Explain the Observer pattern and how it could be implemented in Go.
The Observer pattern is a behavioral design pattern that defines a one-to-many dependency between objects so that when one object changes state, all its dependents are notified and updated automatically. It's commonly used in scenarios where you need to maintain consistency between related objects without making them tightly coupled.
In Go, the Observer pattern can be implemented using interfaces and channels. Here's a step-by-step approach to implementing the Observer pattern in Go:
-
Define the Subject Interface: This interface will define methods for attaching, detaching, and notifying observers.
type Subject interface { Attach(Observer) Detach(Observer) Notify() }
-
Define the Observer Interface: This interface will define the method that will be called when the subject's state changes.
type Observer interface { Update(state string) }
-
Implement the Concrete Subject: This is the actual object that will be observed. It will maintain a list of observers and implement the
Subject
interface.type ConcreteSubject struct { state string observers []Observer } func (s *ConcreteSubject) Attach(o Observer) { s.observers = append(s.observers, o) } func (s *ConcreteSubject) Detach(o Observer) { for i, observer := range s.observers { if observer == o { s.observers = append(s.observers[:i], s.observers[i 1:]...) break } } } func (s *ConcreteSubject) Notify() { for _, observer := range s.observers { observer.Update(s.state) } } func (s *ConcreteSubject) SetState(state string) { s.state = state s.Notify() }
-
Implement the Concrete Observer: This is the object that will be notified when the subject's state changes.
type ConcreteObserver struct { name string } func (o *ConcreteObserver) Update(state string) { fmt.Printf("%s received update: %s\n", o.name, state) }
-
Usage Example: Here's how you might use the above implementation.
func main() { subject := &ConcreteSubject{} observer1 := &ConcreteObserver{name: "Observer1"} observer2 := &ConcreteObserver{name: "Observer2"} subject.Attach(observer1) subject.Attach(observer2) subject.SetState("New State") subject.Detach(observer2) subject.SetState("Another State") }
This implementation allows the subject to notify multiple observers when its state changes, and observers can be dynamically added or removed.
What are the key components of the Observer pattern in Go?
The key components of the Observer pattern in Go are:
-
Subject: The object being observed. It maintains a list of observers and provides methods to attach, detach, and notify observers. In the example above,
Subject
is an interface, andConcreteSubject
is its implementation. -
Observer: The object that observes the subject. It defines an interface with a method that will be called when the subject's state changes. In the example,
Observer
is an interface, andConcreteObserver
is its implementation. -
Attach and Detach Methods: These methods allow observers to be added to or removed from the subject's list of observers. In the example, these are part of the
Subject
interface and implemented inConcreteSubject
. -
Notify Method: This method is called by the subject to notify all its observers of a state change. In the example, this is part of the
Subject
interface and implemented inConcreteSubject
. -
Update Method: This method is called on each observer when the subject's state changes. In the example, this is part of the
Observer
interface and implemented inConcreteObserver
.
How can you effectively manage multiple observers in a Go application?
Managing multiple observers in a Go application can be done effectively by following these strategies:
-
Use Slices for Observers: Store observers in a slice within the subject. This allows for easy addition and removal of observers.
type ConcreteSubject struct { state string observers []Observer }
-
Concurrency Safety: If your application is concurrent, ensure that operations on the observers slice are thread-safe. You can use mutexes to protect the slice.
type ConcreteSubject struct { state string observers []Observer mutex sync.Mutex } func (s *ConcreteSubject) Attach(o Observer) { s.mutex.Lock() defer s.mutex.Unlock() s.observers = append(s.observers, o) } func (s *ConcreteSubject) Detach(o Observer) { s.mutex.Lock() defer s.mutex.Unlock() for i, observer := range s.observers { if observer == o { s.observers = append(s.observers[:i], s.observers[i 1:]...) break } } }
-
Efficient Notification: When notifying observers, consider using goroutines to notify them concurrently, which can improve performance for a large number of observers.
func (s *ConcreteSubject) Notify() { for _, observer := range s.observers { go observer.Update(s.state) } }
- Observer Prioritization: If some observers need to be notified before others, you can maintain multiple slices or use a priority queue to manage the order of notifications.
-
Error Handling: Implement error handling in the
Update
method of observers to handle any issues that might occur during notification.
What are some common pitfalls to avoid when implementing the Observer pattern in Go?
When implementing the Observer pattern in Go, there are several common pitfalls to avoid:
- Memory Leaks: If observers are not properly detached, they can cause memory leaks. Always ensure that observers are detached when they are no longer needed.
- Concurrency Issues: Without proper synchronization, concurrent access to the observers slice can lead to race conditions. Use mutexes or other synchronization primitives to protect shared resources.
- Performance Overhead: Notifying a large number of observers can be slow. Consider using goroutines for concurrent notification or implementing a batch notification system to improve performance.
- Tight Coupling: While the Observer pattern aims to reduce coupling, it can still lead to tight coupling if the subject and observers are too closely tied to each other's implementation details. Use interfaces to maintain loose coupling.
- Order of Notification: If the order of notification matters, ensure that the notification mechanism respects this order. Using a priority queue or multiple slices can help manage this.
-
Error Handling: Failing to handle errors during the notification process can lead to silent failures. Implement proper error handling in the
Update
method of observers. - Over-Notification: Notifying observers too frequently can lead to performance issues. Consider implementing a debounce mechanism to reduce the frequency of notifications.
By being aware of these pitfalls and implementing the Observer pattern carefully, you can create a robust and efficient system in Go.
The above is the detailed content of Explain the Observer pattern and how it could be implemented in Go.. For more information, please follow other related articles on the PHP Chinese website!
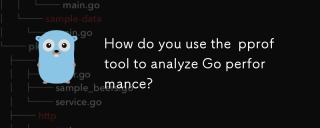
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
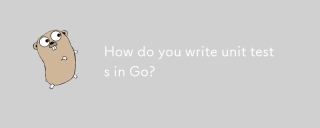
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
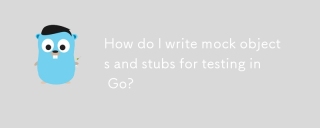
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
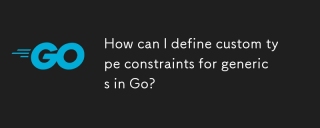
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
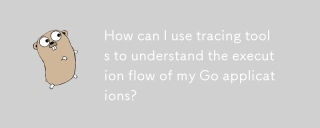
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization
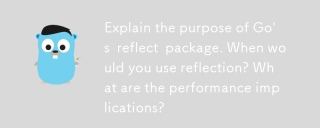
The article discusses Go's reflect package, used for runtime manipulation of code, beneficial for serialization, generic programming, and more. It warns of performance costs like slower execution and higher memory use, advising judicious use and best
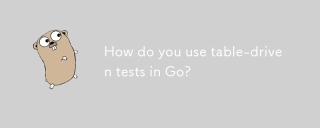
The article discusses using table-driven tests in Go, a method that uses a table of test cases to test functions with multiple inputs and outcomes. It highlights benefits like improved readability, reduced duplication, scalability, consistency, and a
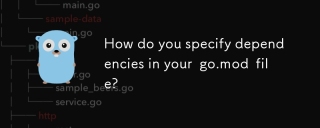
The article discusses managing Go module dependencies via go.mod, covering specification, updates, and conflict resolution. It emphasizes best practices like semantic versioning and regular updates.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
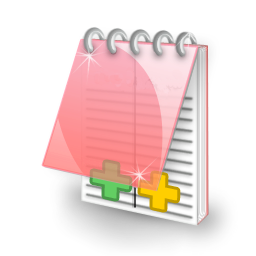
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SublimeText3 Linux new version
SublimeText3 Linux latest version
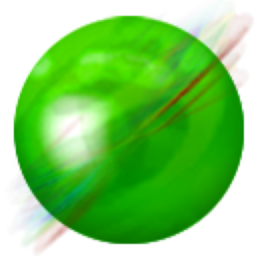
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 English version
Recommended: Win version, supports code prompts!
