The EXPLAIN statement can be used to analyze and improve SQL query performance. 1. Execute the EXPLAIN statement to view the query plan. 2. Analyze the output results, pay attention to access type, index usage and JOIN order. 3. Create or adjust indexes based on the analysis results, optimize JOIN operations, and avoid full table scanning to improve query efficiency.
introduction
In database optimization, how to efficiently analyze and improve query performance has always been a big concern for us programmers. Today, I want to talk to you about how to use EXPLAIN statements to peek into the internal mechanism of SQL queries, which is one of my secret weapons when tuning databases over the years. Through this article, you will learn how to use EXPLAIN statement to diagnose query performance problems, and master some practical skills that I have summarized from actual combat.
Review of basic knowledge
The EXPLAIN statement can be said to be a good helper for database tuning players. It can help us understand the decision-making process of the database when executing queries, such as what kind of index is selected, how to scan tables, and estimated execution costs. Simply put, EXPLAIN allows us to see the query plan, which is crucial for optimizing queries.
Before using EXPLAIN, make sure you have a certain understanding of the basic SQL syntax and database execution plan, such as what are indexes, table scanning, JOIN operations, etc. These are the basis for understanding the output of EXPLAIN.
Core concept or function analysis
Definition and function of EXPLAIN
The purpose of the EXPLAIN statement is to let you see what happens inside the database when executing your query. It returns a series of information to help you analyze the execution plan of the query and identify performance bottlenecks.
For example, in MySQL, you can use EXPLAIN like this:
EXPLAIN SELECT * FROM users WHERE age > 30;
This command will return the execution plan of the query, including the index used, the scanning method, the estimated number of rows, etc.
How it works
When you execute the EXPLAIN statement, the database analyzes your query and then generates an execution plan. This plan includes steps the database will take when executing a query, such as:
- Selected access method (such as index scan, full table scan)
- Estimated row count and cost
- Index used
- The order of JOIN operations
By analyzing this information, you can determine whether the query effectively utilizes the index, whether there are unnecessary full table scans, and whether the order of JOIN operations can be optimized.
When analyzing, pay special attention to type
field, which represents the access type. ALL
means full table scanning, with the worst performance; index
means index scanning, with average performance; ref
, eq_ref
, etc. represent search using indexes, with good performance.
Example of usage
Basic usage
Let's look at a simple example, suppose we have an orders
table and we want to find all orders with status shipped
:
EXPLAIN SELECT * FROM orders WHERE status = 'shipped';
The output might look like this:
---- ------------- -------- ------------ ------ --------------- ------ --------- ------- ------ ---------- ------- | id | select_type | table | partitions | type | possible_keys | key | key_len | ref | rows | filtered | Extra | ---- ------------- -------- ------------ ------ --------------- ------ --------- ------- ------ ---------- ------- | 1 | SIMPLE | orders | NULL | ALL | status_index | NULL | NULL | NULL | 1000 | 10.00 | Using where | ---- ------------- -------- ------------ ------ --------------- ------ --------- ------- ------ ---------- -------
From this output, we can see that the database has selected a full table scan ( type
is ALL
) and no index is used ( key
is NULL
). This suggests that we may need to create an index on status
field to optimize the query.
Advanced Usage
Sometimes, queries involve JOIN operations of multiple tables, and EXPLAIN can help us optimize the JOIN order. Suppose we have two tables, orders
and customers
, we want to query customer information for all shipped orders:
EXPLAIN SELECT c.name, o.order_date FROM customers c JOIN orders o ON c.id = o.customer_id WHERE o.status = 'shipped';
The output might look like this:
---- ------------- ------- ------------ ------ --------------- ---------- --------- ------------------- ------ ---------- ------------- | id | select_type | table | partitions | type | possible_keys | key | key_len | ref | rows | filtered | Extra | ---- ------------- ------- ------------ ------ --------------- ---------- --------- ------------------- ------ ---------- ------------- | 1 | SIMPLE | o | NULL | ref | status_index | status_index | 768 | const | 100 | 100.00 | Using where | | 1 | SIMPLE | c | NULL | eq_ref | PRIMARY | PRIMARY | 4 | db.o.customer_id | 1 | 100.00 | NULL | ---- ------------- ------- ------------ ------ --------------- ---------- --------- ------------------- ------ ---------- -------------
From the output, we can see that the database first scans the orders
table, and then looks up customers
table through customer_id
index. This JOIN order is reasonable because it first filters out orders that meet the criteria and then looks for the corresponding customer information.
Common Errors and Debugging Tips
When using EXPLAIN, there are some common misunderstandings that need to be noted:
- Misunderstanding output result : The output result of EXPLAIN needs to be interpreted in combination with actual situation. For example, the
rows
field represents the estimated number of rows, but may vary when actually executed. - Ignore JOIN order : When multi-table JOIN, the JOIN order has a great impact on performance. Through EXPLAIN, the JOIN order can be adjusted to optimize queries.
- Not using indexes : If EXPLAIN shows that there is no index used, you may need to create an index on the relevant fields.
Debugging skills include:
- Adjust index : Create or adjust indexes to optimize queries based on the output of EXPLAIN.
- Rewrite queries : Sometimes you can optimize performance by rewriting queries, such as avoiding subqueries.
- Analyze the JOIN order : Adjust the JOIN order through EXPLAIN to ensure that the minimum data is filtered out first and then perform the JOIN operation.
Performance optimization and best practices
In practical applications, how to use EXPLAIN to optimize query performance? Here are some suggestions I have summarized from years of experience:
- Index optimization : Based on the output of EXPLAIN, make sure that the query uses the appropriate index. If the index is not used, you may need to create an index on the relevant fields.
- Avoid full table scans : Try to avoid full table scans (
type
ALL
), which can be optimized by creating indexes or rewriting queries. - Optimize JOIN operations : Analyze the order of JOIN operations through EXPLAIN, ensuring that the minimum data is filtered out before performing JOIN operations.
- Using Overlay Index : Using Overlay Index (
Extra
field isUsing index
) can significantly improve query performance if possible.
In an actual project, I once encountered a case: a complex query involves JOIN operations of multiple tables. Through EXPLAIN analysis, it was found that the order of JOINs was unreasonable, resulting in performance problems. By adjusting the JOIN order and creating the appropriate index, the query time is finally reduced from minutes to seconds.
In short, the EXPLAIN statement is a powerful tool for us database tuners. Through it, we can have an in-depth understanding of the execution plan of the query, thereby finding performance bottlenecks and optimizing. I hope this article can help you better master the skills of EXPLAIN and improve query performance in actual projects.
The above is the detailed content of How can you use the EXPLAIN statement to analyze query performance?. For more information, please follow other related articles on the PHP Chinese website!

MySQL processes data replication through three modes: asynchronous, semi-synchronous and group replication. 1) Asynchronous replication performance is high but data may be lost. 2) Semi-synchronous replication improves data security but increases latency. 3) Group replication supports multi-master replication and failover, suitable for high availability requirements.
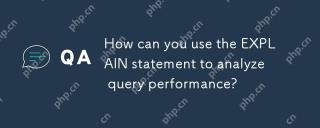
The EXPLAIN statement can be used to analyze and improve SQL query performance. 1. Execute the EXPLAIN statement to view the query plan. 2. Analyze the output results, pay attention to access type, index usage and JOIN order. 3. Create or adjust indexes based on the analysis results, optimize JOIN operations, and avoid full table scanning to improve query efficiency.
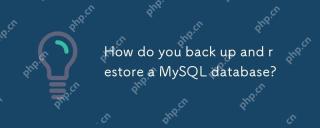
Using mysqldump for logical backup and MySQLEnterpriseBackup for hot backup are effective ways to back up MySQL databases. 1. Use mysqldump to back up the database: mysqldump-uroot-pmydatabase>mydatabase_backup.sql. 2. Use MySQLEnterpriseBackup for hot backup: mysqlbackup--user=root-password=password--backup-dir=/path/to/backupbackup. When recovering, use the corresponding life
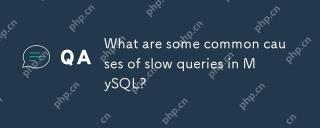
The main reasons for slow MySQL query include missing or improper use of indexes, query complexity, excessive data volume and insufficient hardware resources. Optimization suggestions include: 1. Create appropriate indexes; 2. Optimize query statements; 3. Use table partitioning technology; 4. Appropriately upgrade hardware.
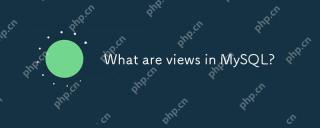
MySQL view is a virtual table based on SQL query results and does not store data. 1) Views simplify complex queries, 2) Enhance data security, and 3) Maintain data consistency. Views are stored queries in databases that can be used like tables, but data is generated dynamically.
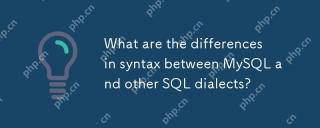
MySQLdiffersfromotherSQLdialectsinsyntaxforLIMIT,auto-increment,stringcomparison,subqueries,andperformanceanalysis.1)MySQLusesLIMIT,whileSQLServerusesTOPandOracleusesROWNUM.2)MySQL'sAUTO_INCREMENTcontrastswithPostgreSQL'sSERIALandOracle'ssequenceandt
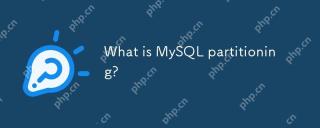
MySQL partitioning improves performance and simplifies maintenance. 1) Divide large tables into small pieces by specific criteria (such as date ranges), 2) physically divide data into independent files, 3) MySQL can focus on related partitions when querying, 4) Query optimizer can skip unrelated partitions, 5) Choosing the right partition strategy and maintaining it regularly is key.
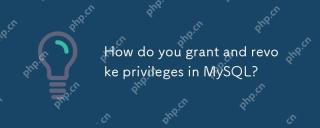
How to grant and revoke permissions in MySQL? 1. Use the GRANT statement to grant permissions, such as GRANTALLPRIVILEGESONdatabase_name.TO'username'@'host'; 2. Use the REVOKE statement to revoke permissions, such as REVOKEALLPRIVILEGESONdatabase_name.FROM'username'@'host' to ensure timely communication of permission changes.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
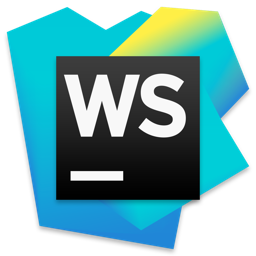
WebStorm Mac version
Useful JavaScript development tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
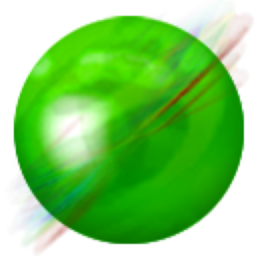
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SublimeText3 Chinese version
Chinese version, very easy to use

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
