MVCC implements non-blocking read operations in InnoDB by saving multiple versions of data to improve concurrency performance. 1) The working principle of MVCC depends on the undo log and read view mechanisms. 2) The basic usage does not require special configuration, InnoDB is enabled by default. 3) Advanced usage can realize the "snapshot reading" function. 4) Common errors such as undo log bloat can be avoided by setting the transaction timeout. 5) Performance optimization includes shortening transaction time, reasonable use of indexes and batch processing of data updates.
introduction
MVCC, full name Multi-Version Concurrency Control, is a key concurrency control mechanism in databases, especially in the InnoDB storage engine, which makes our database operations more efficient and secure. Today, we will explore the implementation and application of MVCC in InnoDB in depth. Through this article, you will understand how MVCC works, how to improve the concurrency performance of a database, and how to use MVCC to avoid common concurrency problems in actual development.
Review of basic knowledge
Before discussing MVCC, let's review the basics of database concurrency control. Database concurrency control is designed to ensure that data integrity and consistency are not compromised when multiple transactions are executed simultaneously. Traditional locking mechanisms, such as row-level locks and table-level locks, can ensure data consistency, but may lead to performance bottlenecks. MVCC provides a more flexible and efficient concurrency control method by introducing the concept of multiple versions.
As a storage engine of MySQL, InnoDB is known for its high performance and reliability. Its support for MVCC allows it to handle high concurrency scenarios with ease.
Core concept or function analysis
Definition and function of MVCC
MVCC is a concurrency control technology that implements non-blocking read operations by saving multiple versions of data. Simply put, when a transaction starts, it will see a consistent view of the database, which means that the transaction will not be disturbed by other transactions during execution, thereby improving the performance of read operations.
For example, in InnoDB, when you execute a SELECT query, MVCC ensures that you see the status of the database at the beginning of the transaction, and that your query results will not be affected even if other transactions are modifying this data.
-- Transaction 1 starts START TRANSACTION; SELECT * FROM users WHERE id = 1; -- Transaction 2 Modify data when transaction 1 executes SELECT UPDATE users SET name = 'Alice' WHERE id = 1; COMMIT; -- Transaction 1 still sees the data at the beginning of the transaction SELECT * FROM users WHERE id = 1; COMMIT;
How it works
The working principle of MVCC depends on InnoDB's undo log and read view mechanisms. Each transaction generates a unique read view at the beginning, which determines which versions of data the transaction can see. undo log saves multiple historical versions of the data.
When a transaction performs a read operation, InnoDB will decide which version of data to return based on the transaction's read view. If the transaction needs to update the data, InnoDB creates a new version of the data and saves the old version in the undo log so that other transactions can still see the old version of the data.
This mechanism not only improves the performance of read operations, but also reduces the use of locks, thereby improving the overall concurrency performance of the database.
Example of usage
Basic usage
MVCC does not require special configuration in daily use, and InnoDB enables MVCC by default. You can simply experience the effects of MVCC through transactions.
-- Transaction 1 START TRANSACTION; SELECT * FROM orders WHERE order_id = 100; -- Transaction 2 Insert new orders when transaction 1 executes SELECT INSERT INTO orders (order_id, customer_id, amount) VALUES (101, 1, 100); COMMIT; -- Transaction 1 still does not see the newly inserted order SELECT * FROM orders WHERE order_id = 101; COMMIT;
Advanced Usage
In some cases, you may need to leverage MVCC to implement some complex business logic. For example, implement a "snapshot reading" function, allowing users to view the data status at a certain point in time.
-- Get a snapshot of a data point in time SET TIMESTAMP = UNIX_TIMESTAMP('2023-01-01 00:00:00'); START TRANSACTION; SELECT * FROM inventory; COMMIT;
Common Errors and Debugging Tips
Although MVCC is powerful, it may also encounter some problems during use. For example, if a transaction is not committed for a long time, it may cause undo log bloat, affecting database performance. To avoid this, the transaction timeout time can be set.
-- Set transaction timeout SET innodb_lock_wait_timeout = 50;
In addition, if you find that some query results do not meet expectations, it may be due to improper isolation level settings of MVCC. This problem can be solved by adjusting the isolation level.
-- Set the transaction isolation level to READ COMMITTED SET TRANSACTION ISOLATION LEVEL READ COMMITTED;
Performance optimization and best practices
Performance optimization is an important aspect when using MVCC. First, by reducing the duration of the transaction, the use of undo log can be reduced, thereby improving the overall performance of the database.
-- Shorten the transaction time as much as possible START TRANSACTION; UPDATE products SET price = price * 1.1 WHERE category = 'Electronics'; COMMIT;
Secondly, rational use of indexes can speed up MVCC reading operations. Make sure your query conditions make full use of the index, thereby reducing dependency on undo logs.
-- Create an index to optimize query CREATE INDEX idx_category ON products(category);
Finally, batch processing of large-scale data updates can avoid long-term transactions, thus reducing the pressure on MVCC.
-- Batch data update START TRANSACTION; UPDATE products SET price = price * 1.1 WHERE category = 'Electronics' LIMIT 1000; COMMIT; -- Repeat the above until all data is processed
In actual development, the use of MVCC also needs to be combined with specific business scenarios. For example, in a high concurrency environment, rational design of table structures and query statements can maximize the advantages of MVCC. In scenarios where data consistency requirements are extremely high, it may be necessary to combine other locking mechanisms to ensure data integrity.
In short, the application of MVCC in InnoDB provides us with strong concurrency control capabilities. By understanding and correct use of MVCC, we can significantly improve the performance and reliability of the database.
The above is the detailed content of What is Multi-Version Concurrency Control (MVCC) in InnoDB?. For more information, please follow other related articles on the PHP Chinese website!
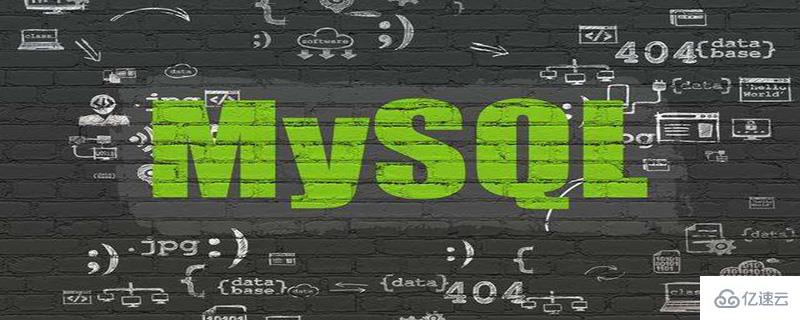
InnoDB是一个将表中的数据存储到磁盘上的存储引擎,所以即使关机后重启我们的数据还是存在的。而真正处理数据的过程是发生在内存中的,所以需要把磁盘中的数据加载到内存中,如果是处理写入或修改请求的话,还需要把内存中的内容刷新到磁盘上。而我们知道读写磁盘的速度非常慢,和内存读写差了几个数量级,所以当我们想从表中获取某些记录时,InnoDB存储引擎需要一条一条的把记录从磁盘上读出来么?InnoDB采取的方式是:将数据划分为若干个页,以页作为磁盘和内存之间交互的基本单位,InnoDB中页的大小一般为16
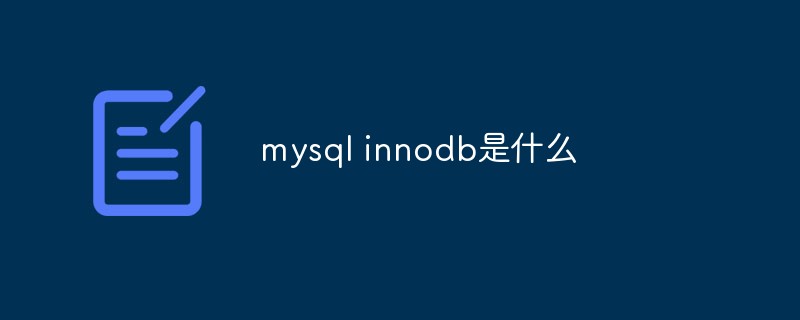
InnoDB是MySQL的数据库引擎之一,现为MySQL的默认存储引擎,为MySQL AB发布binary的标准之一;InnoDB采用双轨制授权,一个是GPL授权,另一个是专有软件授权。InnoDB是事务型数据库的首选引擎,支持事务安全表(ACID);InnoDB支持行级锁,行级锁可以最大程度的支持并发,行级锁是由存储引擎层实现的。
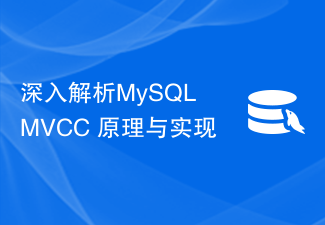
深入解析MySQLMVCC原理与实现MySQL是目前最流行的关系型数据库管理系统之一,它提供了多版本并发控制(MultiversionConcurrencyControl,MVCC)机制来支持高效并发处理。MVCC是一种在数据库中处理并发事务的方法,可以提供高并发和隔离性。本文将深入解析MySQLMVCC的原理与实现,并结合代码示例进行说明。一、M
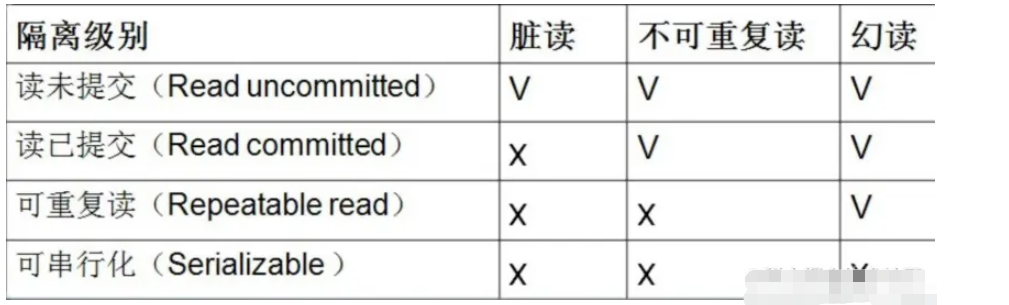
1.Mysql的事务隔离级别这四种隔离级别,当存在多个事务并发冲突的时候,可能会出现脏读,不可重复读,幻读的一些问题,而innoDB在可重复读隔离级别模式下解决了幻读的一个问题,2.什么是幻读幻读是指在同一个事务中,前后两次查询相同范围的时候得到的结果不一致如图,第一个事务里面,我们执行一个范围查询,这个时候满足条件的数据只有一条,而在第二个事务里面,它插入一行数据并且进行了提交,接着第一个事务再去查询的时候,得到的结果比第一次查询的结果多出来一条数据,注意第一个事务的第一次和第二次查询,都在同
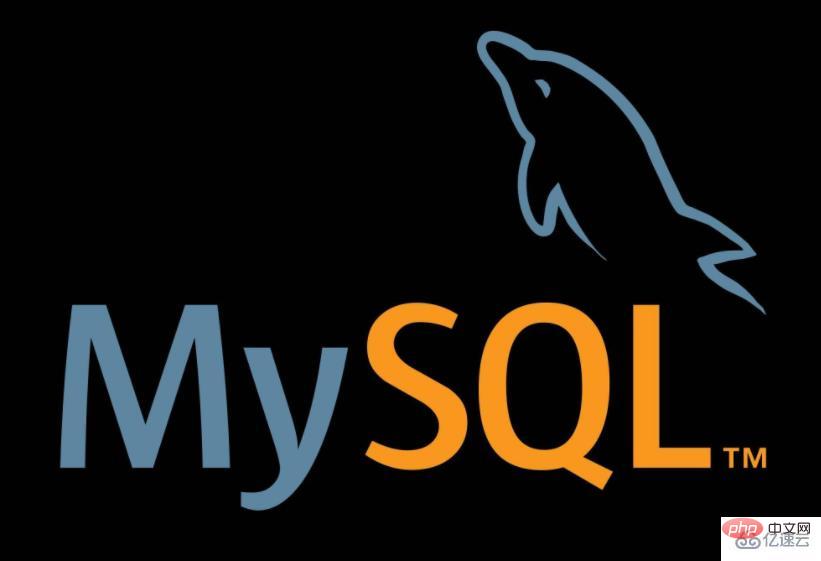
一、回退重新装mysql为避免再从其他地方导入这个数据的麻烦,先对当前库的数据库文件做了个备份(/var/lib/mysql/位置)。接下来将Perconaserver5.7包进行了卸载,重新安装原先老的5.1.71的包,启动mysql服务,提示Unknown/unsupportedtabletype:innodb,无法正常启动。11050912:04:27InnoDB:Initializingbufferpool,size=384.0M11050912:04:27InnoDB:Complete
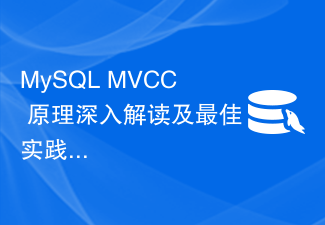
MySQLMVCC原理深入解读及最佳实践一、概述MySQL是使用最广泛的关系型数据库管理系统之一,其支持多版本并发控制(Multi-VersionConcurrencyControl,MVCC)机制来处理并发访问问题。本文将深入解读MySQLMVCC的原理,并给出一些最佳实践的例子。二、MVCC原理版本号MVCC是通过为每个数据行添加额外
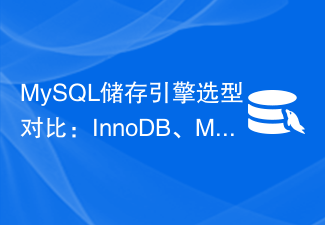
MySQL储存引擎选型对比:InnoDB、MyISAM与Memory性能指标评估引言:在MySQL数据库中,储存引擎的选择对于系统性能和数据完整性起着至关重要的作用。MySQL提供了多种储存引擎,其中最常用的引擎包括InnoDB、MyISAM和Memory。本文将就这三种储存引擎进行性能指标评估,并通过代码示例进行比较。一、InnoDB引擎InnoDB是My

MySQL是一款广泛使用的数据库管理系统,不同的存储引擎对数据库性能有不同的影响。MyISAM和InnoDB是MySQL中最常用的两种存储引擎,它们的特点各有不同,使用不当可能会影响数据库的性能。本文将介绍如何使用这两种存储引擎来优化MySQL性能。一、MyISAM存储引擎MyISAM是MySQL最常用的存储引擎,它的优点是速度快,存储占用空间小。MyISA


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

Notepad++7.3.1
Easy-to-use and free code editor

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
