API Error Handling Best Practices: Format and status codes
Effective API error handling is crucial for maintaining a robust and user-friendly application. It involves not only the proper use of HTTP status codes but also the clear and consistent formatting of error messages. Let's delve into the best practices for API error handling, focusing on format and status codes.
What are the most effective formats for presenting error messages in API responses?
When it comes to presenting error messages in API responses, the format should be clear, consistent, and informative. Here are some best practices for formatting error messages:
-
JSON Format: JSON is widely used due to its readability and ease of parsing. A typical JSON error response might look like this:
{ "error": { "code": "400", "message": "Invalid request parameters", "details": "The 'username' field is required." } }
This format includes an error object with a code, a human-readable message, and additional details that can help developers understand and fix the issue.
-
Standardized Fields: Use standardized fields such as
code
,message
, anddetails
to ensure consistency across different error responses. This helps developers quickly identify and handle errors. -
Localization: Consider including a
language
field to support multiple languages, allowing for more user-friendly error messages:{ "error": { "code": "400", "message": "Invalid request parameters", "details": "The 'username' field is required.", "language": "en" } }
-
Error Types: Categorize errors into types such as
validation
,authentication
,authorization
, andserver
to provide more context:{ "error": { "code": "401", "type": "authentication", "message": "Unauthorized access", "details": "Invalid credentials provided." } }
-
Timestamps: Including a timestamp can be helpful for logging and debugging purposes:
{ "error": { "code": "500", "message": "Internal server error", "details": "An unexpected error occurred.", "timestamp": "2023-10-01T12:34:56Z" } }
By following these guidelines, you can ensure that your API error messages are clear, consistent, and helpful to developers.
How should different HTTP status codes be utilized to indicate specific error types in APIs?
HTTP status codes are essential for indicating the outcome of an API request. Here’s how different status codes should be used to indicate specific error types:
-
4xx Client Error Codes:
- 400 Bad Request: Used when the server cannot process the request due to client error, such as malformed request syntax or invalid request message framing.
- 401 Unauthorized: Indicates that the request has not been applied because it lacks valid authentication credentials for the target resource.
- 403 Forbidden: The server understood the request but refuses to authorize it.
- 404 Not Found: The server can't find the requested resource.
- 405 Method Not Allowed: Specifies that the method received in the request-line is known by the origin server but not supported by the target resource.
- 409 Conflict: Indicates that the request could not be processed because of conflict in the request, such as an edit conflict in the target resource.
- 422 Unprocessable Entity: Used when the server understands the content type of the request entity, and the syntax of the request entity is correct, but it was unable to process the contained instructions.
-
5xx Server Error Codes:
- 500 Internal Server Error: A generic error message, given when an unexpected condition was encountered and no more specific message is suitable.
- 502 Bad Gateway: The server, while acting as a gateway or proxy, received an invalid response from the upstream server it accessed in attempting to fulfill the request.
- 503 Service Unavailable: The server is currently unable to handle the request due to a temporary overload or scheduled maintenance.
- 504 Gateway Timeout: The server, while acting as a gateway or proxy, did not receive a timely response from an upstream server it needed to access in order to complete the request.
By using these status codes appropriately, you can provide clear indications of what went wrong, helping developers to diagnose and resolve issues more efficiently.
What strategies can be implemented to ensure consistent error handling across various API endpoints?
Ensuring consistent error handling across various API endpoints is crucial for maintaining a reliable and user-friendly API. Here are some strategies to achieve this:
- Centralized Error Handling: Implement a centralized error handling mechanism that can be used across all endpoints. This can be achieved by creating a middleware or a utility function that formats and returns error responses consistently.
-
Error Handling Middleware: Use middleware to catch and handle errors uniformly. For example, in Node.js with Express, you can create an error handling middleware:
app.use((err, req, res, next) => { const statusCode = err.statusCode || 500; res.status(statusCode).json({ error: { code: statusCode.toString(), message: err.message || 'An error occurred', details: err.details || '' } }); });
-
Error Classes: Define custom error classes for different types of errors. This helps in categorizing errors and ensures that each error type is handled consistently:
class ValidationError extends Error { constructor(message, details) { super(message); this.name = 'ValidationError'; this.details = details; this.statusCode = 400; } }
- Documentation: Maintain comprehensive documentation that outlines the error handling strategy, including the format of error responses and the meaning of different status codes. This helps developers understand how to handle errors in their applications.
- Testing: Implement thorough testing to ensure that error handling is consistent across all endpoints. Use automated tests to check that errors are returned in the expected format and with the correct status codes.
- Logging: Implement a robust logging system to track errors. This can help in identifying inconsistencies in error handling and in debugging issues.
- Code Reviews: Regularly conduct code reviews to ensure that all developers are following the established error handling practices. This helps in maintaining consistency and catching any deviations early.
By implementing these strategies, you can ensure that your API provides a consistent and reliable error handling experience across all endpoints.
The above is the detailed content of API Error Handling Best Practices: Format and status codes.. For more information, please follow other related articles on the PHP Chinese website!
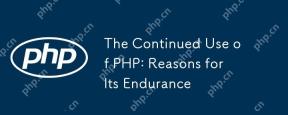
What’s still popular is the ease of use, flexibility and a strong ecosystem. 1) Ease of use and simple syntax make it the first choice for beginners. 2) Closely integrated with web development, excellent interaction with HTTP requests and database. 3) The huge ecosystem provides a wealth of tools and libraries. 4) Active community and open source nature adapts them to new needs and technology trends.
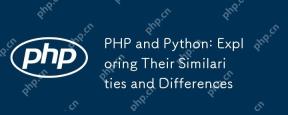
PHP and Python are both high-level programming languages that are widely used in web development, data processing and automation tasks. 1.PHP is often used to build dynamic websites and content management systems, while Python is often used to build web frameworks and data science. 2.PHP uses echo to output content, Python uses print. 3. Both support object-oriented programming, but the syntax and keywords are different. 4. PHP supports weak type conversion, while Python is more stringent. 5. PHP performance optimization includes using OPcache and asynchronous programming, while Python uses cProfile and asynchronous programming.
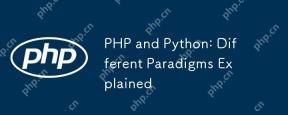
PHP is mainly procedural programming, but also supports object-oriented programming (OOP); Python supports a variety of paradigms, including OOP, functional and procedural programming. PHP is suitable for web development, and Python is suitable for a variety of applications such as data analysis and machine learning.
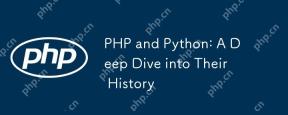
PHP originated in 1994 and was developed by RasmusLerdorf. It was originally used to track website visitors and gradually evolved into a server-side scripting language and was widely used in web development. Python was developed by Guidovan Rossum in the late 1980s and was first released in 1991. It emphasizes code readability and simplicity, and is suitable for scientific computing, data analysis and other fields.
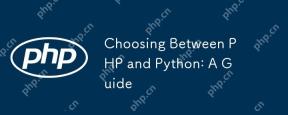
PHP is suitable for web development and rapid prototyping, and Python is suitable for data science and machine learning. 1.PHP is used for dynamic web development, with simple syntax and suitable for rapid development. 2. Python has concise syntax, is suitable for multiple fields, and has a strong library ecosystem.
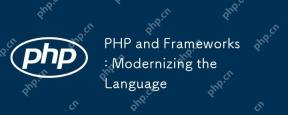
PHP remains important in the modernization process because it supports a large number of websites and applications and adapts to development needs through frameworks. 1.PHP7 improves performance and introduces new features. 2. Modern frameworks such as Laravel, Symfony and CodeIgniter simplify development and improve code quality. 3. Performance optimization and best practices further improve application efficiency.
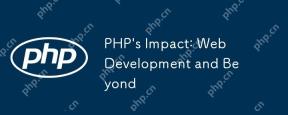
PHPhassignificantlyimpactedwebdevelopmentandextendsbeyondit.1)ItpowersmajorplatformslikeWordPressandexcelsindatabaseinteractions.2)PHP'sadaptabilityallowsittoscaleforlargeapplicationsusingframeworkslikeLaravel.3)Beyondweb,PHPisusedincommand-linescrip
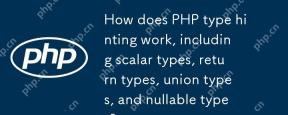
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type prompt: Since PHP8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
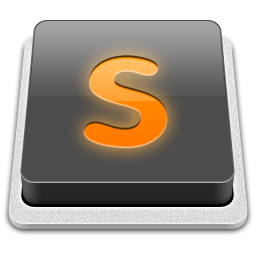
SublimeText3 Mac version
God-level code editing software (SublimeText3)

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

Atom editor mac version download
The most popular open source editor