How to create responsive web applications using Bootstrap and React? By combining Bootstrap's CSS framework and React's componentized architecture, modern, flexible and easy to maintain can be created. The specific steps include: 1) Importing the CSS file of Bootstrap and using its class to style React components; 2) Using React's componentization to manage state and logic; 3) Loading Bootstrap styles as needed to optimize performance; 4) Creating a dynamic interface using React's Hooks and Bootstrap's JavaScript components.
introduction
Do you want to know how to leverage Bootstrap and React to create responsive web applications? In this article, we will dive into how to combine these two powerful tools to create modern, flexible and easy-to-maintain web applications. You will learn how to use Bootstrap's CSS framework and React's component architecture to build a user interface that can be perfectly displayed on various devices.
The combination of Bootstrap and React can be said to be the golden partner in front-end development. The former provides a powerful CSS framework, while the latter is famous for its efficient component development methods. With this article, you will not only learn about their respective strengths, but also see how they work together to create amazing responsive web applications.
Review of basic knowledge
Bootstrap is an open source CSS framework designed to help developers quickly build responsive websites. It contains a set of predefined CSS classes and JavaScript components, greatly simplifying the front-end development process. React is a JavaScript library developed by Facebook, focusing on building user interfaces. The core concept of React is component development. Each component is independent and can be reused, which makes the maintenance and extension of code extremely simple.
When combining Bootstrap and React, you can use Bootstrap's CSS class to quickly define the style of components, and React is responsible for managing the state and logic of components. This combination not only improves development efficiency, but also enhances the readability and maintainability of the code.
Core concept or function analysis
The combination of Bootstrap and React
The combination of Bootstrap and React is mainly reflected in using Bootstrap's CSS class to style React components. The advantage of this approach is that you can take advantage of Bootstrap's responsive design without having to write complex CSS code from scratch. At the same time, React's componentized architecture allows you to easily apply Bootstrap styles to various components.
Let's look at a simple example showing how to use Bootstrap's style in React:
import React from 'react'; import 'bootstrap/dist/css/bootstrap.min.css'; <p>const ButtonComponent = () => { Return ( <button classname="btn btn-primary">Click me</button> ); };</p><p> export default ButtonComponent;</p>
In this example, we import the CSS file of Bootstrap and use btn
and btn-primary
classes to style a button component. This is the basic principle of combining Bootstrap and React.
How it works
When you use Bootstrap in React, you are actually using React's componentized architecture to manage Bootstrap's styles. React components are responsible for managing the state and logic of the component, while Bootstrap's CSS class is responsible for the style and layout of the component. This separation makes the code structure clearer and easier to maintain.
In terms of performance, Bootstrap's CSS files may increase the loading time of the page, especially on mobile devices. Therefore, when using Bootstrap, it is recommended to use on-demand loading method to only load the style files you actually need. In addition, React's virtual DOM can help you optimize the rendering performance of components and ensure the smoothness of the user interface.
Example of usage
Basic usage
Let's look at a more complex example showing how to create a responsive navigation bar using Bootstrap in React:
import React from 'react'; import 'bootstrap/dist/css/bootstrap.min.css'; <p>const Navbar = () => { Return ( <nav classname="navbar navbar-expand-lg navbar-light bg-light"> <a classname="navbar-brand" href="#">Navbar</a> <button classname="navbar-toggler" type="button" data-toggle="collapse" data-target="#navbarNav" aria-controls="navbarNav" aria-expanded="false" aria-label="Toggle navigation"> <span classname="navbar-toggler-icon"></span> </button> <div classname="collapse navbar-collapse" id="navbarNav"> <ul classname="navbar-nav"> <li classname="nav-item active"> <a classname="nav-link" href="#">Home <span classname="sr-only">(current)</span></a> </li> <li classname="nav-item"> <a classname="nav-link" href="#">Features</a> </li> <li classname="nav-item"> <a classname="nav-link" href="#">Pricing</a> </li> </ul> </div> </nav> ); };</p><p> export default Navbar;</p>
In this example, we use Bootstrap's navbar
class to create a responsive navigation bar. With navbar-expand-lg
class, we can ensure that the navigation bar collapses on small screen devices, thereby improving the user experience.
Advanced Usage
In more advanced usage, you can use React's Hooks and Bootstrap's JavaScript components to create dynamic user interfaces. For example, you can use useState
hook to manage the state of the component and combine the Bootstrap modal box component to create a popup window:
import React, { useState } from 'react'; import 'bootstrap/dist/css/bootstrap.min.css'; <p>const ModalExample = () => { const [showModal, setShowModal] = useState(false);</p><p> const handleOpenModal = () => setShowModal(true); const handleCloseModal = () => setShowModal(false);</p><p> Return ( </p><div> <button type="button" classname="btn btn-primary" onclick="{handleOpenModal}"> Launch demo modal </button><pre class='brush:php;toolbar:false;'> <div className={`modal ${showModal ? 'show' : ''}`} style={{display: showModal ? 'block' : 'none'}} tabIndex="-1" role="dialog"> <div className="modal-dialog" role="document"> <div className="modal-content"> <div className="modal-header"> <h5 id="Modal-title">Modal title</h5> <button type="button" className="close" onClick={handleCloseModal}> <span aria-hidden="true"></span> </button> </div> <div className="modal-body"> <p>Modal body text goes here.</p> </div> <div className="modal-footer"> <button type="button" className="btn btn-secondary" onClick={handleCloseModal}>Close</button> <button type="button" className="btn btn-primary">Save changes</button> </div> </div> </div> </div> </div>
); };
export default ModalExample;
In this example, we use the useState
hook to manage the display state of the modal box and combine the modal box component of Bootstrap to create a dynamic popup window. This approach not only improves the user experience, but also demonstrates the power of the combination of React and Bootstrap.
Common Errors and Debugging Tips
When using Bootstrap and React, you may encounter some common errors such as style conflicts or component rendering issues. Here are some common errors and their solutions:
Style conflict : Sometimes Bootstrap's styles may conflict with your custom styles, causing confusing page layout. To avoid this, it is recommended to use Scoped CSS or CSS Modules to isolate the style of the component. In addition, you can also use Bootstrap's custom build tool to load only the style files you need, thereby reducing the possibility of style conflicts.
Component rendering problem : In React, the rendering of components may be affected by state changes. If you find that a component is not rendering correctly, first check whether the component's status is updated correctly. Second, make sure that the component's lifecycle method (such as
componentDidMount
oruseEffect
) is called correctly to ensure that the component is rendered at the correct point in time.
Performance optimization and best practices
Performance optimization and best practices are crucial when building responsive web applications using Bootstrap and React. Here are some suggestions:
Loading on demand : In order to improve the loading speed of the page, it is recommended to use loading on demand to only load the Bootstrap style files you actually need. You can use tools such as Webpack to implement this function.
Optimize component rendering : In React, the rendering performance of components directly affects the user experience. To optimize component rendering, you can use
React.memo
oruseMemo
hooks to avoid unnecessary re-rendering. In addition, the rational use ofshouldComponentUpdate
method can also help you control the rendering behavior of the component.Code readability and maintenance : Always keep the code readability and maintenance when writing code. Use meaningful component names and variable names to write detailed comments and follow a consistent code style. With these best practices, you can ensure that your code is not only efficient, but is also easy to maintain and scale.
In practical applications, the combination of Bootstrap and React can help you quickly build responsive and efficient web applications. With the introduction and examples of this article, you should have mastered how to use these two tools to create a modern user interface. I hope these knowledge and skills can play a role in your development process and I wish you a happy development!
The above is the detailed content of Bootstrap and React: Creating Responsive Web Applications. For more information, please follow other related articles on the PHP Chinese website!

Bootstrap is an open source front-end framework that simplifies web development. 1. It is based on HTML, CSS, JavaScript, and provides predefined styles and components. 2. Use predefined classes and JavaScript plug-ins to implement responsive layout and interactive functions. 3. The basic usage is to introduce CSS and JavaScript files, use classes to create navigation bars, etc. 4. Advanced usage includes custom complex layouts. 5. Check the introduction of class names and files during debugging and use developer tools. 6. The optimization suggestion is to only introduce necessary files, use CDN, and use LESS or Sass when customizing.

How to create responsive web applications using Bootstrap and React? By combining Bootstrap's CSS framework and React's componentized architecture, modern, flexible and easy to maintain can be created. The specific steps include: 1) Importing the CSS file of Bootstrap and using its class to style React components; 2) Using React's componentization to manage state and logic; 3) Loading Bootstrap styles as needed to optimize performance; 4) Creating a dynamic interface using React's Hooks and Bootstrap's JavaScript components.

Bootstrap is an open source front-end framework that helps developers quickly build responsive websites. 1) It provides predefined styles and components such as grid systems and navigation bars. 2) Implement style and dynamic interaction through CSS and JavaScript files. 3) The basic usage is to introduce files and build pages with class names. 4) Advanced usage includes custom styles through Sass. 5) Frequently asked questions include style conflicts and JavaScript component issues, which can be solved through developer tools and modular management. 6) Performance optimization is recommended to selectively introduce modules and rationally use grid systems.

React and Bootstrap are ideal combinations. 1) Use Bootstrap's CSS classes and JavaScript components, 2) integrate through React-Bootstrap or reactstrap, 3) load and optimize rendering performance on demand, and build an efficient and beautiful user interface.

Bootstrap is an open source front-end framework for creating modern, responsive, and user-friendly websites. 1) It provides grid systems and predefined styles to simplify layout and development. 2) Mobile-first design ensures compatibility and performance. 3) Through custom styles and components, the website can be personalized. 4) Performance optimization and best practices include selective loading and responsive images. 5) Common errors such as layout problems and style conflicts can be resolved through debugging techniques.

Bootstrap is an open source front-end framework developed by Twitter, suitable for building responsive websites quickly. 1) Its grid system is based on a 12-column structure, allowing for the creation of flexible layouts. 2) Responsive design function enables the website to adapt to different devices. 3) The basic usage includes building a navigation bar, and the advanced usage involves card components. 4) Common errors such as misuse of grid systems can be avoided by correctly setting the column width. 5) Performance optimization includes loading only necessary components, using CDN and file compression. 6) Best practices emphasize tidy code, custom styles and responsive design.

The reason for combining Bootstrap and React is their complementarity: 1. Bootstrap provides predefined styles and components to simplify UI design; 2. React improves efficiency and performance through component development and virtual DOM. Use it in conjunction to enjoy fast UI construction and complex interaction management.

Bootstrap is an open source front-end framework based on HTML, CSS and JavaScript, designed to help developers quickly build responsive websites. Its design philosophy is "mobile first", providing a wealth of predefined components and tools, such as grid systems, buttons, forms, navigation bars, etc., simplifying the front-end development process, improving development efficiency, and ensuring the responsiveness and consistency of the website. Using Bootstrap can start with a simple page and gradually add advanced components such as cards and modal boxes. Best practices for optimizing performance include customizing Bootstrap, using CDNs, and avoiding overuse of class names.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

Zend Studio 13.0.1
Powerful PHP integrated development environment
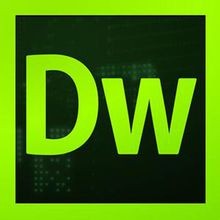
Dreamweaver CS6
Visual web development tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

Notepad++7.3.1
Easy-to-use and free code editor
