How do I create and use custom plugins in Vue.js?
Creating and using custom plugins in Vue.js involves a few key steps that enable developers to extend the functionality of their Vue applications. Here’s a comprehensive guide on how to do it:
-
Define the Plugin: Start by defining a function that will serve as the plugin itself. This function will receive the Vue constructor as an argument, allowing you to modify it directly. Here’s an example of a basic plugin structure:
const MyPlugin = { install(Vue, options) { // Add global methods or properties Vue.myGlobalMethod = function () { // Logic for the method } // Add a global asset Vue.directive('my-directive', { bind(el, binding, vnode, oldVnode) { // Logic for the directive } }) // Inject some component options Vue.mixin({ created: function () { // Logic to be applied to all components } }) // Add an instance method Vue.prototype.$myMethod = function (methodOptions) { // Logic for the method } } }
-
Register the Plugin: Once your plugin is defined, you need to register it in your Vue application. This is typically done in the main file where you create the Vue instance. Here’s how you can do it:
import Vue from 'vue' import MyPlugin from './path-to-my-plugin' Vue.use(MyPlugin) new Vue({ // Your app options }).$mount('#app')
-
Using the Plugin: After registration, you can use the functionalities provided by your plugin throughout your application. Depending on what you’ve defined in the plugin, you might use global methods, directives, or instance methods. For instance, if you defined a global method:
Vue.myGlobalMethod()
Or if you added an instance method:
this.$myMethod(options)
By following these steps, you can successfully create and integrate custom plugins into your Vue.js application, enhancing its capabilities as needed.
What are the essential steps to develop a custom Vue.js plugin?
Developing a custom Vue.js plugin requires a structured approach to ensure that it integrates seamlessly into Vue applications. Here are the essential steps:
- Identify the Need: Before starting to code, clearly define what the plugin should do. Whether it's adding global methods, directives, or mixins, the purpose should be well-defined.
-
Set Up the Plugin Structure: Create a new JavaScript file for your plugin and define the plugin using the
install
method. This method receives the Vue constructor, allowing you to augment it:const MyPlugin = { install(Vue, options) { // Plugin code here } }
-
Implement Functionality: Add the necessary logic inside the
install
method. This can include:- Adding global methods or properties.
- Registering global directives.
- Injecting component options via mixins.
- Adding instance methods to
Vue.prototype
.
- Testing: Thoroughly test your plugin in isolation and within a Vue application to ensure it works as expected. Use unit tests if possible.
- Documentation: Write clear documentation explaining how to install and use your plugin. This should include any configuration options, usage examples, and potential caveats.
-
Export the Plugin: Export the plugin so it can be imported and used in Vue applications:
export default MyPlugin
Following these steps will help you develop a functional and well-documented Vue.js plugin.
How can I effectively integrate a custom plugin into an existing Vue.js application?
Integrating a custom plugin into an existing Vue.js application can be straightforward if done correctly. Here’s how to do it effectively:
-
Import the Plugin: First, ensure the plugin file is accessible in your project. Import it into your main application file, usually
main.js
:import Vue from 'vue' import MyPlugin from './path-to-my-plugin'
-
Register the Plugin: Use the
Vue.use()
method to install the plugin. This should be done before creating the Vue instance:Vue.use(MyPlugin, { /* Optional configuration options */ })
-
Create the Vue Instance: Proceed to create your Vue instance as usual. The plugin will be active from this point on:
new Vue({ // Your app options }).$mount('#app')
- Utilize the Plugin: Throughout your application, you can now use the features provided by your plugin. If it includes global methods, directives, or instance methods, these can be accessed as defined in your plugin.
- Testing and Validation: After integration, thoroughly test your application to ensure the plugin works as expected and doesn’t introduce any conflicts with existing code.
By following these steps, you can successfully integrate a custom plugin into your existing Vue.js application, enhancing its functionality without disrupting its operation.
What are some best practices for maintaining and updating custom Vue.js plugins?
Maintaining and updating custom Vue.js plugins is crucial to ensure their continued usefulness and compatibility with evolving frameworks and applications. Here are some best practices:
- Version Control: Use version control systems like Git to track changes in your plugin. Semantic versioning (e.g., 1.0.0) helps manage updates and compatibility.
- Regular Updates: Keep your plugin up-to-date with the latest Vue.js versions and best practices. Monitor Vue’s release notes and update your plugin to take advantage of new features and address deprecations.
- Testing: Maintain a suite of tests for your plugin. Automated tests (unit and integration tests) can help ensure that updates do not break existing functionality.
- Documentation: Update your documentation with each release to reflect new features, changes, and breaking changes. Good documentation is key to the usability of your plugin.
- Backward Compatibility: When making updates, consider the impact on existing users. Provide clear migration paths or deprecated warnings to help users transition to new versions.
- Community Engagement: If your plugin is publicly available, engage with the community for feedback and contributions. Consider opening a GitHub repository for issues and pull requests.
- Performance Optimization: Regularly profile your plugin to ensure it performs well. Optimize where necessary to minimize impact on application load times and runtime performance.
- Security Audits: Conduct security audits to identify and fix potential vulnerabilities, especially if your plugin interacts with external data or APIs.
By following these best practices, you can ensure that your custom Vue.js plugin remains reliable, secure, and beneficial to its users over time.
The above is the detailed content of How do I create and use custom plugins in Vue.js?. For more information, please follow other related articles on the PHP Chinese website!

The main disadvantages of Vue.js include: 1. The ecosystem is relatively new, and third-party libraries and tools are not as rich as other frameworks; 2. The learning curve becomes steep in complex functions; 3. Community support and resources are not as extensive as React and Angular; 4. Performance problems may be encountered in large applications; 5. Version upgrades and compatibility challenges are greater.

Netflix uses React as its front-end framework. 1.React's component development and virtual DOM mechanism improve performance and development efficiency. 2. Use Webpack and Babel to optimize code construction and deployment. 3. Use code segmentation, server-side rendering and caching strategies for performance optimization.

Reasons for Vue.js' popularity include simplicity and easy learning, flexibility and high performance. 1) Its progressive framework design is suitable for beginners to learn step by step. 2) Component-based development improves code maintainability and team collaboration efficiency. 3) Responsive systems and virtual DOM improve rendering performance.

Vue.js is easier to use and has a smooth learning curve, which is suitable for beginners; React has a steeper learning curve, but has strong flexibility, which is suitable for experienced developers. 1.Vue.js is easy to get started with through simple data binding and progressive design. 2.React requires understanding of virtual DOM and JSX, but provides higher flexibility and performance advantages.

Vue.js is suitable for fast development and small projects, while React is more suitable for large and complex projects. 1.Vue.js is simple and easy to learn, suitable for rapid development and small projects. 2.React is powerful and suitable for large and complex projects. 3. The progressive features of Vue.js are suitable for gradually introducing functions. 4. React's componentized and virtual DOM performs well when dealing with complex UI and data-intensive applications.

Vue.js and React each have their own advantages and disadvantages. When choosing, you need to comprehensively consider team skills, project size and performance requirements. 1) Vue.js is suitable for fast development and small projects, with a low learning curve, but deep nested objects can cause performance problems. 2) React is suitable for large and complex applications, with a rich ecosystem, but frequent updates may lead to performance bottlenecks.

Vue.js is suitable for small to medium-sized projects, while React is suitable for large projects and complex application scenarios. 1) Vue.js is easy to use and is suitable for rapid prototyping and small applications. 2) React has more advantages in handling complex state management and performance optimization, and is suitable for large projects.

Vue.js and React each have their own advantages: Vue.js is suitable for small applications and rapid development, while React is suitable for large applications and complex state management. 1.Vue.js realizes automatic update through a responsive system, suitable for small applications. 2.React uses virtual DOM and diff algorithms, which are suitable for large and complex applications. When selecting a framework, you need to consider project requirements and team technology stack.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
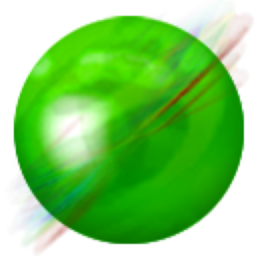
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
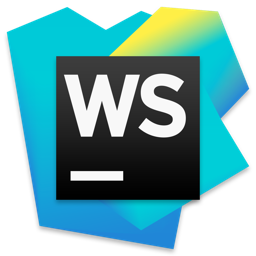
WebStorm Mac version
Useful JavaScript development tools
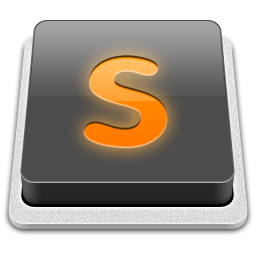
SublimeText3 Mac version
God-level code editing software (SublimeText3)
