What is the difference between / and // in Python?
In Python, the operators /
and //
are used for division, but they serve different purposes and produce different results.
-
/ (Division Operator): The
/
operator performs "true division" or "float division." It always returns a floating-point number, regardless of the types of the operands. For example,5 / 2
will return2.5
. This operator is used when you need the exact quotient of the division, including the fractional part. -
// (Floor Division Operator): The
//
operator performs "floor division." It returns the largest possible integer that is less than or equal to the result of the division. For example,5 // 2
will return2
. If the operands are both integers, the result will be an integer. If at least one of the operands is a floating-point number, the result will be a floating-point number, but it will still be rounded down to the nearest integer. For example,5.0 // 2
will return2.0
.
In summary, /
always returns a float, while //
returns the floor of the division result, which can be an integer or a float depending on the types of the operands.
What are the use cases for the division and floor division operators in Python?
The division and floor division operators in Python have distinct use cases based on the type of result needed:
-
Division Operator (/):
- Exact Quotient: When you need the exact result of a division, including the fractional part. This is useful in scenarios where precision is important, such as in financial calculations, scientific computations, or any situation where you need to know the exact ratio between two numbers.
- Floating-Point Arithmetic: When working with floating-point numbers and you need to perform division that results in a floating-point number. For example, calculating the average of a set of numbers.
-
Floor Division Operator (//):
-
Integer Division: When you need the result of a division to be an integer, such as when calculating the number of items that can fit into a container without considering the remainder. For example, if you have 10 apples and want to distribute them into bags that can hold 3 apples each,
10 // 3
will tell you that you can fill 3 bags. - Rounding Down: When you need to round down the result of a division to the nearest integer. This is useful in scenarios where you need to ensure that the result does not exceed a certain threshold, such as calculating the number of pages needed to print a document where each page can hold a certain number of words.
-
Performance Optimization: In some cases, using
//
can be more efficient than using/
and then converting the result to an integer, especially when working with large datasets or in performance-critical code.
-
Integer Division: When you need the result of a division to be an integer, such as when calculating the number of items that can fit into a container without considering the remainder. For example, if you have 10 apples and want to distribute them into bags that can hold 3 apples each,
How does Python handle division with integers versus floating-point numbers?
Python's handling of division depends on the types of the operands and the operator used:
-
Division Operator (/):
-
Integers: When both operands are integers, Python 3 will return a floating-point number. For example,
5 / 2
will return2.5
. In Python 2, this would return2
, but Python 2 is no longer supported, and this behavior is considered outdated. -
Floating-Point Numbers: When at least one of the operands is a floating-point number, the result will always be a floating-point number. For example,
5.0 / 2
or5 / 2.0
will both return2.5
.
-
Integers: When both operands are integers, Python 3 will return a floating-point number. For example,
-
Floor Division Operator (//):
-
Integers: When both operands are integers, the result will be an integer. For example,
5 // 2
will return2
. -
Floating-Point Numbers: When at least one of the operands is a floating-point number, the result will be a floating-point number, but it will be rounded down to the nearest integer. For example,
5.0 // 2
will return2.0
, and5 // 2.0
will also return2.0
.
-
Integers: When both operands are integers, the result will be an integer. For example,
In summary, the /
operator always returns a float, while the //
operator returns an integer if both operands are integers, and a float rounded down to the nearest integer if at least one operand is a float.
What are the potential pitfalls to watch out for when using // in Python?
When using the floor division operator //
in Python, there are several potential pitfalls to be aware of:
-
Loss of Precision: Since
//
rounds down to the nearest integer, you may lose precision if you need the fractional part of the result. For example,5 // 2
will return2
, but you might need2.5
for certain calculations. -
Unexpected Results with Negative Numbers: When working with negative numbers, the result of
//
can be counterintuitive. For example,-5 // 2
will return-3
, not-2
, because-3
is the largest integer less than or equal to-2.5
. This can lead to errors if you are not careful. -
Type Mismatch: If you are expecting an integer result but one of your operands is a float, you will get a float result instead. For example,
5.0 // 2
will return2.0
, not2
. This can cause issues if you are passing the result to a function that expects an integer. -
Performance Considerations: While
//
can be more efficient than/
followed by a conversion to an integer, in some cases, the performance difference may be negligible, and using//
might make your code less readable or more prone to errors. -
Compatibility Issues: If you are working with code that needs to be compatible with both Python 2 and Python 3, you need to be aware that the behavior of
/
with integer operands changed between the two versions. In Python 2,5 / 2
would return2
, while in Python 3, it returns2.5
. Using//
consistently can help avoid these issues, but you need to be aware of the differences.
By understanding these potential pitfalls, you can use the //
operator more effectively and avoid common mistakes in your Python code.
The above is the detailed content of What is the difference between / and // in Python?. For more information, please follow other related articles on the PHP Chinese website!
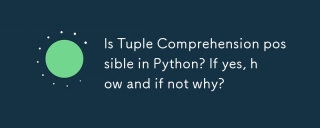
Article discusses impossibility of tuple comprehension in Python due to syntax ambiguity. Alternatives like using tuple() with generator expressions are suggested for creating tuples efficiently.(159 characters)
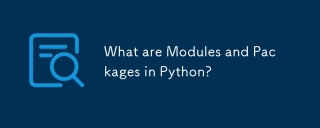
The article explains modules and packages in Python, their differences, and usage. Modules are single files, while packages are directories with an __init__.py file, organizing related modules hierarchically.
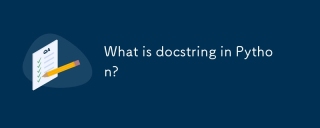
Article discusses docstrings in Python, their usage, and benefits. Main issue: importance of docstrings for code documentation and accessibility.
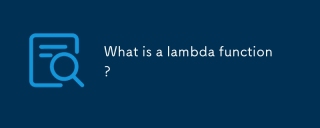
Article discusses lambda functions, their differences from regular functions, and their utility in programming scenarios. Not all languages support them.
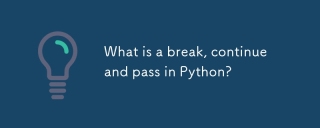
Article discusses break, continue, and pass in Python, explaining their roles in controlling loop execution and program flow.
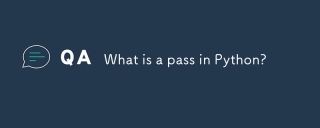
The article discusses the 'pass' statement in Python, a null operation used as a placeholder in code structures like functions and classes, allowing for future implementation without syntax errors.
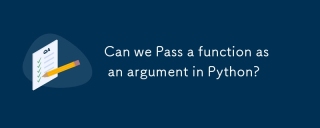
Article discusses passing functions as arguments in Python, highlighting benefits like modularity and use cases such as sorting and decorators.
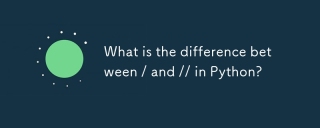
Article discusses / and // operators in Python: / for true division, // for floor division. Main issue is understanding their differences and use cases.Character count: 158


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
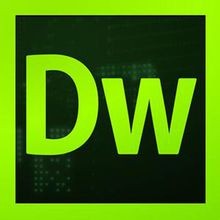
Dreamweaver CS6
Visual web development tools
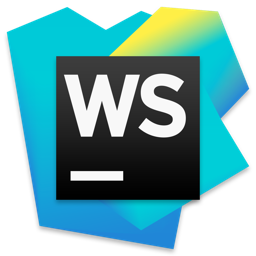
WebStorm Mac version
Useful JavaScript development tools

Atom editor mac version download
The most popular open source editor
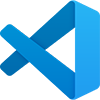
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
