Use Docker and Kubernetes to build scalable applications. 1) Create container images using Dockerfile, 2) Deployment and Service of Kubernetes through kubectl command, 3) Use Horizontal Pod Autoscaler to achieve automatic scaling, thereby building an efficient and scalable application architecture.
introduction
Do you want to know how to use Docker and Kubernetes to build scalable applications? This article will take you into the deep understanding of how to use these two container technologies to achieve this. We will start with the basic concepts and gradually deepen into practical operations and best practices to ensure that you can not only understand the principles of these tools, but also apply them flexibly in actual projects.
After reading this article, you will learn how to create and manage containers using Docker, and how to orchestrate them with Kubernetes to build an efficient and scalable application architecture.
Review of basic knowledge
Docker and Kubernetes are the cornerstones of modern application development and deployment. Docker allows us to package applications and their dependencies into a lightweight container, while Kubernetes provides powerful container orchestration capabilities, allowing us to manage these containers in complex distributed environments.
Docker containers can be regarded as a lightweight virtual machine, but they start quickly and consume less resources. Kubernetes helps us manage and expand these containers through concepts such as Pod, Service, and Deployment.
Core concept or function analysis
The definition and function of Docker
Docker is a leader in containerization technology, which allows developers to package applications and all their dependencies into one container. This container can run in any Docker-enabled environment, ensuring application consistency and portability.
# Use the official Node.js to mirror FROM node:14 # Set the working directory WORKDIR /usr/src/app # Copy package.json and package-lock.json COPY package*.json ./ # Install dependency on RUN npm install # Copy the application code COPY. . # Exposed port EXPOSE 3000 # Define the startup command CMD ["node", "app.js"]
This Dockerfile shows how to create a container image of a Node.js application. It defines where to get the basic image, how to set up the working directory, install dependencies, copy code, and how to start the app.
How Kubernetes works
How Kubernetes works can be simplified to the following steps: First, it creates pods based on your configuration files, such as YAML files, that contain one or more containers. Kubernetes then manages network access to these Pods through Service and manages the life cycle and extension of Pods through Deployment.
apiVersion: apps/v1 kind: Deployment metadata: name: my-app spec: replicas: 3 selector: matchLabels: app: my-app template: metadata: labels: app: my-app spec: containers: - name: my-app-container image: my-app:v1 Ports: - containerPort: 3000
This YAML file defines a Deployment that creates three pods running my-app:v1
image and ensures that these pods remain running at all times.
Example of usage
Build an application using Docker
Building an application with Docker is very simple. You just need to write a Dockerfile, then use the docker build
command to build the image, and then use the docker run
command to run the container.
# Build the image docker build -t my-app:v1. # Run container docker run -p 3000:3000 my-app:v1
This command will build an image called my-app:v1
and run a container locally, mapping the container's port 3000 to the host's port 3000.
Deploy apps using Kubernetes
Deploying an application in Kubernetes requires writing a YAML configuration file and then using the kubectl
command to apply these configurations.
# Create a Deployment kubectl apply -f deployment.yaml # Create Service kubectl apply -f service.yaml
These commands create Deployment and Service in the Kubernetes cluster based on the contents of deployment.yaml
and service.yaml
files.
Common Errors and Debugging Tips
Common errors when using Docker and Kubernetes include image building failure, container failure, Pod failure to schedule, etc. When debugging these problems, you can use the following tips:
- Use
docker logs
to view container logs and find out the reason for the startup failure. - Use
kubectl describe
command to view the details of the Pod or Service to understand its status and events. - Use
kubectl logs
to view container logs in the Pod to help diagnose application problems.
Performance optimization and best practices
When using Docker and Kubernetes, there are several ways to optimize the performance and maintainability of your application:
- Use multi-stage builds to reduce the image size, thus speeding up container startup.
- Rationally configure resource requests and restrictions to ensure that Pods can run efficiently in the cluster.
- Use Horizontal Pod Autoscaler to automatically scale Pods and adjust resources dynamically according to load.
apiVersion: autoscaling/v2beta1 kind: HorizontalPodAutoscaler metadata: name: my-app-hpa spec: scaleTargetRef: apiVersion: apps/v1 kind: Deployment name: my-app minReplicas: 1 maxReplicas: 10 metrics: - type: Resource resource: name: cpu targetAverageUtilization: 50
This YAML file defines a Horizontal Pod Autoscaler, which automatically adjusts the number of pods in my-app
Deployment based on CPU usage.
In actual projects, I found that using Docker and Kubernetes can not only improve the scalability of applications, but also greatly simplify development and operation and maintenance work. By using these tools reasonably, you can build an efficient and reliable application architecture that can easily meet demands of all sizes.
However, there are also some potential challenges and pitfalls to be paid attention to. For example, improper image management may lead to security issues, and complex Kubernetes configuration may increase operational and maintenance difficulties. Therefore, when using these tools, you need to constantly learn and practice to find the best solution for your project.
The above is the detailed content of Docker and Kubernetes: Building Scalable Applications. For more information, please follow other related articles on the PHP Chinese website!

Use Docker and Kubernetes to build scalable applications. 1) Create container images using Dockerfile, 2) Deployment and Service of Kubernetes through kubectl command, 3) Use HorizontalPodAutoscaler to achieve automatic scaling, thereby building an efficient and scalable application architecture.

The main difference between Docker and Kubernetes is that Docker is used for containerization, while Kubernetes is used for container orchestration. 1.Docker provides a consistent environment to develop, test and deploy applications, and implement isolation and resource limitation through containers. 2. Kubernetes manages containerized applications, provides automated deployment, expansion and management functions, and supports load balancing and automatic scaling. The combination of the two can improve application deployment and management efficiency.

Installing and configuring Docker on Linux requires ensuring that the system is 64-bit and kernel version 3.10 and above, use the command "sudoapt-getupdate" and install it with the command "sudoapt-getupdate" and verify it with "sudoapt-getupdate" and. Docker uses the namespace and control groups of the Linux kernel to achieve container isolation and resource limitation. The image is a read-only template, and the container can be modified. Examples of usage include running an Nginx server and creating images with custom Dockerfiles. common

The reason for using Docker is that it provides an efficient, portable and consistent environment to package, distribute, and run applications. 1) Docker is a containerized platform that allows developers to package applications and their dependencies into lightweight, portable containers. 2) It is based on Linux container technology and joint file system to ensure fast startup and efficient operation. 3) Docker supports multi-stage construction, optimizes image size and deployment speed. 4) Using Docker can simplify development and deployment processes, improve efficiency and ensure consistency across environments.

Docker's application scenarios in actual projects include simplifying deployment, managing multi-container applications and performance optimization. 1.Docker simplifies application deployment, such as using Dockerfile to deploy Node.js applications. 2. DockerCompose manages multi-container applications, such as web and database services in microservice architecture. 3. Performance optimization uses multi-stage construction to reduce the image size and monitor the container status through health checks.

Select Docker in a small project or development environment, and Kubernetes in a large project or production environment. 1.Docker is suitable for rapid iteration and testing, 2. Kubernetes provides powerful container orchestration capabilities, suitable for managing and expanding large applications.

Docker is important on Linux because Linux is its native platform that provides rich tools and community support. 1. Install Docker: Use sudoapt-getupdate and sudoapt-getinstalldocker-cedocker-ce-clicotainerd.io. 2. Create and manage containers: Use dockerrun commands, such as dockerrun-d--namemynginx-p80:80nginx. 3. Write Dockerfile: Optimize the image size and use multi-stage construction. 4. Optimization and debugging: Use dockerlogs and dockerex

Docker is a containerization tool, and Kubernetes is a container orchestration tool. 1. Docker packages applications and their dependencies into containers that can run in any Docker-enabled environment. 2. Kubernetes manages these containers, implementing automated deployment, scaling and management, and making applications run efficiently.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
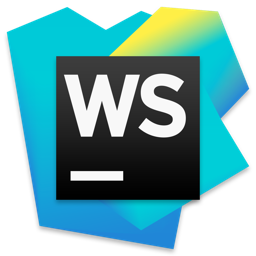
WebStorm Mac version
Useful JavaScript development tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

Notepad++7.3.1
Easy-to-use and free code editor
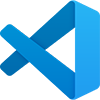
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
