practices. For more information, please follow other related articles on the PHP Chinese website!
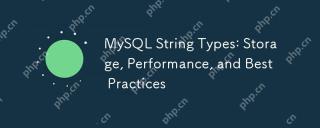
MySQLstringtypesimpactstorageandperformanceasfollows:1)CHARisfixed-length,alwaysusingthesamestoragespace,whichcanbefasterbutlessspace-efficient.2)VARCHARisvariable-length,morespace-efficientbutpotentiallyslower.3)TEXTisforlargetext,storedoutsiderows,
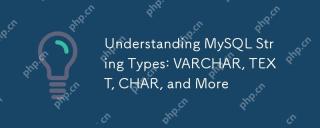
MySQLstringtypesincludeVARCHAR,TEXT,CHAR,ENUM,andSET.1)VARCHARisversatileforvariable-lengthstringsuptoaspecifiedlimit.2)TEXTisidealforlargetextstoragewithoutadefinedlength.3)CHARisfixed-length,suitableforconsistentdatalikecodes.4)ENUMenforcesdatainte
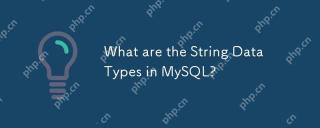
MySQLoffersvariousstringdatatypes:1)CHARforfixed-lengthstrings,2)VARCHARforvariable-lengthtext,3)BINARYandVARBINARYforbinarydata,4)BLOBandTEXTforlargedata,and5)ENUMandSETforcontrolledinput.Eachtypehasspecificusesandperformancecharacteristics,sochoose
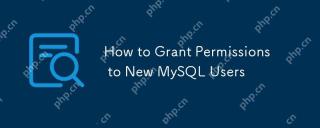
TograntpermissionstonewMySQLusers,followthesesteps:1)AccessMySQLasauserwithsufficientprivileges,2)CreateanewuserwiththeCREATEUSERcommand,3)UsetheGRANTcommandtospecifypermissionslikeSELECT,INSERT,UPDATE,orALLPRIVILEGESonspecificdatabasesortables,and4)
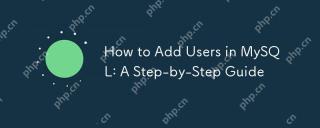
ToaddusersinMySQLeffectivelyandsecurely,followthesesteps:1)UsetheCREATEUSERstatementtoaddanewuser,specifyingthehostandastrongpassword.2)GrantnecessaryprivilegesusingtheGRANTstatement,adheringtotheprincipleofleastprivilege.3)Implementsecuritymeasuresl
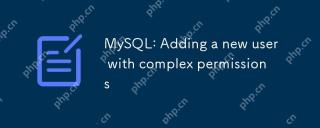
ToaddanewuserwithcomplexpermissionsinMySQL,followthesesteps:1)CreatetheuserwithCREATEUSER'newuser'@'localhost'IDENTIFIEDBY'password';.2)Grantreadaccesstoalltablesin'mydatabase'withGRANTSELECTONmydatabase.TO'newuser'@'localhost';.3)Grantwriteaccessto'
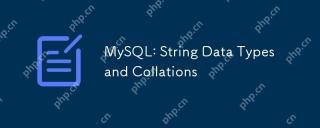
The string data types in MySQL include CHAR, VARCHAR, BINARY, VARBINARY, BLOB, and TEXT. The collations determine the comparison and sorting of strings. 1.CHAR is suitable for fixed-length strings, VARCHAR is suitable for variable-length strings. 2.BINARY and VARBINARY are used for binary data, and BLOB and TEXT are used for large object data. 3. Sorting rules such as utf8mb4_unicode_ci ignores upper and lower case and is suitable for user names; utf8mb4_bin is case sensitive and is suitable for fields that require precise comparison.
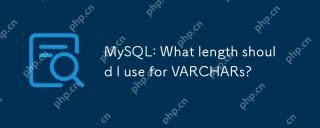
The best MySQLVARCHAR column length selection should be based on data analysis, consider future growth, evaluate performance impacts, and character set requirements. 1) Analyze the data to determine typical lengths; 2) Reserve future expansion space; 3) Pay attention to the impact of large lengths on performance; 4) Consider the impact of character sets on storage. Through these steps, the efficiency and scalability of the database can be optimized.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
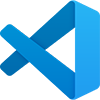
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

Atom editor mac version download
The most popular open source editor
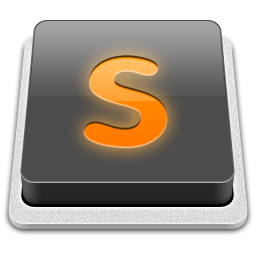
SublimeText3 Mac version
God-level code editing software (SublimeText3)
