The core role of SQL in data analysis is to extract valuable information from the database through query statements. 1) Basic usage: Use GROUP BY and SUM functions to calculate the total order amount for each customer. 2) Advanced usage: Use CTE and subqueries to find the product with the highest sales per month. 3) Common errors: syntax errors, logic errors and performance problems. 4) Performance optimization: Use indexes, avoid SELECT * and optimize JOIN operations. Through these tips and practices, SQL can help us extract insights from our data and ensure queries are efficient and easy to maintain.
introduction
In a data-driven world, SQL (Structured Query Language) is not only a query language, but also a powerful tool for us to extract insights from massive data. Today, we will explore in-depth how to use SQL for data analysis and reveal the stories hidden behind the data. Whether you are a data analyst, business analyst, or a developer interested in data, this article will provide you with basic to advanced SQL data analysis skills to help you better understand and utilize data.
Review of basic knowledge
SQL is the standard language for interacting with databases, which allows us to query, insert, update and delete data. In data analysis, we mainly focus on query operations, extracting the required information from the database through SELECT statements. Understanding table structure, JOIN operations and aggregate functions is the basis for effective data analysis.
For example, suppose we have a sales database that contains order tables and customer tables. We can associate these two tables through the JOIN operation to obtain order information for each customer.
Core concept or function analysis
The role of SQL in data analysis
The core role of SQL in data analysis is to extract valuable information from the database through query statements. It not only helps us answer specific questions, such as "What is the total sales in a certain month", but also reveals trends and patterns in the data through complex queries.
For example, we can use SQL to calculate monthly sales and sort by monthly by GROUP BY and ORDER BY:
SELECT DATE_TRUNC('month', order_date) AS month, SUM(total_amount) AS monthly_sales FROM orders GROUP BY DATE_TRUNC('month', order_date) ORDER BY month;
How SQL query works
The working principle of SQL query can be simplified to the following steps:
- Analysis : The SQL engine parses the query statement and generates a query plan.
- Optimization : The query optimizer optimizes query plans based on statistics and index conditions.
- Execution : Execute the optimized query plan and extract data from the database.
- Return result : Return the query result to the user.
Understanding these steps helps us write more efficient queries. For example, the rational use of indexes can significantly improve query performance.
Example of usage
Basic usage
Let's start with a simple example, suppose we want to know the total order amount for each customer:
SELECT customer_id, SUM(total_amount) AS total_spent FROM orders GROUP BY customer_id;
This query uses GROUP BY to group by customers and calculates the total consumption amount for each customer using the SUM function.
Advanced Usage
Now, let's look at a more complex example, suppose we want to find the product with the highest sales per month:
WITH monthly_sales AS ( SELECT DATE_TRUNC('month', order_date) AS month, product_id, SUM(total_amount) AS sales FROM orders GROUP BY DATE_TRUNC('month', order_date), product_id ) SELECT month, product_id, Sales FROM monthly_sales m1 WHERE sales = ( SELECT MAX(sales) FROM monthly_sales m2 WHERE m2.month = m1.month ) ORDER BY month;
This query uses common table expressions (CTEs) and subqueries to find products with the highest sales per month. This approach, while complex, provides deeper insights.
Common Errors and Debugging Tips
Common errors when using SQL for data analysis include:
- Syntax error : For example, forget to use the semicolon end statement, or use a column name that does not exist.
- Logical error : For example, the JOIN condition was used incorrectly, resulting in incorrect results.
- Performance issues : For example, unused indexes result in slow query speed.
Methods to debug these problems include:
- Use EXPLAIN : View the query plan and understand the query execution path.
- Step-by-step debugging : Split complex queries into multiple simple queries and gradually verify the results.
- Using test data : Test queries on small-scale datasets to ensure the logic is correct.
Performance optimization and best practices
In practical applications, it is crucial to optimize SQL queries to improve performance. Here are some optimization tips:
- Using Index : Create indexes for frequently queried columns can significantly improve query speed.
- **Avoid using SELECT ***: Select only the required columns to reduce the amount of data transmission.
- Optimize JOIN operations : Make sure that the JOIN conditions use the index and minimize the number of JOINs.
For example, suppose we have a large order table and we can optimize the query by creating indexes for customer_id
and order_date
:
CREATE INDEX idx_customer_id ON orders(customer_id); CREATE INDEX idx_order_date ON orders(order_date);
In addition, writing SQL code that is readable and maintained is also part of best practice. For example, using meaningful aliases and comments can make the code easier to understand and maintain:
-- Calculate the total order amount for each customer SELECT c.customer_id, SUM(o.total_amount) AS total_spent FROM customers c JOIN orders o ON c.customer_id = o.customer_id GROUP BY c.customer_id;
Through these techniques and practices, we not only extract valuable insights from our data, but also ensure our queries are efficient and easy to maintain.
SQL is an indispensable tool for us in the journey of data analysis. I hope this article can help you better grasp SQL and reveal the story behind the data.
The above is the detailed content of SQL and Data Analysis: Extracting Insights from Information. For more information, please follow other related articles on the PHP Chinese website!

The diversity and power of SQL make it a powerful tool for data processing. 1. The basic usage of SQL includes data query, insertion, update and deletion. 2. Advanced usage covers multi-table joins, subqueries, and window functions. 3. Common errors include syntax, logic and performance issues, which can be debugged by gradually simplifying queries and using EXPLAIN commands. 4. Performance optimization tips include using indexes, avoiding SELECT* and optimizing JOIN operations.

The core role of SQL in data analysis is to extract valuable information from the database through query statements. 1) Basic usage: Use GROUPBY and SUM functions to calculate the total order amount for each customer. 2) Advanced usage: Use CTE and subqueries to find the product with the highest sales per month. 3) Common errors: syntax errors, logic errors and performance problems. 4) Performance optimization: Use indexes, avoid SELECT* and optimize JOIN operations. Through these tips and practices, SQL can help us extract insights from our data and ensure queries are efficient and easy to maintain.

The role of SQL in database management includes data definition, operation, control, backup and recovery, performance optimization, and data integrity and consistency. 1) DDL is used to define and manage database structures; 2) DML is used to operate data; 3) DCL is used to manage access rights; 4) SQL can be used for database backup and recovery; 5) SQL plays a key role in performance optimization; 6) SQL ensures data integrity and consistency.

SQLisessentialforinteractingwithrelationaldatabases,allowinguserstocreate,query,andmanagedata.1)UseSELECTtoextractdata,2)INSERT,UPDATE,DELETEtomanagedata,3)Employjoinsandsubqueriesforadvancedoperations,and4)AvoidcommonpitfallslikeomittingWHEREclauses

SQLisnotinherentlydifficulttolearn.Itbecomesmanageablewithpracticeandunderstandingofdatastructures.StartwithbasicSELECTstatements,useonlineplatformsforpractice,workwithrealdata,learndatabasedesign,andengagewithSQLcommunitiesforsupport.

MySQL is a database system, and SQL is the language for operating databases. 1.MySQL stores and manages data and provides a structured environment. 2. SQL is used to query, update and delete data, and flexibly handle various query needs. They work together, optimizing performance and design are key.

The difference between SQL and MySQL is that SQL is a language used to manage and operate relational databases, while MySQL is an open source database management system that implements these operations. 1) SQL allows users to define, operate and query data, and implement it through commands such as CREATETABLE, INSERT, SELECT, etc. 2) MySQL, as an RDBMS, supports these SQL commands and provides high performance and reliability. 3) The working principle of SQL is based on relational algebra, and MySQL optimizes performance through mechanisms such as query optimizers and indexes.

The core function of SQL query is to extract, filter and sort information from the database through SELECT statements. 1. Basic usage: Use SELECT to query specific columns from the table, such as SELECTname, departmentFROMemployees. 2. Advanced usage: Combining subqueries and ORDERBY to implement complex queries, such as finding employees with salary above average and sorting them in descending order of salary. 3. Debugging skills: Check for syntax errors, use small-scale data to verify logical errors, and use the EXPLAIN command to optimize performance. 4. Performance optimization: Use indexes, avoid SELECT*, and use subqueries and JOIN reasonably to improve query efficiency.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor
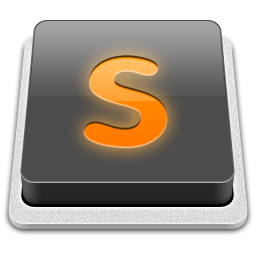
SublimeText3 Mac version
God-level code editing software (SublimeText3)

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
