Implement the Singleton pattern in Go safely with concurrency in mind
To implement the Singleton pattern in Go safely with concurrency in mind, we need to ensure that only one instance of the class is created, even in a multi-threaded environment. The main challenge is to make sure that multiple goroutines (Go's lightweight threads) do not create multiple instances due to race conditions.
The key to implementing a thread-safe Singleton in Go is to use synchronization mechanisms such as mutexes or atomic operations. Here's a basic outline of how to achieve this:
- Define the Singleton structure: Create a structure that represents the Singleton.
-
Initialize the Singleton instance: Use a pointer to this structure, initially set to
nil
. - Use a mutex: Implement a mutex to ensure that only one goroutine can initialize the Singleton at a time.
- Double-checked locking: Implement a check to see if the instance already exists before locking the mutex. This helps to reduce the frequency of mutex locking.
How can I ensure thread safety when using the Singleton pattern in Go?
Ensuring thread safety in Go for the Singleton pattern involves preventing multiple instances of the Singleton from being created when multiple goroutines are accessing the initialization method concurrently. The following steps help in achieving this:
- Use of Mutex: A mutex (short for mutual exclusion) can be used to ensure that only one goroutine can access the critical section (where the Singleton is created) at any time. This prevents multiple goroutines from initializing the Singleton simultaneously.
- Double-Checked Locking: Before locking the mutex, check if the Singleton instance has already been created. If it hasn't, then lock the mutex, check again (to ensure another goroutine didn't create it while this one was waiting to acquire the lock), and then create the instance if necessary. This pattern is called double-checked locking and helps to minimize the use of mutex locks.
-
Use of Atomic Operations: Go provides the
sync/atomic
package, which can be used to implement thread-safe operations without using mutexes. For instance, atomic operations can be used to ensure that the check and set operations for the Singleton instance are done atomically.
What are the best practices for implementing the Singleton pattern in a concurrent environment in Go?
Implementing the Singleton pattern in a concurrent environment in Go should adhere to the following best practices:
- Minimize Mutex Usage: Use double-checked locking to minimize the amount of time the mutex is held. This increases performance by reducing contention.
-
Use Atomic Operations: When applicable, use atomic operations from the
sync/atomic
package to perform thread-safe operations, which can be more efficient than using mutexes. - Lazy Initialization: Initialize the Singleton instance only when it's first needed, rather than at program start. This improves startup time and can help with dependency management.
- Avoid Global State: While the Singleton pattern inherently uses global state, try to minimize its usage elsewhere in your code. This helps in maintaining a clean and modular code structure.
- Testing: Ensure that your Singleton implementation is thoroughly tested, especially in concurrent scenarios, to catch any race conditions.
- Documentation: Clearly document the use of the Singleton pattern in your code to help other developers understand its implications and use.
Can you provide a code example of a safe Singleton implementation in Go that handles multiple goroutines?
Below is a thread-safe Singleton implementation in Go that uses double-checked locking with a mutex:
package main import ( "fmt" "sync" ) // Singleton represents the singleton instance type Singleton struct { data string } var ( instance *Singleton once sync.Once ) // GetInstance returns the singleton instance func GetInstance() *Singleton { once.Do(func() { instance = &Singleton{data: "Initialized"} }) return instance } func main() { // Simulating multiple goroutines accessing the Singleton for i := 0; i < 10; i { go func(num int) { singleton := GetInstance() fmt.Printf("Goroutine %d: Singleton data: %s\n", num, singleton.data) }(i) } // Wait for goroutines to finish (in a real application, you'd use a WaitGroup) select {} }
This example uses sync.Once
to ensure that the initialization function is called only once, even if GetInstance
is called multiple times concurrently. The sync.Once
type internally uses a mutex and is optimized for this use case, making it an efficient choice for implementing a thread-safe Singleton in Go.
The above is the detailed content of Implement the Singleton pattern in Go safely with concurrency in mind.. For more information, please follow other related articles on the PHP Chinese website!
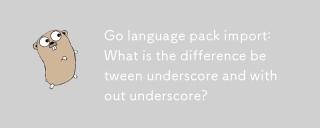
This article explains Go's package import mechanisms: named imports (e.g., import "fmt") and blank imports (e.g., import _ "fmt"). Named imports make package contents accessible, while blank imports only execute t
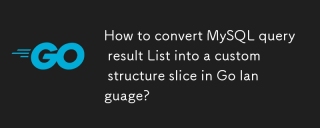
This article details efficient conversion of MySQL query results into Go struct slices. It emphasizes using database/sql's Scan method for optimal performance, avoiding manual parsing. Best practices for struct field mapping using db tags and robus

This article explains Beego's NewFlash() function for inter-page data transfer in web applications. It focuses on using NewFlash() to display temporary messages (success, error, warning) between controllers, leveraging the session mechanism. Limita
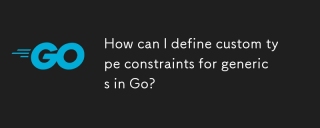
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
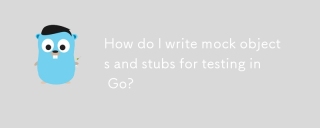
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl

This article details efficient file writing in Go, comparing os.WriteFile (suitable for small files) with os.OpenFile and buffered writes (optimal for large files). It emphasizes robust error handling, using defer, and checking for specific errors.
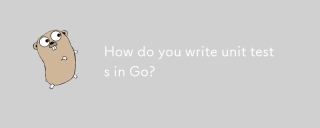
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
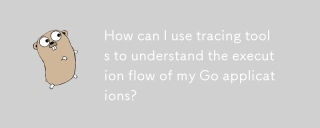
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
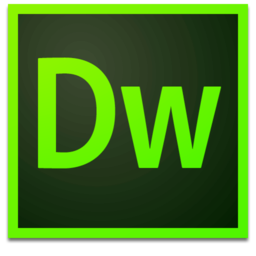
Dreamweaver Mac version
Visual web development tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

Zend Studio 13.0.1
Powerful PHP integrated development environment

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

SublimeText3 English version
Recommended: Win version, supports code prompts!
