


What are some common architectural patterns used with Go (e.g., Clean Architecture)?
When developing software with Go, several architectural patterns are commonly employed to structure applications effectively. Here are some of the most prevalent ones:
-
Clean Architecture:
Clean Architecture, also known as the Onion Architecture or Hexagonal Architecture, is a design pattern that emphasizes separation of concerns and dependency inversion. It aims to create a system where the business logic is at the center, surrounded by layers of interfaces and adapters that interact with the outside world. This pattern is particularly popular in Go due to its simplicity and the language's support for interfaces. -
Microservices Architecture:
Go is well-suited for microservices due to its lightweight nature and efficient concurrency model. In this pattern, an application is broken down into smaller, independent services that communicate over a network. Each service can be developed, deployed, and scaled independently. -
Layered Architecture:
This traditional approach divides the application into layers such as presentation, business logic, and data access. While simpler than Clean Architecture, it can still be effective for smaller projects or when transitioning from other languages. -
Event-Driven Architecture:
Go's support for goroutines and channels makes it an excellent choice for event-driven systems. In this pattern, components communicate through events, allowing for loose coupling and scalability. -
Service-Oriented Architecture (SOA):
Similar to microservices but often with larger services, SOA focuses on providing services that can be reused across different parts of an organization. Go's performance and ease of deployment make it a good fit for this pattern.
Each of these patterns has its strengths and is chosen based on the specific needs of the project, such as scalability, maintainability, and the team's familiarity with the pattern.
What are the benefits of using Clean Architecture with Go for software development?
Using Clean Architecture with Go offers several significant benefits for software development:
-
Separation of Concerns:
Clean Architecture enforces a clear separation between the business logic and the infrastructure. This separation makes the code more modular and easier to maintain, as changes in one layer do not necessarily affect others. -
Testability:
By isolating the business logic from external dependencies, Clean Architecture makes it easier to write unit tests. The core logic can be tested independently of databases, web frameworks, or other external systems. -
Flexibility and Adaptability:
The use of interfaces and dependency inversion allows for easy swapping of different implementations. For example, you can switch from one database to another without changing the core business logic. -
Scalability:
Clean Architecture helps in scaling the application both in terms of code and infrastructure. As the project grows, new features can be added without disrupting existing functionality. -
Reusability:
The business logic at the center of the architecture can be reused across different applications or services, promoting code reuse and reducing duplication. -
Go's Language Features:
Go's support for interfaces and its simplicity make it an ideal language for implementing Clean Architecture. The language's built-in features align well with the principles of Clean Architecture, making it easier to follow the pattern.
How can one implement Clean Architecture in a Go project effectively?
Implementing Clean Architecture in a Go project involves several steps and considerations. Here's a step-by-step guide to effectively implement it:
-
Define the Core Domain:
Start by identifying the core business logic of your application. This should be independent of any external systems and should be placed at the center of your architecture. -
Create Interfaces for Dependencies:
Define interfaces for any external dependencies such as databases, web frameworks, or third-party services. These interfaces should be part of the core domain and will be implemented by the outer layers. -
Implement the Use Cases:
Develop use cases that encapsulate the business logic. These use cases should interact with the core domain and the defined interfaces. -
Create the Outer Layers:
Implement the outer layers that will interact with the outside world. These layers should implement the interfaces defined in the core domain. For example, you might have a database layer that implements the database interface. -
Dependency Injection:
Use dependency injection to wire the different layers together. This can be done manually or with the help of a dependency injection framework. Go's interfaces make this process straightforward. -
Testing:
Write unit tests for the core domain and use cases. Since these are independent of external systems, they should be easy to test. Integration tests can be written to ensure the outer layers work correctly with the core. -
Organize the Code:
Structure your project in a way that reflects the Clean Architecture. A common approach is to have separate packages for the core domain, use cases, and the outer layers.
Here's a simple example of how the directory structure might look:
<code>project/ ├── cmd/ │ └── main.go ├── internal/ │ ├── core/ │ │ ├── domain/ │ │ │ └── entity.go │ │ └── usecase/ │ │ └── usecase.go │ ├── adapter/ │ │ ├── database/ │ │ │ └── database.go │ │ └── web/ │ │ └── web.go │ └── port/ │ └── port.go └── go.mod</code>
Are there any notable case studies or examples of Go projects using Clean Architecture?
Yes, there are several notable case studies and examples of Go projects that have successfully implemented Clean Architecture. Here are a few:
-
Golang Clean Architecture Example by Bxcodec:
This is a well-documented example of a Go project using Clean Architecture. It includes a simple REST API for managing books and demonstrates how to structure a project following Clean Architecture principles. The repository can be found on GitHub at [github.com/bxcodec/go-clean-arch](https://github.com/bxcodec/go-clean-arch). -
Go Clean Architecture Boilerplate by Thangchung:
This project provides a boilerplate for starting a new Go project with Clean Architecture. It includes examples of how to set up the core domain, use cases, and adapters. The repository is available at [github.com/thangchung/go-coffeeshop](https://github.com/thangchung/go-coffeeshop). -
Clean Architecture with Go by GolangCafe:
GolangCafe has a series of articles and a GitHub repository that explain how to implement Clean Architecture in Go. The project includes a simple todo list application and is a great resource for learning. The repository can be found at [github.com/golangcafe/clean-architecture](https://github.com/golangcafe/clean-architecture). -
Go Clean Architecture by Evrone:
Evrone has developed a project that showcases Clean Architecture in Go. It includes a simple CRUD application and demonstrates how to structure the code according to Clean Architecture principles. The repository is available at [github.com/evrone/go-clean-template](https://github.com/evrone/go-clean-template).
These examples provide valuable insights and practical implementations of Clean Architecture in Go, helping developers understand how to apply the pattern in their own projects.
The above is the detailed content of What are some common architectural patterns used with Go (e.g., Clean Architecture)?. For more information, please follow other related articles on the PHP Chinese website!
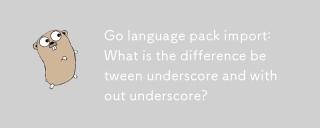
This article explains Go's package import mechanisms: named imports (e.g., import "fmt") and blank imports (e.g., import _ "fmt"). Named imports make package contents accessible, while blank imports only execute t
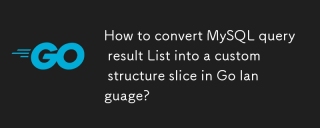
This article details efficient conversion of MySQL query results into Go struct slices. It emphasizes using database/sql's Scan method for optimal performance, avoiding manual parsing. Best practices for struct field mapping using db tags and robus

This article explains Beego's NewFlash() function for inter-page data transfer in web applications. It focuses on using NewFlash() to display temporary messages (success, error, warning) between controllers, leveraging the session mechanism. Limita
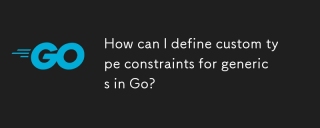
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
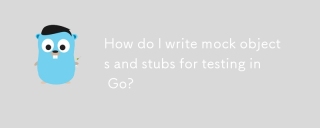
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl

This article details efficient file writing in Go, comparing os.WriteFile (suitable for small files) with os.OpenFile and buffered writes (optimal for large files). It emphasizes robust error handling, using defer, and checking for specific errors.
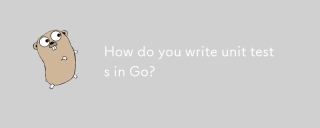
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
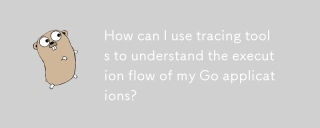
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
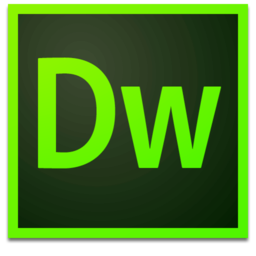
Dreamweaver Mac version
Visual web development tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

Zend Studio 13.0.1
Powerful PHP integrated development environment

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

SublimeText3 English version
Recommended: Win version, supports code prompts!
