


How can you use Go's escape analysis to understand where variables are allocated (stack vs. heap)?
Go's escape analysis is a crucial feature of the Go compiler that helps determine whether a variable should be allocated on the stack or the heap. Understanding where variables are allocated can significantly impact the performance and memory usage of your Go programs. Here's how you can use escape analysis to gain insights into variable allocation:
-
Compile with
-gcflags='-m'
: To perform escape analysis, you need to compile your Go program with the-gcflags='-m'
flag. This flag instructs the compiler to output information about escape analysis. For example, you can run:<code>go build -gcflags='-m' your_program.go</code>
This will generate output that includes details about which variables escape to the heap and which remain on the stack.
-
Interpreting the Output: The output from the
-gcflags='-m'
flag will contain lines that start withescapes to heap
ordoes not escape
. For example:<code>./main.go:10:20: leaking param: v ./main.go:10:20: v escapes to heap</code>
This indicates that the variable
v
is allocated on the heap because it escapes the function's scope. -
Understanding Escape Reasons: The output will also provide reasons why a variable escapes. Common reasons include:
- The variable is returned from the function.
- The variable is passed to another function that might store it.
- The variable is used in a closure.
- The variable is too large to fit on the stack.
By analyzing this output, you can understand which variables are allocated on the heap and why, helping you make informed decisions about your code's structure and performance.
What tools can help visualize the results of Go's escape analysis for better understanding of memory allocation?
While Go's built-in escape analysis provides textual output, several tools can help visualize this data for a better understanding of memory allocation:
-
Go Escape Analysis Visualizer: This is a web-based tool that allows you to paste the output of
go build -gcflags='-m'
and generates a visual representation of the escape analysis results. It can help you quickly identify which variables escape and why. -
Go Heap Profiler: While not specifically designed for escape analysis, the Go heap profiler can be used in conjunction with escape analysis to understand heap allocations. You can use the
pprof
tool to visualize heap profiles, which can complement the escape analysis output. - Third-Party Tools: There are several third-party tools and plugins available for popular IDEs like Visual Studio Code and GoLand that can parse the escape analysis output and provide visual cues or annotations directly in your code editor. These tools can highlight variables that escape to the heap, making it easier to spot potential performance issues.
- Custom Scripts: You can also write custom scripts to parse the escape analysis output and generate visualizations using libraries like Graphviz or D3.js. This approach allows you to tailor the visualization to your specific needs.
Using these tools can help you quickly grasp the impact of escape analysis on your code and make it easier to optimize memory allocation.
How does understanding Go's escape analysis impact performance optimization in Go programming?
Understanding Go's escape analysis is crucial for performance optimization in Go programming for several reasons:
- Memory Efficiency: Variables allocated on the stack are generally more efficient than those on the heap because stack allocation is faster and stack memory is automatically reclaimed when the function returns. By understanding escape analysis, you can minimize heap allocations, leading to more efficient memory usage.
- Garbage Collection: Variables allocated on the heap are subject to garbage collection, which can introduce pauses in your program. By reducing the number of variables that escape to the heap, you can decrease the frequency and duration of garbage collection cycles, improving overall performance.
- Code Optimization: Escape analysis can guide you in restructuring your code to prevent unnecessary heap allocations. For example, if a function returns a large struct that escapes to the heap, you might consider passing a pointer to the struct instead, or breaking the struct into smaller components that can be allocated on the stack.
- Performance Profiling: By understanding which variables escape and why, you can focus your performance profiling efforts on the parts of your code that are most likely to impact performance. This targeted approach can lead to more effective optimizations.
- Design Decisions: Knowledge of escape analysis can influence design decisions, such as choosing between value types and reference types, or deciding whether to use interfaces or concrete types. These decisions can have significant performance implications.
In summary, understanding Go's escape analysis allows you to make informed decisions about memory allocation, leading to more efficient and performant Go programs.
Can you explain the key indicators in Go's escape analysis output that suggest a variable is allocated on the heap?
The output of Go's escape analysis, generated by compiling with -gcflags='-m'
, contains several key indicators that suggest a variable is allocated on the heap. Here are the main indicators to look for:
-
escapes to heap
: This is the most direct indicator. If you see a line likev escapes to heap
, it means the variablev
is allocated on the heap. For example:<code>./main.go:10:20: v escapes to heap</code>
-
leaking param
: This indicates that a parameter passed to a function escapes to the heap. For example:<code>./main.go:10:20: leaking param: v</code>
This often accompanies the
escapes to heap
message and suggests that the parameter is stored in a way that it outlives the function call. -
moved to heap
: This indicates that a variable was initially considered for stack allocation but was moved to the heap due to its size or other factors. For example:<code>./main.go:10:20: large struct moved to heap</code>
-
... escapes to heap
: Sometimes, the output will specify why a variable escapes, such as:<code>./main.go:10:20: v escapes to heap because it is returned from the function</code>
This provides additional context about the reason for the heap allocation.
-
... does not escape
: Conversely, if you see a message likev does not escape
, it means the variable is allocated on the stack and does not escape to the heap.
By paying attention to these indicators, you can quickly identify which variables are allocated on the heap and why, helping you optimize your Go code for better performance and memory efficiency.
The above is the detailed content of How can you use Go's escape analysis to understand where variables are allocated (stack vs. heap)?. For more information, please follow other related articles on the PHP Chinese website!
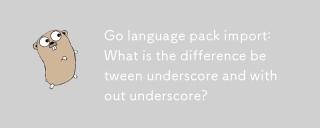
This article explains Go's package import mechanisms: named imports (e.g., import "fmt") and blank imports (e.g., import _ "fmt"). Named imports make package contents accessible, while blank imports only execute t

This article explains Beego's NewFlash() function for inter-page data transfer in web applications. It focuses on using NewFlash() to display temporary messages (success, error, warning) between controllers, leveraging the session mechanism. Limita
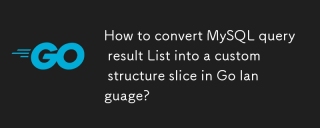
This article details efficient conversion of MySQL query results into Go struct slices. It emphasizes using database/sql's Scan method for optimal performance, avoiding manual parsing. Best practices for struct field mapping using db tags and robus
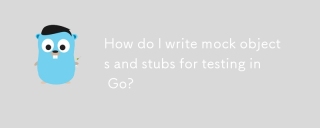
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
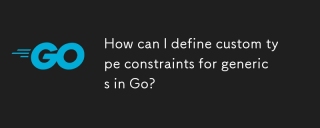
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices

This article details efficient file writing in Go, comparing os.WriteFile (suitable for small files) with os.OpenFile and buffered writes (optimal for large files). It emphasizes robust error handling, using defer, and checking for specific errors.
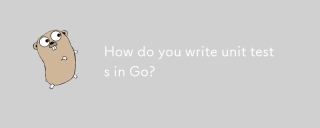
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
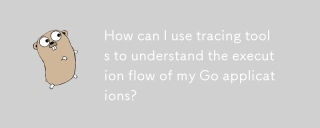
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SublimeText3 English version
Recommended: Win version, supports code prompts!
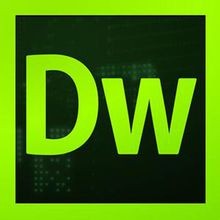
Dreamweaver CS6
Visual web development tools
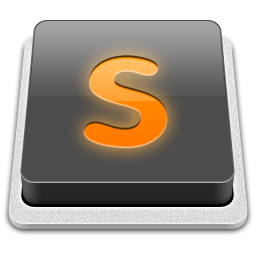
SublimeText3 Mac version
God-level code editing software (SublimeText3)
