


What is the use of session_start() and session_destroy() functions in PHP?
In PHP, session_start()
and session_destroy()
are essential functions used for managing sessions, which allow a server to store and retrieve data related to a specific user across multiple page requests. Here's a detailed explanation of their uses:
-
session_start(): This function initiates a new session or resumes an existing one. It must be called at the beginning of every PHP page where you want to access or set session variables. When
session_start()
is called, it looks for a session ID passed in a cookie or URL and loads the associated session data. If no session ID is found, a new one is generated, and a new session is created. This function is crucial because it allows PHP to store and retrieve session variables ($_SESSION
) across different pages. -
session_destroy(): This function is used to terminate a session. When called, it destroys all of the data registered to a session. It does not, however, automatically delete the session cookie, which means the session ID can still be passed to the server in subsequent requests unless you manually delete the session cookie using
setcookie()
. It's typically used when a user logs out or when you want to ensure that no session data persists.
Both functions play a critical role in managing user sessions and maintaining state in stateless HTTP environments.
How can I effectively manage user sessions in PHP using session_start() and session_destroy()?
To manage user sessions effectively in PHP using session_start()
and session_destroy()
, follow these steps:
-
Initiate a Session: At the beginning of your PHP script (before any output is sent to the browser), call
session_start()
. This should be done on every page where you intend to use session data.<?php session_start(); // Now you can set or retrieve session data $_SESSION['username'] = 'JohnDoe';
-
Set and Retrieve Session Data: Use the
$_SESSION
superglobal array to set and retrieve data. This data is stored on the server and associated with the user's session ID.$_SESSION['username'] = 'JohnDoe'; // Set session data $username = $_SESSION['username']; // Retrieve session data
-
Destroy a Session: When you need to end a session (e.g., when a user logs out), use
session_destroy()
. Remember to unset all session variables and delete the session cookie to prevent session fixation attacks.session_start(); session_unset(); // Unset all session variables session_destroy(); // Destroy the session setcookie(session_name(), '', time() - 3600); // Delete session cookie
-
Regenerate Session ID: To enhance security, periodically regenerate the session ID using
session_regenerate_id()
. This helps mitigate session fixation attacks.session_start(); session_regenerate_id(true); // Regenerate session ID, delete old session file
-
Timeout and Expiration: Configure session timeouts using
session.gc_maxlifetime
to automatically destroy inactive sessions. This helps manage resource usage on the server.
By following these practices, you can effectively manage user sessions, maintain state across requests, and enhance security.
What are the security implications of using session_start() and session_destroy() in PHP applications?
The security implications of using session_start()
and session_destroy()
in PHP applications include the following:
-
Session Fixation Attacks: If an attacker can manipulate the session ID, they can hijack a user's session.
session_start()
does not change the session ID unlesssession_regenerate_id()
is called. Failing to regenerate the session ID after authentication can leave applications vulnerable. -
Session Hijacking: Since
session_start()
uses the session ID sent by the client, if this ID is intercepted or stolen, an attacker could use it to gain unauthorized access to a user's session. Secure transmission of session IDs (e.g., over HTTPS) and proper session management practices can mitigate this risk. - Session Data Tampering: Session data stored on the server can be tampered with if the server's security is compromised. Ensuring server security and possibly encrypting sensitive session data can mitigate this risk.
-
Incomplete Session Termination: When using
session_destroy()
, not deleting the session cookie can leave the session ID active, potentially allowing session fixation attacks. Manually deleting the session cookie aftersession_destroy()
is crucial. - Denial of Service (DoS) Attacks: If session data is not properly managed, an attacker could create numerous sessions to exhaust server resources. Regular cleanup of expired sessions and proper session configuration can help prevent this.
By understanding and addressing these security implications, you can enhance the security of PHP applications that use session management.
What common mistakes should be avoided when implementing session management with session_start() and session_destroy() in PHP?
When implementing session management in PHP using session_start()
and session_destroy()
, common mistakes to avoid include:
-
Calling session_start() After Output:
session_start()
must be called before any output is sent to the browser because it needs to set or read the session cookie. Outputting data before callingsession_start()
will result in a "headers already sent" error. -
Not Regenerating the Session ID: After a user logs in, failing to call
session_regenerate_id()
can leave the application vulnerable to session fixation attacks. Always regenerate the session ID following a successful authentication. -
Failing to Unset All Session Variables: Simply calling
session_destroy()
does not unset all session variables. Usesession_unset()
beforesession_destroy()
to clear all session data. -
Not Deleting the Session Cookie: After calling
session_destroy()
, manually delete the session cookie to ensure the session ID is not reused. This can be done withsetcookie(session_name(), '', time() - 3600)
. -
Ignoring Session Configuration: Not setting proper session configuration settings like
session.gc_maxlifetime
can lead to issues with session expiration and resource management on the server. - Insecure Transmission of Session IDs: Always use HTTPS to transmit session IDs. Failing to do so can allow session IDs to be intercepted during transmission.
- Overusing Sessions: Storing large amounts of data in sessions can strain server resources. Store only necessary data in sessions, and consider alternative storage mechanisms for large data sets.
By avoiding these common mistakes, you can implement more secure and efficient session management in your PHP applications.
The above is the detailed content of What is the use of session_start() and session_destroy() functions in PHP?. For more information, please follow other related articles on the PHP Chinese website!
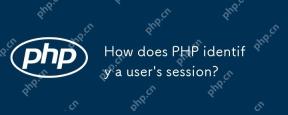
PHPidentifiesauser'ssessionusingsessioncookiesandsessionIDs.1)Whensession_start()iscalled,PHPgeneratesauniquesessionIDstoredinacookienamedPHPSESSIDontheuser'sbrowser.2)ThisIDallowsPHPtoretrievesessiondatafromtheserver.
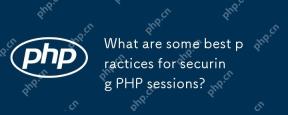
The security of PHP sessions can be achieved through the following measures: 1. Use session_regenerate_id() to regenerate the session ID when the user logs in or is an important operation. 2. Encrypt the transmission session ID through the HTTPS protocol. 3. Use session_save_path() to specify the secure directory to store session data and set permissions correctly.
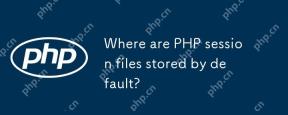
PHPsessionfilesarestoredinthedirectoryspecifiedbysession.save_path,typically/tmponUnix-likesystemsorC:\Windows\TemponWindows.Tocustomizethis:1)Usesession_save_path()tosetacustomdirectory,ensuringit'swritable;2)Verifythecustomdirectoryexistsandiswrita
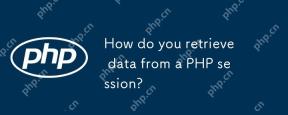
ToretrievedatafromaPHPsession,startthesessionwithsession_start()andaccessvariablesinthe$_SESSIONarray.Forexample:1)Startthesession:session_start().2)Retrievedata:$username=$_SESSION['username'];echo"Welcome,".$username;.Sessionsareserver-si
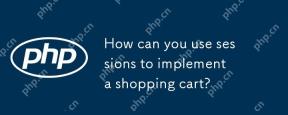
The steps to build an efficient shopping cart system using sessions include: 1) Understand the definition and function of the session. The session is a server-side storage mechanism used to maintain user status across requests; 2) Implement basic session management, such as adding products to the shopping cart; 3) Expand to advanced usage, supporting product quantity management and deletion; 4) Optimize performance and security, by persisting session data and using secure session identifiers.
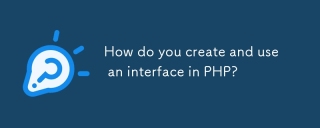
The article explains how to create, implement, and use interfaces in PHP, focusing on their benefits for code organization and maintainability.
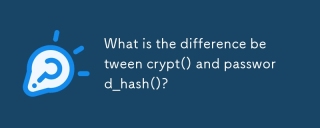
The article discusses the differences between crypt() and password_hash() in PHP for password hashing, focusing on their implementation, security, and suitability for modern web applications.
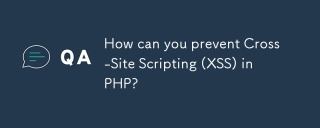
Article discusses preventing Cross-Site Scripting (XSS) in PHP through input validation, output encoding, and using tools like OWASP ESAPI and HTML Purifier.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SublimeText3 Chinese version
Chinese version, very easy to use

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
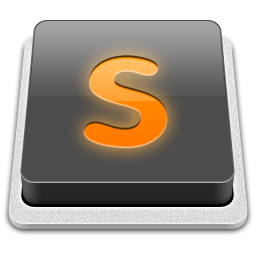
SublimeText3 Mac version
God-level code editing software (SublimeText3)
