Converting XML into images can be achieved through the following steps: parse XML data and extract visual element information. Select the appropriate graphics library (such as Pillow in Python, JFreeChart in Java) to render the picture. Understand the XML structure and determine how data is processed. Select the appropriate tools and methods based on the XML structure and image complexity. Consider using multithreaded or asynchronous programming to optimize performance while maintaining code readability and maintainability.
Convert XML to image? This question is awesome! It looks simple on the surface, but it actually has a secret. Just use the code to force it? Of course, but efficiency and maintainability... you know. Best practices? It depends on what your XML looks like and what kind of images you want. Don't worry, let me tell you slowly.
Let’s talk about the basics first. XML itself is just a data description language, and it cannot be displayed directly into an image. You need an intermediate layer to parse the XML data into visual elements, and then render it into pictures using the graphics library. In this middle layer, you can choose various tools and languages, which are competent for Python, Java, and even JavaScript. The key is to choose the right library. For example, in Python, you may use xml.etree.ElementTree
to parse XML, Pillow
or ReportLab
to generate images. For Java, DOM4J
and JFreeChart
are good choices.
The core lies in understanding XML structure. How is the information organized in your XML file? Is it a simple key-value pair? Or a complex tree structure? This directly determines how you need to process the data. Suppose your XML describes a simple chart containing data point coordinates, which is relatively simple to process. But if XML describes chapters, paragraphs, and even typesetting information of a whole book, then the workload will be much more.
Let's take a look at a simple example, suppose your XML is like this:
<code class="xml"><chart> <data point="1,10"></data> <data point="2,20"></data> <data point="3,15"></data> </chart></code>
In Python and Pillow, you can write this:
<code class="python">import xml.etree.ElementTree as ET from PIL import Image, ImageDraw tree = ET.parse('chart.xml') root = tree.getroot() width, height = 200, 150 img = Image.new('RGB', (width, height), 'white') draw = ImageDraw.Draw(img) points = [] for data in root.findall('data'): point = data.get('point').split(',') points.append((int(point[0])*10, height - int(point[1]))) draw.line(points, fill='red', width=2) img.save('chart.png')</code>
This code first parses XML, extracts coordinate data, and then draws a line with Pillow. Simple and clear, but only for this simple scenario. For more complex XML, you may need more complex logic, and even need to introduce a template engine to control the layout and style of images.
More advanced usage? Imagine that your XML contains multiple elements such as text, pictures, tables, etc. You need to dynamically generate complex pictures based on the XML structure. At this point, you may want to consider using a more powerful graphics library, or writing a rendering engine yourself. This will involve font rendering, image processing, layout algorithms, etc., and the difficulty will be significantly increased.
Common errors? XML parsing errors are the most common. Make sure your XML file is formatted correctly and avoid missing tags or attributes. In addition, pay attention to data type conversion to avoid program crashes due to type mismatch. When debugging, printing the value of the intermediate variable can help you quickly locate the problem.
Performance optimization? For large XML files, parsing and rendering can take a long time. Multithreading or asynchronous programming can be considered to improve efficiency. In addition, choosing the right algorithm and data structure can also improve performance. For example, using a suitable layout algorithm can reduce rendering time. Remember, the readability and maintainability of the code are also important, and don't write difficult code to pursue extreme performance.
In short, there is no "universal" best practice for XML to convert images, only the solution that best suits your specific needs. You need to choose the right tools and methods based on the structure of the XML, the complexity of the image, and your technology stack. Remember to figure out the requirements first, then choose tools, and finally write code. Don't dive into the code from the beginning, otherwise you will find that what you write may not be what you want at all.
The above is the detailed content of What are the best practices for converting XML into images?. For more information, please follow other related articles on the PHP Chinese website!
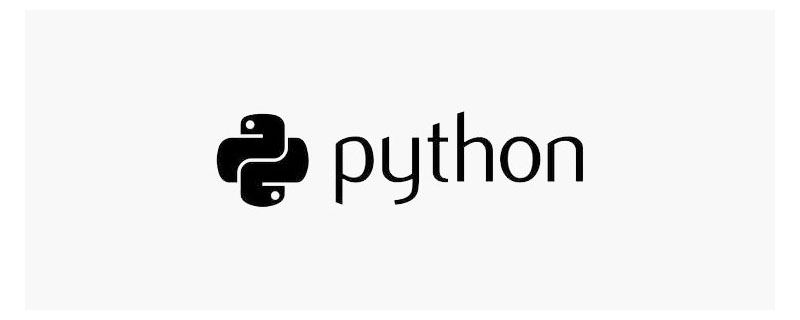
本篇文章给大家带来了关于Python的相关知识,其中主要介绍了关于Seaborn的相关问题,包括了数据可视化处理的散点图、折线图、条形图等等内容,下面一起来看一下,希望对大家有帮助。
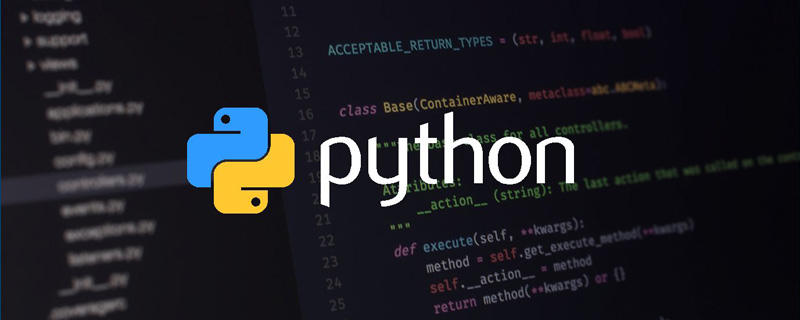
本篇文章给大家带来了关于Python的相关知识,其中主要介绍了关于进程池与进程锁的相关问题,包括进程池的创建模块,进程池函数等等内容,下面一起来看一下,希望对大家有帮助。
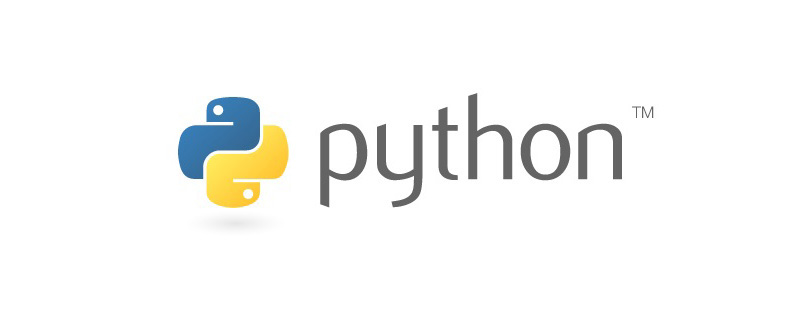
本篇文章给大家带来了关于Python的相关知识,其中主要介绍了关于简历筛选的相关问题,包括了定义 ReadDoc 类用以读取 word 文件以及定义 search_word 函数用以筛选的相关内容,下面一起来看一下,希望对大家有帮助。
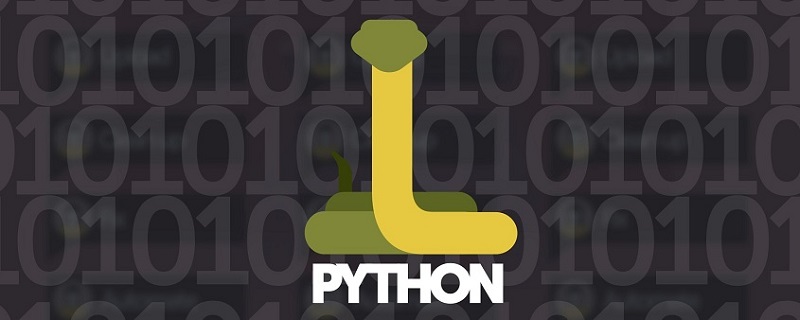
本篇文章给大家带来了关于Python的相关知识,其中主要介绍了关于数据类型之字符串、数字的相关问题,下面一起来看一下,希望对大家有帮助。
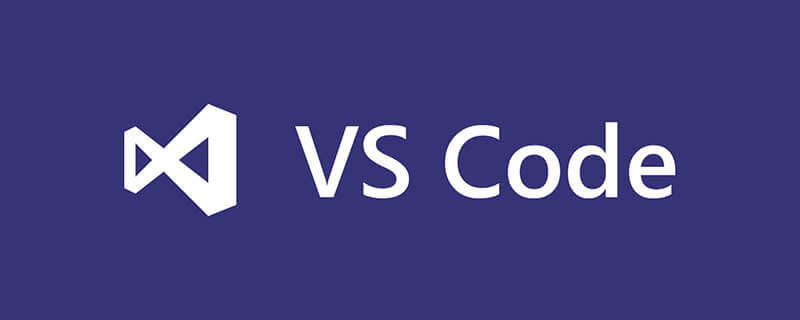
VS Code的确是一款非常热门、有强大用户基础的一款开发工具。本文给大家介绍一下10款高效、好用的插件,能够让原本单薄的VS Code如虎添翼,开发效率顿时提升到一个新的阶段。
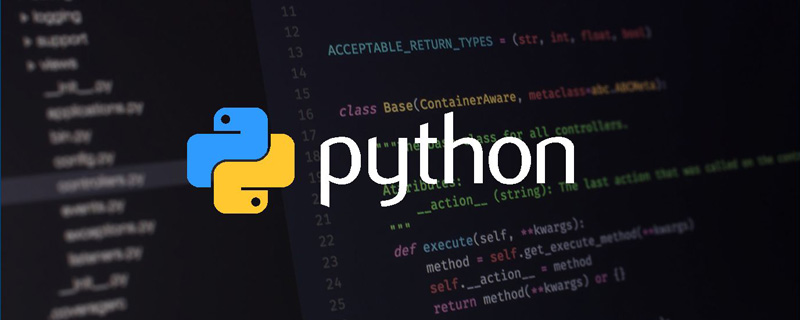
本篇文章给大家带来了关于Python的相关知识,其中主要介绍了关于numpy模块的相关问题,Numpy是Numerical Python extensions的缩写,字面意思是Python数值计算扩展,下面一起来看一下,希望对大家有帮助。
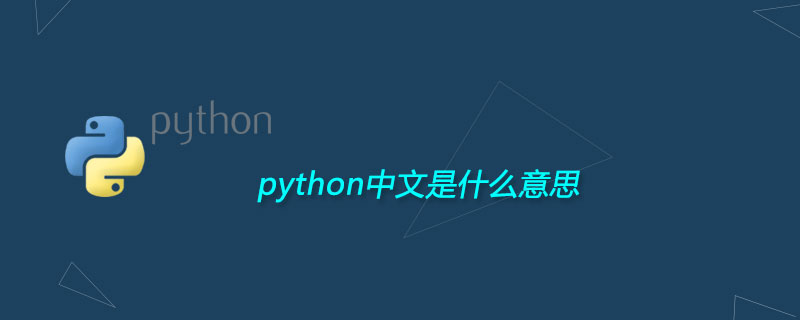
pythn的中文意思是巨蟒、蟒蛇。1989年圣诞节期间,Guido van Rossum在家闲的没事干,为了跟朋友庆祝圣诞节,决定发明一种全新的脚本语言。他很喜欢一个肥皂剧叫Monty Python,所以便把这门语言叫做python。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor
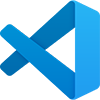
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SublimeText3 Linux new version
SublimeText3 Linux latest version

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
