How do you organize your Vue.js project structure?
Organizing a Vue.js project structure effectively is crucial for maintaining the project's readability, scalability, and ease of collaboration. Here's a common approach to structuring a Vue.js project:
-
Root Directory: At the top level of your project, you'll typically find configuration files like
package.json
,.gitignore
, andREADME.md
. -
src Directory: This is where your application's source code lives. Inside the
src
directory, you might organize your project as follows:- assets: For static files like images, fonts, or any other media.
- components: For reusable Vue components.
- views or pages: For the main views or pages of your application.
-
router: For routing configurations, typically containing
index.js
. - store: For Vuex store files if you're using Vuex for state management.
- styles: For global stylesheets or CSS modules.
- utils: For utility functions and helpers.
- App.vue: The root component of your application.
- main.js: The entry point that initializes your Vue app.
-
public Directory: For static assets that are copied over without being processed by webpack, including
index.html
. - tests Directory: For unit and integration tests.
This structure helps developers quickly understand where different parts of the application are located and makes it easier to maintain and scale the application over time.
What are the best practices for managing components in a Vue.js project?
Managing components effectively is key to developing a maintainable Vue.js application. Here are some best practices:
- Single Responsibility Principle: Ensure that each component is responsible for only one thing. This makes components easier to test and reuse.
- Component Naming: Use descriptive names for components and follow a consistent naming convention. For example, use PascalCase for component names and kebab-case for file names.
- Component Size: Keep components small and focused. If a component is getting too complex, consider breaking it down into smaller sub-components.
- Props and Events: Use props for passing data down to child components and custom events for passing data back up to parent components. This helps maintain a unidirectional data flow.
- Scoped Styles: Use scoped CSS or CSS modules to ensure styles don't bleed across components.
- State Management: For complex applications, consider using Vuex or another state management solution to manage global state, reducing the need for prop drilling.
- Documentation: Document your components, especially if they are to be used by other team members. Use JSDoc comments to describe props, events, and methods.
- Reusability: Design components with reusability in mind. Generic components should be placed in a shared folder, while specific components can be placed closer to their usage.
How can folder organization improve the scalability of a Vue.js application?
Proper folder organization plays a significant role in the scalability of a Vue.js application by:
- Reducing Complexity: As the application grows, a well-organized folder structure helps developers navigate the codebase more easily, reducing the cognitive load when making changes or adding new features.
- Enhancing Collaboration: When team members understand where to find different parts of the application, collaboration becomes more efficient. A clear structure helps new team members get up to speed quickly.
- Improving Maintainability: With a logical organization, it's easier to identify where to make updates or fix bugs. This can reduce the time spent on maintenance tasks.
-
Facilitating Reuse: Organizing components into logical categories (e.g.,
components/common
,components/forms
) makes it easier to reuse code across different parts of the application. - Supporting Modular Architecture: A well-organized structure supports a modular approach, where different parts of the application can be developed, tested, and maintained independently.
- Scaling with Features: As new features are added, they can be slotted into the existing structure without causing chaos, ensuring the application remains scalable.
What tools or methods can help maintain a clean Vue.js project structure?
Maintaining a clean Vue.js project structure can be aided by the following tools and methods:
-
Linters: Tools like ESLint can enforce coding standards and catch errors early. For Vue.js projects, you can use
eslint-plugin-vue
to specifically lint Vue-related code. - Code Formatters: Tools like Prettier can automatically format your code to maintain consistency across the project.
- Modularization: Use tools like Vue CLI to set up a project with a recommended structure, or use a monorepo approach with tools like Lerna for managing multiple packages.
- Version Control: Utilize Git with proper branching strategies to keep the codebase clean and track changes effectively.
- Static Analysis Tools: Tools like SonarQube can help identify code smells and potential issues that might lead to a messy structure.
- Automated Testing: Implement a robust testing strategy using tools like Jest and Vue Test Utils to ensure components and modules function correctly within the structure.
- Documentation Tools: Use documentation generators like JSDoc to keep component and module documentation up to date, which helps in maintaining clarity in the project structure.
- Code Review: Regular code reviews by peers can help catch issues related to structure and organization early on.
- Refactoring Tools: Use IDEs with refactoring capabilities, like VSCode with the Vue VSCode Extension, to safely restructure your project.
By leveraging these tools and methods, you can maintain a clean and organized Vue.js project, making it easier to manage as it grows in complexity and size.
The above is the detailed content of How do you organize your Vue.js project structure?. For more information, please follow other related articles on the PHP Chinese website!

The future trends and forecasts of Vue.js and React are: 1) Vue.js will be widely used in enterprise-level applications and have made breakthroughs in server-side rendering and static site generation; 2) React will innovate in server components and data acquisition, and further optimize the concurrency model.

Netflix's front-end technology stack is mainly based on React and Redux. 1.React is used to build high-performance single-page applications, and improves code reusability and maintenance through component development. 2. Redux is used for state management to ensure that state changes are predictable and traceable. 3. The toolchain includes Webpack, Babel, Jest and Enzyme to ensure code quality and performance. 4. Performance optimization is achieved through code segmentation, lazy loading and server-side rendering to improve user experience.

Vue.js is a progressive framework suitable for building highly interactive user interfaces. Its core functions include responsive systems, component development and routing management. 1) The responsive system realizes data monitoring through Object.defineProperty or Proxy, and automatically updates the interface. 2) Component development allows the interface to be split into reusable modules. 3) VueRouter supports single-page applications to improve user experience.

The main disadvantages of Vue.js include: 1. The ecosystem is relatively new, and third-party libraries and tools are not as rich as other frameworks; 2. The learning curve becomes steep in complex functions; 3. Community support and resources are not as extensive as React and Angular; 4. Performance problems may be encountered in large applications; 5. Version upgrades and compatibility challenges are greater.

Netflix uses React as its front-end framework. 1.React's component development and virtual DOM mechanism improve performance and development efficiency. 2. Use Webpack and Babel to optimize code construction and deployment. 3. Use code segmentation, server-side rendering and caching strategies for performance optimization.

Reasons for Vue.js' popularity include simplicity and easy learning, flexibility and high performance. 1) Its progressive framework design is suitable for beginners to learn step by step. 2) Component-based development improves code maintainability and team collaboration efficiency. 3) Responsive systems and virtual DOM improve rendering performance.

Vue.js is easier to use and has a smooth learning curve, which is suitable for beginners; React has a steeper learning curve, but has strong flexibility, which is suitable for experienced developers. 1.Vue.js is easy to get started with through simple data binding and progressive design. 2.React requires understanding of virtual DOM and JSX, but provides higher flexibility and performance advantages.

Vue.js is suitable for fast development and small projects, while React is more suitable for large and complex projects. 1.Vue.js is simple and easy to learn, suitable for rapid development and small projects. 2.React is powerful and suitable for large and complex projects. 3. The progressive features of Vue.js are suitable for gradually introducing functions. 4. React's componentized and virtual DOM performs well when dealing with complex UI and data-intensive applications.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
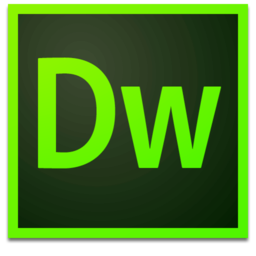
Dreamweaver Mac version
Visual web development tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
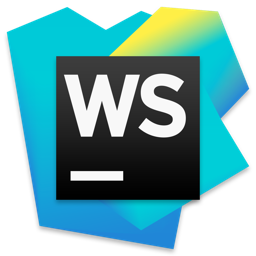
WebStorm Mac version
Useful JavaScript development tools
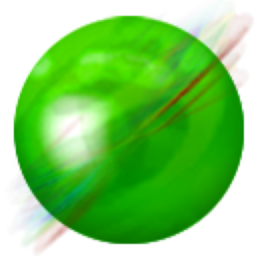
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
