NULL is essentially a null pointer to an empty address and does not mean invalid. Relying solely on ptr == NULL to determine that the pointer is valid is not enough to capture uninitialized, released or out of boundary memory. More reliable validity checking strategies include: checking the return value after allocating memory, setting the pointer to NULL after freeing memory, checking NULL for function parameters, using assertions and developing good programming habits (initializing pointers, checking validity, setting it to NULL after freeing, be careful of pointer operation).
NULL pointer in C language: truth and trap
Many newbies (even some veterans) think that using ptr == NULL
can handle all pointer validity checks. This idea is too naive! This article will explore in-depth the essence of NULL
pointers and why relying solely on ptr == NULL
to judge the validity of pointers often fall into the pit. After reading it, you will have a deeper understanding of the underlying mechanism of C language pointers and write more robust and less error-prone code.
The nature of NULL
NULL
is not a magical magic value, it is essentially a macro, usually defined as (void *)0
. This means that NULL
is a null pointer to an empty address. The key is that "empty address" itself does not necessarily mean "invalid". The operating system may reserve certain address areas, for example, for kernel or system calls. Trying to access these addresses will have unimaginable consequences - program crashes, system instability, and even security vulnerabilities.
Why is ptr == NULL
not enough
The problem is that ptr == NULL
only determines whether the pointer points to an empty address. It cannot distinguish whether the pointer has been initialized, whether it points to freed memory, or points to out-of-bounds memory. These situations will also cause program errors, but ptr == NULL
cannot be detected.
For example:
<code class="c">#include <stdio.h> #include <stdlib.h> int main() { int *ptr; // 未初始化的指针if (ptr == NULL) { printf("ptr is NULL\n"); // 这条语句会执行,但ptr并非安全可用的} // 尝试访问未初始化的指针*ptr = 10; // 这行代码很危险!可能导致程序崩溃int *ptr2 = (int *)malloc(sizeof(int)); free(ptr2); // 释放内存*ptr2 = 20; // 使用已释放的内存,程序行为未定义,可能崩溃或出现奇怪的结果return 0; }</stdlib.h></stdio.h></code>
In this code, although ptr
is NULL
, it is not initialized, and using it directly will lead to undefined behavior. Although ptr2
is effective at first, it is also dangerous to use it again after release. ptr == NULL
cannot catch these errors.
More reliable pointer validity check
So, how to check the validity of pointers more reliably? The answer is: adopt different strategies according to the specific situation .
- For dynamically allocated memory: After
malloc
,calloc
, orrealloc
, the return value must be checked whether it isNULL
. IfNULL
is returned, it means that memory allocation failed. After freeing memory, set the pointer toNULL
immediately to prevent dangling pointer errors. - For function parameters: If the function receives pointer parameters, it should check whether the pointer is
NULL
inside the function body to avoid program crashes due to null pointer dereference. - For global or static variables: Global or static variables are usually automatically initialized to
NULL
(or a default value) when the program starts, so there is no need to explicitly checkNULL
. However, before use, be sure to confirm whether the memory it points to is valid. - Consider using assertions: During the debug phase, you can use
assert
macro to check the validity of the pointer.assert(ptr != NULL);
If the assertion fails, the program will terminate and print the error message.
Talk about experience
When writing C code, pointers are double-edged swords. Proficient in pointers, you can write efficient and elegant code; but if you are not careful, you will fall into the pointer trap. It is crucial to develop good programming habits:
- Always initialize the pointer.
- Before using the pointer, carefully check its validity.
- Once the memory is freed, set the pointer to
NULL
immediately. - Make full use of debugging tools to promptly discover and resolve pointer-related problems.
- Use pointer arithmetic with caution to avoid out-of-bounds access.
In short, relying solely on ptr == NULL
to judge the validity of a pointer is far from enough. It is necessary to combine multiple methods according to the specific situation to effectively avoid pointer-related errors and write safer and more reliable C code. Remember, be careful to sail the ship for thousands of years!
The above is the detailed content of How to determine whether the pointer is valid in C language. For more information, please follow other related articles on the PHP Chinese website!
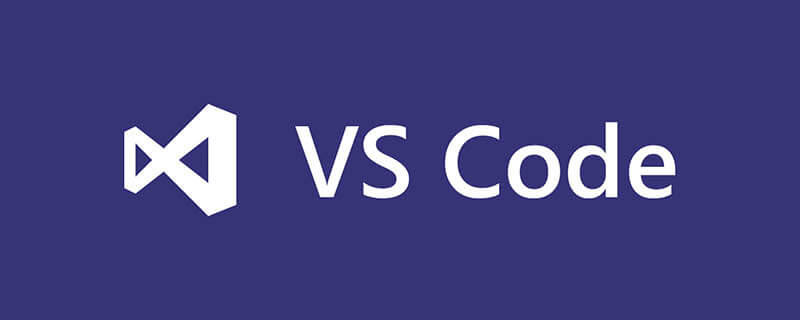
VScode中怎么配置C语言环境?下面本篇文章给大家介绍一下VScode配置C语言环境的方法(超详细),希望对大家有所帮助!
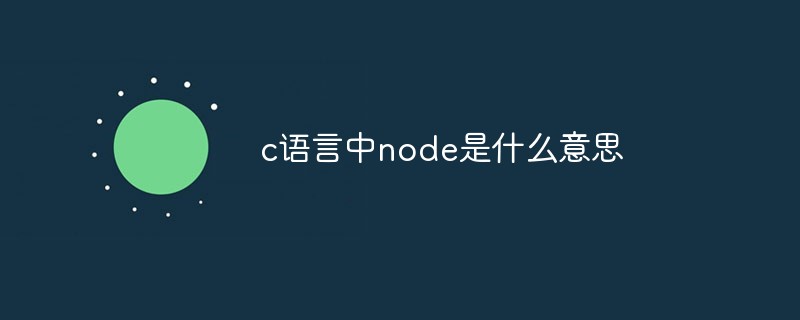
在C语言中,node是用于定义链表结点的名称,通常在数据结构中用作结点的类型名,语法为“struct Node{...};”;结构和类在定义出名称以后,直接用该名称就可以定义对象,C语言中还存在“Node * a”和“Node* &a”。

c语言将数字转换成字符串的方法:1、ascii码操作,在原数字的基础上加“0x30”,语法“数字+0x30”,会存储数字对应的字符ascii码;2、使用itoa(),可以把整型数转换成字符串,语法“itoa(number1,string,数字);”;3、使用sprintf(),可以能够根据指定的需求,格式化内容,存储至指针指向的字符串。
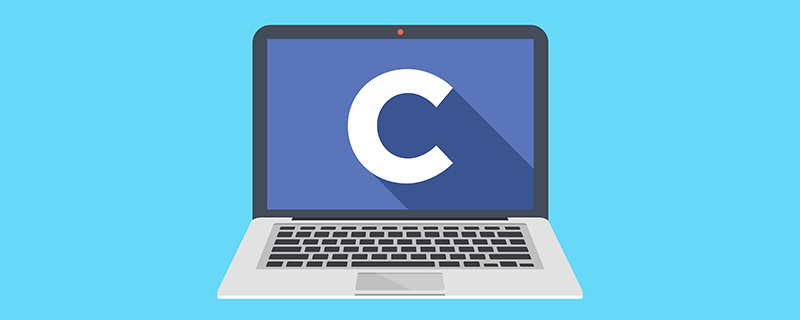
在c语言中,没有开根号运算符,开根号使用的是内置函数“sqrt()”,使用语法“sqrt(数值x)”;例如“sqrt(4)”,就是对4进行平方根运算,结果为2。sqrt()是c语言内置的开根号运算函数,其运算结果是函数变量的算术平方根;该函数既不能运算负数值,也不能输出虚数结果。

C语言数组初始化的三种方式:1、在定义时直接赋值,语法“数据类型 arrayName[index] = {值};”;2、利用for循环初始化,语法“for (int i=0;i<3;i++) {arr[i] = i;}”;3、使用memset()函数初始化,语法“memset(arr, 0, sizeof(int) * 3)”。
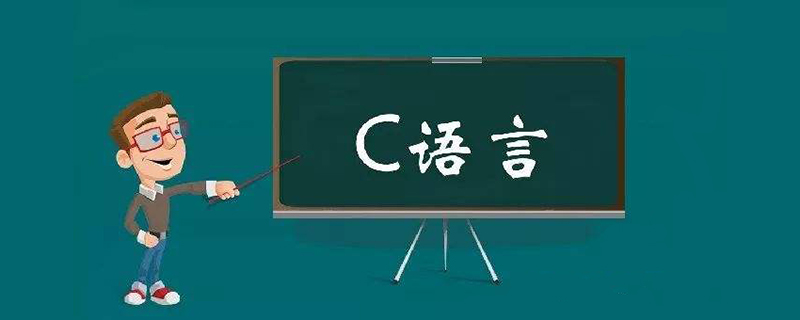
c语言合法标识符的要求是:1、标识符只能由字母(A~Z, a~z)、数字(0~9)和下划线(_)组成;2、第一个字符必须是字母或下划线,不能是数字;3、标识符中的大小写字母是有区别的,代表不同含义;4、标识符不能是关键字。
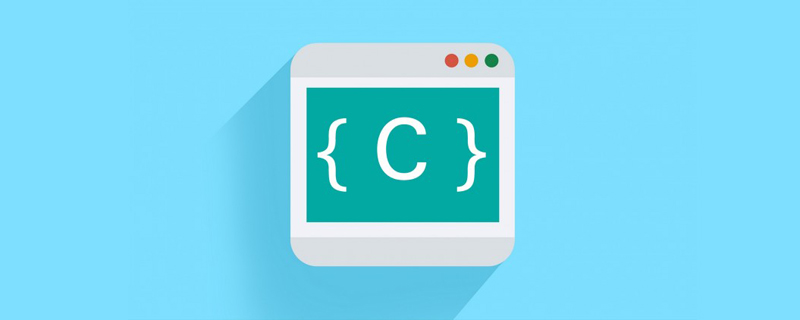
c语言编译后生成“.OBJ”的二进制文件(目标文件)。在C语言中,源程序(.c文件)经过编译程序编译之后,会生成一个后缀为“.OBJ”的二进制文件(称为目标文件);最后还要由称为“连接程序”(Link)的软件,把此“.OBJ”文件与c语言提供的各种库函数连接在一起,生成一个后缀“.EXE”的可执行文件。
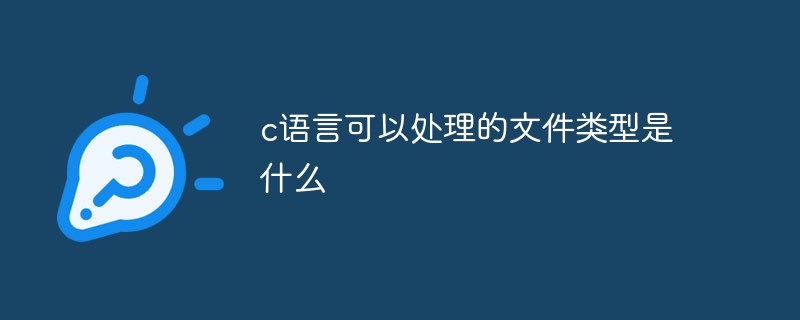
c语言可以处理的文件类型是:文本文件和二进制文件。C语言所能够处理文件是按照存放形式分为文本文件和二进制文件:1、文本文件存储的是一个ASCII码,文件的内容可以直接进行输入输出;2、二进制文件直接将字符存储,不能将二进制文件的内容直接输出到屏幕上。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
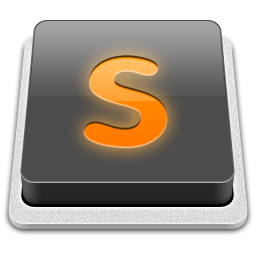
SublimeText3 Mac version
God-level code editing software (SublimeText3)

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
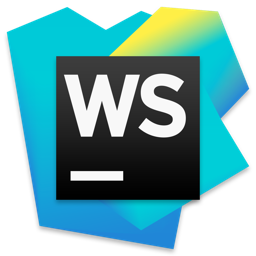
WebStorm Mac version
Useful JavaScript development tools
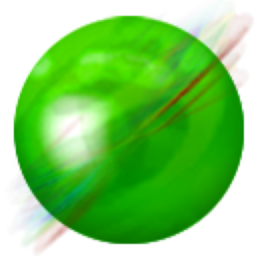
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.