Introduction
The backtracking algorithm is a powerful problem-solving technique that incrementally builds candidate solutions. It's a widely used method in computer science, systematically exploring all possible avenues before discarding any potentially unsuccessful strategies. This approach is particularly well-suited for puzzles, pathfinding, and constraint satisfaction problems. Mastering backtracking significantly enhances problem-solving capabilities.
Key Learning Objectives
This guide will cover:
- Grasping the fundamental concept of the backtracking algorithm.
- Applying backtracking to solve combinatorial challenges.
- Identifying real-world applications of this technique.
- Implementing backtracking solutions in coding exercises.
- Recognizing the limitations and potential difficulties of using backtracking.
Table of contents
- What is Backtracking?
- How Backtracking Functions?
- Coding Backtracking Solutions
- Optimal Use Cases for Backtracking
- Solving Sudoku with Backtracking
- Practical Applications of Backtracking
- Challenges and Limitations
- Frequently Asked Questions
What is Backtracking?
Backtracking is an algorithmic approach that constructs candidate solutions iteratively. If a candidate proves invalid, the algorithm "backtracks" to the previous step and explores alternative options. This process continues until a valid solution is found or all possibilities are exhausted.
How Backtracking Functions?
Backtracking is a decision-making algorithm that systematically explores possibilities, reversing decisions that lead to infeasible states. It's a form of depth-first search, building solutions incrementally and retracting steps when necessary.
Recursive Exploration and Decision Making
The algorithm starts from an initial state, making choices at each step. Each choice is added to the current solution, and the algorithm checks for constraint violations.
Constraint Validation and Backtracking
If constraints are satisfied, the algorithm continues; otherwise, it backtracks, undoing the last choice and trying alternatives. This ensures exhaustive exploration without getting trapped in invalid paths.
Solution Verification and Termination
The algorithm terminates when a valid solution is found or all possibilities are explored.
Also Read: What is the Water Jug Problem in AI?
Coding Backtracking Solutions
Here’s a Python example demonstrating backtracking for the N-Queens problem:
def is_safe(board, row, col): # Check for queen conflicts in the column, left diagonal, and right diagonal for i in range(row): if board[i][col] == 'Q' or (col-i-1 >= 0 and board[row-i-1][col-i-1] == 'Q') or (col i 1 <p></p><h2 id="Optimal-Use-Cases-for-Backtracking">Optimal Use Cases for Backtracking</h2> <p></p><p>Let's examine scenarios where backtracking shines.</p> <p></p><h3 id="Constraint-Based-Search-Problems">Constraint-Based Search Problems</h3> <p></p><p>Backtracking excels in scenarios requiring exhaustive search while adhering to specific constraints. For instance, in Sudoku, numbers must be unique within rows, columns, and 3x3 subgrids. Backtracking efficiently handles constraint violations by reverting incorrect placements.</p> <p></p><h3 id="Combinatorial-Problem-Solving">Combinatorial Problem Solving</h3> <p></p><p>When generating all permutations or combinations, backtracking systematically explores possibilities. The Eight Queens problem, placing eight queens on a chessboard without mutual threats, perfectly illustrates this application.</p> <p></p><h3 id="Optimization-Problem-Solving">Optimization Problem Solving</h3> <p></p><p>Backtracking is useful in optimization problems where the best choice among many must be found while satisfying constraints. The knapsack problem—selecting items to maximize value within a weight limit—benefits from backtracking's systematic exploration and constraint checking.</p> <p></p><h3 id="Pathfinding-and-Maze-Navigation">Pathfinding and Maze Navigation</h3> <p></p><p>Backtracking effectively navigates through spaces with obstacles. In maze solving, the algorithm explores paths, backtracking from dead ends to find a solution path.</p> <p></p><h3 id="Pattern-Matching-and-String-Manipulation">Pattern Matching and String Manipulation</h3> <p></p><p>Backtracking is valuable for tasks like regular expression matching, where it systematically checks different pattern matching possibilities against a string.</p> <p></p><h3 id="Game-Strategy-and-Decision-Making">Game Strategy and Decision Making</h3> <p></p><p>In game playing, backtracking can explore different move sequences, evaluating potential outcomes and retracting unsuccessful strategies.</p> <p></p><h2 id="Solving-Sudoku-with-Backtracking">Solving Sudoku with Backtracking</h2> <p></p><p>Sudoku, a number placement puzzle, is a classic backtracking problem.</p> <p></p><h4 id="Algorithmic-Breakdown">Algorithmic Breakdown</h4> <p></p><p>The backtracking Sudoku solver follows these steps:</p> <pre class="brush:php;toolbar:false"><code>1. **Locate Empty Cell:** Find the next empty cell (represented by 0). 2. **Try Numbers:** Attempt to place numbers 1-9 in the empty cell. 3. **Validate Placement:** Check if the placement is valid (no conflicts in row, column, or 3x3 subgrid). 4. **Recursive Call:** If valid, recursively call the solver to continue filling the grid. 5. **Backtrack:** If the recursive call fails (dead end), remove the number and try the next. 6. **Termination:** The algorithm stops when the grid is full or all possibilities are exhausted. </code>
Validation Function
def is_valid(board, row, col, num): # Check row, column, and 3x3 subgrid for conflicts # ... (implementation as before)
Sudoku Solver Function
def solve_sudoku(board): # Find empty cell, try numbers, validate, recurse, backtrack # ... (implementation as before)
Example and Solution
# Example board (0s are empty cells) sudoku_board = [ [5, 3, 0, 0, 7, 0, 0, 0, 0], # ... (rest of the board) ] # Solve and print the solution # ... (implementation as before)
Applications of Backtracking
Backtracking finds applications in diverse areas:
- Constraint Satisfaction Problems (CSPs): Sudoku, crossword puzzles, map coloring.
- Combinatorial Optimization: Knapsack problem, traveling salesman problem (approximation).
- Artificial Intelligence (AI): Game playing (chess, checkers), planning.
- Operations Research: Scheduling, resource allocation.
- Bioinformatics: Sequence alignment.
Challenges and Limitations
While powerful, backtracking has limitations:
- Exponential Complexity: The time required can grow exponentially with problem size.
- Inefficiency in Certain Cases: Other algorithms may be more efficient for specific problems.
- Pruning Difficulty: Effectively eliminating unproductive paths can be challenging.
- Memory Usage: Deep recursion can lead to high memory consumption.
- Sequential Nature: Difficult to parallelize effectively.
- Implementation Complexity: Can be complex to implement correctly for intricate problems.
Conclusion
Backtracking is a versatile algorithm for solving problems by systematically exploring possibilities and discarding infeasible solutions. While its exponential time complexity can be a limitation, its effectiveness in solving complex combinatorial problems makes it a valuable tool in a programmer's arsenal.
Frequently Asked Questions
Q1: What is backtracking in algorithms? A: A recursive problem-solving technique that explores all potential solutions, abandoning unpromising paths.
Q2: Common applications of backtracking? A: Sudoku, N-Queens, maze solving, constraint satisfaction problems.
Q3: Is backtracking always efficient? A: No, its exponential time complexity can make it inefficient for large problems.
Q4: How does backtracking differ from brute force? A: Backtracking prunes unpromising paths, while brute force tries all possibilities.
Q5: Does backtracking guarantee the optimal solution? A: Not necessarily; it finds a solution, but not always the best one.
The above is the detailed content of A Comprehensive Guide on Backtracking Algorithm. For more information, please follow other related articles on the PHP Chinese website!

Introduction Suppose there is a farmer who daily observes the progress of crops in several weeks. He looks at the growth rates and begins to ponder about how much more taller his plants could grow in another few weeks. From th
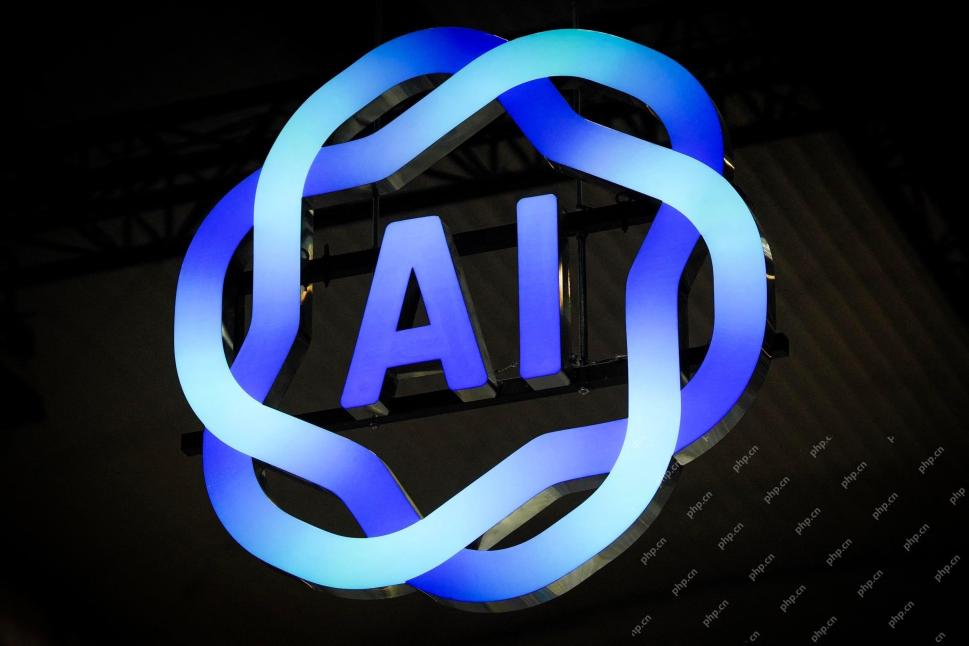
Soft AI — defined as AI systems designed to perform specific, narrow tasks using approximate reasoning, pattern recognition, and flexible decision-making — seeks to mimic human-like thinking by embracing ambiguity. But what does this mean for busine
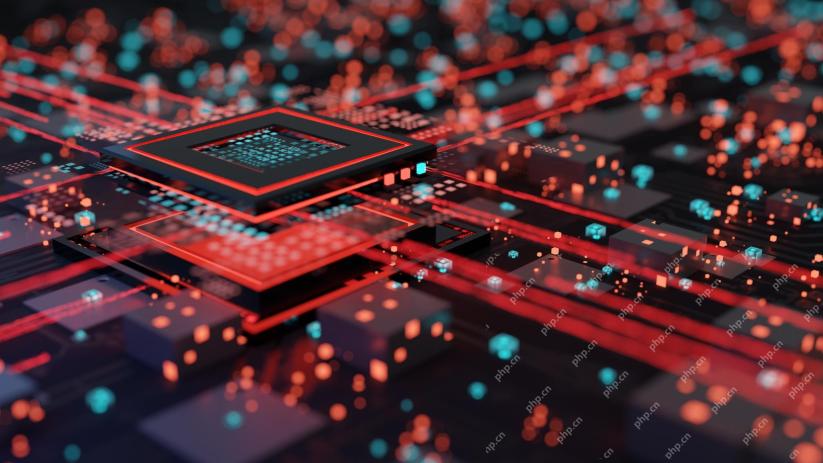
The answer is clear—just as cloud computing required a shift toward cloud-native security tools, AI demands a new breed of security solutions designed specifically for AI's unique needs. The Rise of Cloud Computing and Security Lessons Learned In th

Entrepreneurs and using AI and Generative AI to make their businesses better. At the same time, it is important to remember generative AI, like all technologies, is an amplifier – making the good great and the mediocre, worse. A rigorous 2024 study o
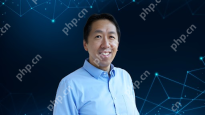
Unlock the Power of Embedding Models: A Deep Dive into Andrew Ng's New Course Imagine a future where machines understand and respond to your questions with perfect accuracy. This isn't science fiction; thanks to advancements in AI, it's becoming a r
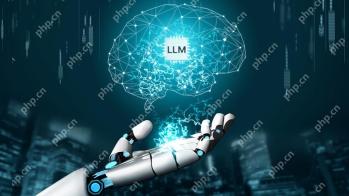
Large Language Models (LLMs) and the Inevitable Problem of Hallucinations You've likely used AI models like ChatGPT, Claude, and Gemini. These are all examples of Large Language Models (LLMs), powerful AI systems trained on massive text datasets to
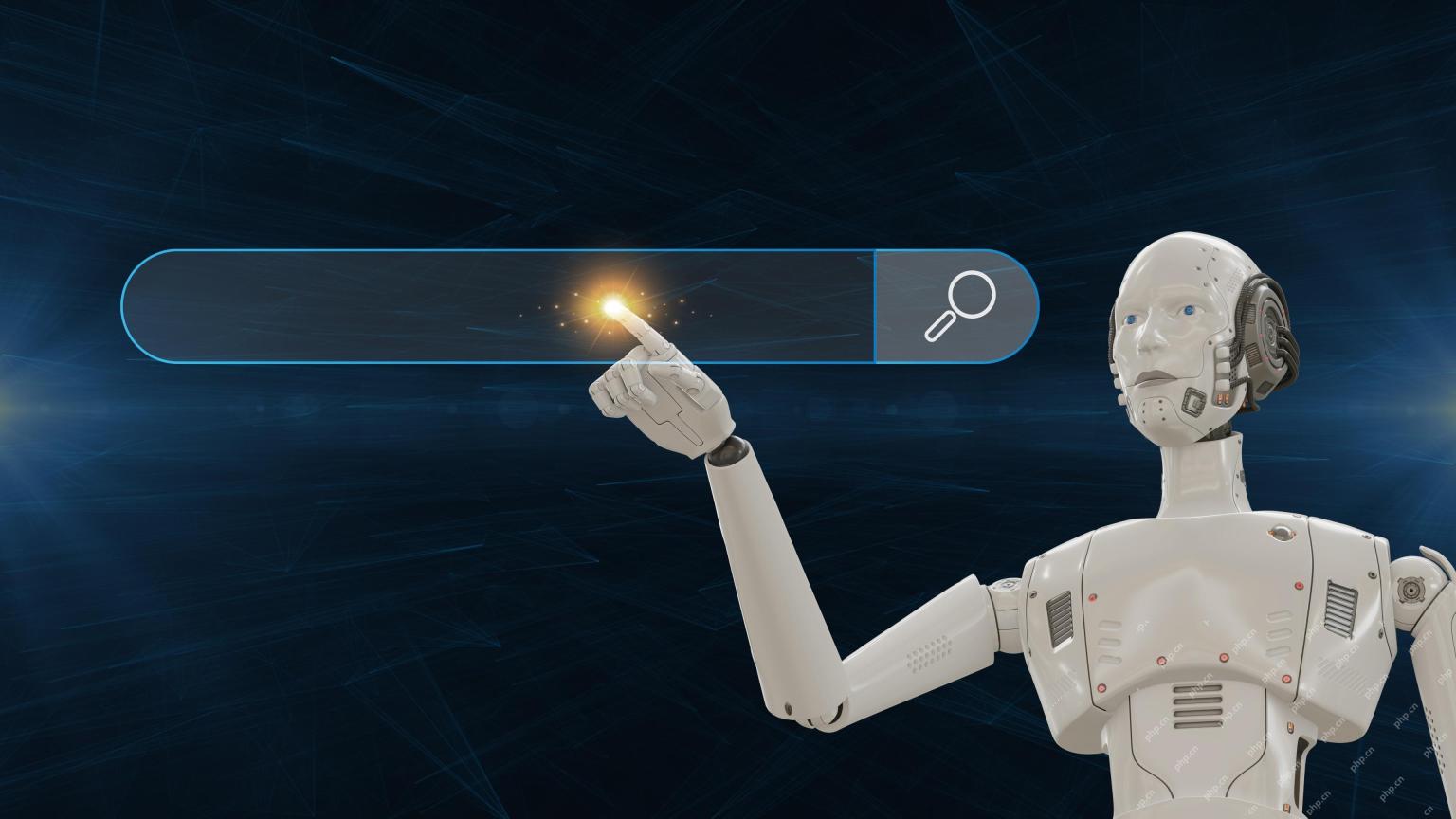
Recent research has shown that AI Overviews can cause a whopping 15-64% decline in organic traffic, based on industry and search type. This radical change is causing marketers to reconsider their whole strategy regarding digital visibility. The New
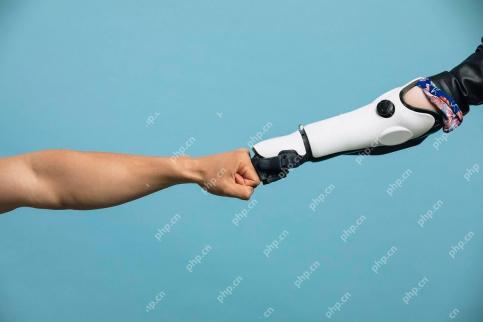
A recent report from Elon University’s Imagining The Digital Future Center surveyed nearly 300 global technology experts. The resulting report, ‘Being Human in 2035’, concluded that most are concerned that the deepening adoption of AI systems over t


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

Zend Studio 13.0.1
Powerful PHP integrated development environment

SublimeText3 Chinese version
Chinese version, very easy to use

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SublimeText3 English version
Recommended: Win version, supports code prompts!