


What are the different frameworks for server-side rendering React applications (e.g., Next.js, Gatsby)?
Server-side rendering (SSR) of React applications has gained popularity for its ability to improve performance and SEO. Several frameworks have emerged to facilitate this process, with Next.js and Gatsby being two of the most prominent ones.
- Next.js: Next.js is a flexible React framework that allows developers to render pages both on the server and the client side. It supports both server-side rendering (SSR) and static site generation (SSG). Next.js is designed for building production-ready applications with features like automatic code splitting, optimized images, and internationalization (i18n) support. It also provides an easy way to implement server-side logic and API routes directly within the framework.
- Gatsby: Gatsby is a static site generator built on top of React that can pre-render pages at build time. It excels in creating fast, secure, and scalable websites with an emphasis on static site generation (SSG). Gatsby uses GraphQL to query data from various sources, which can be used to populate pages during the build process. While Gatsby is primarily focused on SSG, recent updates have introduced support for SSR and Deferred Static Generation (DSG), allowing for more flexible rendering options.
Other frameworks for server-side rendering of React applications include:
- Razzle: An open-source framework that abstracts away the complexity of server-side rendering, allowing developers to focus on building their application without worrying about the underlying configuration.
- After.js: A framework that simplifies the process of creating universal JavaScript applications by providing a server-rendered approach that works out of the box with React Router.
Each of these frameworks has its own strengths and use cases, and the choice between them often depends on the specific requirements of the project, such as performance needs, development team expertise, and the desired balance between server and client-side rendering.
How does Next.js compare to Gatsby in terms of performance and ease of use for server-side rendering?
Performance:
- Next.js: Next.js offers robust performance for server-side rendering. It supports on-demand rendering, where pages can be generated on each request, which is ideal for content that changes frequently. Next.js also includes features like automatic code splitting, which reduces the initial load time of pages. Additionally, its built-in support for server-side logic and API routes allows for efficient data fetching and processing on the server, which can further enhance performance.
- Gatsby: Gatsby's primary focus on static site generation results in excellent performance for websites with static content. By pre-rendering pages at build time, Gatsby can serve content quickly from a CDN, leading to fast page loads. However, for dynamic content, Gatsby's recent updates to support SSR and DSG provide more flexibility, but the performance benefits of these features may not be as pronounced as Next.js's native SSR capabilities.
Ease of Use:
- Next.js: Next.js is often praised for its ease of use, especially for developers familiar with React. It follows a file-system-based routing approach, making it straightforward to organize and manage routes. Additionally, Next.js's built-in support for server-side logic and API routes simplifies the development process, as developers can handle both frontend and backend concerns within the same project.
- Gatsby: Gatsby is user-friendly for those who prefer a static site generator, especially if they are comfortable with GraphQL. Its data layer, powered by GraphQL, allows for easy data querying and integration from multiple sources. However, setting up and managing the data layer can add complexity, particularly for developers new to GraphQL. Additionally, while Gatsby's recent updates have improved its support for SSR and DSG, the learning curve may be steeper compared to Next.js's straightforward SSR implementation.
In summary, Next.js might be preferred for its ease of use and performance in server-side rendering scenarios, especially for applications with dynamic content. Gatsby, on the other hand, excels in performance for static sites and offers a unique data management approach that can be advantageous for certain projects.
What are the key features of Next.js that make it suitable for server-side rendering React applications?
Next.js has several key features that make it an excellent choice for server-side rendering of React applications:
- Automatic Code Splitting: Next.js automatically splits code into smaller chunks, which can be loaded on demand. This feature reduces the initial load time of pages, enhancing overall application performance.
- Server-Side Rendering (SSR): Next.js natively supports SSR, allowing pages to be rendered on the server before being sent to the client. This can improve SEO and initial page load times, especially for content-heavy applications.
- Static Site Generation (SSG): In addition to SSR, Next.js supports SSG, enabling developers to pre-render pages at build time. This is useful for creating static sites that can still benefit from React's interactivity.
- API Routes: Next.js includes built-in support for API routes, allowing developers to handle server-side logic and create RESTful APIs directly within the application. This simplifies the development process and enhances the integration between frontend and backend.
- File-System-Based Routing: Next.js uses a file-system-based approach to routing, which makes it easy to manage and organize routes. This simplifies the development process and reduces the complexity of setting up routing.
- Internationalization (i18n) Support: Next.js provides built-in support for internationalization, allowing developers to easily create multi-language applications. This feature is particularly useful for applications that need to serve a global audience.
- Optimized Images: Next.js includes features for optimizing images, which can significantly improve the performance of applications by reducing the file size and load time of images.
- Incremental Static Regeneration (ISR): Next.js's ISR feature allows for the regeneration of static pages at runtime without rebuilding the entire site. This is useful for updating static content without incurring the cost of a full rebuild.
These features collectively make Next.js a powerful and versatile framework for building server-side rendered React applications, capable of handling a wide range of use cases from static sites to dynamic, data-driven applications.
Can you explain how Gatsby's static site generation differs from Next.js's server-side rendering approach?
Gatsby's Static Site Generation (SSG):
Gatsby primarily focuses on static site generation, where pages are pre-rendered at build time. Here's how it works:
- Build Time Pre-Rendering: When you run a build command in Gatsby, it fetches data from various sources (e.g., local files, APIs, databases) using GraphQL. Based on this data, Gatsby generates static HTML files for each page.
- Static Serving: The generated HTML files are then served directly from a CDN, ensuring fast page loads as the content is already pre-rendered and ready to be displayed.
- Client-Side Hydration: Once the initial HTML is loaded, Gatsby uses client-side JavaScript to hydrate the static content, making it interactive. This approach combines the benefits of static site performance with the interactivity of React.
- Recent Updates: Recent updates to Gatsby have introduced support for Server-Side Rendering (SSR) and Deferred Static Generation (DSG), allowing developers to choose the appropriate rendering method for different parts of their site. However, the core strength of Gatsby remains in its static site generation capabilities.
Next.js's Server-Side Rendering (SSR):
Next.js, on the other hand, supports both server-side rendering and static site generation, but it is particularly strong in server-side rendering. Here's how it works:
- On-Demand Rendering: With Next.js's SSR, pages are generated on each request. When a user visits a page, Next.js renders it on the server, incorporating any necessary data fetching or processing, and then sends the HTML to the client.
- Dynamic Content: This approach is ideal for content that changes frequently or for applications that require real-time data. Next.js can handle server-side logic and API routes directly within the application, making it well-suited for dynamic content.
- Hybrid Approach: Next.js also supports Static Site Generation (SSG), allowing developers to pre-render pages at build time like Gatsby. However, Next.js goes a step further with Incremental Static Regeneration (ISR), which enables updating static pages at runtime without a full rebuild.
- Flexibility: Next.js provides more flexibility in choosing the rendering method for each page. Developers can decide whether a page should be rendered on the server, statically generated, or a combination of both, depending on the specific requirements of their application.
In summary, Gatsby's static site generation focuses on pre-rendering content at build time, which is optimal for static sites but has limitations for dynamic content. Next.js's server-side rendering, on the other hand, allows for on-demand rendering of pages, making it suitable for applications with dynamic content and real-time data requirements. Both frameworks offer versatile rendering options, but their approaches and strengths differ based on the use case.
The above is the detailed content of What are the different frameworks for server-side rendering React applications (e.g., Next.js, Gatsby)?. For more information, please follow other related articles on the PHP Chinese website!

React is the preferred tool for building interactive front-end experiences. 1) React simplifies UI development through componentization and virtual DOM. 2) Components are divided into function components and class components. Function components are simpler and class components provide more life cycle methods. 3) The working principle of React relies on virtual DOM and reconciliation algorithm to improve performance. 4) State management uses useState or this.state, and life cycle methods such as componentDidMount are used for specific logic. 5) Basic usage includes creating components and managing state, and advanced usage involves custom hooks and performance optimization. 6) Common errors include improper status updates and performance issues, debugging skills include using ReactDevTools and Excellent

React is a JavaScript library for building user interfaces, with its core components and state management. 1) Simplify UI development through componentization and state management. 2) The working principle includes reconciliation and rendering, and optimization can be implemented through React.memo and useMemo. 3) The basic usage is to create and render components, and the advanced usage includes using Hooks and ContextAPI. 4) Common errors such as improper status update, you can use ReactDevTools to debug. 5) Performance optimization includes using React.memo, virtualization lists and CodeSplitting, and keeping code readable and maintainable is best practice.

React combines JSX and HTML to improve user experience. 1) JSX embeds HTML to make development more intuitive. 2) The virtual DOM mechanism optimizes performance and reduces DOM operations. 3) Component-based management UI to improve maintainability. 4) State management and event processing enhance interactivity.

React components can be defined by functions or classes, encapsulating UI logic and accepting input data through props. 1) Define components: Use functions or classes to return React elements. 2) Rendering component: React calls render method or executes function component. 3) Multiplexing components: pass data through props to build a complex UI. The lifecycle approach of components allows logic to be executed at different stages, improving development efficiency and code maintainability.
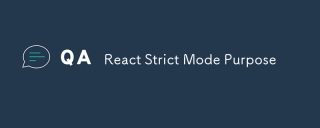
React Strict Mode is a development tool that highlights potential issues in React applications by activating additional checks and warnings. It helps identify legacy code, unsafe lifecycles, and side effects, encouraging modern React practices.
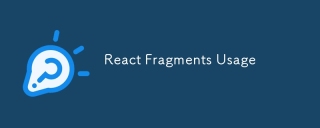
React Fragments allow grouping children without extra DOM nodes, enhancing structure, performance, and accessibility. They support keys for efficient list rendering.
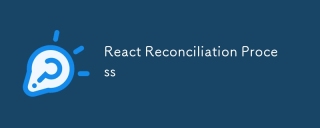
The article discusses React's reconciliation process, detailing how it efficiently updates the DOM. Key steps include triggering reconciliation, creating a Virtual DOM, using a diffing algorithm, and applying minimal DOM updates. It also covers perfo
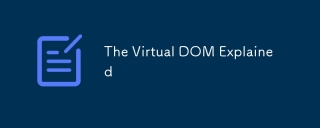
The article discusses the Virtual DOM, a key concept in web development that enhances performance by minimizing direct DOM manipulation and optimizing updates.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
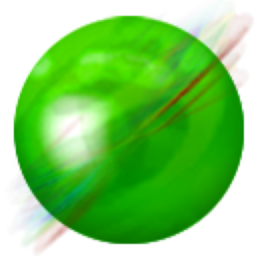
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Atom editor mac version download
The most popular open source editor

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
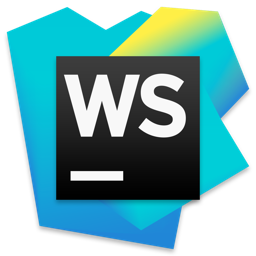
WebStorm Mac version
Useful JavaScript development tools