


Explain the SPL SplFixedArray and its performance characteristics compared to regular PHP arrays.
SplFixedArray is a fixed-size array in PHP, suitable for scenarios where high performance and low memory usage are required. 1) It needs to specify the size when creating to avoid the overhead caused by dynamic adjustment. 2) Based on C language arrays, directly operate memory and fast access speed. 3) Suitable for large-scale data processing and memory-sensitive environments, but it needs to be used with caution because its size is fixed.
introduction
Today we will talk about SplFixedArray in PHP, this baby that sounds a bit unpopular. Why pay attention to it? Because in some scenarios, it can make your code run faster and use less memory. Through this article, you will learn about the basic concepts, usage of SplFixedArray, and its performance characteristics compared to ordinary PHP arrays. Ready to explore the world of PHP's performance optimization together?
Review of basic knowledge
In PHP, arrays are one of the most commonly used data structures in our daily development. They are flexible and easy to use, but sometimes they can bring performance challenges due to their dynamic characteristics. As part of SPL (Standard PHP Library), SplFixedArray provides a fixed-size array implementation, which in some cases can lead to significant performance improvements.
Core concept or function analysis
The definition and function of SplFixedArray
SplFixedArray is a fixed-size array that needs to be specified when created. Once created, its size cannot be changed. Its main purpose is to provide higher performance, especially when processing large-scale data. Why? Because it avoids the overhead of normal arrays when dynamically resize.
$fixedArray = new SplFixedArray(5); $fixedArray[0] = 'PHP'; $fixedArray[1] = 'SplFixedArray'; echo $fixedArray[0]; // Output PHP
This example shows how to create a SplFixedArray with 5 elements and set and read the values therein.
How it works
The internal implementation of SplFixedArray is based on C-language arrays, which means it manipulates memory directly, avoiding the hash table structure of PHP arrays. Its working principle can be simply described as:
- Allocate fixed-size continuous memory blocks during initialization
- Access elements directly through indexes, fast
- Due to the fixed size, no dynamic adjustment is required, which reduces the overhead of memory operations
This design can significantly improve performance when processing large amounts of data, especially in memory-sensitive environments.
Example of usage
Basic usage
Let's see how to do some basic operations using SplFixedArray:
$fixedArray = new SplFixedArray(3); $fixedArray[0] = 'Hello'; $fixedArray[1] = 'World'; $fixedArray[2] = '!'; // traverse SplFixedArray foreach ($fixedArray as $value) { echo $value . ' '; } // Output: Hello World!
This code shows how to create, fill and traverse SplFixedArray. Note that when traversing, we can directly use the foreach loop, which is similar to a normal array.
Advanced Usage
Now let’s take a look at some more advanced usages, such as using SplFixedArray for data caching:
class DataCache { private $cache; public function __construct(int $size) { $this->cache = new SplFixedArray($size); } public function set(int $index, $value) { if ($index >= 0 && $index < $this->cache->getSize()) { $this->cache[$index] = $value; } } public function get(int $index) { if ($index >= 0 && $index < $this->cache->getSize()) { return $this->cache[$index]; } return null; } } $cache = new DataCache(1000); $cache->set(0, 'Cached Data'); echo $cache->get(0); // Output: Cached Data
This example shows how to build a simple cache system using SplFixedArray. Due to the fixed size of SplFixedArray, it is ideal for caching such fixed-size data structures.
Common Errors and Debugging Tips
The most common error when using SplFixedArray is trying to access or set an index that exceeds the size of the array:
$fixedArray = new SplFixedArray(3); $fixedArray[3] = 'Error'; // RuntimeException will be thrown
To avoid this error, you can check whether the index is within the valid range before the operation:
if ($index >= 0 && $index < $fixedArray->getSize()) { $fixedArray[$index] = 'Value'; }
Performance optimization and best practices
SplFixedArray can significantly improve performance in some scenarios, but it does not work in all cases. Let's compare the performance of SplFixedArray and normal PHP arrays:
- Memory usage : SplFixedArray usually takes up less memory than normal arrays because it has no hash table structure.
- Access speed : SplFixedArray's element access speed is usually faster because it operates directly on memory.
- Initialization time : SplFixedArray needs to specify the size when initializing, which may increase the initialization time in some cases.
Here is a simple benchmark that compares the performance of SplFixedArray with normal arrays:
$size = 1000000; // Use SpliftedArray $start = microtime(true); $fixedArray = new SplFixedArray($size); for ($i = 0; $i < $size; $i ) { $fixedArray[$i] = $i; } $end = microtime(true); echo "SplFixedArray Initialization and Filling Time: " . ($end - $start) . " seconds\n"; // Use the normal array $start = microtime(true); $normalArray = []; for ($i = 0; $i < $size; $i ) { $normalArray[] = $i; } $end = microtime(true); echo "Normal array initialization and fill time: " . ($end - $start) . " seconds\n";
In practical applications, best practices for using SplFixedArray include:
- Determine the size : Before creating a SplFixedArray, try to estimate the required size as accurately as possible to avoid wasting memory.
- Batch Operation : If you need to perform a large amount of operations on SplFixedArray, try to batch processing to reduce performance overhead.
- Code readability : Although SplFixedArray can improve performance, you should also pay attention to maintaining the readability and maintenance of the code.
Overall, SplFixedArray is a powerful tool, especially in memory-sensitive scenarios where large amounts of data are required to process. However, its use requires caution, as its fixed size may cause inconvenience in some cases. When choosing to use SplFixedArray, you need to weigh its performance advantages and usage limitations.
The above is the detailed content of Explain the SPL SplFixedArray and its performance characteristics compared to regular PHP arrays.. For more information, please follow other related articles on the PHP Chinese website!

ThesecrettokeepingaPHP-poweredwebsiterunningsmoothlyunderheavyloadinvolvesseveralkeystrategies:1)ImplementopcodecachingwithOPcachetoreducescriptexecutiontime,2)UsedatabasequerycachingwithRedistolessendatabaseload,3)LeverageCDNslikeCloudflareforservin
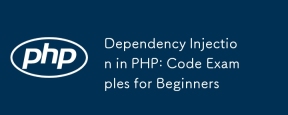
You should care about DependencyInjection(DI) because it makes your code clearer and easier to maintain. 1) DI makes it more modular by decoupling classes, 2) improves the convenience of testing and code flexibility, 3) Use DI containers to manage complex dependencies, but pay attention to performance impact and circular dependencies, 4) The best practice is to rely on abstract interfaces to achieve loose coupling.
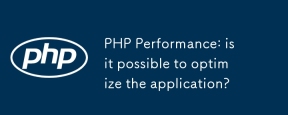
Yes,optimizingaPHPapplicationispossibleandessential.1)ImplementcachingusingAPCutoreducedatabaseload.2)Optimizedatabaseswithindexing,efficientqueries,andconnectionpooling.3)Enhancecodewithbuilt-infunctions,avoidingglobalvariables,andusingopcodecaching
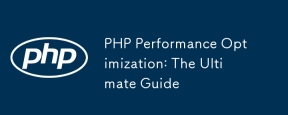
ThekeystrategiestosignificantlyboostPHPapplicationperformanceare:1)UseopcodecachinglikeOPcachetoreduceexecutiontime,2)Optimizedatabaseinteractionswithpreparedstatementsandproperindexing,3)ConfigurewebserverslikeNginxwithPHP-FPMforbetterperformance,4)
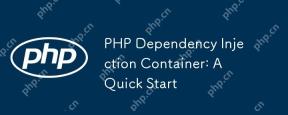
APHPDependencyInjectionContainerisatoolthatmanagesclassdependencies,enhancingcodemodularity,testability,andmaintainability.Itactsasacentralhubforcreatingandinjectingdependencies,thusreducingtightcouplingandeasingunittesting.
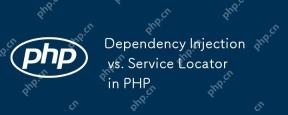
Select DependencyInjection (DI) for large applications, ServiceLocator is suitable for small projects or prototypes. 1) DI improves the testability and modularity of the code through constructor injection. 2) ServiceLocator obtains services through center registration, which is convenient but may lead to an increase in code coupling.
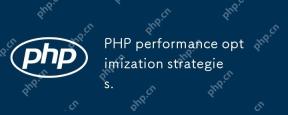
PHPapplicationscanbeoptimizedforspeedandefficiencyby:1)enablingopcacheinphp.ini,2)usingpreparedstatementswithPDOfordatabasequeries,3)replacingloopswitharray_filterandarray_mapfordataprocessing,4)configuringNginxasareverseproxy,5)implementingcachingwi
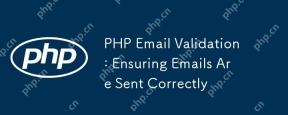
PHPemailvalidationinvolvesthreesteps:1)Formatvalidationusingregularexpressionstochecktheemailformat;2)DNSvalidationtoensurethedomainhasavalidMXrecord;3)SMTPvalidation,themostthoroughmethod,whichchecksifthemailboxexistsbyconnectingtotheSMTPserver.Impl


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

Zend Studio 13.0.1
Powerful PHP integrated development environment

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
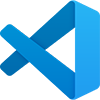
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
