How can you use v-once directive to prevent unnecessary updates?
The v-once
directive in Vue.js is a powerful tool for optimizing the performance of your application by preventing unnecessary updates to parts of your template. When you apply the v-once
directive to an element or component, Vue will render it only once and then treat it as static content. This means that any subsequent updates to the data that would normally cause the element to re-render will be ignored.
To use the v-once
directive, you simply add it as an attribute to the element you want to render only once. Here's an example:
<template> <div v-once> <p>{{ message }}</p> </div> </template> <script> export default { data() { return { message: 'Hello, Vue!' }; } }; </script>
In this example, the div
element and its contents will be rendered once with the initial value of message
. If message
is later updated, the content inside the div
will not change.
This directive is particularly useful for elements that contain static content or data that does not change frequently. By using v-once
, you can prevent Vue from performing unnecessary re-renders, which can improve the overall performance of your application.
What are the performance benefits of using the v-once directive in Vue.js?
Using the v-once
directive in Vue.js can offer several performance benefits:
-
Reduced Re-rendering: By marking elements or components with
v-once
, you ensure that they are rendered only once. This prevents Vue from re-rendering these elements when the data changes, which can significantly reduce the computational overhead of your application. -
Improved Memory Usage: Since elements marked with
v-once
are treated as static content, Vue does not need to keep track of their reactive dependencies. This can lead to lower memory usage, as Vue does not need to maintain a dependency graph for these elements. -
Faster DOM Updates: When you have a large number of elements that do not need to be updated frequently, using
v-once
can speed up DOM updates. By isolating static content, you allow Vue to focus on updating only the parts of the DOM that actually need to change. -
Enhanced Initial Load Time: Although
v-once
primarily affects runtime performance, it can also contribute to a faster initial load time by reducing the amount of work Vue needs to do during the initial render.
Overall, the v-once
directive is a simple yet effective way to optimize the performance of your Vue.js application by minimizing unnecessary updates and improving resource utilization.
Can the v-once directive be applied to improve the initial load time of a Vue.js application?
Yes, the v-once
directive can be applied to improve the initial load time of a Vue.js application, although its primary benefit is in reducing runtime re-renders. Here's how it can contribute to faster initial load times:
-
Reduced Initial Render Complexity: By marking certain elements or components with
v-once
, you can simplify the initial render process. Vue will treat these elements as static content, which means it does not need to set up reactive dependencies for them. This can lead to a faster initial render, especially in applications with complex templates. -
Faster Hydration in Server-Side Rendering (SSR): If you are using server-side rendering, applying
v-once
to static content can speed up the hydration process on the client side. During hydration, Vue needs to match the server-rendered DOM with the client-side application state. By marking static content withv-once
, you can reduce the amount of work Vue needs to do during hydration, leading to faster initial load times. - Optimized Resource Allocation: By ensuring that certain parts of your application are rendered only once, you can allocate more resources to other parts of your application that require dynamic updates. This can lead to a more efficient use of resources during the initial load, contributing to a faster overall load time.
While v-once
is not a silver bullet for improving initial load times, it can be a valuable tool in your optimization toolkit, especially when used in conjunction with other performance optimization techniques.
How does the v-once directive affect the re-rendering of components in Vue.js?
The v-once
directive has a significant impact on the re-rendering of components in Vue.js. Here's how it works:
-
Single Render: When a component or element is marked with
v-once
, Vue will render it only once during the initial render. After the initial render, Vue will treat the content as static and will not re-render it, even if the underlying data changes. -
No Reactive Updates: Elements or components with
v-once
are not part of Vue's reactive system. This means that any changes to the data that would normally trigger a re-render will be ignored for these elements. As a result, the DOM for these elements remains unchanged, which can significantly reduce the number of re-renders in your application. -
Static Content: By marking content as static with
v-once
, you can isolate parts of your application that do not need to be updated dynamically. This can be particularly useful for headers, footers, or other parts of your UI that remain constant throughout the user's interaction with your application. -
Performance Optimization: The primary benefit of using
v-once
is performance optimization. By preventing unnecessary re-renders, you can improve the responsiveness and efficiency of your application, especially in scenarios where you have large amounts of static content.
Here's an example of how v-once
can be used to prevent re-rendering of a component:
<template> <div> <header v-once> <h1 id="title">{{ title }}</h1> </header> <main> <p>{{ dynamicContent }}</p> </main> </div> </template> <script> export default { data() { return { title: 'Welcome to My App', dynamicContent: 'This content can change.' }; }, methods: { updateDynamicContent() { this.dynamicContent = 'The content has been updated.'; } } }; </script>
In this example, the header
element is marked with v-once
, so it will be rendered only once with the initial value of title
. If title
is later updated, the header
will not change. However, the main
section will re-render when dynamicContent
changes.
By strategically using the v-once
directive, you can control which parts of your application are re-rendered, leading to more efficient and performant Vue.js applications.
The above is the detailed content of How can you use v-once directive to prevent unnecessary updates?. For more information, please follow other related articles on the PHP Chinese website!
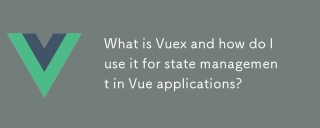
This article explains Vuex, a state management library for Vue.js. It details core concepts (state, getters, mutations, actions) and demonstrates usage, emphasizing its benefits for larger projects over simpler alternatives. Debugging and structuri
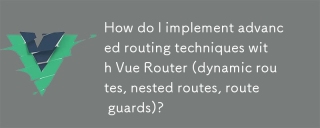
This article explores advanced Vue Router techniques. It covers dynamic routing (using parameters), nested routes for hierarchical navigation, and route guards for controlling access and data fetching. Best practices for managing complex route conf

Article discusses creating and using custom Vue.js plugins, including development, integration, and maintenance best practices.

Vue.js enhances web development with its Component-Based Architecture, Virtual DOM for performance, and Reactive Data Binding for real-time UI updates.

The article explains how to configure Vue CLI for different build targets, switch environments, optimize production builds, and ensure source maps in development for debugging.
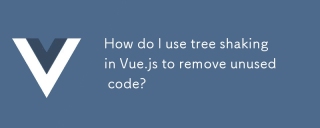
The article discusses using tree shaking in Vue.js to remove unused code, detailing setup with ES6 modules, Webpack configuration, and best practices for effective implementation.Character count: 159
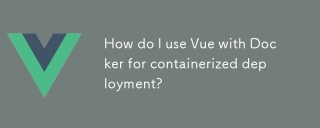
The article discusses using Vue with Docker for deployment, focusing on setup, optimization, management, and performance monitoring of Vue applications in containers.
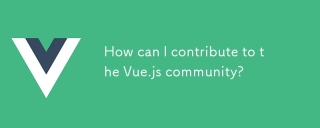
The article discusses various ways to contribute to the Vue.js community, including improving documentation, answering questions, coding, creating content, organizing events, and financial support. It also covers getting involved in open-source proje


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!
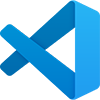
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
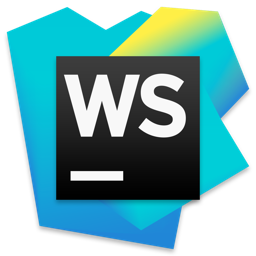
WebStorm Mac version
Useful JavaScript development tools
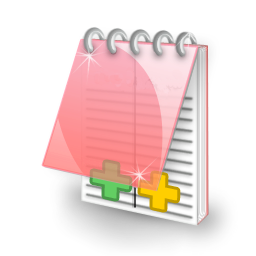
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
