Key traits of a successful composer include: 1) rich creativity and imagination, 2) solid technical skills and tools. These traits are similar to creative and structured thinking in programming, helping composers realize creativity and optimize their work in music creation.
introduction
As a programming master, what I want to talk about today is an interesting topic related to music - the traits of successful composers. Why? Because I found that programming and composition had surprising similarities in creative and structured thinking. By exploring the qualities of successful composers, we can not only better understand the art of music creation, but also draw inspiration from it and apply it to our programming practices. After reading this article, you will learn about the key traits that a successful composer possesses and think about how these traits combine with our growth as programmers.
Review of basic knowledge
Before we dive into the qualities of successful composers, let’s first look at the basics of music creation. Composers express their thoughts and emotions through elements such as melody, harmony, rhythm, etc. Just like when we use variables, functions, and algorithms when we program, these elements are the basic building blocks of music. Composers need to have a deep understanding of these elements, just as we need to understand the grammar and logic of programming languages.
In addition, music creation is also inseparable from the support of tools and technologies. Modern composers may use digital audio workstations (DAWs) such as Ableton Live or Logic Pro to create and produce music. As programmers, we also rely on various IDEs and development tools to improve our productivity.
Core concept or function analysis
The traits of a successful composer: creativity and imagination
A successful composer first needs to have rich creativity and imagination. They are able to conceive unique melody and harmonic combinations in their minds, which is similar to the need to conceive algorithms and solutions when programming. Creativity not only comes from the flash of inspiration, but also comes from the in-depth understanding and continuous practice of music theory.
// Simulation of the creative process class Composer { private String melody; private String harmony; <pre class='brush:php;toolbar:false;'>public void createMelody() { melody = "C4 D4 E4 C4 E4 D4 G4"; // Simple melody} public void createHarmony() { harmony = "Cmaj7 Dmin7 G7"; // Harmony} public void compose() { createMelody(); createHarmony(); System.out.println("Composed: " melody " with " harmony); }
}
public class Main { public static void main(String[] args) { Composer composer = new Composer(); composer.compose(); // Output: Composed: C4 D4 E4 C4 E4 D4 G4 with Cmaj7 Dmin7 G7 } }
This simple Java code example shows the abstraction of the composition process. We can see that composers need to transform creativity into concrete melodies and harmonies, just as we transform algorithmic ideas into executable code.
The traits of a successful composer: Mastery of technical skills and tools
In addition to creativity, a successful composer also needs solid technical skills and proficiency in tools. They need to be able to use a variety of instruments and music software, which is similar to what we need to master programming languages and development tools. Technical skills include not only the ability to play and produce music, but also the in-depth understanding and application of music theory.
// Simulated class DAW using DAW { private String track; <pre class='brush:php;toolbar:false;'>public void record(String instrument, String notes) { track = instrument ": " notes; } public void mix() { System.out.println("Mixing: " track); }
}
public class Main { public static void main(String[] args) { DAW daw = new DAW(); daw.record("Piano", "C4 D4 E4"); daw.mix(); // Output: Mixing: Piano: C4 D4 E4 } }
This code example shows how a composer can record and mix using DAW. We can see that mastering technical skills and tools is the key to achieving creativity, just like we need to master various libraries and frameworks in programming.
Example of usage
Basic usage: melody creation
Let's look at a simple example of melody creation. The composer might start with a basic scale and then enrich the melody by adding decorative notes and variations.
// Melody Creator Example class MelodyCreator { private String[] scale = {"C4", "D4", "E4", "F4", "G4", "A4", "B4"}; <pre class='brush:php;toolbar:false;'>public String createMelody() { StringBuilder melody = new StringBuilder(); for (int i = 0; i < 8; i ) { melody.append(scale[i % scale.length]).append(" "); } return melody.toString().trim(); }
}
public class Main { public static void main(String[] args) { MelodyCreator creator = new MelodyCreator(); String melody = creator.createMelody(); System.out.println("Melody: " melody); // Output: Melody: C4 D4 E4 F4 G4 A4 B4 C4 } }
This code example shows how to generate a melody from a basic scale. We can see that composers need to have a basic understanding of music theory in order to create meaningful melodies.
Advanced Usage: Harmonic Orchestration
In more advanced musical creation, composers need to consider the arrangement of harmony. Harmony not only enhances the expressiveness of the melody, but also adds depth and complexity to the music.
// Harmony Arranger { private String[] chords = {"Cmaj7", "Dmin7", "Em7", "Fmaj7", "G7", "Am7", "Bdim"}; <pre class='brush:php;toolbar:false;'>public String arrangementHarmony(String melody) { StringBuilder harmony = new StringBuilder(); String[] notes = melody.split(" "); for (int i = 0; i < notes.length; i ) { harmony.append(chords[i % chords.length]).append(" "); } return harmony.toString().trim(); }
}
public class Main { public static void main(String[] args) { HarmonyArranger arranger = new HarmonyArranger(); String melody = "C4 D4 E4 F4 G4 A4 B4 C4"; String harmony = arranger.arrangeHarmony(melody); System.out.println("Harmony: " harmony); // Output: Harmony: Cmaj7 Dmin7 Em7 Fmaj7 G7 Am7 Bdim Cmaj7 } }
This code example shows how to arrange harmony according to melody. We can see that harmonic choreography requires a deeper understanding of music theory, which is similar to the need for understanding complex data structures and algorithms in programming.
Common Errors and Debugging Tips
In music creation, composers may encounter common mistakes, such as melody discord, excessive harmony, etc. Here are some common errors and their debugging tips:
Melody discord : It can be solved by simplifying the melody or adjusting the relationship between notes. For example, simpler scales can be used or decorative sounds can be used.
Harmony is too complex : it can be solved by reducing the number of chords or choosing simpler chords. For example, basic major and minor triads can be used instead of complex seventh chords.
// Example of debugging melody discord class MelodyDebugger { public String simplifyMelody(String melody) { String[] notes = melody.split(" "); StringBuilder simplified = new StringBuilder(); for (String note : notes) { if (!note.contains("#") && !note.contains("b")) { simplified.append(note).append(" "); } } return simplified.toString().trim(); } } <p>public class Main { public static void main(String[] args) { MelodyDebugger debugger = new MelodyDebugger(); String melody = "C4 D4# E4 F4# G4 A4 B4 C5"; String simplified = debugger.simplifyMelody(melody); System.out.println("Simplified Melody: " simplified); // Output: Simplified Melody: C4 D4 E4 F4 G4 A4 B4 } }</p>
This code example shows how to debug the problem of melody discord by simplifying the melody. We can see that debugging skills are very important in music creation and programming.
Performance optimization and best practices
In music creation, composers need to consider how to optimize their work to achieve the best performance. Here are some recommendations for optimization and best practices:
Melody Fluency : Ensure the smoothness and coherence of the melody, which can be achieved by reusing the theme melody or using transition sections.
The simplicity of harmony : Keeping the simplicity of harmony can be achieved by choosing simpler chords or reducing the number of chords.
Diversity of rhythm : Increase the fun and expressiveness of the music by using different rhythm patterns.
// Example of optimizing melody fluency class MelodyOptimizer { public String optimizeMelody(String melody) { String[] notes = melody.split(" "); StringBuilder optimized = new StringBuilder(); for (int i = 0; i public class Main { public static void main(String[] args) { MelodyOptimizer optimizer = new MelodyOptimizer(); String melody = "C4 D4 E4 F4 G4 A4 B4 C5"; String optimized = optimizer.optimizeMelody(melody); System.out.println("Optimized Melody: " optimized); // Output: Optimized Melody: C4 D4 E4 F4 C4 A4 B4 C5 } }
This code example shows how to optimize the fluency of a melody by reusing the theme melody. We can see that optimization and best practices are crucial in music creation and programming.
In short, the traits of a successful composer include not only creativity and imagination, but also the mastery of technical skills and tools. These traits have many similarities to our growth as programmers. By understanding and learning these traits, we not only enjoy music better, but also draw inspiration from it and improve our programming skills.
The above is the detailed content of The Attributes of a Successful Composer. For more information, please follow other related articles on the PHP Chinese website!

Composer itself does not include AI capabilities, but can be enhanced by AI tools. 1) AI can analyze composer.json files, and it is recommended to optimize dependencies and predict version conflicts. 2) AI-driven platforms such as GitHubCopilot can provide real-time code suggestions to improve development efficiency. When using AI tools, you need to verify and adjust them in combination with actual situations.

The key traits of a successful composer include: 1) rich creativity and imagination, 2) solid mastery of technical skills and tools. These traits are similar to creative and structured thinking in programming, helping composers realize creativity and optimize their work in music creation.

To become a composer, you need to master music theory, instrumental performance, be familiar with music style and history, and be creative and inspiring. Specific steps include: 1. Learn music theory, such as chord structure and rhythm mode; 2. Master the performance of musical instruments and improve creative inspiration; 3. Be familiar with music production software, such as AbletonLive, to improve creative efficiency; 4. Continuous practice and adjustment, create complex melodies and use discordant chords to increase music tension.

Composer is a dependency management tool for PHP, and manages project dependencies through composer.json file. 1) parse composer.json to obtain dependency information; 2) parse dependencies to form a dependency tree; 3) download and install dependencies from Packagist to the vendor directory; 4) generate composer.lock file to lock the dependency version to ensure team consistency and project maintainability.

AI can show its strengths in the field of music creation. 1) AI generates music through machine learning and deep learning, enhancing diversity and innovation. 2) AI composers can assist composers and provide inspiration and creativity. 3) In actual applications, performance needs to be optimized to solve the problems of coherence and innovation in the generation of music.

We need Composer because it can effectively manage dependencies of PHP projects and avoid the hassle of version conflicts and manual library management. Composer declares dependencies through composer.json and uses composer.lock to ensure the version consistency, simplifying the dependency management process and improving project stability and development efficiency.

AI can help optimize the use of Composer. Specific methods include: 1. Dependency management optimization: AI analyzes dependencies, recommends the best version combination, and reduces conflicts. 2. Automated code generation: AI generates composer.json files that conform to best practices. 3. Improve code quality: AI detects potential problems, provides optimization suggestions, and improves code quality. These methods are implemented through machine learning and natural language processing technologies to help developers improve efficiency and code quality.

To become a composer, you need to master music theory, harmonization, counterpoint, and be familiar with the tone and performance skills of the instrument. Composers express emotions and stories through music, and the creative process involves the construction and improvement of ideas to works.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
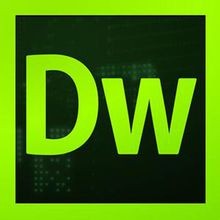
Dreamweaver CS6
Visual web development tools
