The integration of SQL and Python/R can be implemented through libraries and APIs. 1) In Python, use the sqlite3 library to connect to the database and execute queries. 2) In R, use DBI and RSQLite packages to perform similar operations. Mastering these technologies can improve data processing capabilities.
introduction
In today's data-driven era, the combination of SQL and Python/R has become an indispensable skill for data analysts and scientists. Through this article, you will learn how to seamlessly integrate Python and R with SQL databases for more efficient database operations and advanced analytics. Whether you are a beginner or an experienced professional, mastering these techniques will greatly improve your data processing capabilities.
Review of basic knowledge
Before we dive into the integration of SQL and Python/R, we will first review the related basic concepts. SQL (Structured Query Language) is the standard language used to manage and operate relational databases, while Python and R are popular programming languages, often used in data analysis and statistical computing. Python and R have rich libraries and tools, making interaction with SQL databases simple and efficient.
For example, Python's sqlite3
and psycopg2
libraries can connect to SQLite and PostgreSQL databases, while R's DBI
and RPostgreSQL
packages provide similar functionality. These libraries not only simplify database operations, but also support complex queries and data processing, making data analysis more flexible and powerful.
Core concept or function analysis
SQL and Python/R integration
The integration of SQL and Python/R is mainly implemented through libraries and APIs, which make it very simple to execute SQL queries in code. Let's start with Python and look at a simple example:
import sqlite3 # Connect to SQLite database conn = sqlite3.connect('example.db') cursor = conn.cursor() # Execute SQL query cursor.execute("SELECT * FROM users WHERE age > 18") # Get query results = cursor.fetchall() for row in results: print(row) # Close the connection conn.close()
This code shows how to connect to a SQLite database using the sqlite3
library, execute a simple SELECT query, and print the results. In R, similar operations can be implemented with the following code:
library(DBI) library(RSQLite) # Connect to SQLite database con <- dbConnect(RSQLite::SQLite(), "example.db") # Execute SQL query res <- dbSendQuery(con, "SELECT * FROM users WHERE age > 18") # Get query result data <- dbFetch(res) # Print result print(data) # Clean dbClearResult(res) dbDisconnect(con)
These examples show how to interact with SQL databases through Python and R to enable query and processing of data.
How it works
When we interact with a SQL database using Python or R, the underlying working principle is to send SQL queries to the database server through libraries and APIs, which executes the query and returns the result. Python's sqlite3
library and R's DBI
package are both responsible for managing connections, executing queries and processing results. These libraries simplify interaction with the database, allowing developers to focus on data analysis and processing.
In terms of performance, the execution efficiency of SQL queries depends on the complexity of the query and the optimization level of the database. Query performance can be significantly improved by using indexes, optimizing query statements and database design. Additionally, Python and R support batch operations and transaction processing, which is very useful when handling large amounts of data.
Example of usage
Basic usage
Let's start with a basic example showing how to use SQL queries in Python to analyze data. Let's assume there is a table called sales
that contains sales data:
import sqlite3 conn = sqlite3.connect('sales.db') cursor = conn.cursor() # Execute SQL query to get total sales cursor.execute("SELECT SUM(amount) FROM sales") total_sales = cursor.fetchone()[0] print(f"Total Sales: {total_sales}") conn.close()
This code shows how to calculate total sales using SQL queries and process results in Python.
Advanced Usage
Now let's look at a more complex example showing how to use SQL queries for data analysis in R. Let's assume that there is a table called customers
that contains customer information:
library(DBI) library(RSQLite) con <- dbConnect(RSQLite::SQLite(), "customers.db") # Execute SQL query to get the number of customers grouped by country res <- dbSendQuery(con, "SELECT country, COUNT(*) as count FROM customers GROUP BY country") # Get query result data <- dbFetch(res) # Print result print(data) # Clean dbClearResult(res) dbDisconnect(con)
This code shows how to use SQL queries to calculate the number of customers by country and process the results in R.
Common Errors and Debugging Tips
Common problems may occur when integrating with Python/R using SQL, such as connection failures, query syntax errors, or data type mismatch. Here are some debugging tips:
- Connection problem : Make sure the database server is running properly and check if the connection string and credentials are correct.
- Query error : Check the SQL query syntax carefully to ensure that it meets the database requirements. Use the
try-except
block ortryCatch
function in R to catch and handle exceptions. - Data type problem : Ensure the consistency of data types between Python/R and the database, and perform type conversion if necessary.
Performance optimization and best practices
In practical applications, optimizing the integration of SQL and Python/R can significantly improve data processing efficiency. Here are some optimization tips and best practices:
- Using Index : Create indexes for commonly used query fields in the database, which can significantly improve query speed.
- Batch operations : Use batch insert or update operations instead of processing data line by line to reduce the number of database interactions.
- Transaction processing : Use transactions to ensure data consistency and improve performance, especially when performing multiple related operations.
- Code readability : Write clear, well-annotated code to ensure that team members can easily understand and maintain the code.
- Performance testing : Perform performance testing regularly, compare the effects of different methods, and select the optimal solution.
Through these techniques and practices, you can use SQL and Python/R more efficiently for data analysis and processing, thereby improving your data processing capabilities and project efficiency.
In short, the integration of SQL with Python/R has provided powerful tools and methods for data analysts and scientists. Through the study and practice of this article, you will be able to better utilize these technologies to achieve more efficient data processing and analysis.
The above is the detailed content of SQL with Python/R: Integrating Databases for Advanced Analytics. For more information, please follow other related articles on the PHP Chinese website!

SQLmakesdatamanagementaccessibletoallbyprovidingasimpleyetpowerfultoolsetforqueryingandmanagingdatabases.1)Itworkswithrelationaldatabases,allowinguserstospecifywhattheywanttodowiththedata.2)SQL'sstrengthliesinfiltering,sorting,andjoiningdataacrosstab

SQL indexes can significantly improve query performance through clever design. 1. Select the appropriate index type, such as B-tree, hash or full text index. 2. Use composite index to optimize multi-field query. 3. Avoid over-index to reduce data maintenance overhead. 4. Maintain indexes regularly, including rebuilding and removing unnecessary indexes.
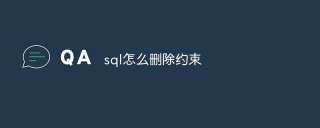
To delete a constraint in SQL, perform the following steps: Identify the constraint name to be deleted; use the ALTER TABLE statement: ALTER TABLE table name DROP CONSTRAINT constraint name; confirm deletion.
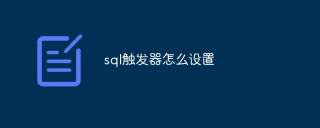
A SQL trigger is a database object that automatically performs specific actions when a specific event is executed on a specified table. To set up SQL triggers, you can use the CREATE TRIGGER statement, which includes the trigger name, table name, event type, and trigger code. The trigger code is defined using the AS keyword and contains SQL or PL/SQL statements or blocks. By specifying trigger conditions, you can use the WHERE clause to limit the execution scope of a trigger. Trigger operations can be performed in the trigger code using the INSERT INTO, UPDATE, or DELETE statement. NEW and OLD keywords can be used to reference the affected keyword in the trigger code.
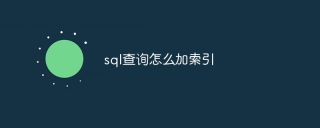
Indexing is a data structure that accelerates data search by sorting data columns. The steps to add an index to an SQL query are as follows: Determine the columns that need to be indexed. Select the appropriate index type (B-tree, hash, or bitmap). Use the CREATE INDEX command to create an index. Reconstruct or reorganize the index regularly to maintain its efficiency. The benefits of adding indexes include improved query performance, reduced I/O operations, optimized sorting and filtering, and improved concurrency. When queries often use specific columns, return large amounts of data that need to be sorted or grouped, involve multiple tables or database tables that are large, you should consider adding an index.
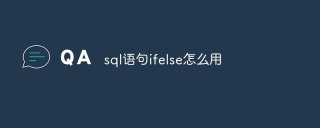
The IFELSE statement is a conditional statement that returns different values based on the conditional evaluation result. Its syntax structure is: IF (condition) THEN return_value_if_condition_is_true ELSE return_value_if_condition_is_false END IF;.
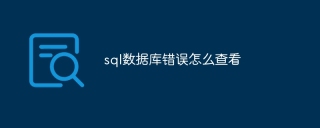
The methods for viewing SQL database errors are: 1. View error messages directly; 2. Use SHOW ERRORS and SHOW WARNINGS commands; 3. Access the error log; 4. Use error codes to find the cause of the error; 5. Check the database connection and query syntax; 6. Use debugging tools.
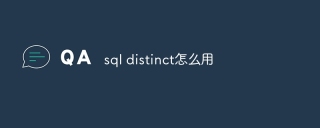
The DISTINCT operator is used to exclude duplicate rows in SQL queries and returns only unique values. It is suitable for scenarios such as obtaining a list of unique values, counting the number of unique values, and using it in combination with GROUP BY.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
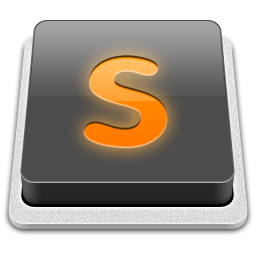
SublimeText3 Mac version
God-level code editing software (SublimeText3)

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SublimeText3 Chinese version
Chinese version, very easy to use

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
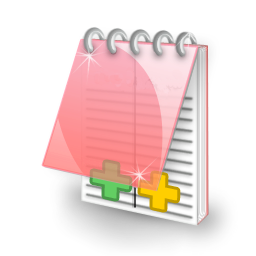
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function