


How does React Streaming work, and how does it improve perceived performance?
How does React Streaming work, and how does it improve perceived performance?
React Streaming is a technique used in web development to improve the user experience by reducing the time it takes for content to be displayed on the screen. This method is particularly effective in React applications, where traditional rendering might lead to slower load times due to the need to fully render the entire page before displaying any content.
How React Streaming Works:
-
Server-Side Rendering (SSR) with Streaming:
React Streaming starts with server-side rendering. Instead of waiting for the entire page to be rendered on the server and then sending the complete HTML to the client, React Streaming allows the server to start sending chunks of HTML as they are ready. This means that the server can send parts of the page that are already rendered, while continuing to process other parts. -
Progressive Hydration:
Once the initial chunks of HTML are sent to the client, the browser can start displaying content immediately. As more chunks arrive, the browser appends them to the DOM. This process is known as progressive hydration. React then hydrates these chunks one by one, converting the server-rendered HTML into fully interactive React components. -
Suspense and Concurrent Rendering:
React's Suspense feature allows components to suspend their rendering if they're waiting for data. With concurrent rendering, React can prioritize rendering components that are ready, while others can be streamed later. This allows for a more flexible and efficient rendering pipeline.
Improving Perceived Performance:
- Faster Time to First Paint (TTFP): By sending HTML chunks as soon as they are ready, users see content on the screen much faster than with traditional rendering methods. This significantly improves the Time to First Paint, which is crucial for user engagement.
- Reduced Time to Interactive (TTI): As components are progressively hydrated, the page becomes interactive in stages. This means that users can start interacting with parts of the page before the entire application is fully loaded, reducing the overall Time to Interactive.
- Better User Experience: Users perceive the application as faster and more responsive, which can lead to lower bounce rates and higher user satisfaction.
What are the specific performance benefits of using React Streaming in web applications?
React Streaming offers several specific performance benefits for web applications:
-
Improved Load Times:
By sending and displaying content in chunks, React Streaming significantly reduces the initial load time of the application. This is particularly beneficial for large, complex applications where traditional rendering might take longer. -
Enhanced User Engagement:
With faster Time to First Paint and Time to Interactive, users are more likely to stay on the page and engage with the content. This can lead to higher conversion rates and better overall user experience. -
Better Resource Management:
React Streaming allows for more efficient use of both server and client resources. The server can process and send data in smaller, manageable chunks, while the client can start rendering content without waiting for the entire page to load. -
Optimized for Slow Networks:
For users on slower networks, React Streaming can make a significant difference. By sending content incrementally, users on slow connections can start interacting with parts of the application much sooner than if they had to wait for the entire page to load. -
SEO Benefits:
Search engines can crawl and index content more quickly since the server sends chunks of HTML as they are ready. This can improve the search engine ranking of the application.
How can developers implement React Streaming to enhance user experience?
To implement React Streaming and enhance user experience, developers can follow these steps:
-
Set Up Server-Side Rendering (SSR):
Start by setting up server-side rendering for your React application. This can be done using frameworks like Next.js, which supports SSR out of the box. -
Enable Streaming:
Configure your server to enable streaming. In Next.js, this can be done by setting thestream
option in thenext.config.js
file. For custom setups, you'll need to implement streaming on your server. -
Use React Suspense:
Utilize React Suspense to handle asynchronous data loading and rendering. Wrap your components with Suspense to manage loading states and render fallbacks while waiting for data.import { Suspense } from 'react'; function MyComponent() { return ( <Suspense fallback={<div>Loading...</div>}> <AsyncComponent /> </Suspense> ); }
-
Implement Progressive Hydration:
Ensure that your application supports progressive hydration. This can be done by using libraries likereact-dom/server
to stream the initial HTML and then hydrate it progressively on the client side.import { renderToPipeableStream } from 'react-dom/server'; import App from './App'; const stream = renderToPipeableStream(<App />);
-
Optimize Components:
Break down your application into smaller, manageable components that can be streamed independently. This allows for more granular control over the rendering process and can improve performance. -
Test and Monitor:
Thoroughly test your application to ensure that streaming is working as expected. Use performance monitoring tools to track metrics like TTFP, TTI, and overall load times to validate the improvements.
What challenges might developers face when adopting React Streaming, and how can they be overcome?
Adopting React Streaming can present several challenges for developers, but with the right approach, these can be overcome:
-
Complexity of Setup:
Setting up streaming can be complex, especially for developers new to server-side rendering and streaming concepts.Solution: Use frameworks like Next.js that provide built-in support for streaming. These frameworks simplify the setup process and handle many of the complexities behind the scenes.
-
Debugging and Error Handling:
Debugging streaming applications can be more challenging due to the asynchronous nature of the rendering process.Solution: Implement robust error handling and logging mechanisms. Use tools like React DevTools and browser developer tools to track the rendering pipeline and identify issues.
-
Performance Overhead:
Streaming can introduce additional overhead, particularly on the server side, as it needs to manage multiple streams and handle partial rendering.Solution: Optimize server performance by using efficient data fetching techniques and caching mechanisms. Ensure that your server infrastructure can handle the increased load.
-
Inconsistent User Experience:
If not implemented correctly, streaming can lead to an inconsistent user experience, where some parts of the page load quickly while others take longer.Solution: Carefully design your component architecture to ensure that critical parts of the page load first. Use Suspense and concurrent rendering to prioritize the rendering of important components.
-
SEO Considerations:
Search engines might have difficulty crawling and indexing content that is loaded asynchronously.Solution: Ensure that the initial chunks of HTML sent to the client contain enough content to be indexed by search engines. Use server-side rendering to generate static HTML that can be easily crawled.
By addressing these challenges with the right strategies and tools, developers can successfully implement React Streaming and significantly enhance the performance and user experience of their web applications.
The above is the detailed content of How does React Streaming work, and how does it improve perceived performance?. For more information, please follow other related articles on the PHP Chinese website!

React is a front-end framework for building user interfaces; a back-end framework is used to build server-side applications. React provides componentized and efficient UI updates, and the backend framework provides a complete backend service solution. When choosing a technology stack, project requirements, team skills, and scalability should be considered.

The relationship between HTML and React is the core of front-end development, and they jointly build the user interface of modern web applications. 1) HTML defines the content structure and semantics, and React builds a dynamic interface through componentization. 2) React components use JSX syntax to embed HTML to achieve intelligent rendering. 3) Component life cycle manages HTML rendering and updates dynamically according to state and attributes. 4) Use components to optimize HTML structure and improve maintainability. 5) Performance optimization includes avoiding unnecessary rendering, using key attributes, and keeping the component single responsibility.

React is the preferred tool for building interactive front-end experiences. 1) React simplifies UI development through componentization and virtual DOM. 2) Components are divided into function components and class components. Function components are simpler and class components provide more life cycle methods. 3) The working principle of React relies on virtual DOM and reconciliation algorithm to improve performance. 4) State management uses useState or this.state, and life cycle methods such as componentDidMount are used for specific logic. 5) Basic usage includes creating components and managing state, and advanced usage involves custom hooks and performance optimization. 6) Common errors include improper status updates and performance issues, debugging skills include using ReactDevTools and Excellent

React is a JavaScript library for building user interfaces, with its core components and state management. 1) Simplify UI development through componentization and state management. 2) The working principle includes reconciliation and rendering, and optimization can be implemented through React.memo and useMemo. 3) The basic usage is to create and render components, and the advanced usage includes using Hooks and ContextAPI. 4) Common errors such as improper status update, you can use ReactDevTools to debug. 5) Performance optimization includes using React.memo, virtualization lists and CodeSplitting, and keeping code readable and maintainable is best practice.

React combines JSX and HTML to improve user experience. 1) JSX embeds HTML to make development more intuitive. 2) The virtual DOM mechanism optimizes performance and reduces DOM operations. 3) Component-based management UI to improve maintainability. 4) State management and event processing enhance interactivity.

React components can be defined by functions or classes, encapsulating UI logic and accepting input data through props. 1) Define components: Use functions or classes to return React elements. 2) Rendering component: React calls render method or executes function component. 3) Multiplexing components: pass data through props to build a complex UI. The lifecycle approach of components allows logic to be executed at different stages, improving development efficiency and code maintainability.
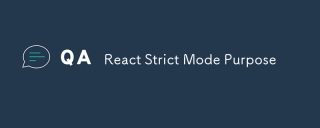
React Strict Mode is a development tool that highlights potential issues in React applications by activating additional checks and warnings. It helps identify legacy code, unsafe lifecycles, and side effects, encouraging modern React practices.
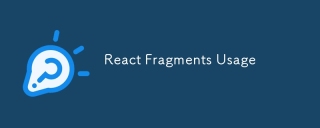
React Fragments allow grouping children without extra DOM nodes, enhancing structure, performance, and accessibility. They support keys for efficient list rendering.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
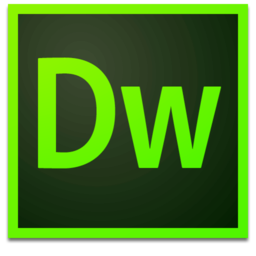
Dreamweaver Mac version
Visual web development tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.