What are the execution orders of c language functions?
The execution order of C functions is not as simple as from top to bottom, but is affected by a variety of factors, including function calling methods, recursion, pointers and asynchronous operations. The function call stack determines the order of function execution, while the calling method, recursion, pointer functions and function pointers, and asynchronous operations complicate this process, bringing flexibility but also increasing difficulty in predictability.
C language function execution order exploration: It is not just as simple as top-down
Have you ever been confused by the execution order of C functions? Think it's just a simple way from top to bottom? That's a big mistake! The execution order of C functions is much more complicated than you think. It is affected by various factors such as calling methods, recursion, pointers, and asynchronous operations. This article will take you into the underlying mechanism of C function execution order and unveil its mystery. After reading it, you will have a deeper understanding of the execution order of C functions and can easily deal with various complex calling scenarios.
Basic knowledge review: Function call stack
To understand the execution order of C functions, you must first understand the function call stack. When a function is called, the system will allocate a piece of stack memory to store information such as local variables, function parameters, and return addresses. After the function is executed, this piece of memory will be released, and the program execution process returns to the next line of the statement calling the function. When multiple functions are called in nested, the stack frame (Stack Frame) will be pushed into the stack layer by layer to form a stack structure. This is the essence of the function call stack. Understanding this is crucial because the execution order of functions is directly related to the order of incoming and outgoing stack frames.
Core concept: Determinants of function call order
The execution order of functions is not determined simply by the writing order of the code. It mainly depends on the following key factors:
- How to call functions: This is the most direct factor.
main
function is the entry point of the program, and its execution order determines the order of call of other functions. When one function calls another function, the called function is executed first, and the control right is returned to the calling function after execution. This is like a baton guiding the execution of the program. - Recursive call: The recursive function calls itself, forming a loop call. The order of execution depends on the recursive terminating conditions and the way the recursive call is called. The key to understanding recursion is to imagine a stack, each recursive call pushes a new stack frame until the termination condition is met, and then returns layer by layer. It's like a Russian doll, opening up layer by layer.
- Pointer functions and function pointers: Pointer functions and function pointers increase the flexibility of function calls. Through pointers, you can call different functions dynamically, which makes the execution order of functions more flexible and difficult to predict. You need to carefully analyze the function pointed to by the pointer to accurately judge the execution order. This is like a remote control that can control different devices (functions).
- Asynchronous Operation: In multithreaded or multi-process programming, the execution order of functions may become parallel or concurrent. At this time, the execution order of functions is no longer a simple linear order, but is determined by operating system scheduling. It's like a symphony orchestra where multiple instruments are played at the same time, but ultimately presenting a harmonious music.
Code Example: Exploring Recursion
Let's look at a simple recursive function example to understand the execution order in recursive calls more intuitively:
<code class="c">#include <stdio.h> void recursive_function(int n) { if (n > 0) { printf("Entering recursive_function, n = %d\n", n); recursive_function(n - 1); // 递归调用printf("Leaving recursive_function, n = %d\n", n); } } int main() { recursive_function(3); return 0; }</stdio.h></code>
This code will output:
<code>Entering recursive_function, n = 3 Entering recursive_function, n = 2 Entering recursive_function, n = 1 Leaving recursive_function, n = 1 Leaving recursive_function, n = 2 Leaving recursive_function, n = 3</code>
Pay attention to the output order, which clearly shows the in and out process of the recursive call stack.
Advanced usage: the wonderful use of pointer functions
Pointer functions can implement more flexible function calls. For example, you can implement a function scheduler using a function pointer array:
<code class="c">#include <stdio.h> void func1() { printf("func1 called\n"); } void func2() { printf("func2 called\n"); } void func3() { printf("func3 called\n"); } int main() { void (*func_ptr_array[])(void) = {func1, func2, func3}; int i; for (i = 0; i </stdio.h></code>
This code demonstrates how to dynamically call different functions through an array of function pointers to change the execution order of functions.
FAQs and debugging tips
The most effective tool for debugging the execution order of C functions is a debugger (such as GDB). Setting breakpoints, stepping through code, observing variable values and stack frame information can help you clearly understand the execution process of the function. Carefully checking the recursive termination conditions and pointer pointers is the key to avoiding errors. Remember, attentiveness and patience are the key to debugging.
Performance optimization and best practices
For recursive functions, you need to be careful to avoid stack overflow. If the recursion is too deep, it may cause a stack overflow error. You can consider using iterative methods instead of recursion, or using tail recursion optimization techniques. For pointer functions, make sure that the memory pointed to by the pointer is valid and avoid wild pointer errors. Clear code style and comments can greatly improve the readability and maintainability of the code and reduce debugging difficulty.
In short, the execution order of C language functions is not static. Only by understanding the function call stack, recursion, pointer, asynchronous operation and other factors can we truly master the execution mechanism of C language functions and write efficient and reliable C language programs. Remember, programming is an art, and understanding the underlying mechanism is the key to creating excellent works.
The above is the detailed content of What are the execution orders of c language functions?. For more information, please follow other related articles on the PHP Chinese website!
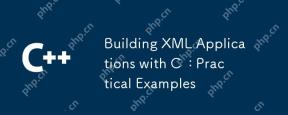
You can use the TinyXML, Pugixml, or libxml2 libraries to process XML data in C. 1) Parse XML files: Use DOM or SAX methods, DOM is suitable for small files, and SAX is suitable for large files. 2) Generate XML file: convert the data structure into XML format and write to the file. Through these steps, XML data can be effectively managed and manipulated.
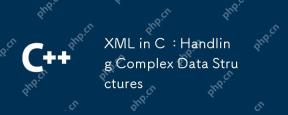
Working with XML data structures in C can use the TinyXML or pugixml library. 1) Use the pugixml library to parse and generate XML files. 2) Handle complex nested XML elements, such as book information. 3) Optimize XML processing code, and it is recommended to use efficient libraries and streaming parsing. Through these steps, XML data can be processed efficiently.
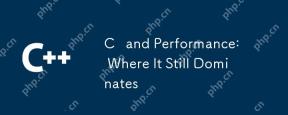
C still dominates performance optimization because its low-level memory management and efficient execution capabilities make it indispensable in game development, financial transaction systems and embedded systems. Specifically, it is manifested as: 1) In game development, C's low-level memory management and efficient execution capabilities make it the preferred language for game engine development; 2) In financial transaction systems, C's performance advantages ensure extremely low latency and high throughput; 3) In embedded systems, C's low-level memory management and efficient execution capabilities make it very popular in resource-constrained environments.
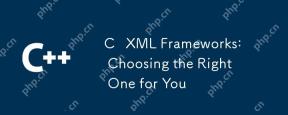
The choice of C XML framework should be based on project requirements. 1) TinyXML is suitable for resource-constrained environments, 2) pugixml is suitable for high-performance requirements, 3) Xerces-C supports complex XMLSchema verification, and performance, ease of use and licenses must be considered when choosing.
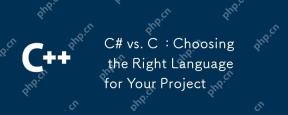
C# is suitable for projects that require development efficiency and type safety, while C is suitable for projects that require high performance and hardware control. 1) C# provides garbage collection and LINQ, suitable for enterprise applications and Windows development. 2)C is known for its high performance and underlying control, and is widely used in gaming and system programming.
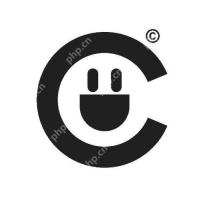
C code optimization can be achieved through the following strategies: 1. Manually manage memory for optimization use; 2. Write code that complies with compiler optimization rules; 3. Select appropriate algorithms and data structures; 4. Use inline functions to reduce call overhead; 5. Apply template metaprogramming to optimize at compile time; 6. Avoid unnecessary copying, use moving semantics and reference parameters; 7. Use const correctly to help compiler optimization; 8. Select appropriate data structures, such as std::vector.
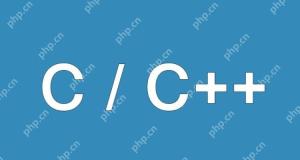
The volatile keyword in C is used to inform the compiler that the value of the variable may be changed outside of code control and therefore cannot be optimized. 1) It is often used to read variables that may be modified by hardware or interrupt service programs, such as sensor state. 2) Volatile cannot guarantee multi-thread safety, and should use mutex locks or atomic operations. 3) Using volatile may cause performance slight to decrease, but ensure program correctness.
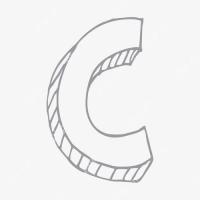
Measuring thread performance in C can use the timing tools, performance analysis tools, and custom timers in the standard library. 1. Use the library to measure execution time. 2. Use gprof for performance analysis. The steps include adding the -pg option during compilation, running the program to generate a gmon.out file, and generating a performance report. 3. Use Valgrind's Callgrind module to perform more detailed analysis. The steps include running the program to generate the callgrind.out file and viewing the results using kcachegrind. 4. Custom timers can flexibly measure the execution time of a specific code segment. These methods help to fully understand thread performance and optimize code.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
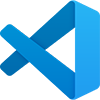
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
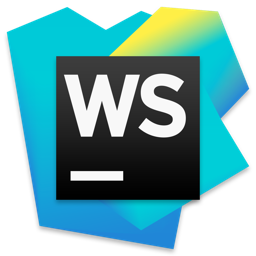
WebStorm Mac version
Useful JavaScript development tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SublimeText3 Linux new version
SublimeText3 Linux latest version
