Vue and Element-UI cascade drop-down box lazy loading
The Element-UI cascading drop-down box does not support lazy loading and needs to be implemented manually. The core of lazy loading is to only load data at the current visible level, and then load child node data asynchronously when nodes are expanded. The key to implementation lies in the load method, which triggers asynchronous loading when the node is clicked through the @node-click event. Pay attention to performance optimization points such as data format optimization, caching mechanism, error handling and unlimited loading strategies.
Vue and Element-UI cascaded drop-down box lazy loading: performance optimization tool
Many friends will encounter the problem of huge data volume of cascading selection boxes causing page stuttering when developing with Vue and Element-UI. This article will talk about how to solve this problem gracefully - lazy loading. After reading it, you will understand the principle of lazy loading, learn how to implement it efficiently, and avoid some common pitfalls.
Let's clarify first: The cascading selection box of Element-UI does not directly support lazy loading. This means we need to do it ourselves and have enough food and clothing. This cannot be done in just a few lines of code. It requires a deeper understanding of Vue's responsive mechanism and asynchronous operations.
Let's start with the basics. The cascading selection box is essentially a tree-shaped data display and selection component. If the data volume is large, directly loading all data will cause excessive rendering burden on the browser, resulting in slow loading of the page or even crashing. The core idea of lazy loading is: only load data at the current visible level, and the user needs to expand the next level before loading the corresponding data.
The key to implementing lazy loading lies in the load
method. The cascading selection box of Element-UI provides a @node-click
event, which is triggered when the node is clicked. We can use this event to load child node data asynchronously when nodes are expanded.
Let’s take a look at an example, suppose our data structure is like this:
<code class="javascript">const data = [ { value: '1', label: '一级菜单1', children: [] // 懒加载,初始为空}, { value: '2', label: '一级菜单2', children: [] // 懒加载,初始为空} ];</code>
Then, in our Vue component:
<code class="vue"><template> <el-cascader v-model="value" :options="data" :props="props"></el-cascader> </template> <script> import { ref, reactive } from 'vue'; export default { setup() { const value = ref([]); const data = reactive([ { value: '1', label: '一级菜单1', children: [] }, { value: '2', label: '一级菜单2', children: [] } ]); const props = reactive({ label: 'label', value: 'value', children: 'children' }); const handleNodeClick = async (node, data) => { if (!node.children && node.children !== undefined) { //只加载未加载的节点node.loading = true; // 显示加载状态const res = await fetch(`/api/data?parentId=${node.value}`); // 异步获取子节点数据const children = await res.json(); node.children = children; node.loading = false; // 隐藏加载状态} }; return { value, data, props, handleNodeClick }; } }; </script></code>
In this code, the handleNodeClick
method will be triggered when the node is clicked. If the node does not have child nodes ( !node.children
), it requests data from the backend and assigns the data to node.children
. node.loading
is used to display the loading status and improve the user experience. Remember, fetch
is an asynchronous operation, be sure to use await
to wait for the result.
It should be noted that the backend interface /api/data?parentId=${node.value}
needs to return the corresponding child node data according to the parent node's value
. This part needs to be adjusted according to your actual backend interface.
Regarding performance optimization, there are several other points that need special attention:
- Data format optimization: The data format returned by the backend should be as concise as possible to avoid unnecessary fields.
- Caching mechanism: You can consider using the caching mechanism to reduce the number of requests to the backend. For example, you can use
localStorage
orsessionStorage
to cache the loaded data. - Error handling: To deal with network request failures to avoid program crashes. For example, you can add
try...catch
statement to catch exceptions. - Unlimited loading: If your data hierarchy is deep, you may need to consider unlimited loading strategies to avoid loading too much data.
In short, the lazy loading implementation of Vue and Element-UI cascaded drop-down boxes requires careful consideration of data structure, asynchronous operations, error handling, and caching mechanisms. This article provides a basic implementation solution that I hope can help you solve practical problems. Remember, practice to achieve true knowledge and try more hands-only to truly master this technology. Don't forget to adjust the code according to your actual situation and conduct sufficient testing.
The above is the detailed content of Vue and Element-UI cascade drop-down box lazy loading. For more information, please follow other related articles on the PHP Chinese website!

Vue.js is a progressive framework suitable for building highly interactive user interfaces. Its core functions include responsive systems, component development and routing management. 1) The responsive system realizes data monitoring through Object.defineProperty or Proxy, and automatically updates the interface. 2) Component development allows the interface to be split into reusable modules. 3) VueRouter supports single-page applications to improve user experience.

The main disadvantages of Vue.js include: 1. The ecosystem is relatively new, and third-party libraries and tools are not as rich as other frameworks; 2. The learning curve becomes steep in complex functions; 3. Community support and resources are not as extensive as React and Angular; 4. Performance problems may be encountered in large applications; 5. Version upgrades and compatibility challenges are greater.

Netflix uses React as its front-end framework. 1.React's component development and virtual DOM mechanism improve performance and development efficiency. 2. Use Webpack and Babel to optimize code construction and deployment. 3. Use code segmentation, server-side rendering and caching strategies for performance optimization.

Reasons for Vue.js' popularity include simplicity and easy learning, flexibility and high performance. 1) Its progressive framework design is suitable for beginners to learn step by step. 2) Component-based development improves code maintainability and team collaboration efficiency. 3) Responsive systems and virtual DOM improve rendering performance.

Vue.js is easier to use and has a smooth learning curve, which is suitable for beginners; React has a steeper learning curve, but has strong flexibility, which is suitable for experienced developers. 1.Vue.js is easy to get started with through simple data binding and progressive design. 2.React requires understanding of virtual DOM and JSX, but provides higher flexibility and performance advantages.

Vue.js is suitable for fast development and small projects, while React is more suitable for large and complex projects. 1.Vue.js is simple and easy to learn, suitable for rapid development and small projects. 2.React is powerful and suitable for large and complex projects. 3. The progressive features of Vue.js are suitable for gradually introducing functions. 4. React's componentized and virtual DOM performs well when dealing with complex UI and data-intensive applications.

Vue.js and React each have their own advantages and disadvantages. When choosing, you need to comprehensively consider team skills, project size and performance requirements. 1) Vue.js is suitable for fast development and small projects, with a low learning curve, but deep nested objects can cause performance problems. 2) React is suitable for large and complex applications, with a rich ecosystem, but frequent updates may lead to performance bottlenecks.

Vue.js is suitable for small to medium-sized projects, while React is suitable for large projects and complex application scenarios. 1) Vue.js is easy to use and is suitable for rapid prototyping and small applications. 2) React has more advantages in handling complex state management and performance optimization, and is suitable for large projects.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
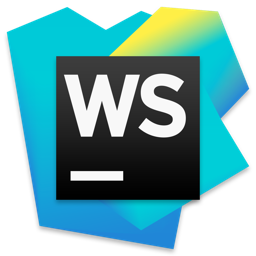
WebStorm Mac version
Useful JavaScript development tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
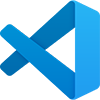
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
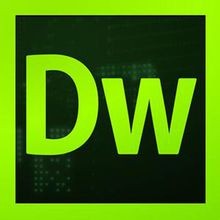
Dreamweaver CS6
Visual web development tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
