What are the different ways to handle side effects in Vue.js components?
In Vue.js, handling side effects efficiently is crucial for maintaining the reactivity and performance of applications. There are several ways to manage side effects in Vue.js components:
-
Lifecycle Hooks: Vue.js components have lifecycle hooks that are invoked at specific stages of a component's life. These hooks, such as
created
,mounted
,updated
, andbeforeDestroy
, allow you to handle side effects at appropriate times. For example, you might use themounted
hook to initiate API calls or set up event listeners. -
Watchers: Vue.js provides the
watch
option to react to data changes. This is useful for handling side effects that depend on reactive data. You can use watchers to trigger actions when a particular piece of data changes, which is useful for managing asynchronous operations or other side effects. - Computed Properties: While not typically used for side effects, computed properties can be employed to handle them in certain scenarios. For instance, you can use a computed property to trigger a side effect based on a dependency, although this is less common and should be used cautiously.
- Methods: Methods in Vue.js components can be used to handle side effects, especially when they need to be triggered by user interactions or other events. For example, a method might be called when a button is clicked to perform an API call.
-
Composition API: With the introduction of the Composition API in Vue 3, you can use
setup
function andref
/reactive
to manage side effects more flexibly. TheonMounted
,onUpdated
, and other lifecycle hooks from the Composition API can be used to handle side effects in a more modular and reusable way.
How can I effectively manage asynchronous operations in Vue.js?
Managing asynchronous operations in Vue.js is essential for creating responsive and efficient applications. Here are some strategies to effectively handle asynchronous operations:
-
Promises and Async/Await: Use Promises or the
async/await
syntax to handle asynchronous operations. This makes your code more readable and easier to manage. For example, you can useasync/await
within lifecycle hooks or methods to wait for API responses before updating the component's state.async mounted() { try { const response = await fetch('api/data'); this.data = await response.json(); } catch (error) { console.error('Error fetching data:', error); } }
-
Loading States: Implement loading states to provide feedback to users while waiting for asynchronous operations to complete. You can use a boolean flag to toggle a loading indicator.
data() { return { isLoading: false, data: null }; }, async mounted() { this.isLoading = true; try { const response = await fetch('api/data'); this.data = await response.json(); } catch (error) { console.error('Error fetching data:', error); } finally { this.isLoading = false; } }
- Error Handling: Properly handle errors that may occur during asynchronous operations. Use try/catch blocks to catch and handle errors gracefully, and consider displaying error messages to the user.
- Debouncing and Throttling: For operations that might be triggered frequently (like search queries), use debouncing or throttling to limit the number of asynchronous calls. Libraries like Lodash can help implement these techniques.
- Optimistic UI Updates: In some cases, you can update the UI optimistically before the asynchronous operation completes, then revert if the operation fails. This can improve perceived performance.
What are the best practices for using lifecycle hooks to manage side effects in Vue.js?
Using lifecycle hooks effectively to manage side effects in Vue.js involves following best practices to ensure your application remains maintainable and efficient. Here are some key practices:
-
Use Appropriate Hooks: Choose the right lifecycle hook for your side effect. For example, use
mounted
for DOM-related side effects,created
for initializing data, andbeforeDestroy
for cleanup tasks. - Avoid Overloading Hooks: Keep lifecycle hooks focused on specific tasks. If a hook becomes too complex, consider breaking it down into smaller, more manageable methods.
-
Cleanup in
beforeDestroy
: Always clean up side effects in thebeforeDestroy
hook. This includes removing event listeners, canceling timers, or aborting ongoing requests to prevent memory leaks.mounted() { this.timer = setInterval(this.updateData, 1000); }, beforeDestroy() { clearInterval(this.timer); }
-
Use
watch
for Reactive Side Effects: If a side effect depends on reactive data, use awatch
instead of a lifecycle hook. This ensures the side effect is triggered whenever the data changes. - Avoid Side Effects in Render Functions: Never perform side effects within the render function or computed properties, as this can lead to performance issues and unexpected behavior.
- Test Lifecycle Hooks: Write unit tests for lifecycle hooks to ensure they behave as expected. This is particularly important for hooks that handle complex side effects.
What tools or libraries can enhance side effect management in Vue.js applications?
Several tools and libraries can enhance side effect management in Vue.js applications, making it easier to handle asynchronous operations and maintain clean, efficient code:
- Vuex: Vuex is the official state management library for Vue.js. It helps manage global state and side effects across your application. Vuex actions are a great way to handle side effects, especially asynchronous operations.
- Vue Router: While primarily used for routing, Vue Router can help manage side effects related to navigation, such as fetching data when a route changes.
- Axios: Axios is a popular HTTP client for making API requests. It integrates well with Vue.js and can be used within lifecycle hooks or Vuex actions to handle asynchronous data fetching.
-
Lodash: Lodash provides utility functions like
debounce
andthrottle
, which are useful for managing side effects triggered by frequent user interactions. -
Vue Composition API: The Composition API, introduced in Vue 3, offers a more flexible way to manage side effects using the
setup
function and lifecycle hooks likeonMounted
andonUpdated
. - Pinia: Pinia is a modern state management library for Vue.js that offers a simpler and more intuitive API than Vuex. It's particularly useful for managing side effects in a modular way.
-
VueUse: VueUse is a collection of composition API utilities that include many functions for handling side effects, such as
useFetch
,useAsyncState
, anduseTimeout
.
By leveraging these tools and following best practices, you can effectively manage side effects in your Vue.js applications, leading to more robust and maintainable code.
The above is the detailed content of What are the different ways to handle side effects in Vue.js components?. For more information, please follow other related articles on the PHP Chinese website!

WhentheVue.jsVirtualDOMdetectsachange,itupdatestheVirtualDOM,diffsit,andappliesminimalchangestotherealDOM.ThisprocessensureshighperformancebyavoidingunnecessaryDOMmanipulations.

Vue.js' VirtualDOM is both a mirror of the real DOM, and not exactly. 1. Create and update: Vue.js creates a VirtualDOM tree based on component definitions, and updates VirtualDOM first when the state changes. 2. Differences and patching: Comparison of old and new VirtualDOMs through diff operations, and apply only the minimum changes to the real DOM. 3. Efficiency: VirtualDOM allows batch updates, reduces direct DOM operations, and optimizes the rendering process. VirtualDOM is a strategic tool for Vue.js to optimize UI updates.

Vue.js and React each have their own advantages in scalability and maintainability. 1) Vue.js is easy to use and is suitable for small projects. The Composition API improves the maintainability of large projects. 2) React is suitable for large and complex projects, with Hooks and virtual DOM improving performance and maintainability, but the learning curve is steeper.

The future trends and forecasts of Vue.js and React are: 1) Vue.js will be widely used in enterprise-level applications and have made breakthroughs in server-side rendering and static site generation; 2) React will innovate in server components and data acquisition, and further optimize the concurrency model.

Netflix's front-end technology stack is mainly based on React and Redux. 1.React is used to build high-performance single-page applications, and improves code reusability and maintenance through component development. 2. Redux is used for state management to ensure that state changes are predictable and traceable. 3. The toolchain includes Webpack, Babel, Jest and Enzyme to ensure code quality and performance. 4. Performance optimization is achieved through code segmentation, lazy loading and server-side rendering to improve user experience.

Vue.js is a progressive framework suitable for building highly interactive user interfaces. Its core functions include responsive systems, component development and routing management. 1) The responsive system realizes data monitoring through Object.defineProperty or Proxy, and automatically updates the interface. 2) Component development allows the interface to be split into reusable modules. 3) VueRouter supports single-page applications to improve user experience.

The main disadvantages of Vue.js include: 1. The ecosystem is relatively new, and third-party libraries and tools are not as rich as other frameworks; 2. The learning curve becomes steep in complex functions; 3. Community support and resources are not as extensive as React and Angular; 4. Performance problems may be encountered in large applications; 5. Version upgrades and compatibility challenges are greater.

Netflix uses React as its front-end framework. 1.React's component development and virtual DOM mechanism improve performance and development efficiency. 2. Use Webpack and Babel to optimize code construction and deployment. 3. Use code segmentation, server-side rendering and caching strategies for performance optimization.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
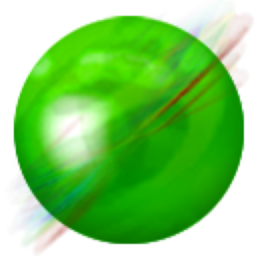
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SublimeText3 Chinese version
Chinese version, very easy to use
