


Scaling XML/RSS Processing: Performance Optimization Techniques
When processing XML and RSS data, you can optimize performance through the following steps: 1) Use efficient parsers such as lxml to improve parsing speed; 2) Use SAX parsers to reduce memory usage; 3) Use XPath expressions to improve data extraction efficiency; 4) implement multi-process parallel processing to improve processing speed.
introduction
Performance optimization becomes a key challenge when dealing with large-scale XML and RSS data. Whether you are developing a news aggregator or need to process large amounts of XML data for data analysis, how to process this data efficiently is crucial. This article will explore in-depth various performance optimization techniques that can be used when processing XML and RSS data. By reading this article, you will learn how to improve the performance of your XML/RSS handlers, avoid common performance bottlenecks, and master some practical best practices.
Review of basic knowledge
Processing XML and RSS data usually involves the process of parsing, transforming and extracting information. XML is a markup language used to store and transfer data, while RSS is an XML-based format used to publish frequently updated content, such as blog posts, news titles, etc. Common tools for processing this data include SAX (Simple API for XML) and DOM (Document Object Model) parsers, as well as specialized RSS parsing libraries.
When working with large-scale data, it is crucial to choose the right analytics method. The SAX parser processes data in a streaming manner and is suitable for handling large files because it does not require the entire document to be loaded into memory. The DOM parser loads the entire XML document into memory and forms a tree structure, suitable for situations where documents need to be frequently accessed and modified.
Core concept or function analysis
Performance optimization of XML/RSS processing
Performance optimization mainly involves the following aspects in XML/RSS processing: parsing speed, memory usage, data extraction efficiency and parallel processing capabilities.
Analysis speed
Parse speed is one of the core indicators of XML/RSS processing. Using efficient parsers such as Expat or libxml2 can significantly improve parsing speed. Here is an example of XML parsing using Python's lxml library:
from lxml import etree # Read XML file with open('example.xml', 'r') as file: xml_content = file.read() # parse XML root = etree.fromstring(xml_content) # Extract data for element in root.findall('.//item'): title = element.find('title').text print(title)
This example shows how to quickly parse XML files and extract data from them using the lxml library. The lxml library is based on libxml2 and has efficient parsing performance.
Memory usage
Memory usage is a special issue when dealing with large-scale XML files. Using a SAX parser can effectively reduce memory footprint, as it does not require the entire document to be loaded into memory. Here is an example using the SAX parser:
import xml.sax class MyHandler(xml.sax.ContentHandler): def __init__(self): self.current_data = "" self.title = "" def startElement(self, tag, attributes): self.current_data = tag def endElement(self, tag): if self.current_data == "title": print(self.title) self.current_data = "" def characters(self, content): if self.current_data == "title": self.title = content parser = xml.sax.make_parser() parser.setContentHandler(MyHandler()) parser.parse("example.xml")
This example shows how to use the SAX parser to process XML files, avoiding the risk of memory overflow.
Data extraction efficiency
When extracting data, selecting the appropriate XPath expression can significantly improve efficiency. XPath is a language used to navigate in XML documents that can quickly locate the required data. Here is an example of extracting data using XPath:
from lxml import etree # Read XML file with open('example.xml', 'r') as file: xml_content = file.read() # parse XML root = etree.fromstring(xml_content) # Use XPath to extract data titles = root.xpath('//item/title/text()') for title in titles: print(title)
This example shows how to use XPath to quickly extract data from XML, improving the efficiency of data extraction.
Parallel processing
When processing large-scale data, utilizing multi-threading or multi-processing can significantly improve processing speed. Here is an example of parallel processing using Python's multiprocessing library:
import multiprocessing from lxml import etree def process_chunk(chunk): root = etree.fromstring(chunk) titles = root.xpath('//item/title/text()') Return titles if __name__ == '__main__': with open('example.xml', 'r') as file: xml_content = file.read() # Divide XML files into multiple chunks chunks = [xml_content[i:i 100000] for i in range(0, len(xml_content), 100000)] # Use multiprocessing with multiprocessing.Pool(processes=4) as pool: results = pool.map(process_chunk, chunks) # Merge result all_titles = [title for chunk_result in results for title in chunk_result] for title in all_titles: print(title)
This example shows how to use multiple processes to process XML files in parallel, which improves processing speed.
Example of usage
Basic usage
When processing XML/RSS data, the most basic usage is to use a parser to read files and extract data. Here is an example of basic parsing using Python's xml.etree.ElementTree library:
import xml.etree.ElementTree as ET # Read XML file tree = ET.parse('example.xml') root = tree.getroot() # Extract data for item in root.findall('item'): title = item.find('title').text print(title)
This example shows how to use the ElementTree library for basic XML parsing and data extraction.
Advanced Usage
When dealing with complex XML/RSS data, more advanced techniques may be required, such as XPath expressions and namespace processing. Here is an example of processing using XPath and namespace:
from lxml import etree # Read XML file with open('example.xml', 'r') as file: xml_content = file.read() # parse XML root = etree.fromstring(xml_content) # Define namespace ns = {'atom': 'http://www.w3.org/2005/Atom'} # Use XPath to extract data titles = root.xpath('//atom:entry/atom:title/text()', namespaces=ns) for title in titles: print(title)
This example shows how to use XPath and namespace to process complex XML data, improving the flexibility of data extraction.
Common Errors and Debugging Tips
Common errors when processing XML/RSS data include parsing errors, namespace conflicts, and memory overflow. Here are some common errors and their debugging tips:
- Parse error : Use the try-except statement to capture the parse error and print the detailed error message. For example:
try: tree = etree.parse('example.xml') except etree.XMLSyntaxError as e: print(f"Parse error: {e}")
- Namespace conflict : Ensure that namespaces are correctly defined and used to avoid namespace conflicts. For example:
ns = {'atom': 'http://www.w3.org/2005/Atom'} titles = root.xpath('//atom:entry/atom:title/text()', namespaces=ns)
- Memory overflow : Use SAX parser to process large files to avoid memory overflow. For example:
import xml.sax class MyHandler(xml.sax.ContentHandler): def __init__(self): self.current_data = "" self.title = "" def startElement(self, tag, attributes): self.current_data = tag def endElement(self, tag): if self.current_data == "title": print(self.title) self.current_data = "" def characters(self, content): if self.current_data == "title": self.title = content parser = xml.sax.make_parser() parser.setContentHandler(MyHandler()) parser.parse("example.xml")
Performance optimization and best practices
In practical applications, the following aspects need to be considered for optimizing XML/RSS processing code:
Choose the right parser : Choose SAX or DOM parser according to the specific needs. SAX parsers are suitable for handling large files, while DOM parsers are suitable for situations where frequent access and modification of documents are required.
Using XPath Expression : XPath Expression can significantly improve the efficiency of data extraction and reduce code complexity.
Parallel processing : Use multi-threading or multi-processing to process data in parallel to improve processing speed.
Memory management : When processing large files, pay attention to memory usage to avoid memory overflow.
Code readability and maintenance : Write clear and readable code to facilitate subsequent maintenance and extension.
Here is an example that combines the above optimization techniques:
import multiprocessing from lxml import etree def process_chunk(chunk): root = etree.fromstring(chunk) titles = root.xpath('//item/title/text()') Return titles if __name__ == '__main__': with open('example.xml', 'r') as file: xml_content = file.read() # Divide XML files into multiple chunks chunks = [xml_content[i:i 100000] for i in range(0, len(xml_content), 100000)] # Use multiprocessing with multiprocessing.Pool(processes=4) as pool: results = pool.map(process_chunk, chunks) # Merge result all_titles = [title for chunk_result in results for title in chunk_result] for title in all_titles: print(title)
This example shows how to use multi-process, XPath expressions and memory management techniques to improve the performance of XML/RSS processing.
In practical applications, performance optimization is a continuous process that requires continuous adjustment and optimization according to specific needs and data characteristics. Hopefully, the techniques and practices provided in this article can help you achieve better performance when processing XML/RSS data.
The above is the detailed content of Scaling XML/RSS Processing: Performance Optimization Techniques. For more information, please follow other related articles on the PHP Chinese website!

When processing XML and RSS data, you can optimize performance through the following steps: 1) Use efficient parsers such as lxml to improve parsing speed; 2) Use SAX parsers to reduce memory usage; 3) Use XPath expressions to improve data extraction efficiency; 4) implement multi-process parallel processing to improve processing speed.

RSS2.0 is an open standard that allows content publishers to distribute content in a structured way. It contains rich metadata such as titles, links, descriptions, release dates, etc., allowing subscribers to quickly browse and access content. The advantages of RSS2.0 are its simplicity and scalability. For example, it allows custom elements, which means developers can add additional information based on their needs, such as authors, categories, etc.

RSS is an XML-based format used to publish frequently updated content. 1. RSSfeed organizes information through XML structure, including title, link, description, etc. 2. Creating RSSfeed requires writing in XML structure, adding metadata such as language and release date. 3. Advanced usage can include multimedia files and classified information. 4. Use XML verification tools during debugging to ensure that the required elements exist and are encoded correctly. 5. Optimizing RSSfeed can be achieved by paging, caching and keeping the structure simple. By understanding and applying this knowledge, content can be effectively managed and distributed.

RSS is an XML-based format used to publish and subscribe to content. The XML structure of an RSS file includes a root element, an element, and multiple elements, each representing a content entry. Read and parse RSS files through XML parser, and users can subscribe and get the latest content.

XML has the advantages of structured data, scalability, cross-platform compatibility and parsing verification in RSS. 1) Structured data ensures consistency and reliability of content; 2) Scalability allows the addition of custom tags to suit content needs; 3) Cross-platform compatibility makes it work seamlessly on different devices; 4) Analytical and verification tools ensure the quality and integrity of the feed.

The implementation of RSS in XML is to organize content through a structured XML format. 1) RSS uses XML as the data exchange format, including elements such as channel information and project list. 2) When generating RSS files, content must be organized according to specifications and published to the server for subscription. 3) RSS files can be subscribed through a reader or plug-in to automatically update the content.

Advanced features of RSS include content namespaces, extension modules, and conditional subscriptions. 1) Content namespace extends RSS functionality, 2) Extended modules such as DublinCore or iTunes to add metadata, 3) Conditional subscription filters entries based on specific conditions. These functions are implemented by adding XML elements and attributes to improve information acquisition efficiency.

RSSfeedsuseXMLtostructurecontentupdates.1)XMLprovidesahierarchicalstructurefordata.2)Theelementdefinesthefeed'sidentityandcontainselements.3)elementsrepresentindividualcontentpieces.4)RSSisextensible,allowingcustomelements.5)Bestpracticesincludeusing


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
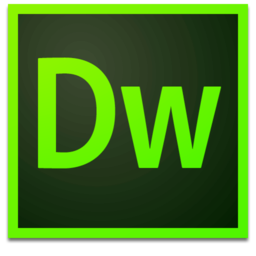
Dreamweaver Mac version
Visual web development tools

Notepad++7.3.1
Easy-to-use and free code editor

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
